Using the Chargebee API to Create or Update Product Catalog (with Python examples)
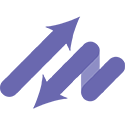
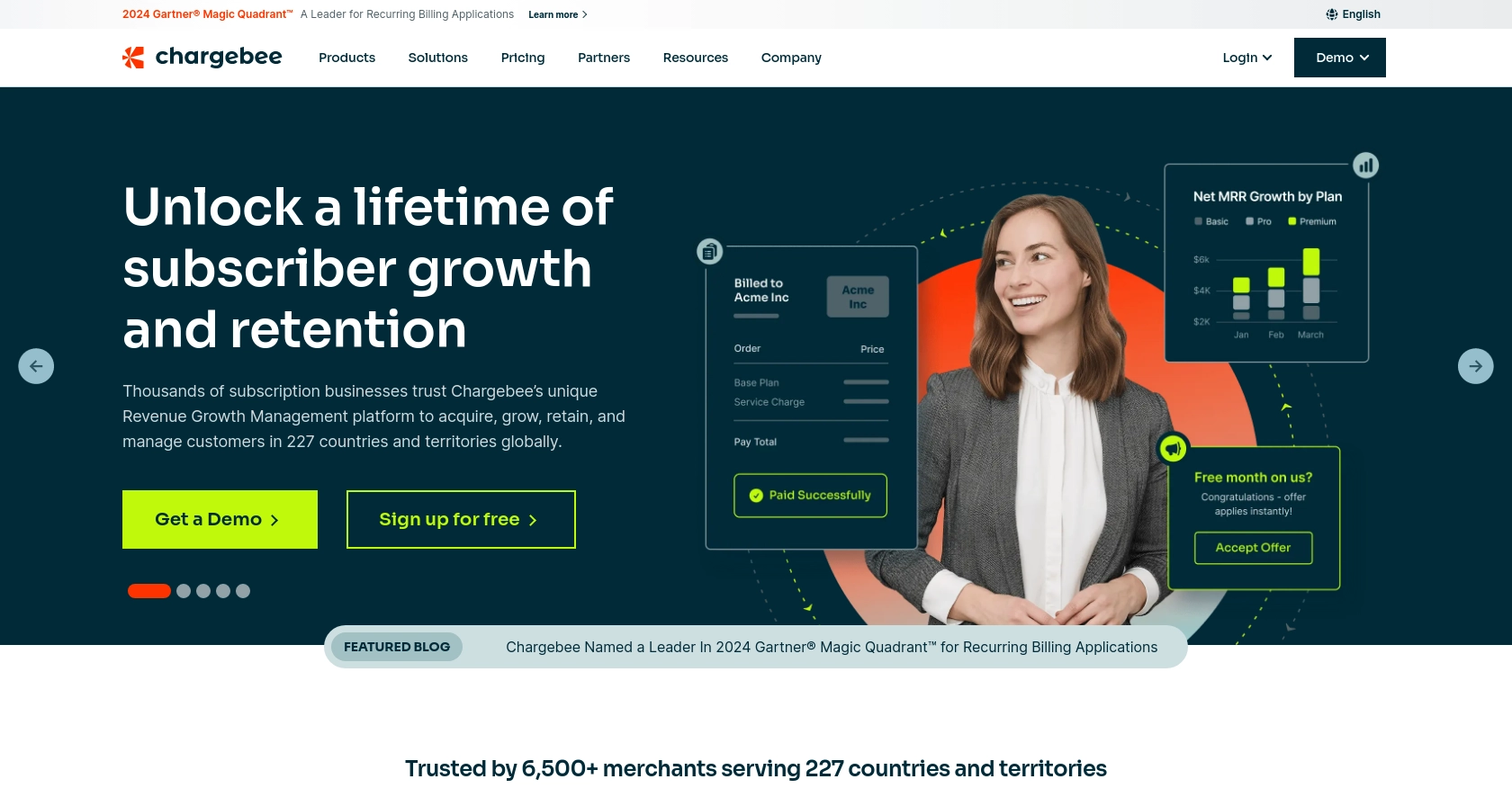
Introduction to Chargebee API for Product Catalog Management
Chargebee is a robust subscription management platform that empowers businesses to streamline their billing and revenue operations. With its comprehensive suite of tools, Chargebee simplifies the complexities of managing subscriptions, invoicing, and revenue recognition, making it a preferred choice for SaaS companies.
Integrating with Chargebee's API allows developers to efficiently manage product catalogs, enabling seamless creation and updating of items such as plans, addons, and charges. For example, a developer might use the Chargebee API to automate the process of updating product details across multiple platforms, ensuring consistency and accuracy in billing operations.
This article will guide you through using Python to interact with the Chargebee API, focusing on creating and updating product catalogs. By leveraging Chargebee's API, developers can enhance their product management capabilities and streamline their subscription workflows.
Setting Up Your Chargebee Test/Sandbox Account for API Integration
Before diving into the Chargebee API for managing your product catalog, it's essential to set up a test or sandbox account. This environment allows you to experiment with API calls without affecting your live data, ensuring a safe space for development and testing.
Create a Chargebee Test Account
If you don't already have a Chargebee account, follow these steps to create a test account:
- Visit the Chargebee website and sign up for a free trial.
- Fill in the required details and complete the registration process.
- Once registered, log in to your Chargebee dashboard.
Generate API Keys for Authentication
Chargebee uses HTTP Basic authentication for API calls, where the username is your API key and the password is left empty. Follow these steps to generate your API keys:
- Navigate to the 'Settings' section in your Chargebee dashboard.
- Select 'API Keys' under the 'Configure Chargebee' menu.
- Click on 'Add API Key' to create a new key.
- Provide a name for your API key and set the necessary permissions.
- Save the key and note it down securely, as it will be used for authenticating your API requests.
Remember, the API keys for your test site are different from those for your live site. Ensure you use the correct keys for your test environment.
Configure OAuth for Chargebee API Access
Although Chargebee primarily uses API keys for authentication, you might need to configure OAuth for certain integrations. Here’s how to set it up:
- In the Chargebee dashboard, go to 'Settings' and select 'OAuth Apps'.
- Click on 'Create OAuth App' and fill in the required details, such as the app name and redirect URL.
- Set the necessary scopes for your application to access specific resources.
- Save the configuration to generate your client ID and client secret.
These credentials will be used to authorize users and access the Chargebee API securely.
With your Chargebee test account and API keys set up, you're ready to start integrating and managing your product catalog using the Chargebee API.
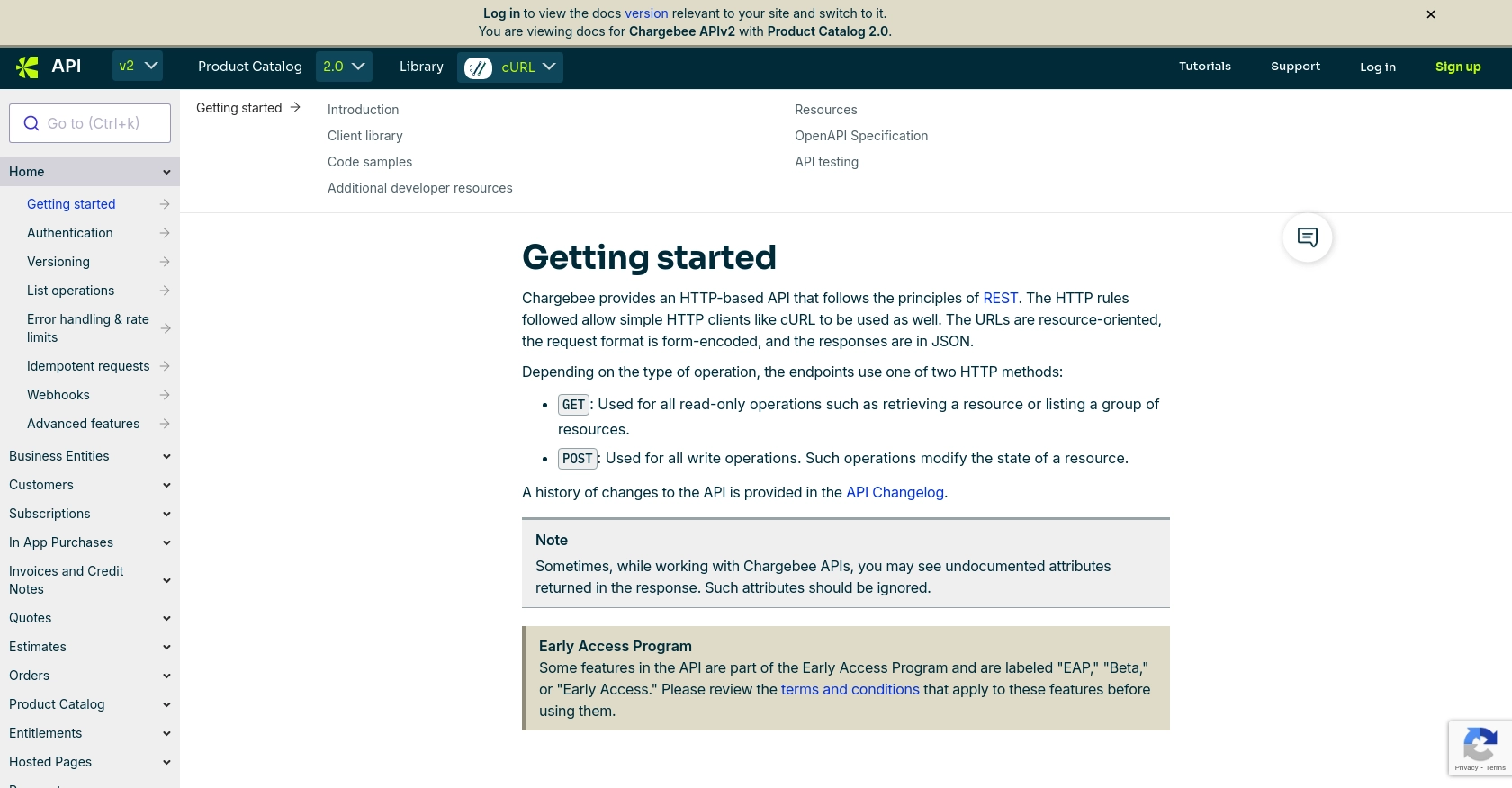
sbb-itb-96038d7
Making API Calls to Chargebee for Product Catalog Management Using Python
To effectively manage your product catalog with Chargebee's API, you'll need to make API calls using Python. This section will guide you through the necessary steps, including setting up your Python environment, writing the code to create or update items, and handling responses and errors.
Setting Up Your Python Environment for Chargebee API Integration
Before making API calls, ensure you have the following prerequisites installed on your machine:
- Python 3.11.1 or later
- The Python package installer, pip
Next, install the requests
library, which will be used to make HTTP requests to the Chargebee API:
pip install requests
Creating or Updating Items in Chargebee Using Python
To create or update items in your Chargebee product catalog, follow these steps:
Creating a New Item
Create a file named create_item.py
and add the following code:
import requests
# Set the API endpoint and authentication
url = "https://{your-site}.chargebee.com/api/v2/items"
api_key = "your_api_key"
# Define the item details
item_data = {
"id": "silver",
"name": "Silver Plan",
"type": "plan",
"item_applicability": "all"
}
# Make a POST request to create the item
response = requests.post(url, auth=(api_key, ''), data=item_data)
# Check the response status
if response.status_code == 200:
print("Item created successfully:", response.json())
else:
print("Failed to create item:", response.json())
Replace your_api_key
with your actual API key. This code sends a POST request to create a new item in Chargebee. If successful, it prints the item details.
Updating an Existing Item
Create a file named update_item.py
and add the following code:
import requests
# Set the API endpoint and authentication
url = "https://{your-site}.chargebee.com/api/v2/items/silver"
api_key = "your_api_key"
# Define the updated item details
update_data = {
"description": "Updated Silver Plan",
"enabled_for_checkout": False
}
# Make a POST request to update the item
response = requests.post(url, auth=(api_key, ''), data=update_data)
# Check the response status
if response.status_code == 200:
print("Item updated successfully:", response.json())
else:
print("Failed to update item:", response.json())
This code updates an existing item by sending a POST request with the new details. Ensure the item ID in the URL matches the item you wish to update.
Handling Responses and Errors from Chargebee API
Chargebee's API provides detailed error messages and status codes. Here are some common scenarios:
- Success (2XX): The API call was successful. Check the response data for details.
- Client Error (4XX): The request was invalid. Review the error message for specifics, such as missing parameters or invalid values.
- Server Error (5XX): An issue occurred on Chargebee's end. Retry the request after some time.
For more information on error handling, refer to the Chargebee API documentation.
Verifying API Call Success in Chargebee Dashboard
After making API calls, verify the changes in your Chargebee dashboard:
- Log in to your Chargebee account.
- Navigate to the 'Product Catalog' section to view the created or updated items.
This ensures that your API calls have been executed correctly and the product catalog reflects the intended changes.
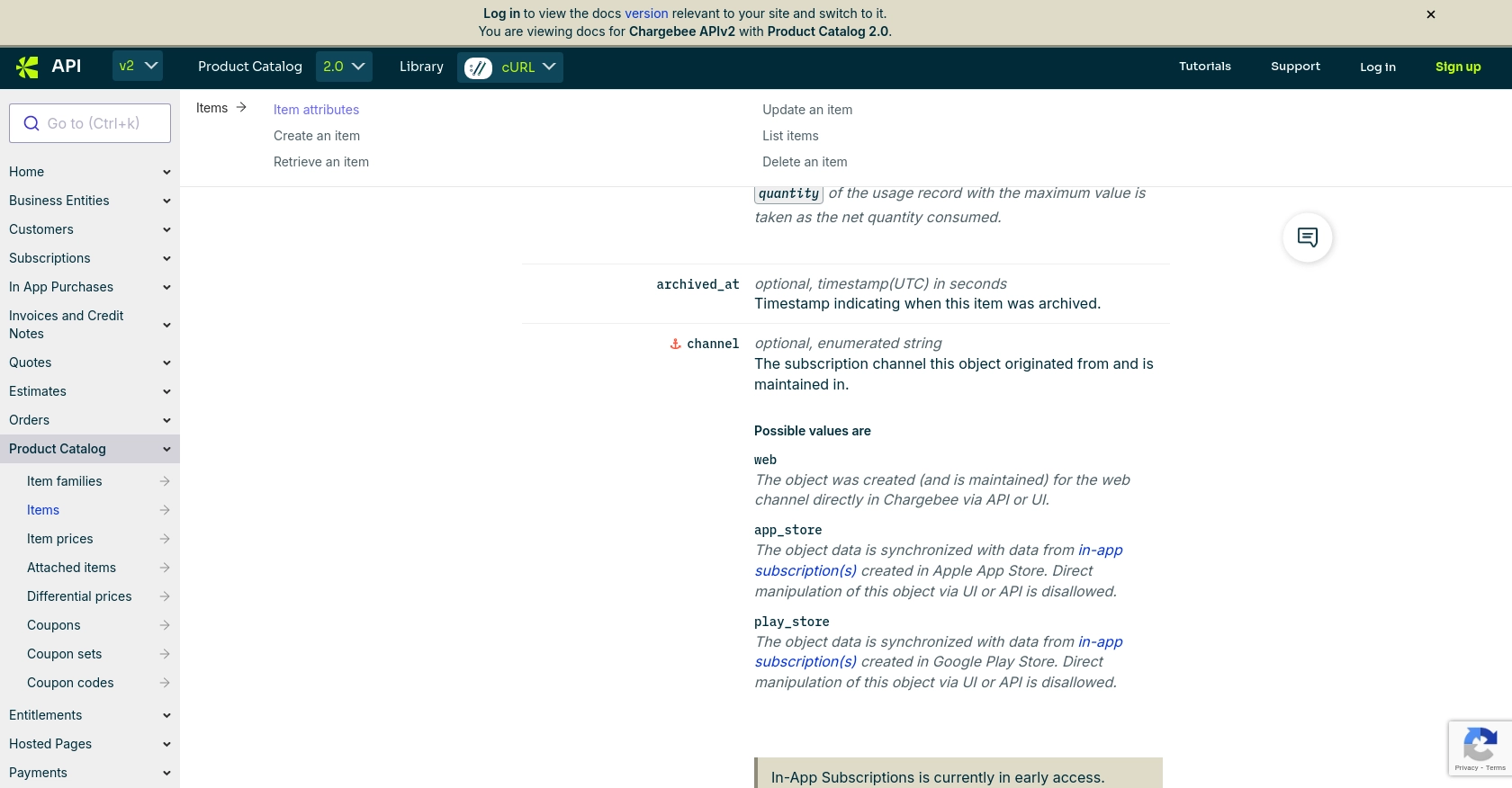
Conclusion and Best Practices for Chargebee API Integration
Integrating with the Chargebee API for managing product catalogs can significantly enhance your subscription management capabilities. By automating the creation and updating of items such as plans, addons, and charges, you ensure consistency and efficiency across your billing operations.
Best Practices for Secure and Efficient Chargebee API Usage
- Secure API Key Management: Store your API keys securely and avoid including them in your codebase. Regularly rotate keys and limit their scope to minimize security risks.
- Handle Rate Limits: Chargebee imposes rate limits on API requests. For test sites, the limit is approximately 750 API calls every 5 minutes, and for live sites, it's around 150 calls per minute. Implement exponential backoff strategies to handle HTTP 429 errors gracefully. For more details, refer to the Chargebee API documentation.
- Error Handling: Implement robust error handling to manage different HTTP status codes. Use Chargebee's detailed error messages to guide your response strategies.
- Data Standardization: Ensure that data fields are standardized across your applications to maintain consistency in your product catalog.
Streamlining Integration with Endgrate
While integrating with Chargebee's API can be powerful, managing multiple integrations can be complex and time-consuming. Endgrate offers a unified API solution that simplifies this process by providing a single endpoint to connect with various platforms, including Chargebee.
By leveraging Endgrate, you can save time and resources, allowing your team to focus on core product development. With Endgrate, you build once for each use case, rather than multiple times for different integrations, ensuring an intuitive experience for your customers.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate.
Read More
Ready to get started?