How to Get Contacts with the Microsoft Dynamics 365 API in Python
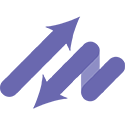
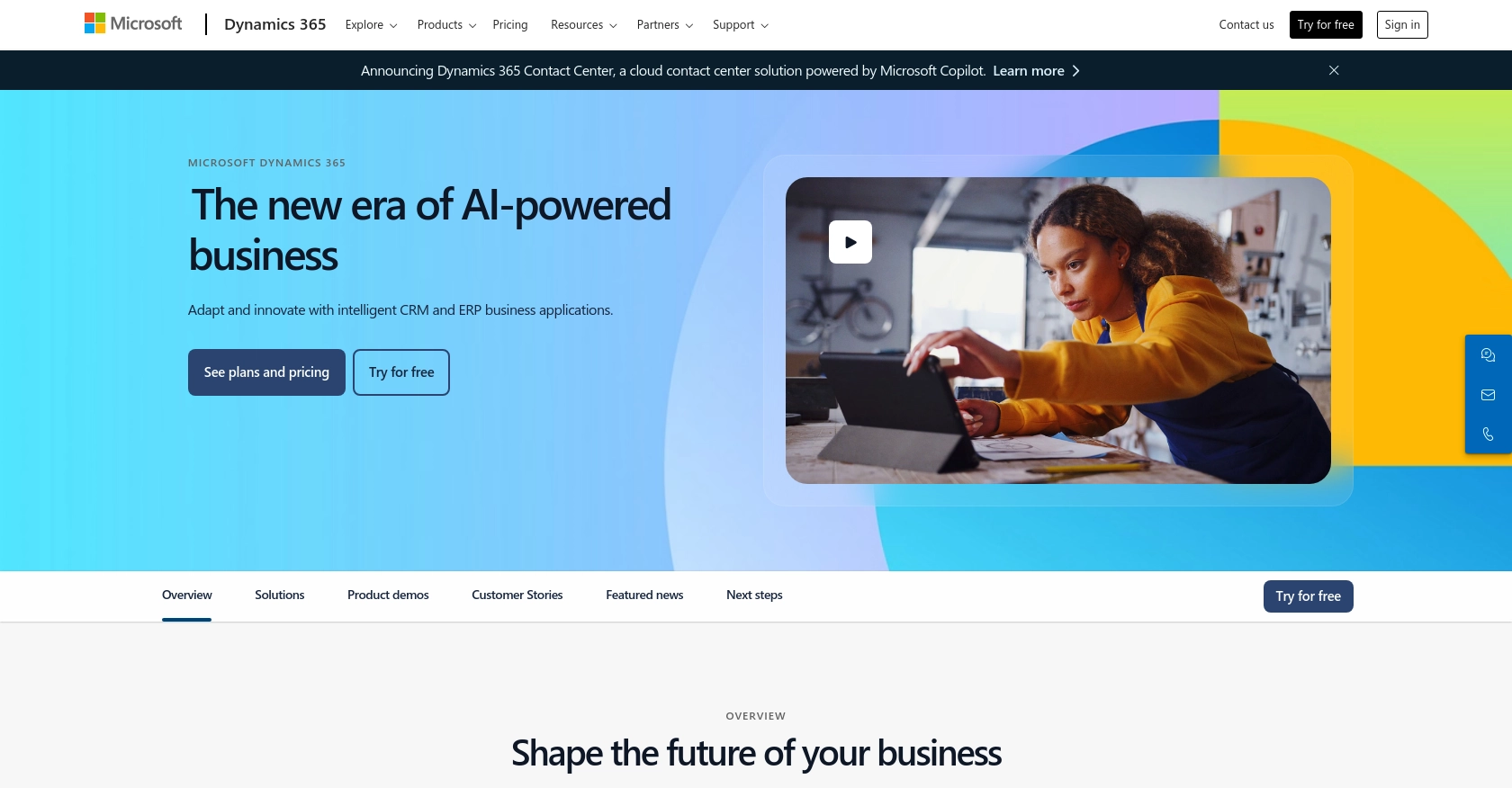
Introduction to Microsoft Dynamics 365 API
Microsoft Dynamics 365 is a comprehensive suite of business applications designed to enhance customer engagement and streamline operations across various industries. It integrates CRM and ERP capabilities, providing businesses with tools for sales, customer service, finance, and operations.
Developers may want to connect with the Microsoft Dynamics 365 API to access and manage customer data, such as contacts, to improve business processes and customer interactions. For example, a developer could use the API to retrieve contact information and integrate it with other systems for personalized marketing campaigns or customer support automation.
Setting Up Your Microsoft Dynamics 365 Test/Sandbox Account
Before you can start integrating with the Microsoft Dynamics 365 API, you'll need to set up a test or sandbox account. This environment allows you to safely develop and test your applications without affecting live data.
Creating a Microsoft Dynamics 365 Sandbox Account
If you don't already have a Microsoft Dynamics 365 account, you can sign up for a free trial or a sandbox account. Follow these steps to get started:
- Visit the Microsoft Dynamics 365 Free Trial page.
- Fill out the necessary information to create your account.
- Once your account is set up, log in to the Microsoft Dynamics 365 dashboard.
Registering an Application for OAuth Authentication
To interact with the Microsoft Dynamics 365 API, you'll need to register an application in your Microsoft Entra ID tenant. This registration will provide you with the necessary credentials to authenticate API requests.
- Navigate to the Azure Portal and sign in with your Microsoft account.
- Go to the "Azure Active Directory" section and select "App registrations."
- Click on "New registration" and fill in the required details, such as the application name and redirect URI.
- Once registered, note down the Application (client) ID and Directory (tenant) ID.
- Under "Certificates & secrets," create a new client secret and save it securely.
Configuring API Permissions
After registering your application, you need to configure the necessary API permissions to access Microsoft Dynamics 365 data:
- In the Azure Portal, navigate to your registered application.
- Select "API permissions" and click on "Add a permission."
- Choose "Dynamics CRM" and select the permissions required for accessing contacts, such as "user_impersonation."
- Grant admin consent for the permissions if required.
With these steps completed, you now have a sandbox account and an application registered with the necessary permissions to interact with the Microsoft Dynamics 365 API using OAuth authentication. For more detailed information on authentication, refer to the Microsoft OAuth Authentication Documentation.
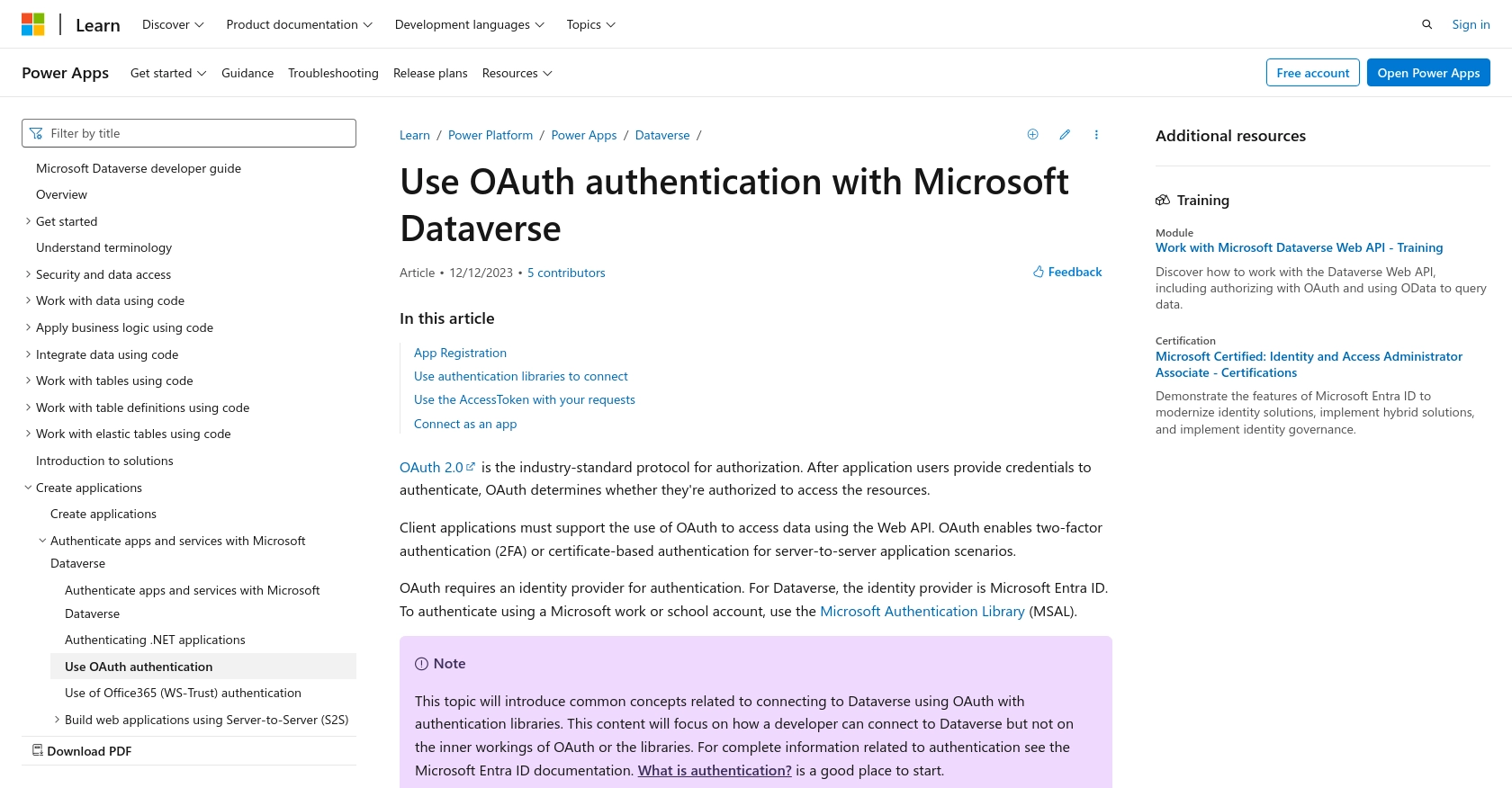
sbb-itb-96038d7
Making API Calls to Retrieve Contacts from Microsoft Dynamics 365 Using Python
To interact with the Microsoft Dynamics 365 API and retrieve contact information, you'll need to use Python. This section will guide you through the process of setting up your environment, writing the code, and executing the API call.
Setting Up Your Python Environment for Microsoft Dynamics 365 API Integration
Before making API calls, ensure you have the following prerequisites installed on your machine:
- Python 3.11.1 or later
- The Python package installer, pip
Next, install the necessary libraries by running the following command in your terminal:
pip install requests msal
The requests
library will help you make HTTP requests, while msal
(Microsoft Authentication Library) will handle OAuth authentication.
Writing Python Code to Authenticate and Retrieve Contacts from Microsoft Dynamics 365
Create a new Python file named get_dynamics_contacts.py
and add the following code:
import requests
from msal import ConfidentialClientApplication
# Set up your credentials
client_id = 'Your_Client_ID'
client_secret = 'Your_Client_Secret'
tenant_id = 'Your_Tenant_ID'
authority_url = f'https://login.microsoftonline.com/{tenant_id}'
scope = ['https://yourorg.crm.dynamics.com/.default']
# Authenticate using MSAL
app = ConfidentialClientApplication(client_id, authority=authority_url, client_credential=client_secret)
token_response = app.acquire_token_for_client(scopes=scope)
# Check if authentication was successful
if 'access_token' in token_response:
access_token = token_response['access_token']
else:
raise Exception("Authentication failed.")
# Set the API endpoint and headers
endpoint = 'https://yourorg.api.crm.dynamics.com/api/data/v9.2/contacts'
headers = {
'Authorization': f'Bearer {access_token}',
'OData-MaxVersion': '4.0',
'OData-Version': '4.0',
'Accept': 'application/json'
}
# Make a GET request to retrieve contacts
response = requests.get(endpoint, headers=headers)
# Check if the request was successful
if response.status_code == 200:
contacts = response.json()
for contact in contacts['value']:
print(contact['fullname'])
else:
print(f"Failed to retrieve contacts: {response.status_code} - {response.text}")
Replace Your_Client_ID
, Your_Client_Secret
, and Your_Tenant_ID
with your actual credentials. The code above authenticates using OAuth, retrieves an access token, and makes a GET request to the Microsoft Dynamics 365 API to fetch contact data.
Executing the Python Script and Verifying Results
Run the script using the following command:
python get_dynamics_contacts.py
If successful, the script will print the full names of the contacts retrieved from your Microsoft Dynamics 365 sandbox environment. You can verify the results by checking the contact list in your sandbox account.
Handling Errors and Understanding Response Codes
It's crucial to handle potential errors when making API calls. Common HTTP status codes include:
- 200 OK: The request was successful.
- 401 Unauthorized: Authentication failed. Check your credentials.
- 403 Forbidden: You do not have permission to access the resource.
- 404 Not Found: The requested resource does not exist.
For more detailed information on error handling, refer to the Microsoft Dynamics 365 API documentation.
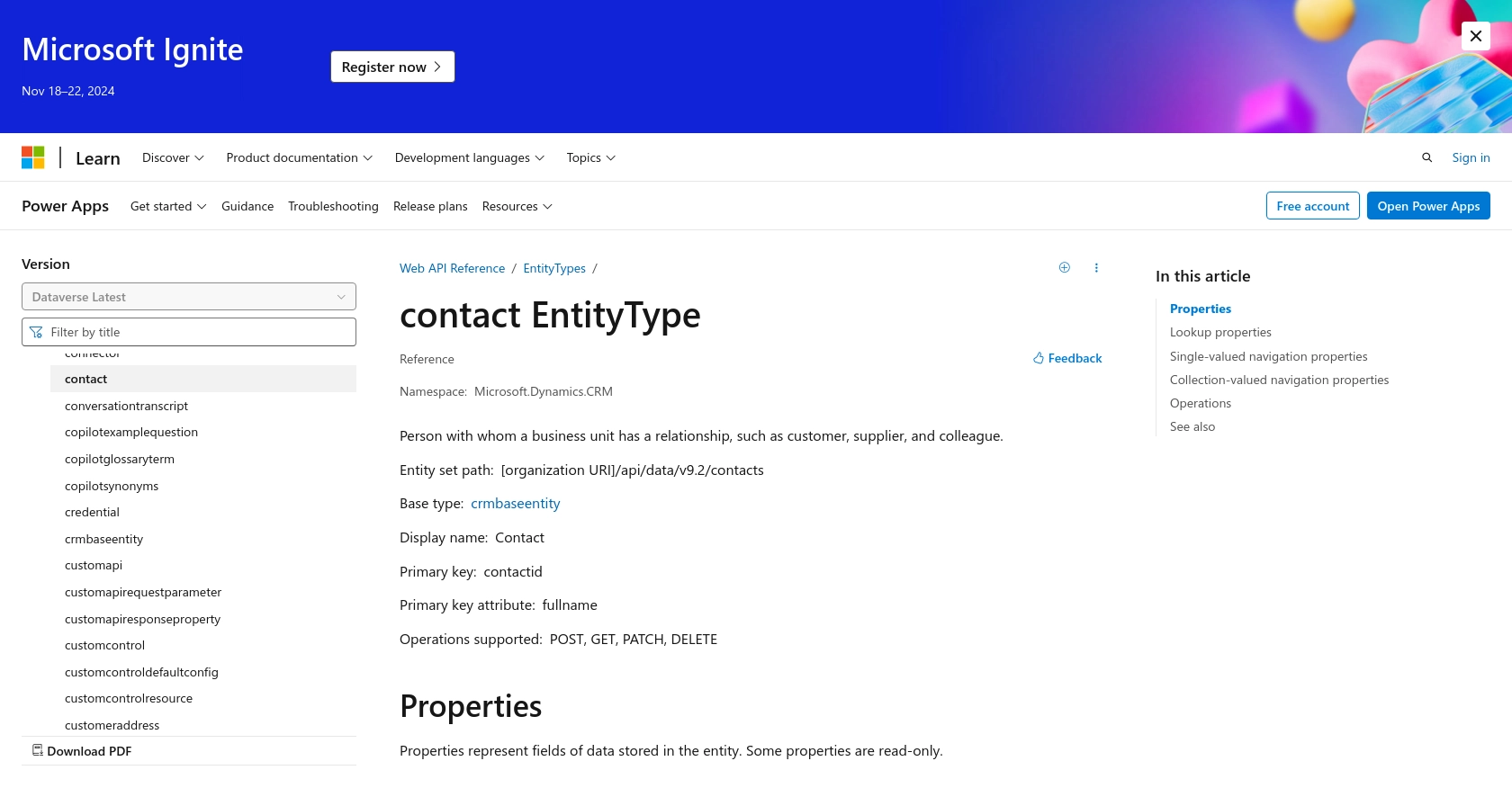
Conclusion and Best Practices for Microsoft Dynamics 365 API Integration
Integrating with the Microsoft Dynamics 365 API using Python provides a powerful way to access and manage customer data, enhancing your business processes and customer interactions. By following the steps outlined in this guide, you can efficiently retrieve contact information and integrate it with other systems.
Best Practices for Secure and Efficient API Usage
- Securely Store Credentials: Always store your client ID, client secret, and access tokens securely. Consider using environment variables or secure vaults to manage sensitive information.
- Handle Rate Limiting: Be aware of the API's rate limits to avoid throttling. Implement retry logic with exponential backoff to handle rate limit responses gracefully.
- Data Standardization: Ensure that data retrieved from Microsoft Dynamics 365 is standardized and transformed as needed to fit your application's requirements.
- Error Handling: Implement robust error handling to manage different HTTP status codes and potential exceptions during API calls.
Streamline Your Integrations with Endgrate
While integrating with Microsoft Dynamics 365 can be highly beneficial, managing multiple integrations can be complex and time-consuming. Endgrate simplifies this process by offering a unified API endpoint that connects to various platforms, including Microsoft Dynamics 365.
By using Endgrate, you can save time and resources, allowing you to focus on your core product. Build once for each use case and enjoy an intuitive integration experience for your customers. Visit Endgrate to learn more about how you can streamline your integrations and enhance your business operations.
Read More
- https://endgrate.com/provider/microsoftdynamics365
- https://learn.microsoft.com/en-us/power-apps/developer/data-platform/authenticate-oauth
- https://learn.microsoft.com/en-us/graph/use-the-api
- https://learn.microsoft.com/en-us/power-apps/developer/data-platform/webapi/reference/contact?view=dataverse-latest
Ready to get started?