Using the Sage 100 API to Create or Update Purchase Orders (with PHP examples)
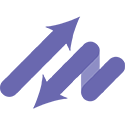
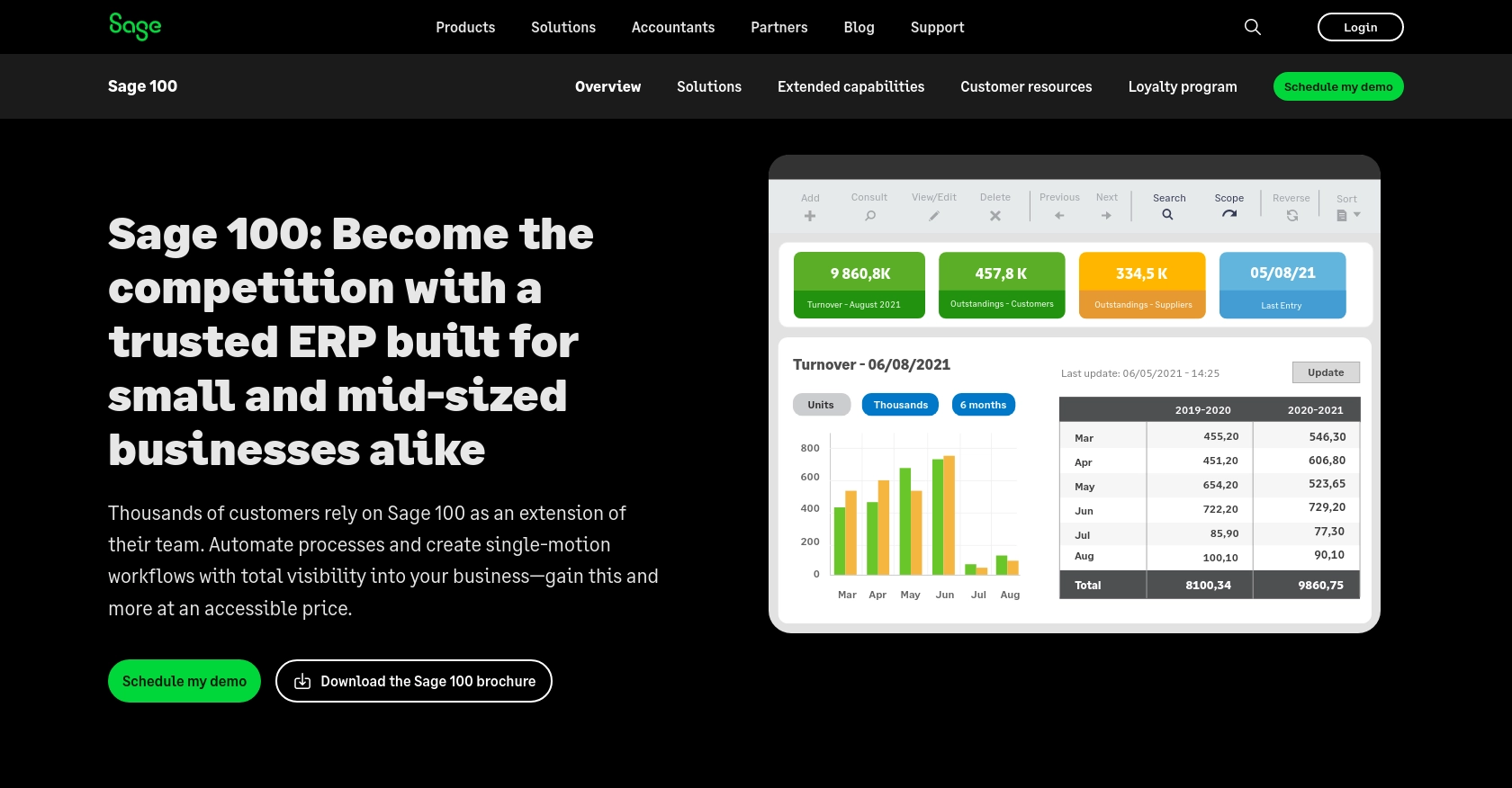
Introduction to Sage 100 API for Purchase Order Management
Sage 100 is a comprehensive enterprise resource planning (ERP) solution tailored for small to medium-sized businesses. It offers a robust suite of tools to manage accounting, inventory, and business operations efficiently. With its extensive capabilities, Sage 100 is a popular choice for businesses looking to streamline their processes and improve productivity.
For developers, integrating with the Sage 100 API can significantly enhance business operations by automating tasks such as creating or updating purchase orders. This integration allows businesses to maintain accurate and up-to-date records, reducing manual entry errors and saving valuable time.
In this article, we will explore how to use the Sage 100 API with PHP to create or update purchase orders. This integration can be particularly useful for businesses that need to manage a high volume of purchase orders efficiently, ensuring seamless operations and improved supply chain management.
Setting Up Your Sage 100 Test/Sandbox Account for API Integration
Before diving into the integration process with the Sage 100 API, it's essential to set up a test or sandbox account. This environment allows developers to safely experiment with API calls without affecting live data, ensuring a smooth integration process.
Installing and Configuring the Sage 100 ODBC Driver
To interact with the Sage 100 API, you need to install and configure the Sage 100 ODBC driver. Follow these steps to set up the driver:
- Access the ODBC Data Source Administrator on your system.
- Create a Data Source Name (DSN) that connects to the Sage 100 ERP system. Ensure you use the correct server, database, and authentication settings.
- Refer to the official Sage 100 documentation for detailed instructions on configuring the ODBC driver.
Creating a Sage 100 Test Account
To create a test account, follow these steps:
- Contact your Sage 100 representative to request access to a sandbox environment.
- Ensure that the test account has the necessary permissions to create and update purchase orders.
Configuring Sage 100 for API Access
Once your test account is set up, configure Sage 100 to allow API access:
- Log in to the Sage 100 interface with administrative privileges.
- Navigate to the Library Master module and select Setup > System Configuration.
- Enable the client/server ODBC driver by checking the appropriate box under the ODBC Driver tab.
- Enter the server name or IP address where the ODBC application or service is running.
- Test the ODBC data source to ensure successful connection.
Generating API Credentials for Sage 100
To authenticate API requests, you'll need to generate the necessary credentials:
- Access the Sage 100 administration panel and navigate to the API settings.
- Create a new API user and assign the required permissions for purchase order management.
- Securely store the generated client ID and client secret, as these will be used for authentication in your PHP code.
With your Sage 100 test account and API credentials ready, you can now proceed to make API calls to create or update purchase orders using PHP.
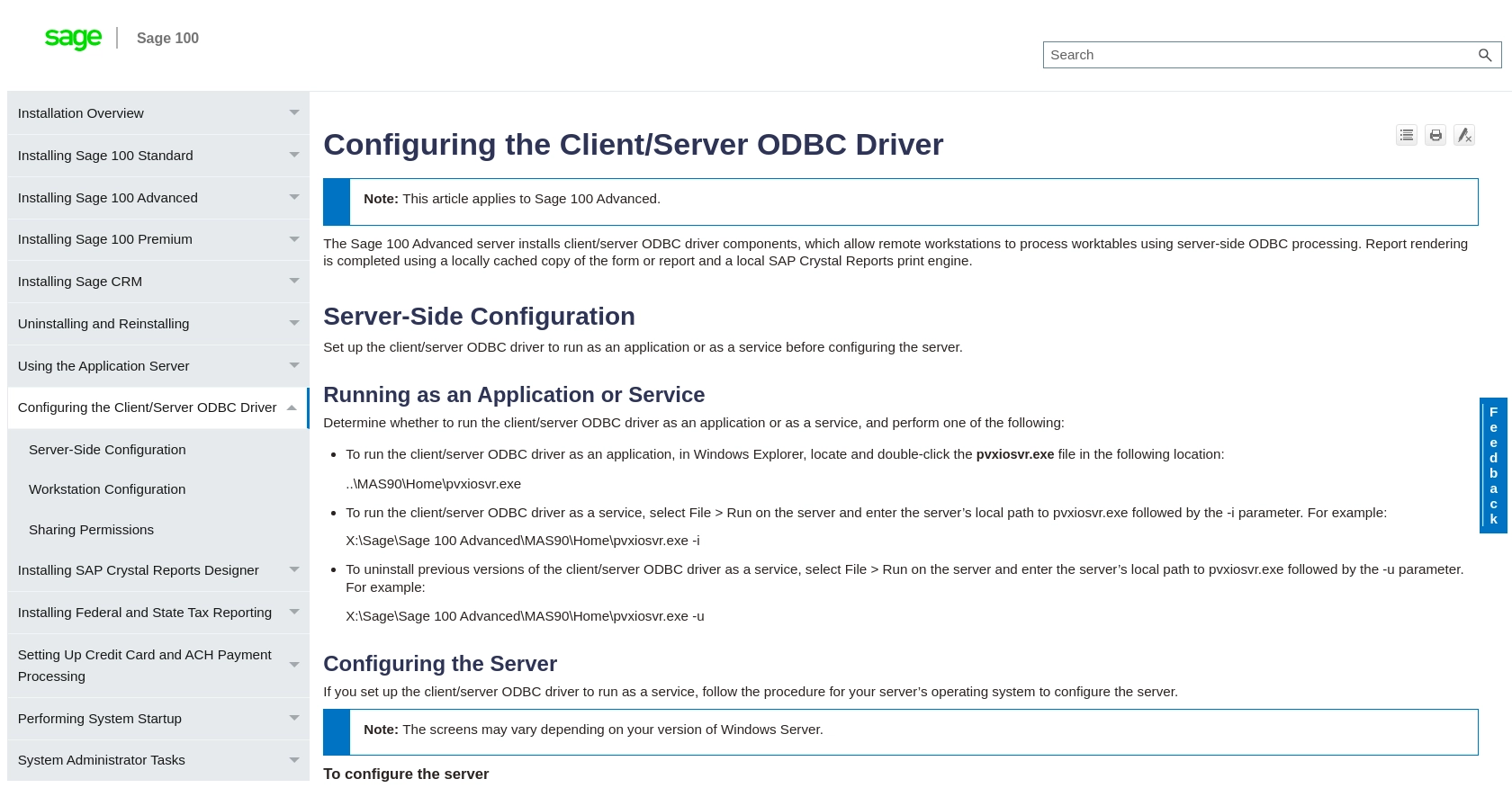
sbb-itb-96038d7
Making API Calls to Sage 100 for Purchase Order Management Using PHP
With your Sage 100 test account and API credentials set up, you can now proceed to make API calls to create or update purchase orders using PHP. This section will guide you through the process of setting up your PHP environment, writing the necessary code, and handling responses from the Sage 100 API.
Setting Up Your PHP Environment for Sage 100 API Integration
Before making API calls, ensure your PHP environment is correctly configured. Follow these steps:
- Ensure you have PHP 7.4 or later installed on your system.
- Install the necessary PHP extensions, such as
php-odbc
, to interact with the Sage 100 ODBC driver. - Use Composer to manage dependencies and include any required libraries for making HTTP requests, such as Guzzle.
Creating or Updating Purchase Orders with Sage 100 API
To create or update purchase orders, you will need to interact with the Sage 100 API endpoints. Below is an example of how to perform these operations using PHP:
// Include the necessary libraries
require 'vendor/autoload.php';
use GuzzleHttp\Client;
// Initialize the HTTP client
$client = new Client();
// Define the Sage 100 API endpoint for purchase orders
$endpoint = 'https://api.sage100.com/purchaseorders';
// Set up the API request headers
$headers = [
'Content-Type' => 'application/json',
'Authorization' => 'Bearer YOUR_ACCESS_TOKEN'
];
// Define the purchase order data
$purchaseOrderData = [
'orderNumber' => 'PO12345',
'vendorID' => 'VENDOR001',
'orderDate' => '2023-10-01',
'items' => [
[
'itemCode' => 'ITEM001',
'quantity' => 10,
'price' => 100.00
]
]
];
try {
// Make the API call to create or update the purchase order
$response = $client->request('POST', $endpoint, [
'headers' => $headers,
'json' => $purchaseOrderData
]);
// Parse the response
$responseData = json_decode($response->getBody(), true);
// Output the response
echo 'Purchase Order Created/Updated Successfully: ' . $responseData['orderNumber'];
} catch (Exception $e) {
// Handle errors
echo 'Error: ' . $e->getMessage();
}
Verifying API Call Success in Sage 100
After executing the API call, verify the success of the operation by checking the Sage 100 test account:
- Log in to the Sage 100 interface and navigate to the Purchase Orders module.
- Search for the purchase order number used in the API call to confirm it has been created or updated.
Handling Errors and Exceptions in Sage 100 API Calls
When making API calls, it's crucial to handle potential errors and exceptions. Sage 100 API may return various error codes, which you should manage appropriately:
- Use try-catch blocks in your PHP code to catch exceptions and handle them gracefully.
- Log error messages for further analysis and debugging.
- Refer to the Sage 100 API documentation for detailed information on error codes and their meanings.
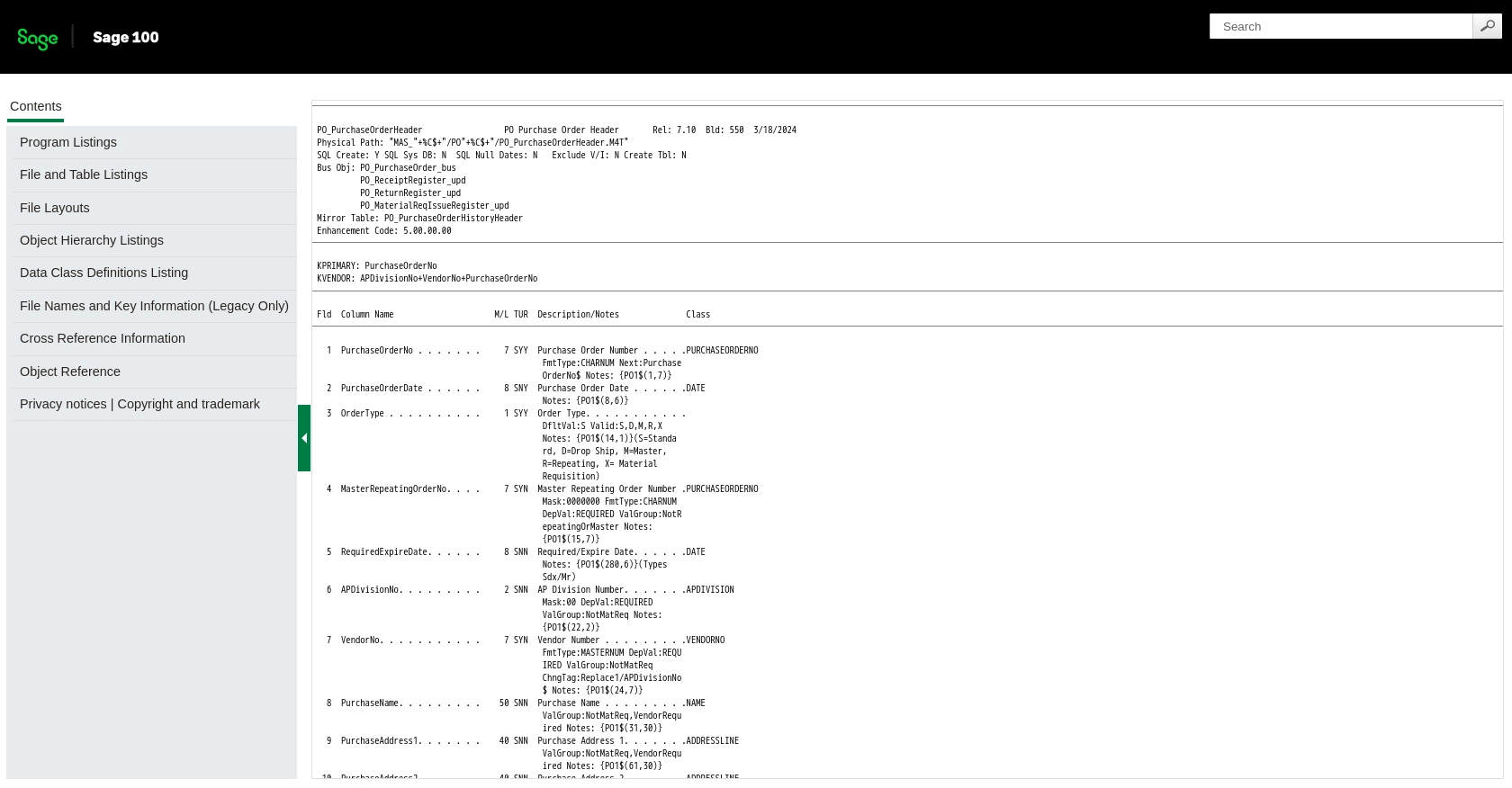
Conclusion and Best Practices for Sage 100 API Integration
Integrating with the Sage 100 API to manage purchase orders can significantly enhance your business operations by automating and streamlining processes. By following the steps outlined in this article, you can efficiently create or update purchase orders using PHP, ensuring that your records are accurate and up-to-date.
Best Practices for Secure and Efficient Sage 100 API Usage
- Securely Store API Credentials: Always store your client ID and client secret securely, using environment variables or a secure vault, to prevent unauthorized access.
- Handle Rate Limiting: Be aware of any rate limits imposed by the Sage 100 API. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Data Standardization: Ensure that data fields are standardized and validated before making API calls to prevent errors and maintain data integrity.
- Error Logging and Monitoring: Implement comprehensive logging and monitoring to quickly identify and resolve any issues that arise during API interactions.
Enhance Your Integration Strategy with Endgrate
While integrating with Sage 100 can be highly beneficial, managing multiple integrations can become complex and time-consuming. Endgrate offers a unified API solution that simplifies the integration process across various platforms, including Sage 100. By leveraging Endgrate, you can:
- Save time and resources by outsourcing integrations and focusing on your core product development.
- Build once for each use case, reducing the need for multiple integrations.
- Provide an intuitive integration experience for your customers, enhancing user satisfaction.
Explore how Endgrate can streamline your integration efforts and improve your business operations by visiting Endgrate today.
Read More
- https://endgrate.com/provider/sage100
- https://help-sage100.na.sage.com/InstallGuide/2022/Content/InstallGuide/Config_ODBC_Driver.htm
- https://help-sage100.na.sage.com/2022/FLOR/index.htm#File_Layouts/Purchase_Order/PO_PurchaseOrderHeader.htm?Highlight=PO_PurchaseOrderHeader
- https://help-sage100.na.sage.com/2022/FLOR/index.htm#File_Layouts/Purchase_Order/PO_PurchaseOrderDetail.htm?Highlight=PO_PurchaseOrderDetail
Ready to get started?