How to Get Companies with the Keap API in Javascript
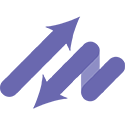
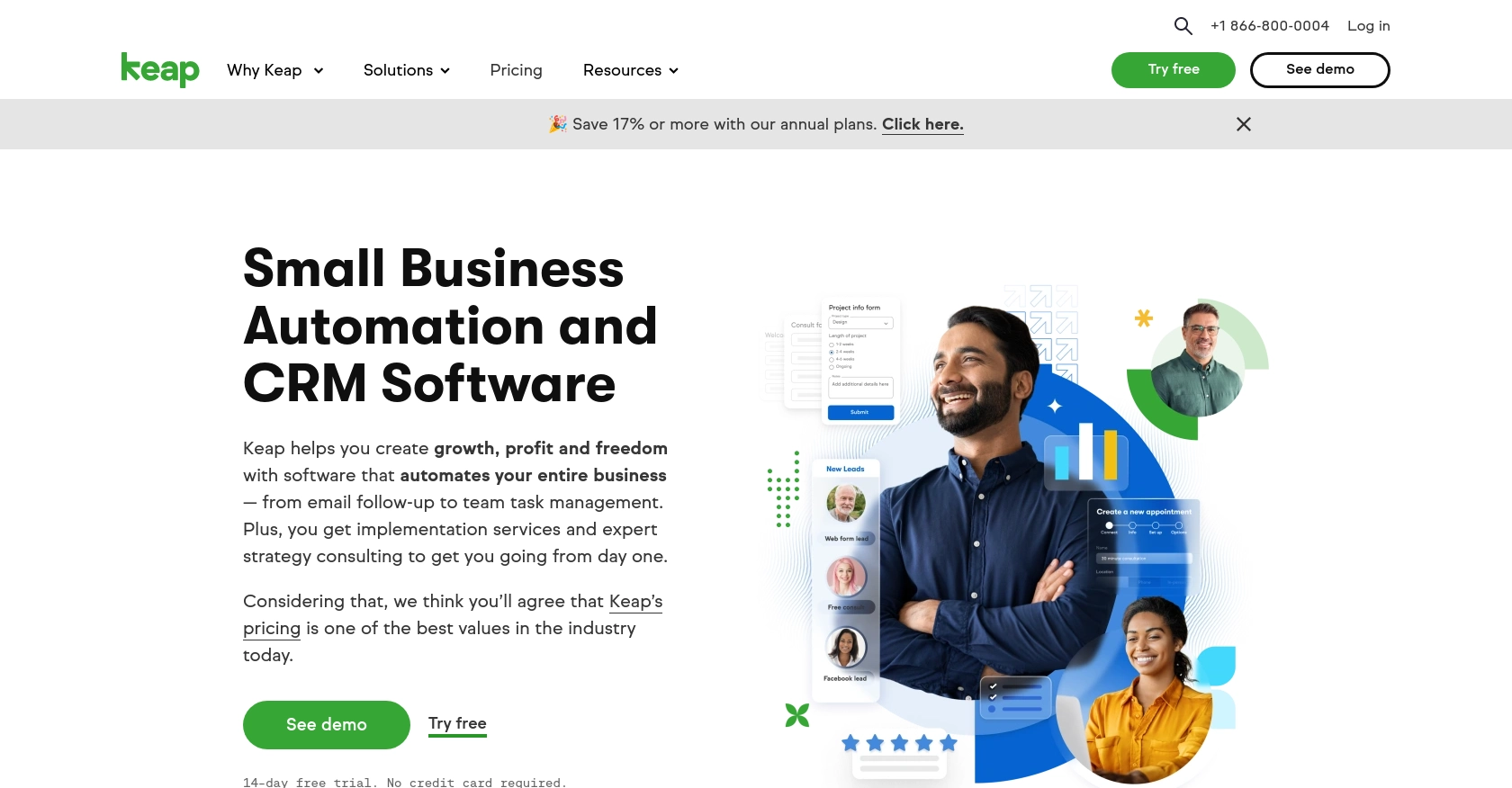
Introduction to Keap API Integration
Keap, formerly known as Infusionsoft, is a comprehensive customer relationship management (CRM) platform designed to help small businesses streamline their sales and marketing efforts. With features like contact management, email marketing, and automation, Keap empowers businesses to enhance their customer interactions and drive growth.
Developers may want to integrate with Keap's API to access and manage company data, enabling seamless automation and data synchronization. For example, using the Keap API, a developer can retrieve company information to update records in a separate database or trigger specific marketing campaigns based on company attributes.
Setting Up a Keap Developer Account and Sandbox Environment
Before you can start interacting with the Keap API, you'll need to set up a developer account and create a sandbox environment. This will allow you to test your integration without affecting live data.
Registering for a Keap Developer Account
To begin, you'll need to register for a Keap developer account. This account will give you access to the necessary tools and resources to build and test your integration.
- Visit the Keap Developer Portal.
- Click on "Register" to create a new developer account.
- Fill in the required information, including your name, email, and company details.
- Submit the form to complete the registration process.
Creating a Sandbox App in Keap
Once your developer account is set up, you can create a sandbox app. This environment will allow you to safely test your API calls.
- Log in to your Keap developer account.
- Navigate to the "Sandbox Apps" section.
- Click on "Create New Sandbox App" and provide the necessary details.
- Once created, you'll receive a client ID and client secret, which are essential for OAuth authentication.
Setting Up OAuth Authentication for Keap API
The Keap API uses OAuth 2.0 for authentication. Follow these steps to set up OAuth for your sandbox app:
- In your sandbox app settings, locate the OAuth section.
- Enter the redirect URI where users will be redirected after authentication.
- Save the changes to generate your OAuth credentials.
- Use the client ID and client secret in your application to request access tokens.
For more detailed information, refer to the Keap REST API documentation.
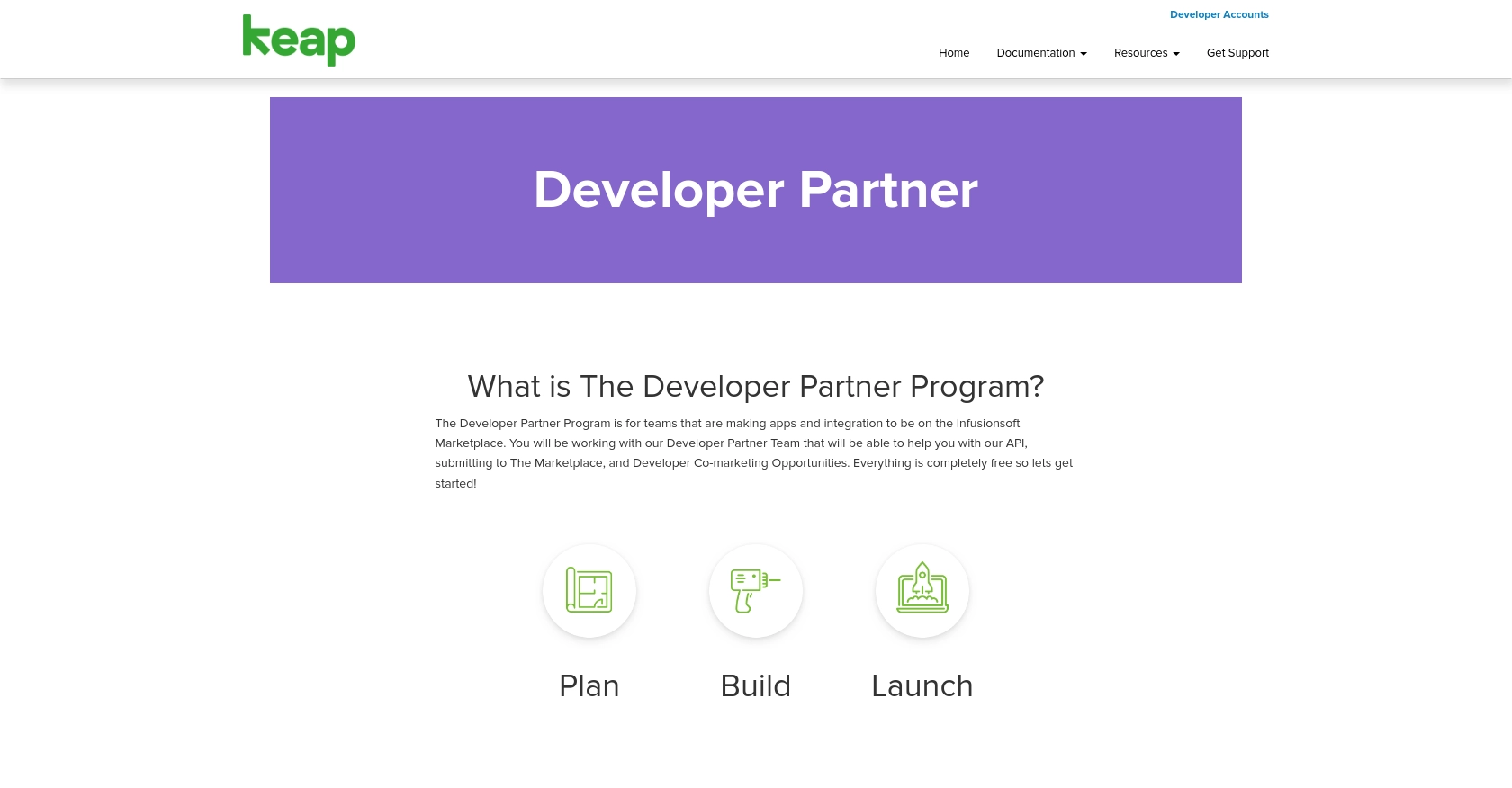
sbb-itb-96038d7
Making API Calls to Retrieve Companies with Keap API in JavaScript
To interact with the Keap API and retrieve company data, you'll use JavaScript to make HTTP requests. This section will guide you through the necessary steps, including setting up your environment, writing the code, and handling potential errors.
Setting Up Your JavaScript Environment for Keap API Integration
Before diving into the code, ensure you have the following prerequisites:
- Node.js installed on your machine.
- A code editor like Visual Studio Code.
- The
axios
library for making HTTP requests. Install it using the command:
npm install axios
Writing JavaScript Code to Fetch Companies from Keap API
Now, let's write the code to make an API call to Keap and retrieve company data. Create a file named getKeapCompanies.js
and add the following code:
const axios = require('axios');
// Set the API endpoint and headers
const endpoint = 'https://api.keap.com/crm/rest/v1/companies';
const headers = {
'Authorization': 'Bearer YOUR_ACCESS_TOKEN',
'Content-Type': 'application/json'
};
// Function to get companies
async function getCompanies() {
try {
const response = await axios.get(endpoint, { headers });
const companies = response.data.companies;
console.log(companies);
} catch (error) {
console.error('Error fetching companies:', error.response ? error.response.data : error.message);
}
}
// Call the function
getCompanies();
Replace YOUR_ACCESS_TOKEN
with the access token obtained from the OAuth process.
Verifying API Call Success and Handling Errors
After running the script, you should see a list of companies printed in the console. To verify the success of your API call, check the response status code and data structure:
- A successful request will return a status code of
200
and a JSON object containing company data. - If an error occurs, the catch block will log the error message or response data, helping you diagnose the issue.
Common error codes include:
401 Unauthorized
: Check your access token and ensure it's valid.403 Forbidden
: Verify your app's permissions and scopes.429 Too Many Requests
: Respect the rate limits imposed by Keap's API.
For more details on error handling, refer to the Keap REST API documentation.
Conclusion and Best Practices for Keap API Integration in JavaScript
Integrating with the Keap API using JavaScript allows developers to efficiently manage and automate company data, enhancing business processes and customer interactions. By following the steps outlined in this guide, you can successfully retrieve company information and handle potential errors effectively.
Best Practices for Secure and Efficient Keap API Usage
- Securely Store Credentials: Always store your OAuth credentials, such as client ID and client secret, securely. Avoid hardcoding them in your source code. Consider using environment variables or secure vaults.
- Handle Rate Limiting: Keap's API may impose rate limits. Implement logic to handle
429 Too Many Requests
errors by retrying requests after a delay. For more details, refer to the Keap REST API documentation. - Data Standardization: Ensure that the data retrieved from Keap is standardized and transformed as needed to fit your application's requirements.
- Monitor API Usage: Regularly monitor your API usage and performance to identify any bottlenecks or issues that may arise.
Streamline Your Integration Process with Endgrate
While integrating with Keap's API can be straightforward, managing multiple integrations across different platforms can become complex. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Keap. By using Endgrate, you can save time and resources, allowing you to focus on your core product development.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate and discover a more efficient way to manage your integrations.
Read More
Ready to get started?