Using the Odoo Online API to Create Records in Javascript
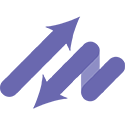
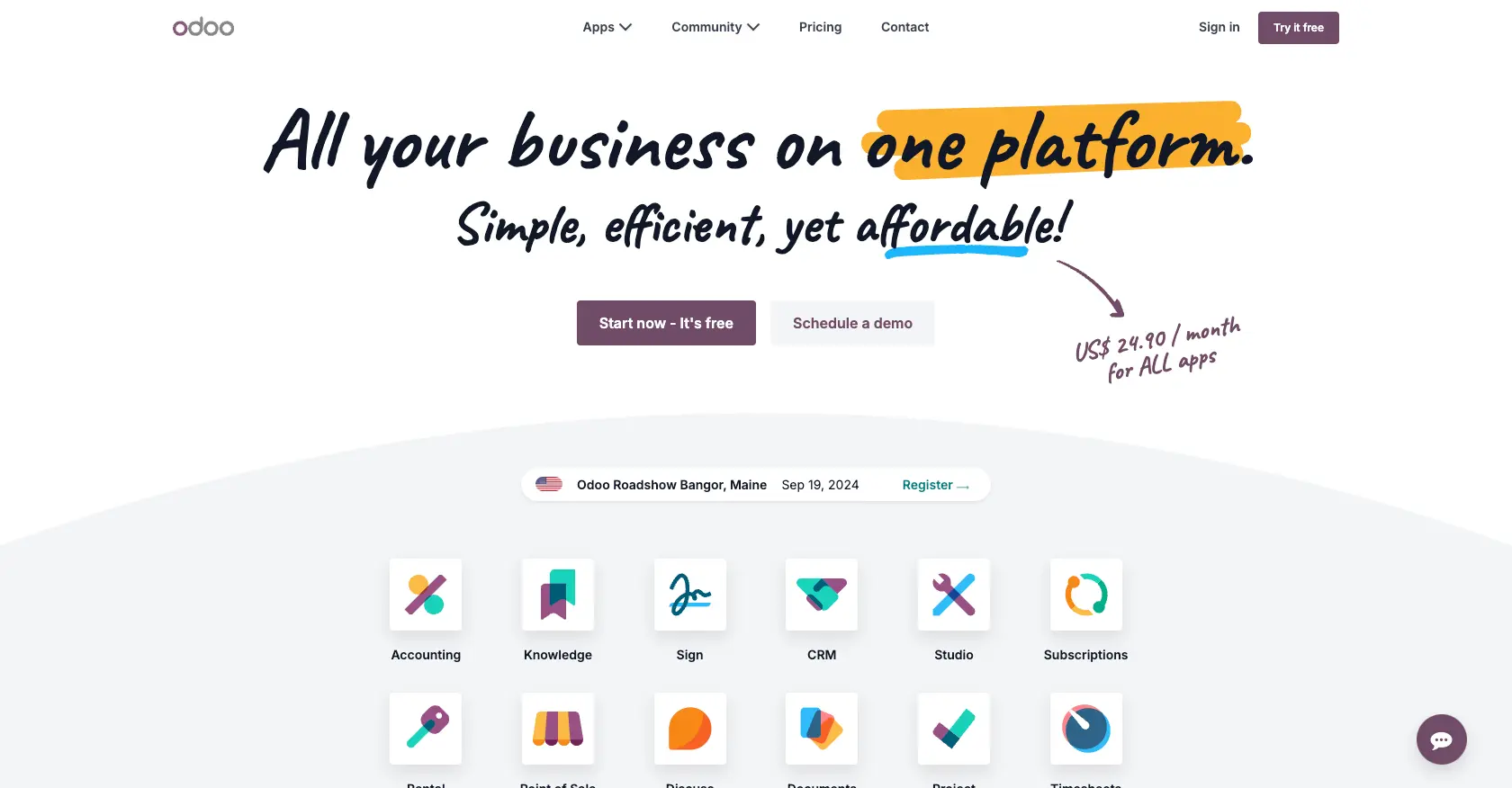
Introduction to Odoo Online API
Odoo Online is a comprehensive suite of business applications that offers a wide range of functionalities, including CRM, e-commerce, billing, accounting, manufacturing, warehouse, project management, and inventory management. Its modular approach allows businesses to customize and scale their operations efficiently.
For developers, integrating with the Odoo Online API can significantly enhance the automation and management of business processes. By leveraging the API, developers can create, update, and manage records programmatically, streamlining operations and reducing manual effort.
In this article, we will explore how to use JavaScript to create records in Odoo Online, providing a practical guide for developers looking to integrate this powerful tool into their applications.
Setting Up Your Odoo Online Test/Sandbox Account
Before you can start creating records in Odoo Online using JavaScript, you'll need to set up a test or sandbox account. This will allow you to safely experiment with the API without affecting live data.
Creating an Odoo Online Account
If you don't already have an Odoo Online account, you can sign up for a free trial on the Odoo website. Follow the instructions to create your account, which will give you access to the Odoo Online platform.
Accessing the Odoo Online Sandbox Environment
Odoo provides a sandbox environment where developers can test their integrations. This environment mimics the live system, allowing you to test API calls without impacting real data.
- Log in to your Odoo Online account.
- Navigate to the developer section to access the sandbox environment.
- Ensure you have the necessary permissions to create and manage records within the sandbox.
Setting Up Custom Authentication for Odoo Online API
Odoo Online uses a custom authentication method for API access. To authenticate your requests, you'll need to create an application within your sandbox account.
- Go to the settings section of your Odoo Online account.
- Navigate to the API access or integrations tab.
- Create a new application and note down the client ID and client secret provided.
- Configure the application to have the necessary scopes for creating records.
These credentials will be used in your JavaScript code to authenticate API requests.
Generating API Keys for Odoo Online
In addition to client credentials, you may need an API key for certain operations. Here's how to generate one:
- In your Odoo Online account, go to the API keys section.
- Click on "Generate New API Key" and provide a name for the key.
- Copy the generated API key and store it securely, as you'll need it for API calls.
With your sandbox account set up and authentication configured, you're ready to start making API calls to create records in Odoo Online using JavaScript.
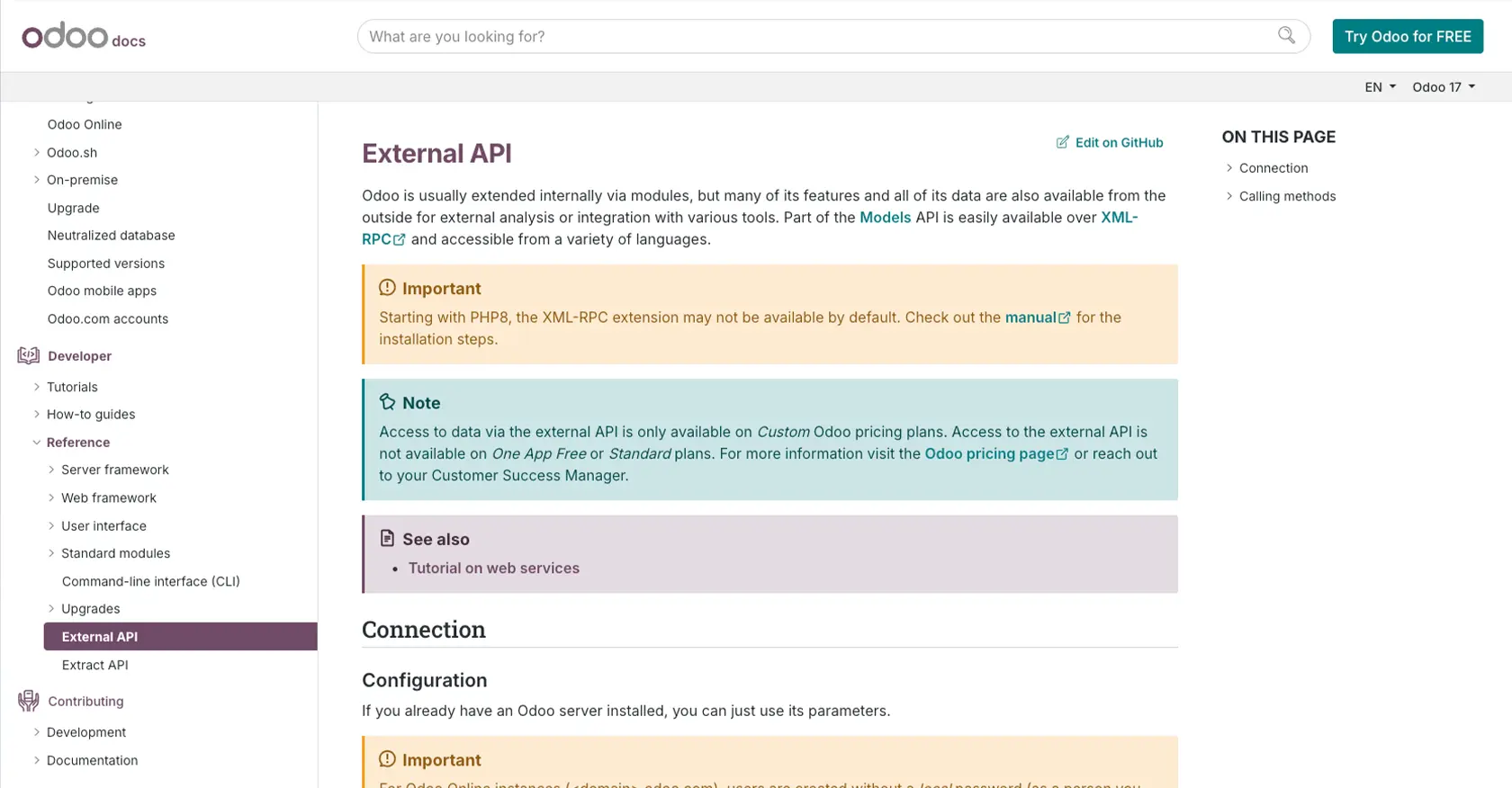
sbb-itb-96038d7
Making API Calls with JavaScript to Create Records in Odoo Online
To interact with the Odoo Online API using JavaScript, you'll need to ensure you have the right setup and dependencies. This section will guide you through the process of making API calls to create records in Odoo Online.
Setting Up Your JavaScript Environment for Odoo Online API
Before making API calls, ensure that your JavaScript environment is properly configured. You'll need Node.js and npm installed on your machine. If you haven't already, download and install them from the official Node.js website.
Once installed, you can use npm to manage your project's dependencies. For this tutorial, we'll use the axios
library to handle HTTP requests. Install it by running the following command in your terminal:
npm install axios
Creating Records in Odoo Online Using JavaScript
With your environment set up, you can now proceed to create records in Odoo Online. Below is a sample code snippet demonstrating how to achieve this using JavaScript and the axios
library.
const axios = require('axios');
// Set the API endpoint and headers
const endpoint = 'https://your-odoo-instance.com/api/v1/records';
const headers = {
'Content-Type': 'application/json',
'Authorization': 'Bearer YOUR_API_KEY'
};
// Define the data for the new record
const newRecord = {
name: 'New Record Name',
description: 'Description of the new record'
};
// Function to create a new record
async function createRecord() {
try {
const response = await axios.post(endpoint, newRecord, { headers });
console.log('Record created successfully:', response.data);
} catch (error) {
console.error('Error creating record:', error.response ? error.response.data : error.message);
}
}
// Call the function to create the record
createRecord();
Replace YOUR_API_KEY
with the API key you generated earlier. The endpoint
should be the URL of your Odoo Online instance's API endpoint for creating records.
Verifying Successful API Requests in Odoo Online
After executing the code, you should verify that the record was created successfully in your Odoo Online sandbox environment. Log in to your Odoo Online account and navigate to the module where the record was created. You should see the new record listed there.
Handling Errors and Common Error Codes in Odoo Online API
When making API calls, it's crucial to handle potential errors gracefully. The Odoo Online API may return various error codes, such as:
- 400 Bad Request: The request was malformed or contains invalid parameters.
- 401 Unauthorized: Authentication failed due to invalid API key or credentials.
- 403 Forbidden: You do not have permission to perform the requested operation.
- 404 Not Found: The requested resource could not be found.
Ensure your code includes error handling logic to manage these scenarios, as demonstrated in the sample code above.
Conclusion and Best Practices for Using Odoo Online API with JavaScript
Integrating with the Odoo Online API using JavaScript can greatly enhance your ability to automate and manage business processes. By following the steps outlined in this guide, you can efficiently create records and interact with Odoo Online's robust suite of applications.
Best Practices for Secure and Efficient Odoo Online API Integration
- Secure Storage of Credentials: Always store your API keys and client credentials securely. Consider using environment variables or secure vaults to prevent unauthorized access.
- Handling Rate Limits: Be mindful of any rate limits imposed by Odoo Online. Implement logic to handle rate limit responses and retry requests as necessary.
- Data Transformation and Standardization: Ensure that data being sent to and received from the API is properly formatted and standardized to maintain consistency across your applications.
- Error Handling: Implement comprehensive error handling to manage various HTTP status codes and ensure your application can gracefully recover from API errors.
Streamlining Integrations with Endgrate
While integrating with Odoo Online can be powerful, managing multiple integrations across different platforms can become complex and time-consuming. Endgrate offers a unified API solution that simplifies this process by providing a single endpoint to connect with multiple services, including Odoo Online.
By leveraging Endgrate, you can save time and resources, allowing you to focus on your core product development. Whether you need to build once for each use case or provide an intuitive integration experience for your customers, Endgrate can help streamline your integration efforts.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate's website and discover the benefits of a unified API approach.
Read More
Ready to get started?