How to Get Contacts with the Hubspot API in PHP
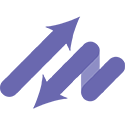
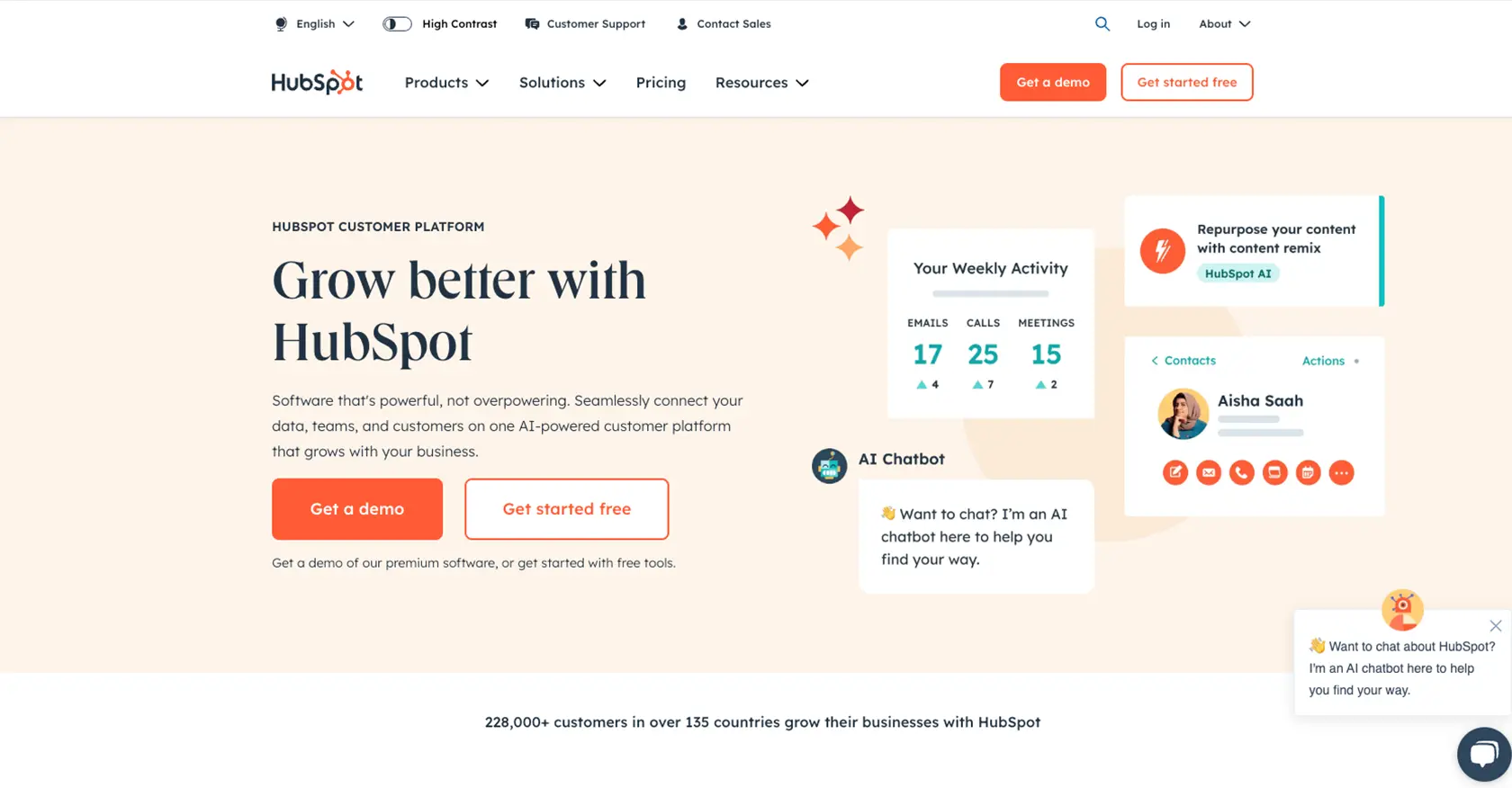
Introduction to HubSpot API Integration
HubSpot is a comprehensive CRM platform that provides a suite of tools to help businesses manage their marketing, sales, and customer service efforts. Known for its user-friendly interface and robust features, HubSpot is a popular choice for companies looking to streamline their customer relationship management processes.
Developers often integrate with HubSpot's API to access and manage customer data, such as contacts, to enhance marketing automation and personalization. For example, a developer might use the HubSpot API to retrieve contact information and tailor marketing campaigns based on user interactions and preferences.
This article will guide you through the process of using PHP to interact with the HubSpot API, specifically focusing on retrieving contact data. By the end of this tutorial, you'll be equipped to efficiently access and manage contacts within the HubSpot platform using PHP.
Setting Up Your HubSpot Test or Sandbox Account
Before diving into the HubSpot API integration with PHP, it's essential to set up a test or sandbox account. This environment allows you to safely experiment with API calls without affecting live data. HubSpot provides developers with the tools needed to create a sandbox account, which is crucial for testing and development purposes.
Creating a HubSpot Developer Account
If you don't already have a HubSpot developer account, you'll need to create one. Follow these steps to get started:
- Visit the HubSpot Developer Portal.
- Click on "Create a developer account" and fill out the necessary information.
- Once your account is set up, log in to access the developer dashboard.
Setting Up a HubSpot Sandbox Account
With your developer account ready, you can now create a sandbox account to test your API integrations:
- Navigate to the "Test Accounts" section in your developer dashboard.
- Click on "Create a test account" to generate a sandbox environment.
- Follow the prompts to configure your sandbox account settings.
Configuring OAuth for HubSpot API Access
HubSpot uses OAuth for authentication, ensuring secure access to the API. Here's how to set it up:
- In your developer dashboard, go to the "Apps" section and click "Create an app."
- Fill in the app details, including name and description.
- Under "Auth," select OAuth 2.0 as the authentication method.
- Specify the required scopes for accessing contacts, such as
crm.objects.contacts.read
. - Save your app settings to generate a client ID and client secret.
For more detailed information on OAuth setup, refer to the HubSpot OAuth Documentation.
Generating OAuth Tokens
Once your app is configured, you can generate OAuth tokens to authenticate API requests:
- Direct users to the authorization URL provided in your app settings.
- Upon user consent, capture the authorization code from the callback URL.
- Exchange the authorization code for an access token using a POST request to HubSpot's token endpoint.
// Example PHP code to exchange authorization code for access token
$client_id = 'your_client_id';
$client_secret = 'your_client_secret';
$redirect_uri = 'your_redirect_uri';
$authorization_code = 'authorization_code_from_callback';
$token_url = 'https://api.hubapi.com/oauth/v1/token';
$data = [
'grant_type' => 'authorization_code',
'client_id' => $client_id,
'client_secret' => $client_secret,
'redirect_uri' => $redirect_uri,
'code' => $authorization_code
];
$options = [
'http' => [
'header' => "Content-type: application/x-www-form-urlencoded\r\n",
'method' => 'POST',
'content' => http_build_query($data),
],
];
$context = stream_context_create($options);
$result = file_get_contents($token_url, false, $context);
$response = json_decode($result, true);
$access_token = $response['access_token'];
Store the access token securely, as it will be used to authenticate your API requests.
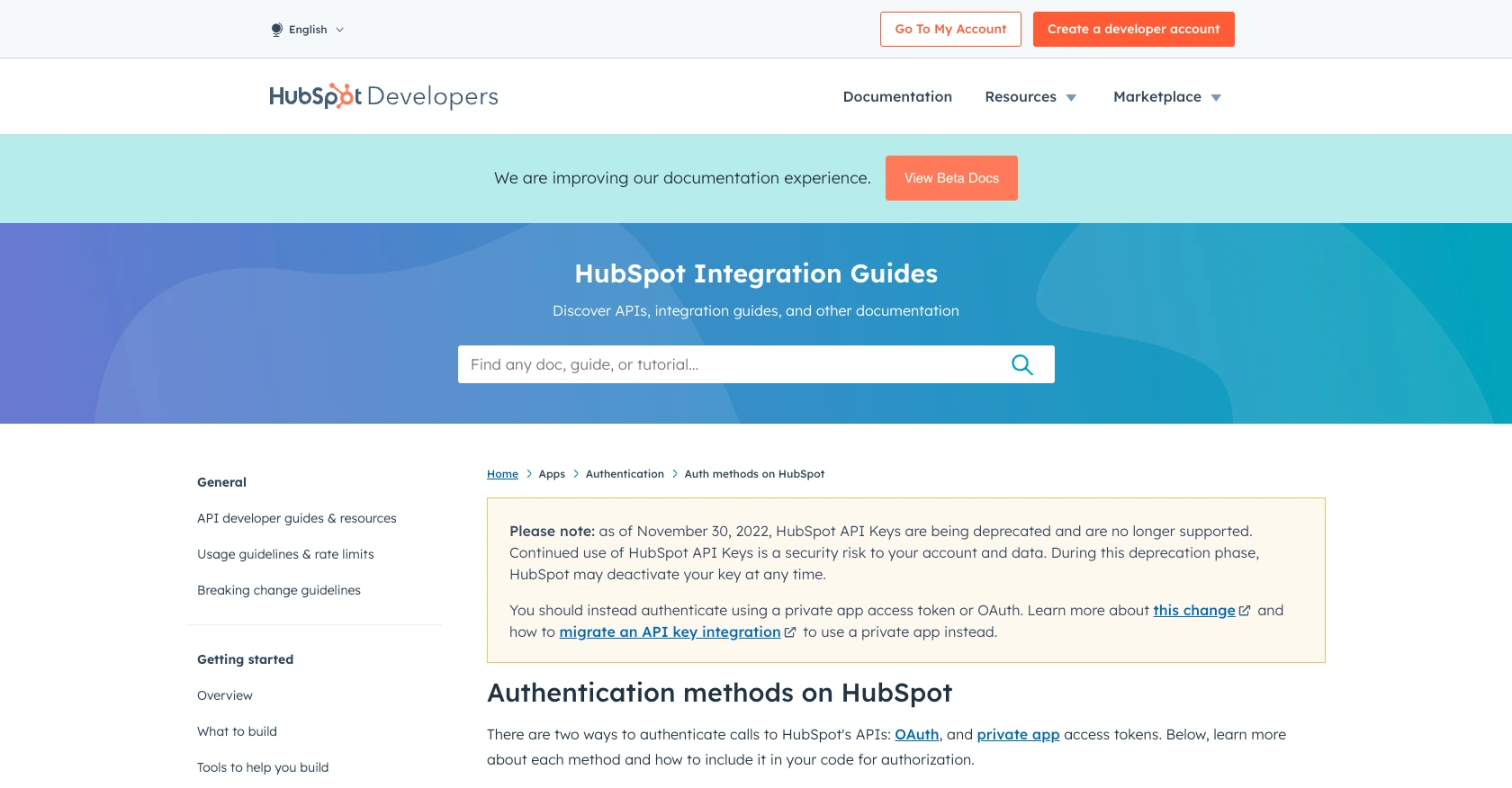
sbb-itb-96038d7
Making API Calls to Retrieve Contacts from HubSpot Using PHP
In this section, we'll explore how to make API calls to retrieve contact data from HubSpot using PHP. This involves setting up your PHP environment, installing necessary dependencies, and writing code to interact with the HubSpot API.
Setting Up Your PHP Environment
Before making API calls, ensure your PHP environment is properly configured. You'll need:
- PHP 7.4 or higher
- Composer for dependency management
Install the HubSpot API client for PHP using Composer:
composer require hubspot/api-client
Writing PHP Code to Retrieve HubSpot Contacts
With your environment set up, you can now write PHP code to interact with the HubSpot API and retrieve contacts. Here's a step-by-step guide:
require 'vendor/autoload.php';
use HubSpot\Factory;
// Initialize the HubSpot client
$accessToken = 'your_access_token';
$client = Factory::createWithAccessToken($accessToken);
try {
// Retrieve contacts from HubSpot
$response = $client->crm()->contacts()->basicApi()->getPage();
$contacts = $response->getResults();
// Display contact information
foreach ($contacts as $contact) {
echo "Name: " . $contact->getProperties()['firstname'] . " " . $contact->getProperties()['lastname'] . "\n";
echo "Email: " . $contact->getProperties()['email'] . "\n\n";
}
} catch (Exception $e) {
echo "Error: " . $e->getMessage();
}
Replace your_access_token
with the access token obtained during the OAuth setup. This code initializes the HubSpot client, retrieves a list of contacts, and prints their names and email addresses.
Verifying API Call Success in HubSpot Sandbox
After running the PHP script, verify the success of your API call by checking the contacts in your HubSpot sandbox account. The retrieved contacts should match those in your HubSpot environment.
Handling Errors and Understanding Error Codes
When making API calls, it's crucial to handle potential errors. The HubSpot API provides error codes to help diagnose issues. Common error codes include:
- 401 Unauthorized: Invalid or expired access token.
- 429 Too Many Requests: Rate limit exceeded. HubSpot allows 100 requests every 10 seconds for OAuth apps. See HubSpot Usage Guidelines for more details.
Implement error handling in your code to manage these scenarios effectively, ensuring a robust integration.
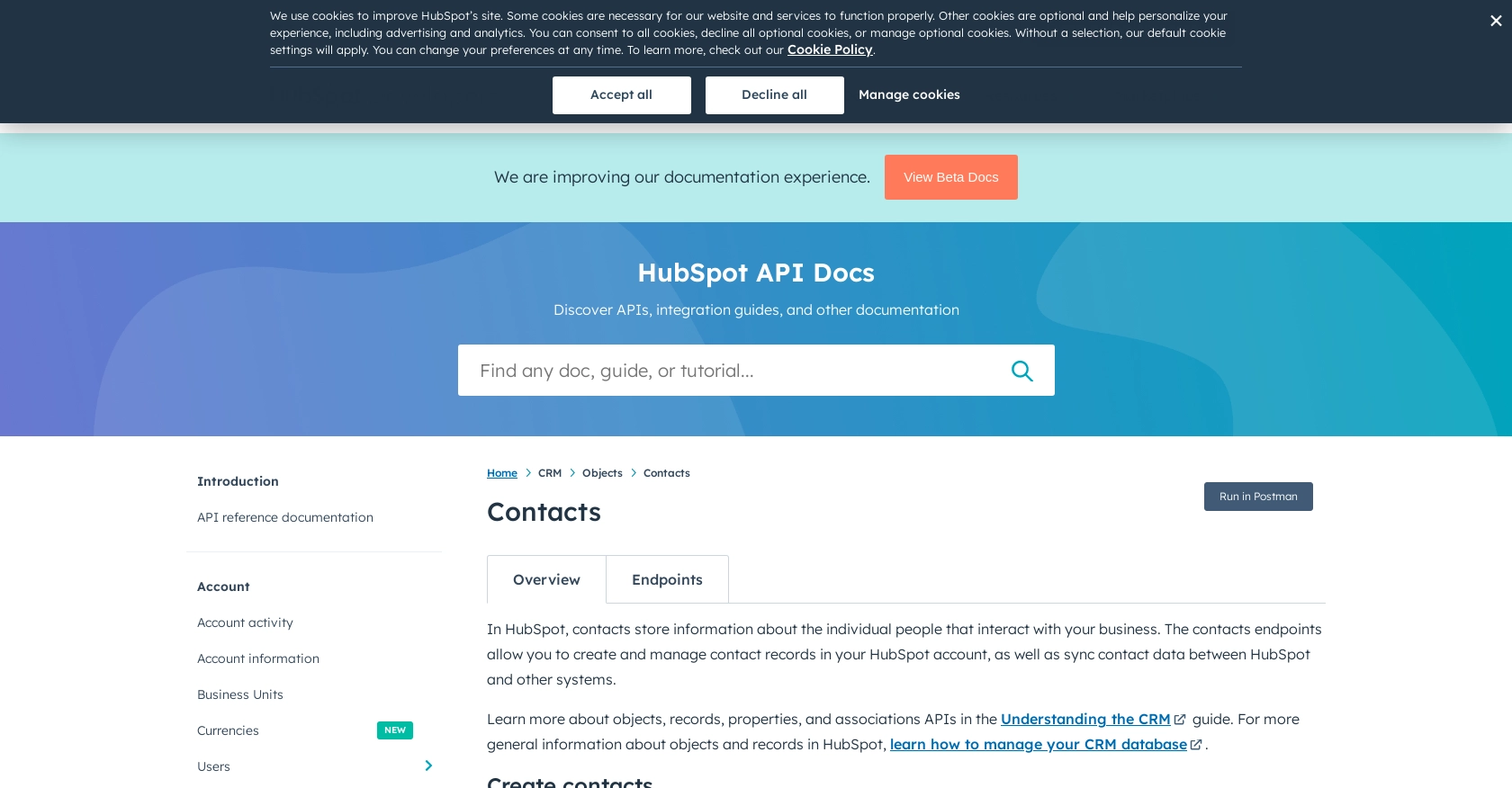
Conclusion and Best Practices for HubSpot API Integration with PHP
Integrating with the HubSpot API using PHP provides developers with powerful capabilities to manage and utilize contact data effectively. By following the steps outlined in this guide, you can seamlessly retrieve and handle contact information, enhancing your application's functionality and user experience.
Best Practices for Secure and Efficient HubSpot API Usage
- Secure Storage of Credentials: Always store your OAuth tokens securely. Consider using environment variables or secure vaults to prevent unauthorized access.
- Handle Rate Limiting: Be mindful of HubSpot's rate limits, which allow 100 requests every 10 seconds for OAuth apps. Implement retry logic and exponential backoff strategies to manage rate limit errors effectively. Refer to the HubSpot Usage Guidelines for more information.
- Data Standardization: Ensure consistent data formats when retrieving and storing contact information. This practice aids in data integrity and simplifies data processing across different systems.
Streamlining Integrations with Endgrate
While building integrations with HubSpot can be rewarding, it can also be time-consuming and complex, especially when managing multiple integrations. Endgrate offers a solution by providing a unified API endpoint that connects to various platforms, including HubSpot. This allows developers to focus on core product development while outsourcing integration complexities.
By leveraging Endgrate, you can build once for each use case instead of multiple times for different integrations, saving both time and resources. Explore the benefits of a streamlined integration experience with Endgrate by visiting Endgrate's website.
With these insights and tools, you're well-equipped to enhance your applications with robust HubSpot integrations, driving better customer engagement and operational efficiency.
Read More
- https://endgrate.com/provider/hubspot
- https://developers.hubspot.com/docs/api/overview
- https://developers.hubspot.com/docs/api/intro-to-auth
- https://developers.hubspot.com/docs/api/usage-details
- https://developers.hubspot.com/docs/api/scopes
- https://developers.hubspot.com/docs/api/crm/contacts
- https://developers.hubspot.com/docs/api/crm/properties
Ready to get started?