How to Get Products (Professional/Enterprise Only) with the Hubspot API in PHP
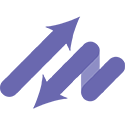
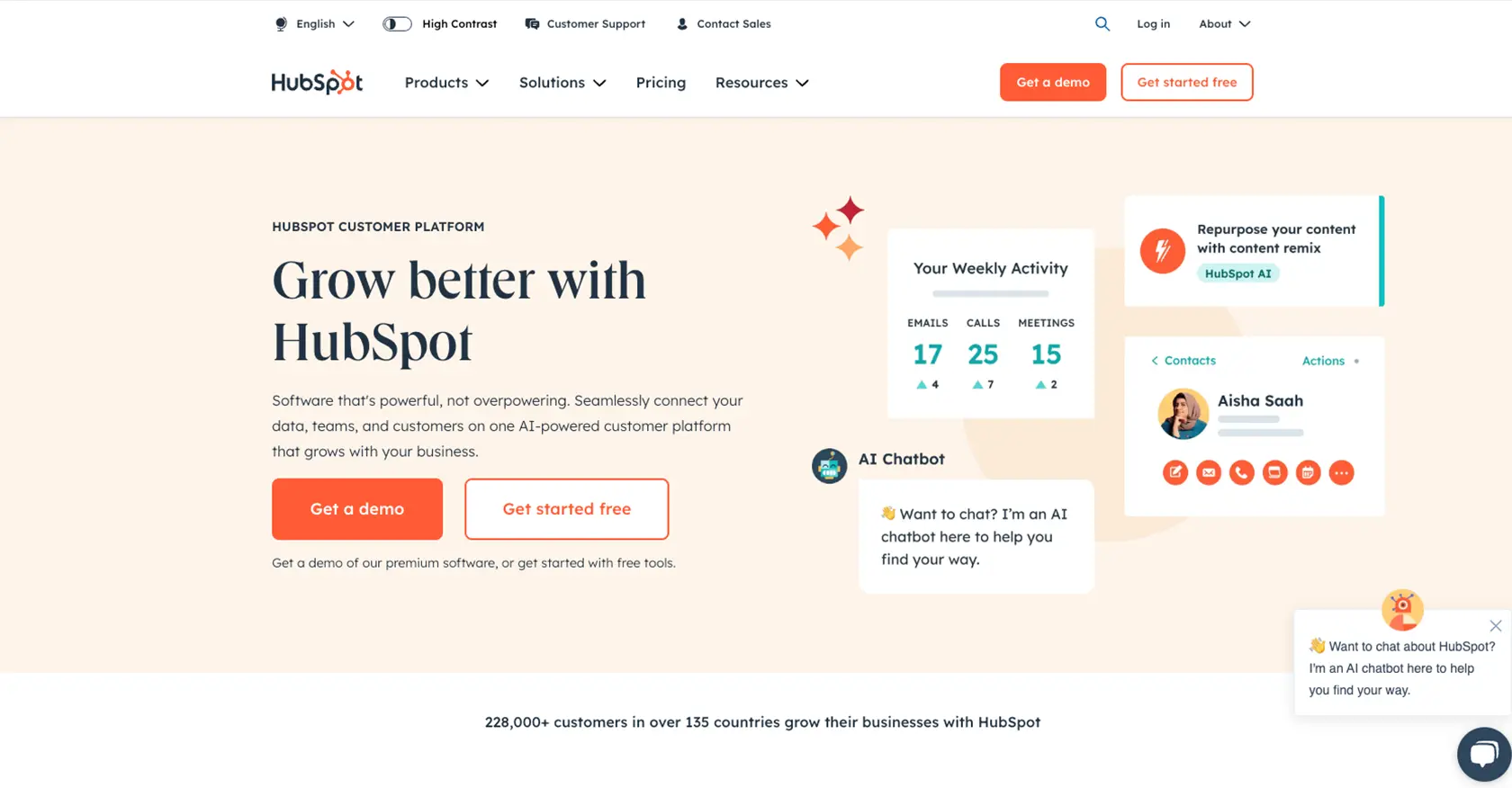
Introduction to HubSpot API for Product Management
HubSpot is a comprehensive CRM platform that offers a suite of tools to help businesses manage their marketing, sales, and customer service efforts. Known for its user-friendly interface and robust features, HubSpot is a popular choice for enterprises looking to streamline their operations.
For developers, integrating with HubSpot's API can unlock powerful capabilities, such as managing product data within the CRM. This is particularly useful for businesses that need to maintain a consistent product catalog across various platforms. By leveraging the HubSpot API, developers can automate the retrieval and management of product information, ensuring that all systems are up-to-date and synchronized.
In this article, we'll explore how to use PHP to interact with HubSpot's API to access product data, specifically for Professional and Enterprise accounts. This integration can be beneficial for scenarios like updating product details in real-time or syncing product information with an e-commerce platform.
Setting Up Your HubSpot Developer Account and Sandbox Environment
Before you can start interacting with the HubSpot API to manage products, you'll need to set up a developer account and create a sandbox environment. This will allow you to test API calls without affecting live data.
Step 1: Create a HubSpot Developer Account
If you don't already have a HubSpot developer account, you'll need to create one. Follow these steps:
- Visit the HubSpot Developer Portal.
- Click on "Create a developer account" and fill out the necessary information.
- Once your account is created, log in to access the developer dashboard.
Step 2: Set Up a HubSpot Sandbox Account
A sandbox account allows you to test your integrations in a safe environment. Here's how to set it up:
- In your developer dashboard, navigate to the "Test Accounts" section.
- Click on "Create a test account" to generate a sandbox environment.
- Follow the prompts to configure your sandbox with the necessary settings.
Step 3: Create a HubSpot App for OAuth Authentication
Since the HubSpot API uses OAuth for authentication, you'll need to create an app to obtain the necessary credentials:
- In your developer account, go to the "Apps" section and click "Create an app."
- Fill in the app details, such as name and description.
- Navigate to the "Auth" tab and select "OAuth 2.0" as the authentication method.
- Specify the required scopes for accessing product data, such as
e-commerce
for Professional and Enterprise accounts. - Save your app settings to generate a client ID and client secret.
Step 4: Obtain OAuth Access Token
With your app set up, you can now obtain an OAuth access token:
- Direct users to the authorization URL provided in your app settings to obtain an authorization code.
- Exchange the authorization code for an access token by making a POST request to the HubSpot token endpoint.
// Example PHP code to exchange authorization code for access token
$client_id = 'your_client_id';
$client_secret = 'your_client_secret';
$redirect_uri = 'your_redirect_uri';
$authorization_code = 'authorization_code_received';
$url = 'https://api.hubapi.com/oauth/v1/token';
$data = [
'grant_type' => 'authorization_code',
'client_id' => $client_id,
'client_secret' => $client_secret,
'redirect_uri' => $redirect_uri,
'code' => $authorization_code
];
$options = [
'http' => [
'header' => "Content-type: application/x-www-form-urlencoded\r\n",
'method' => 'POST',
'content' => http_build_query($data),
],
];
$context = stream_context_create($options);
$result = file_get_contents($url, false, $context);
$response = json_decode($result, true);
$access_token = $response['access_token'];
Store the access token securely, as it will be used to authenticate your API requests.
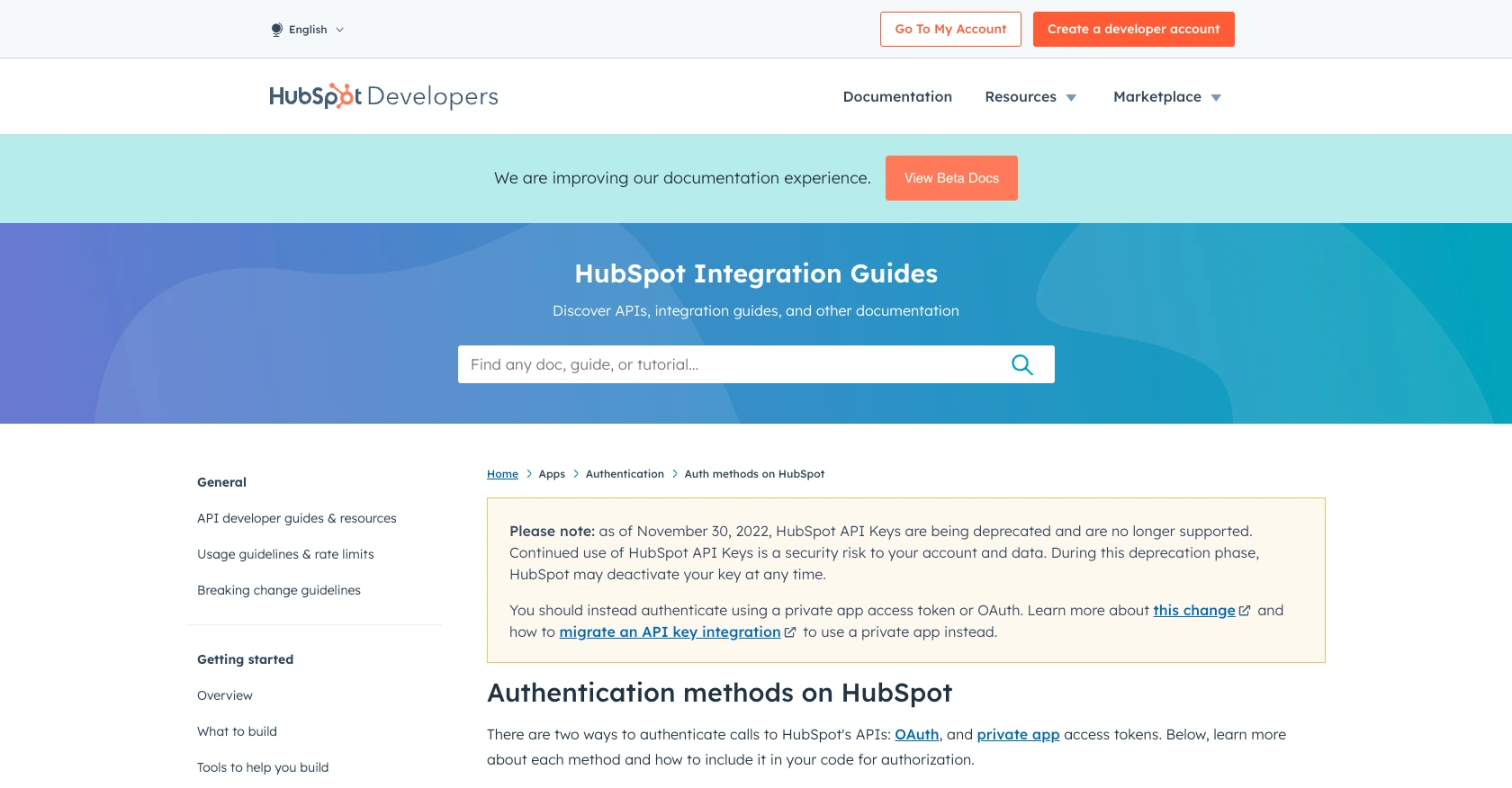
sbb-itb-96038d7
Making API Calls to Retrieve Products from HubSpot Using PHP
In this section, we'll guide you through the process of making API calls to retrieve product data from HubSpot using PHP. This involves setting up your PHP environment, installing necessary dependencies, and writing the code to interact with the HubSpot API.
Setting Up Your PHP Environment for HubSpot API Integration
Before making API calls, ensure your PHP environment is properly configured. You'll need PHP 7.4 or higher and the Composer package manager to install dependencies.
- Ensure PHP is installed on your system. You can verify this by running
php -v
in your terminal. - Install Composer by following the instructions on the Composer website.
Installing the HubSpot PHP Client Library
The HubSpot PHP client library simplifies API interactions. Install it using Composer:
composer require hubspot/api-client
Writing PHP Code to Retrieve Products from HubSpot
With your environment set up, you can now write PHP code to retrieve products from HubSpot. The following example demonstrates how to make a GET request to the HubSpot API to fetch product data.
require 'vendor/autoload.php';
use HubSpot\Factory;
use HubSpot\Client\Crm\Products\ApiException;
// Initialize the HubSpot client
$client = Factory::createWithAccessToken('your_access_token');
try {
// Fetch products
$productsPage = $client->crm()->products()->basicApi()->getPage();
$products = $productsPage->getResults();
// Display product information
foreach ($products as $product) {
echo "Product ID: " . $product->getId() . "\n";
echo "Name: " . $product->getProperties()['name'] . "\n";
echo "Price: " . $product->getProperties()['price'] . "\n\n";
}
} catch (ApiException $e) {
echo "Exception when calling HubSpot API: ", $e->getMessage(), PHP_EOL;
}
Replace your_access_token
with the OAuth access token obtained earlier. This script initializes the HubSpot client, retrieves a list of products, and prints their details.
Verifying API Call Success and Handling Errors
After running the script, verify the output to ensure the API call was successful. The product details should match those in your HubSpot sandbox account. If an error occurs, the script will catch and display the exception message.
HubSpot's API returns specific error codes that can help diagnose issues. For example, a 401 error indicates an authentication problem, while a 429 error signifies rate limit exhaustion. Refer to the HubSpot API documentation for more details on error codes and handling.
.webp)
Best Practices for Secure and Efficient HubSpot API Integration
Successfully integrating with the HubSpot API requires attention to best practices to ensure security and efficiency. Here are some key recommendations:
Securely Storing OAuth Credentials
It's crucial to store your OAuth credentials securely. Avoid hardcoding them in your source code. Instead, use environment variables or secure vaults to manage sensitive information like client IDs, client secrets, and access tokens.
Handling HubSpot API Rate Limits
HubSpot imposes rate limits on API requests to ensure fair usage. For Professional and Enterprise accounts, the limit is 150 requests per 10 seconds per private app. Implement request throttling and exponential backoff strategies to handle rate limit errors gracefully. For more details, refer to the HubSpot API usage guidelines.
Transforming and Standardizing Product Data
When retrieving product data from HubSpot, consider transforming and standardizing it to match your application's data model. This ensures consistency across different platforms and systems.
Regularly Refreshing OAuth Tokens
OAuth tokens have a limited lifespan. Implement a mechanism to refresh tokens automatically before they expire to maintain uninterrupted access to the HubSpot API. This involves handling the expires_in
parameter and using refresh tokens as needed.
Streamlining HubSpot Integrations with Endgrate
Building and maintaining integrations can be time-consuming and complex. Endgrate simplifies this process by providing a unified API endpoint that connects to multiple platforms, including HubSpot. By using Endgrate, you can:
- Save time and resources by outsourcing integration development and focusing on your core product.
- Build once for each use case instead of multiple times for different integrations.
- Offer an intuitive integration experience for your customers, enhancing their satisfaction and engagement.
Explore how Endgrate can transform your integration strategy by visiting Endgrate's website.
Read More
- https://endgrate.com/provider/hubspot
- https://developers.hubspot.com/docs/api/overview
- https://developers.hubspot.com/docs/api/intro-to-auth
- https://developers.hubspot.com/docs/api/usage-details
- https://developers.hubspot.com/docs/api/scopes
- https://developers.hubspot.com/docs/api/crm/products
- https://developers.hubspot.com/docs/api/crm/properties
Ready to get started?