How to Get Contacts with the Hubspot API in Python
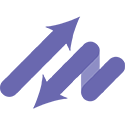
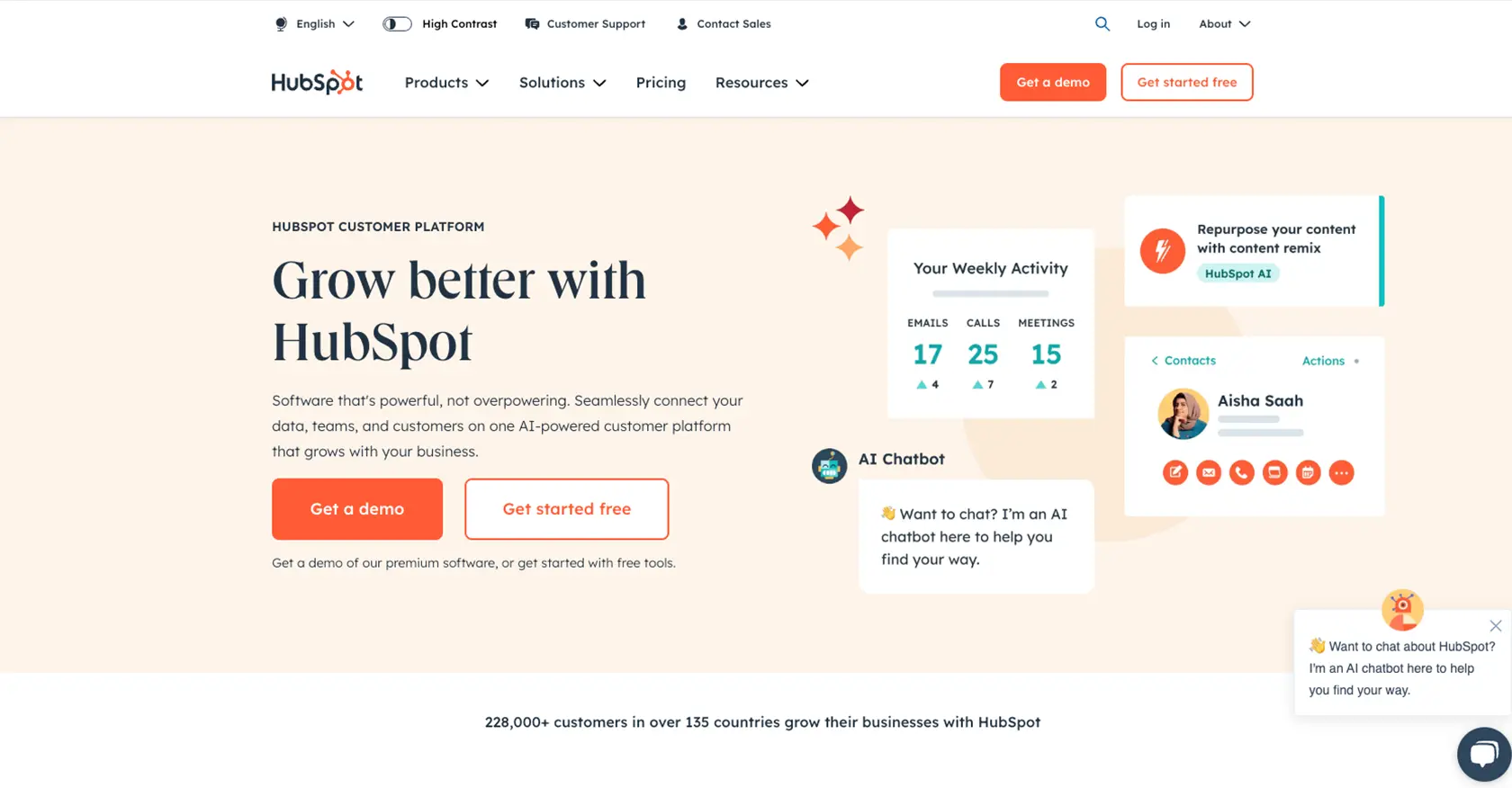
Introduction to HubSpot CRM Integration
HubSpot is a comprehensive CRM platform that offers a suite of tools to help businesses manage their marketing, sales, and customer service efforts. Known for its user-friendly interface and robust features, HubSpot is a popular choice for businesses looking to streamline their customer relationship management processes.
Integrating with HubSpot's API allows developers to access and manipulate customer data, such as contacts, to enhance marketing strategies and automate workflows. For example, a developer might use the HubSpot API to retrieve contact information and trigger personalized marketing campaigns based on user interactions.
This article will guide you through the process of using Python to interact with the HubSpot API, specifically focusing on retrieving contact data. By the end of this tutorial, you'll be equipped to efficiently access and manage contacts within the HubSpot platform using Python.
Setting Up Your HubSpot Test Account for API Integration
Before diving into the HubSpot API, you need to set up a test or sandbox account. This will allow you to safely experiment with API calls without affecting live data. HubSpot offers a developer account that you can use to create apps and test integrations.
Creating a HubSpot Developer Account
To begin, you'll need a HubSpot developer account. If you don't have one, follow these steps:
- Visit the HubSpot Developer Portal.
- Click on "Create a developer account" and fill in the required information.
- Once your account is set up, log in to access the developer dashboard.
Setting Up a HubSpot Sandbox Environment
With your developer account, you can create a sandbox environment to test your API integrations:
- Navigate to the "Test Accounts" section in your developer dashboard.
- Click on "Create a test account" and follow the prompts to set up your sandbox environment.
- This sandbox will mimic a real HubSpot account, allowing you to test API calls safely.
Configuring OAuth for HubSpot API Access
HubSpot uses OAuth for authentication, ensuring secure access to its APIs. Follow these steps to configure OAuth:
- In your developer account, go to the "Apps" section and click "Create an app."
- Fill in the app details, including name and description.
- Under "Auth," select OAuth 2.0 as the authentication method.
- Specify the necessary scopes for your app, such as
crm.objects.contacts.read
for reading contacts. - Save your app to generate the client ID and client secret, which you'll use for authentication.
For more detailed information on OAuth setup, refer to the HubSpot OAuth Documentation.
Generating OAuth Tokens
To interact with the HubSpot API, you'll need to generate OAuth tokens:
- Direct users to the authorization URL provided by HubSpot, including your client ID and requested scopes.
- Once users authorize your app, they'll be redirected to your specified redirect URI with an authorization code.
- Exchange this authorization code for an access token by making a POST request to HubSpot's token endpoint.
import requests
# Define the token endpoint and payload
token_url = "https://api.hubapi.com/oauth/v1/token"
payload = {
'grant_type': 'authorization_code',
'client_id': 'YOUR_CLIENT_ID',
'client_secret': 'YOUR_CLIENT_SECRET',
'redirect_uri': 'YOUR_REDIRECT_URI',
'code': 'AUTHORIZATION_CODE'
}
# Make the POST request to get the access token
response = requests.post(token_url, data=payload)
tokens = response.json()
print(tokens)
Replace YOUR_CLIENT_ID
, YOUR_CLIENT_SECRET
, YOUR_REDIRECT_URI
, and AUTHORIZATION_CODE
with your actual values.
With your OAuth tokens ready, you can now proceed to make API calls to retrieve contacts from HubSpot.
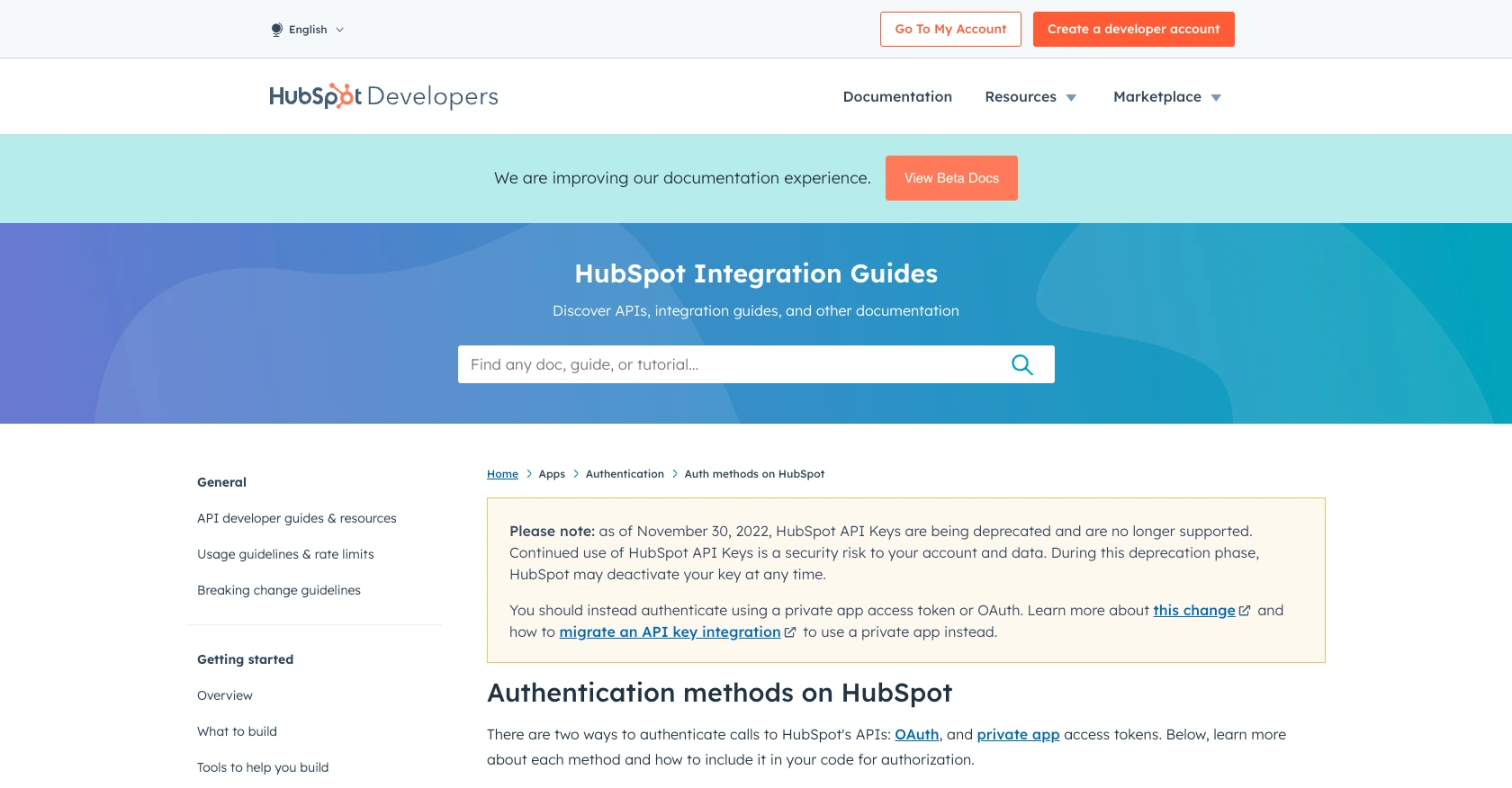
sbb-itb-96038d7
Making API Calls to Retrieve Contacts from HubSpot Using Python
Now that you have your OAuth tokens, you can proceed to make API calls to retrieve contact data from HubSpot. This section will guide you through the process of setting up your Python environment and executing the necessary API calls.
Setting Up Your Python Environment for HubSpot API Integration
Before making API calls, ensure you have the correct version of Python and necessary dependencies installed. For this tutorial, you'll need:
- Python 3.11.1
- The
requests
library for handling HTTP requests
Install the requests
library using pip if you haven't already:
pip install requests
Executing the HubSpot API Call to Retrieve Contacts
With your environment set up, you can now write a Python script to interact with the HubSpot API and retrieve contact information. Follow these steps:
import requests
# Define the API endpoint and headers
endpoint = "https://api.hubapi.com/crm/v3/objects/contacts"
headers = {
"Authorization": "Bearer YOUR_ACCESS_TOKEN",
"Content-Type": "application/json"
}
# Make a GET request to the API
response = requests.get(endpoint, headers=headers)
# Check if the request was successful
if response.status_code == 200:
contacts = response.json()
for contact in contacts['results']:
print(f"Name: {contact['properties']['firstname']} {contact['properties']['lastname']}, Email: {contact['properties']['email']}")
else:
print(f"Failed to retrieve contacts: {response.status_code} - {response.text}")
Replace YOUR_ACCESS_TOKEN
with the access token you obtained earlier. This script sends a GET request to the HubSpot API endpoint to retrieve contacts. If successful, it prints out the first name, last name, and email of each contact.
Verifying Successful API Requests in HubSpot Sandbox
After running your script, verify the retrieved data by checking your HubSpot sandbox environment. Ensure the contacts displayed in your script output match those in your sandbox account.
Handling Errors and Understanding HubSpot API Error Codes
When making API calls, it's crucial to handle potential errors gracefully. HubSpot's API may return various error codes, such as:
- 401 Unauthorized: Indicates an issue with authentication. Check your access token.
- 429 Too Many Requests: You've hit the rate limit. Implement rate limiting strategies.
- 500 Internal Server Error: A server-side issue. Retry the request or contact support if persistent.
For more detailed information on error handling, refer to the HubSpot Contacts API Documentation.
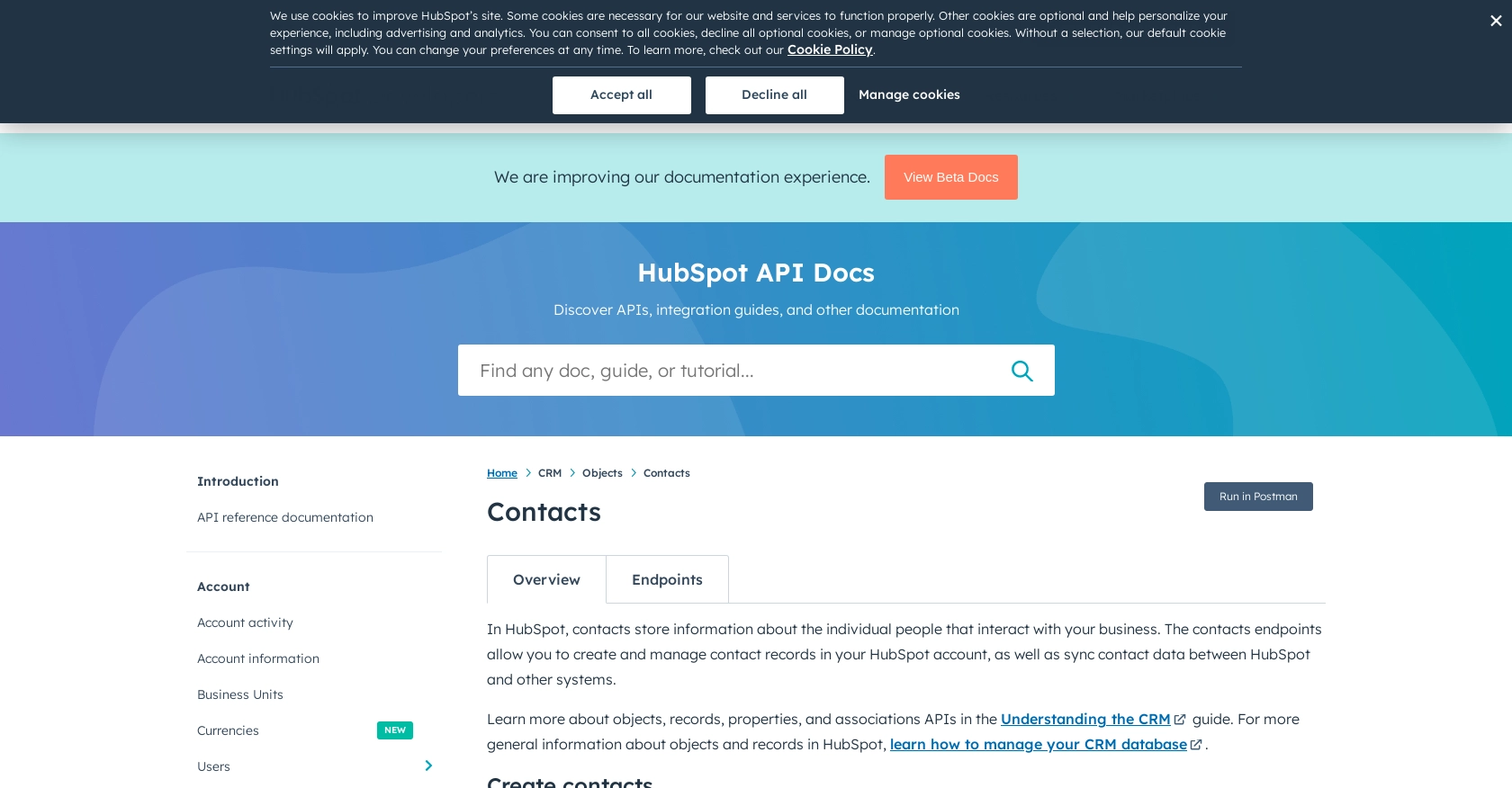
Conclusion and Best Practices for HubSpot API Integration with Python
Integrating with the HubSpot API using Python allows developers to efficiently manage and retrieve contact data, enhancing marketing strategies and automating workflows. By following the steps outlined in this tutorial, you can seamlessly access HubSpot's CRM capabilities to improve your business processes.
Best Practices for Secure and Efficient HubSpot API Usage
- Securely Store Credentials: Always store your OAuth tokens securely. Avoid hardcoding them in your scripts and consider using environment variables or secure vaults.
- Handle Rate Limits: HubSpot enforces rate limits to ensure fair usage. Implement strategies to handle the
429 Too Many Requests
error by queuing requests or using exponential backoff. - Data Standardization: Ensure consistent data formats when retrieving and storing contact information. This helps maintain data integrity across different systems.
- Error Handling: Implement robust error handling to manage API errors gracefully. Log errors for further analysis and retry requests when appropriate.
Streamline Your Integrations with Endgrate
While integrating with HubSpot's API can be straightforward, managing multiple integrations can become complex. Endgrate simplifies this process by providing a unified API endpoint for various platforms, including HubSpot. By leveraging Endgrate, you can:
- Save time and resources by outsourcing integrations, allowing you to focus on your core product.
- Build once for each use case, reducing the need for multiple integration efforts.
- Offer an intuitive integration experience for your customers, enhancing user satisfaction.
Explore how Endgrate can transform your integration strategy by visiting Endgrate.
Read More
- https://endgrate.com/provider/hubspot
- https://developers.hubspot.com/docs/api/overview
- https://developers.hubspot.com/docs/api/intro-to-auth
- https://developers.hubspot.com/docs/api/usage-details
- https://developers.hubspot.com/docs/api/scopes
- https://developers.hubspot.com/docs/api/crm/contacts
- https://developers.hubspot.com/docs/api/crm/properties
Ready to get started?