How to Get Product Variants with the BigCommerce API in Python
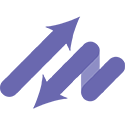
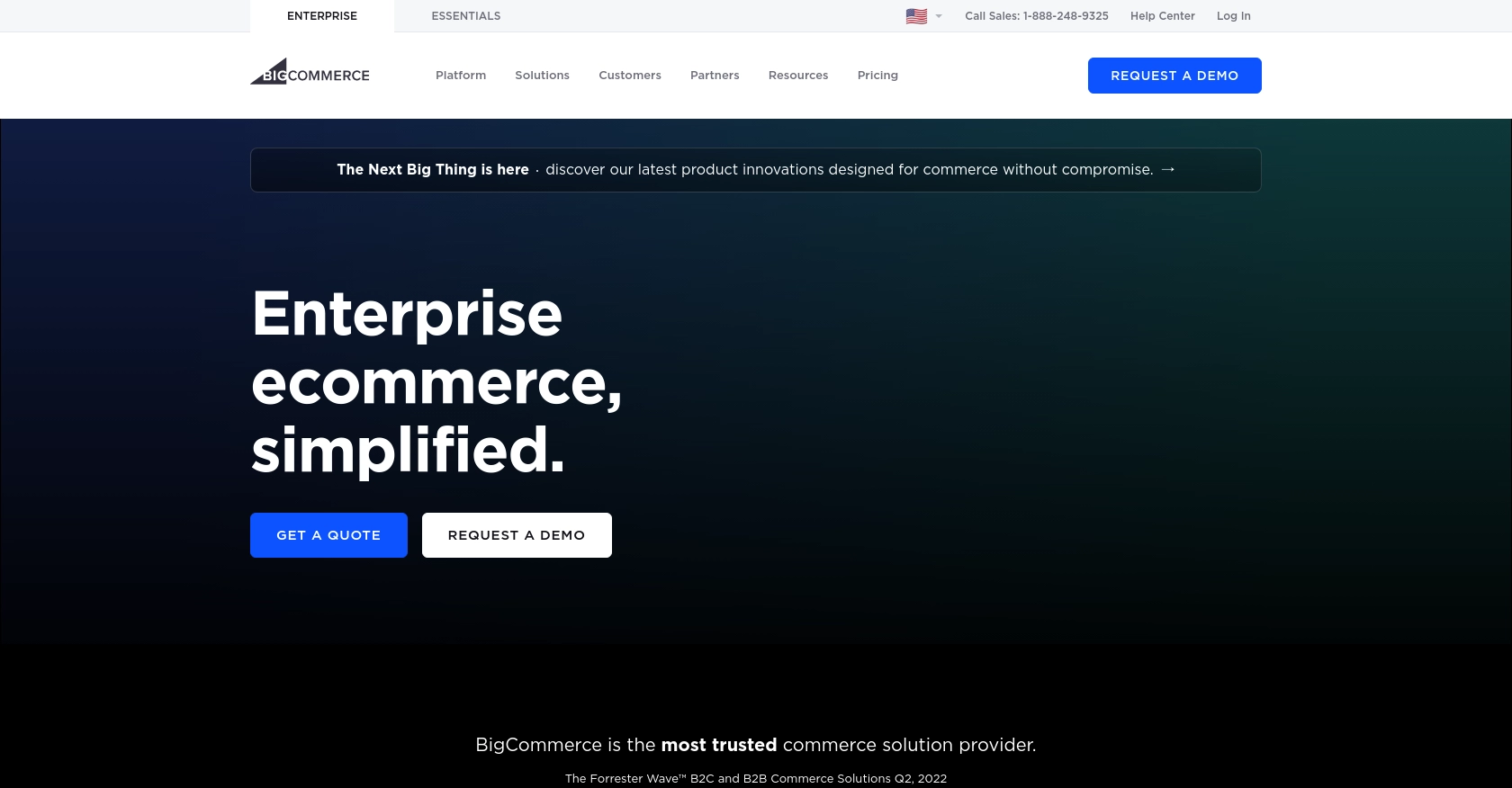
Introduction to BigCommerce API for Product Variants
BigCommerce is a robust e-commerce platform that empowers businesses to create and manage online stores with ease. It offers a wide range of features, including product management, order processing, and customer engagement tools, making it a popular choice for businesses looking to expand their online presence.
Integrating with the BigCommerce API allows developers to efficiently manage store data, including product variants. Product variants are essential for businesses offering products in different sizes, colors, or configurations. For example, a developer might use the BigCommerce API to retrieve all product variants for inventory management or to display detailed product options on a storefront.
This article will guide you through the process of using Python to interact with the BigCommerce API, specifically focusing on retrieving product variants. By the end of this tutorial, you'll be equipped with the knowledge to seamlessly integrate BigCommerce's product variant data into your applications.
Setting Up Your BigCommerce Test Account for API Integration
Before you can start interacting with the BigCommerce API to retrieve product variants, you'll need to set up a test account. This will allow you to safely experiment with API calls without affecting a live store. BigCommerce provides a sandbox environment specifically for developers to test their integrations.
Creating a BigCommerce Sandbox Account
To begin, you'll need to create a sandbox account on BigCommerce. Follow these steps to set up your account:
- Visit the BigCommerce Developer Portal.
- Sign up for a developer account if you haven't already. This will give you access to the sandbox environment.
- Once registered, log in to your developer account and navigate to the sandbox store creation section.
- Create a new sandbox store by following the on-screen instructions. This store will be used for testing your API integrations.
Generating API Credentials for BigCommerce
After setting up your sandbox store, you'll need to generate API credentials to authenticate your requests. BigCommerce uses OAuth-based authentication, which involves creating an API account to obtain access tokens.
- In your BigCommerce control panel, go to Advanced Settings and select API Accounts.
- Click on Create API Account and choose V2/V3 API Token.
- Fill in the required details, such as the name and permissions for the API account. Ensure you grant the necessary scopes for accessing product variants.
- Once created, you'll receive an API Path, Client ID, and Access Token. Keep these credentials secure as you'll need them to authenticate your API requests.
Configuring OAuth Scopes for BigCommerce API
It's crucial to configure the OAuth scopes correctly to ensure your API account has the necessary permissions. For retrieving product variants, make sure the following scopes are included:
- Products - Read-only access to product data.
- Inventory - Access to inventory levels and management.
Refer to the BigCommerce Authentication Documentation for more details on setting up OAuth scopes.
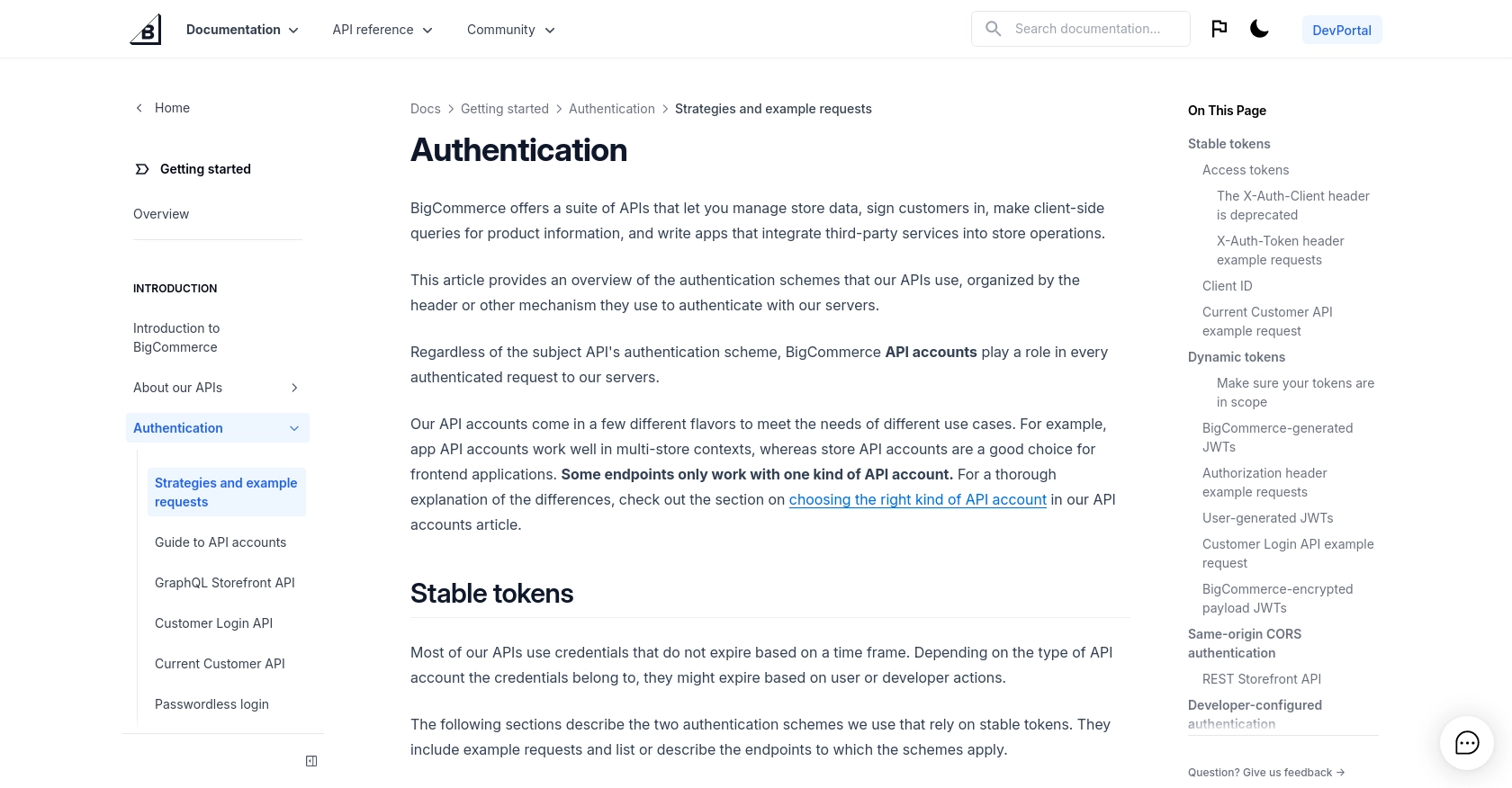
sbb-itb-96038d7
How to Make API Calls to Retrieve Product Variants from BigCommerce Using Python
To interact with the BigCommerce API and retrieve product variants, you'll need to use Python, a versatile programming language known for its simplicity and readability. This section will guide you through the necessary steps to set up your environment, write the code, and execute API calls to fetch product variant data.
Setting Up Your Python Environment for BigCommerce API Integration
Before making API calls, ensure you have the following prerequisites installed on your machine:
- Python 3.11.1 or later
- The Python package installer, pip
Once you have these installed, open your terminal or command prompt and install the requests
library, which will be used to make HTTP requests:
pip install requests
Writing Python Code to Fetch Product Variants from BigCommerce
Create a new Python file named get_product_variants.py
and add the following code:
import requests
# Define the API endpoint and headers
store_hash = 'your_store_hash'
product_id = 'your_product_id'
endpoint = f"https://api.bigcommerce.com/stores/{store_hash}/v3/catalog/products/{product_id}/variants"
headers = {
"X-Auth-Token": "your_access_token",
"Accept": "application/json"
}
# Make a GET request to the API
response = requests.get(endpoint, headers=headers)
# Check if the request was successful
if response.status_code == 200:
data = response.json()
# Loop through the product variants and print their details
for variant in data['data']:
print(f"Variant ID: {variant['id']}, SKU: {variant['sku']}, Price: {variant['price']}")
else:
print(f"Failed to retrieve product variants. Status code: {response.status_code}")
Replace your_store_hash
, your_product_id
, and your_access_token
with your actual store hash, product ID, and access token obtained from your BigCommerce API account.
Executing the Python Script to Retrieve BigCommerce Product Variants
Run the script from your terminal or command prompt using the following command:
python get_product_variants.py
If successful, you should see a list of product variants with their IDs, SKUs, and prices printed to the console. This confirms that your API call was successful and that you can now access product variant data from BigCommerce.
Handling Errors and Verifying API Call Success
To ensure your API requests are successful, always check the response status code. A status code of 200 indicates success, while other codes may indicate errors. Refer to the BigCommerce API documentation for more details on error codes and their meanings.
Additionally, verify the retrieved data by checking your BigCommerce sandbox account to ensure the product variants match the data returned by the API.
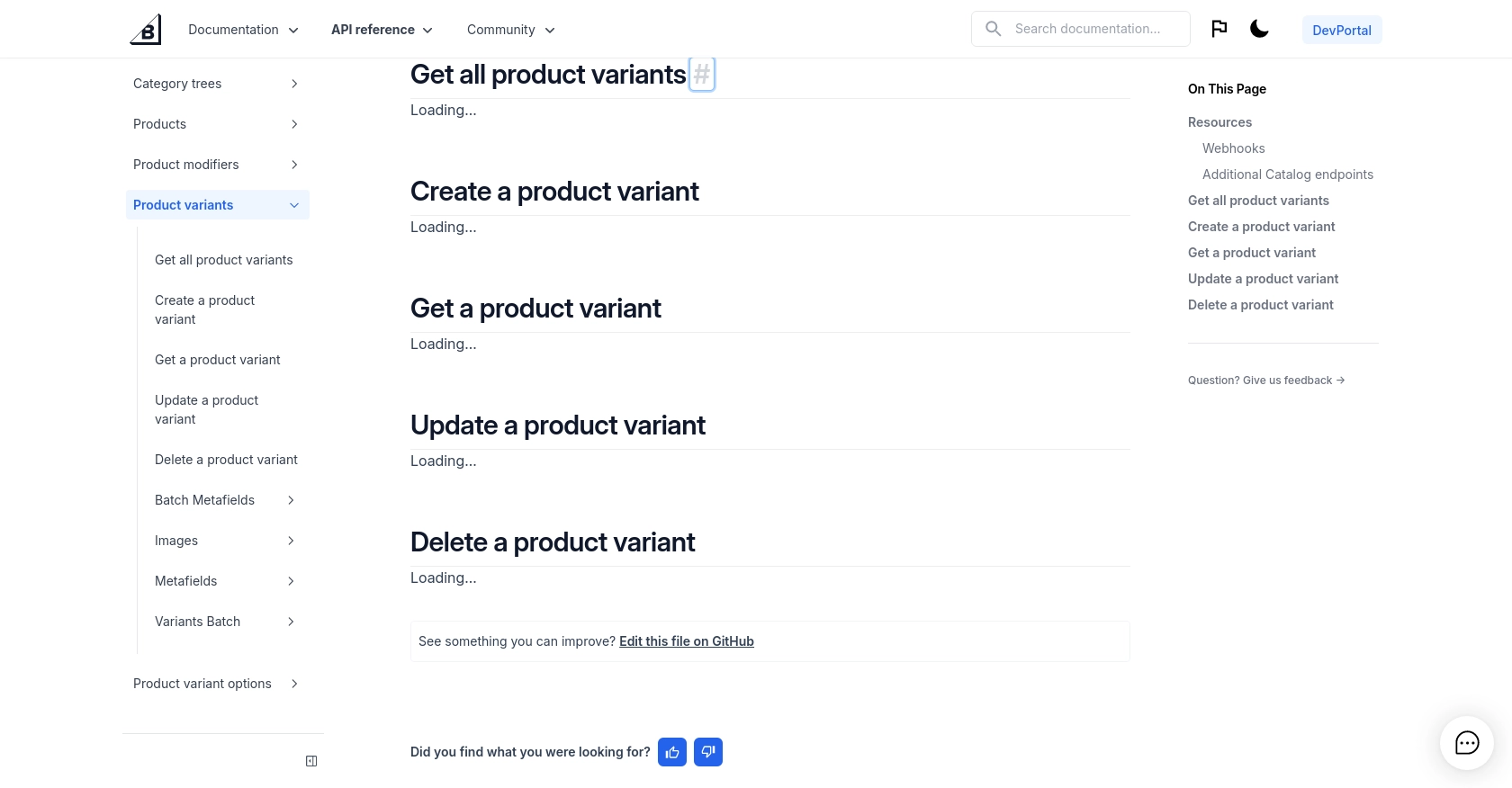
Conclusion and Best Practices for Integrating BigCommerce API with Python
Integrating with the BigCommerce API using Python provides a powerful way to manage and retrieve product variant data, enhancing your e-commerce operations. By following the steps outlined in this guide, you can efficiently access and manipulate product variants, ensuring your store's inventory and product offerings are always up-to-date.
Best Practices for Secure and Efficient BigCommerce API Integration
- Securely Store API Credentials: Always keep your API credentials, such as the access token, secure. Avoid hardcoding them in your scripts and consider using environment variables or secure vaults.
- Handle API Rate Limits: Be mindful of BigCommerce's API rate limits to avoid throttling. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Validate API Responses: Always check the status codes and response data to ensure successful API interactions. Implement error handling to manage unexpected responses or failures.
- Optimize Data Handling: When dealing with large datasets, consider paginating your requests to improve performance and reduce load on the server.
Enhance Your Integration Strategy with Endgrate
While integrating with the BigCommerce API can be straightforward, managing multiple integrations across different platforms can become complex. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including BigCommerce. This allows you to focus on your core product while outsourcing integration complexities.
Explore how Endgrate can streamline your integration efforts, saving you time and resources. Visit Endgrate to learn more about how you can enhance your integration strategy and deliver a seamless experience to your customers.
Read More
Ready to get started?