How to Get Purchase Orders with the Sage 100 API in Javascript
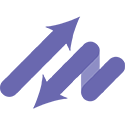
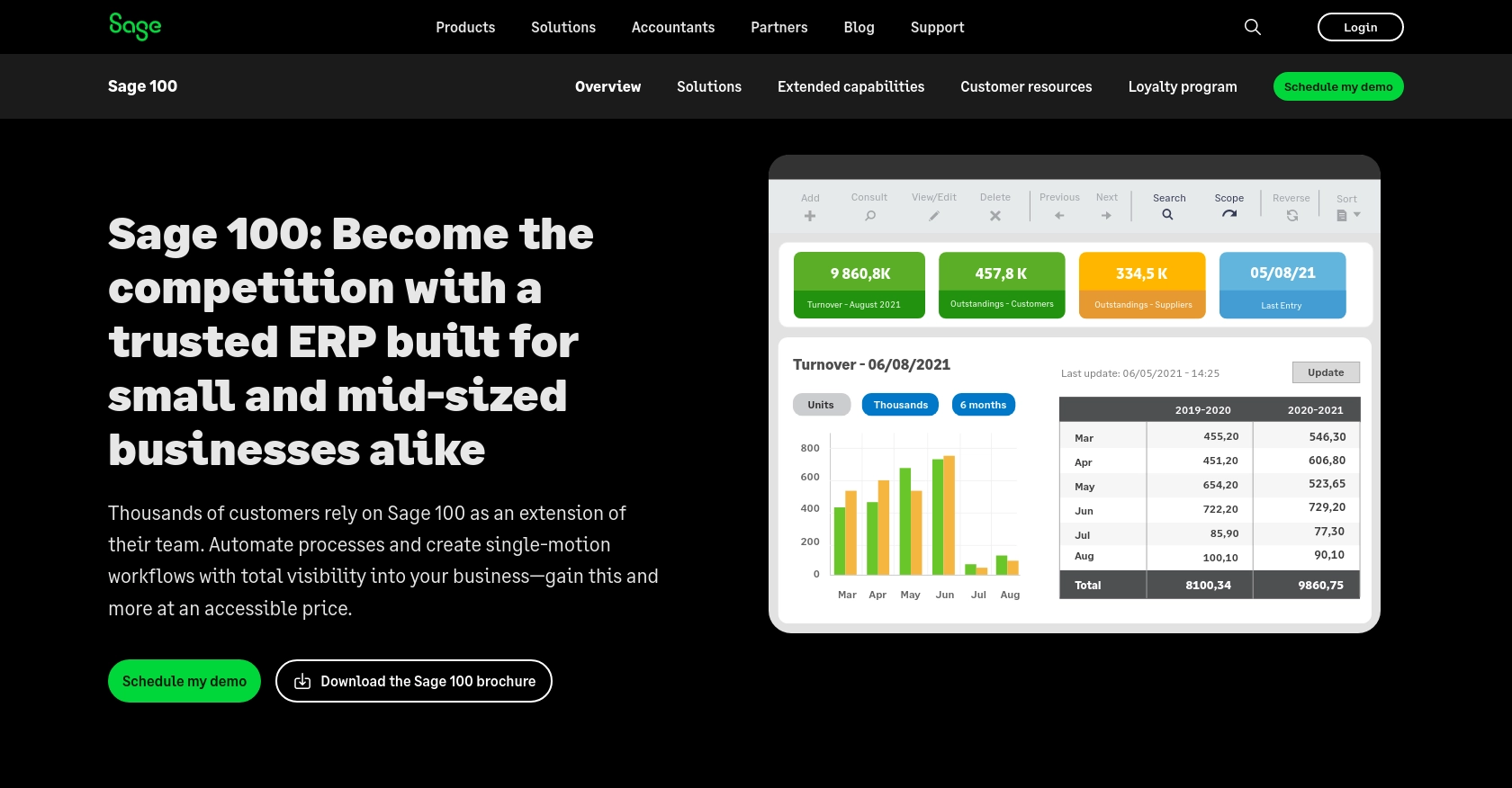
Introduction to Sage 100 API Integration
Sage 100 is a comprehensive ERP solution that provides robust tools for managing accounting, inventory, and business operations. It's widely used by businesses to streamline their financial processes and improve operational efficiency.
Integrating with the Sage 100 API allows developers to access and manage essential business data, such as purchase orders, directly from their applications. For example, a developer might want to retrieve purchase order information to automate inventory updates or generate detailed financial reports.
Setting Up a Sage 100 Test/Sandbox Account for API Integration
Before you can start interacting with the Sage 100 API, it's essential to set up a test or sandbox account. This allows you to safely experiment with API calls without affecting live data. Follow these steps to configure your Sage 100 environment for development purposes.
Installing and Configuring the Sage 100 ODBC Driver
To interact with the Sage 100 API, you need to install and configure the Sage 100 ODBC driver. This driver facilitates the connection between your application and the Sage 100 database.
- Ensure the Sage 100 ODBC driver is installed on your system. You can download it from the Sage website or contact your system administrator for assistance.
- Access the ODBC Data Source Administrator on your system and create a Data Source Name (DSN) that connects to the Sage 100 ERP system. Make sure to use the correct server, database, and authentication settings.
- For detailed instructions, refer to the Sage 100 ODBC Driver Configuration Guide.
Creating a Sage 100 Sandbox Environment
Setting up a sandbox environment is crucial for testing your API interactions without impacting real data. Here's how you can create a sandbox:
- Contact your Sage 100 administrator to request access to a sandbox environment. This may involve setting up a separate instance of Sage 100 specifically for testing purposes.
- Ensure that the sandbox environment mirrors your production setup as closely as possible to provide realistic testing conditions.
Configuring Sage 100 API Authentication
Sage 100 uses custom authentication for API access. Follow these steps to configure authentication for your sandbox account:
- In the Sage 100 interface, navigate to the Library Master module and select Setup > System Configuration.
- Under the ODBC Driver tab, enable the client/server ODBC driver by checking the appropriate box.
- Enter the server name or IP address where the ODBC application or service is running.
- Specify the server port or leave it blank to use the default port, 20222.
- Test the connection to ensure that the ODBC service is running correctly.
With your Sage 100 sandbox account and ODBC driver configured, you're ready to start making API calls to retrieve purchase order data. In the next section, we'll explore how to perform these operations using JavaScript.
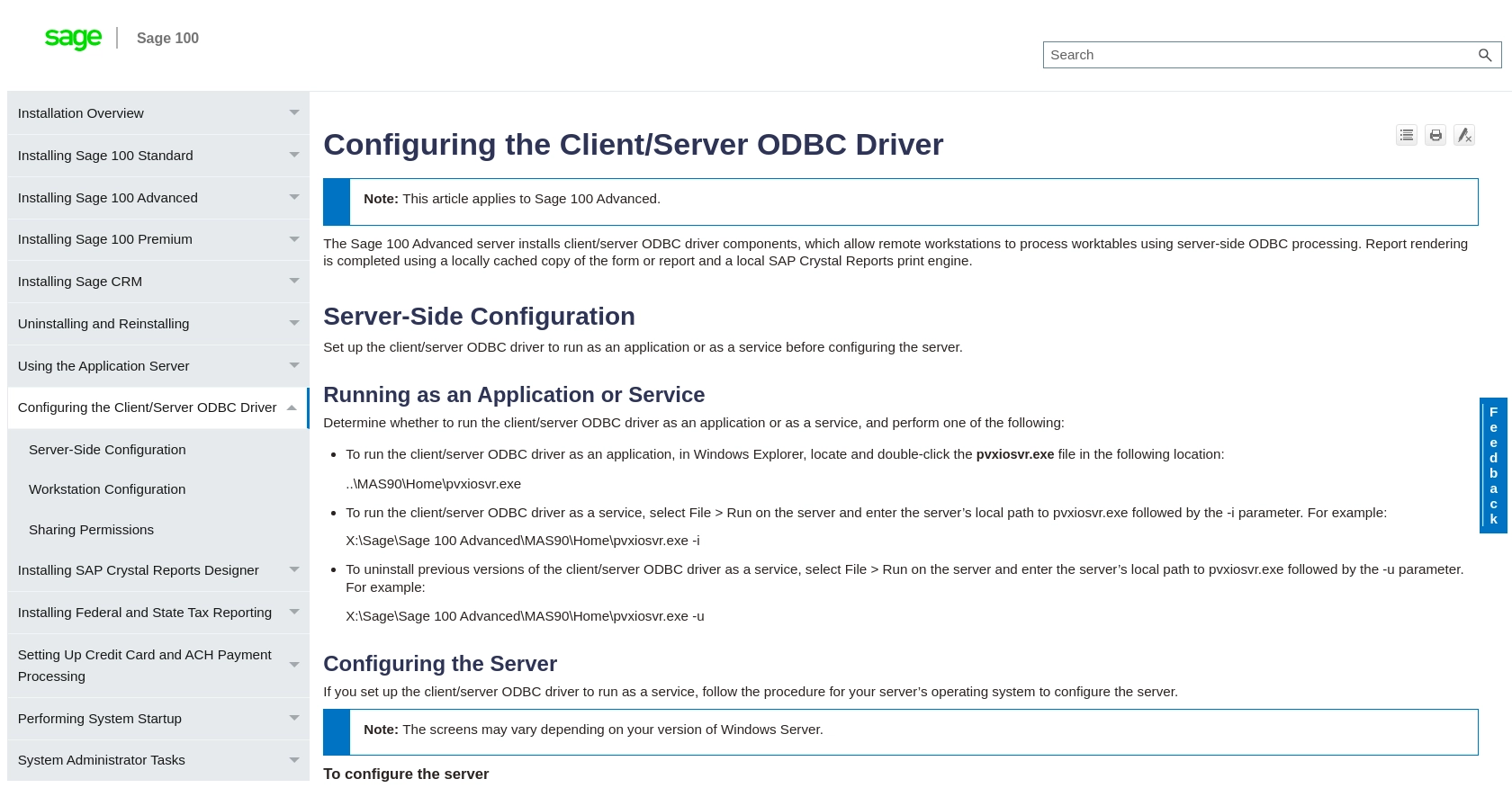
sbb-itb-96038d7
Making API Calls to Retrieve Purchase Orders from Sage 100 Using JavaScript
To interact with the Sage 100 API and retrieve purchase orders, you'll need to set up your JavaScript environment and write code to make the necessary API calls. This section will guide you through the process, including setting up your environment, writing the code, and handling potential errors.
Setting Up Your JavaScript Environment for Sage 100 API Integration
Before making API calls, ensure your JavaScript environment is ready. You'll need Node.js installed on your machine to run JavaScript outside the browser. Additionally, you'll use the odbc
package to interact with the Sage 100 ODBC driver.
- Install Node.js from the official website if it's not already installed.
- Initialize a new Node.js project by running
npm init -y
in your project directory. - Install the
odbc
package by runningnpm install odbc
.
Writing JavaScript Code to Retrieve Purchase Orders from Sage 100
With your environment set up, you can now write the JavaScript code to connect to Sage 100 and retrieve purchase orders. The following example demonstrates how to establish a connection and execute a query to fetch purchase order data.
// Import the odbc package
const odbc = require('odbc');
// Define the DSN and SQL query
const dsn = 'Your_DSN_Name';
const sqlQuery = 'SELECT * FROM PO_PurchaseOrderHeader';
// Function to retrieve purchase orders
async function getPurchaseOrders() {
try {
// Establish a connection to the Sage 100 database
const connection = await odbc.connect(`DSN=${dsn}`);
// Execute the SQL query
const result = await connection.query(sqlQuery);
// Log the purchase orders
console.log('Purchase Orders:', result);
// Close the connection
await connection.close();
} catch (error) {
console.error('Error retrieving purchase orders:', error);
}
}
// Call the function to get purchase orders
getPurchaseOrders();
Replace Your_DSN_Name
with the Data Source Name you configured for the Sage 100 ODBC driver. The SQL query SELECT * FROM PO_PurchaseOrderHeader
retrieves all purchase orders from the Sage 100 database.
Verifying API Call Success and Handling Errors
After running the code, verify the output to ensure the API call was successful. The console should display the retrieved purchase orders. If there are any issues, the error message will help diagnose the problem.
- Check your DSN configuration if you encounter connection errors.
- Ensure the SQL query is correct and matches the Sage 100 database schema.
- Handle exceptions using try-catch blocks to manage potential errors gracefully.
By following these steps, you can successfully retrieve purchase orders from Sage 100 using JavaScript. This integration allows you to automate and streamline your business processes by accessing critical data directly from your applications.
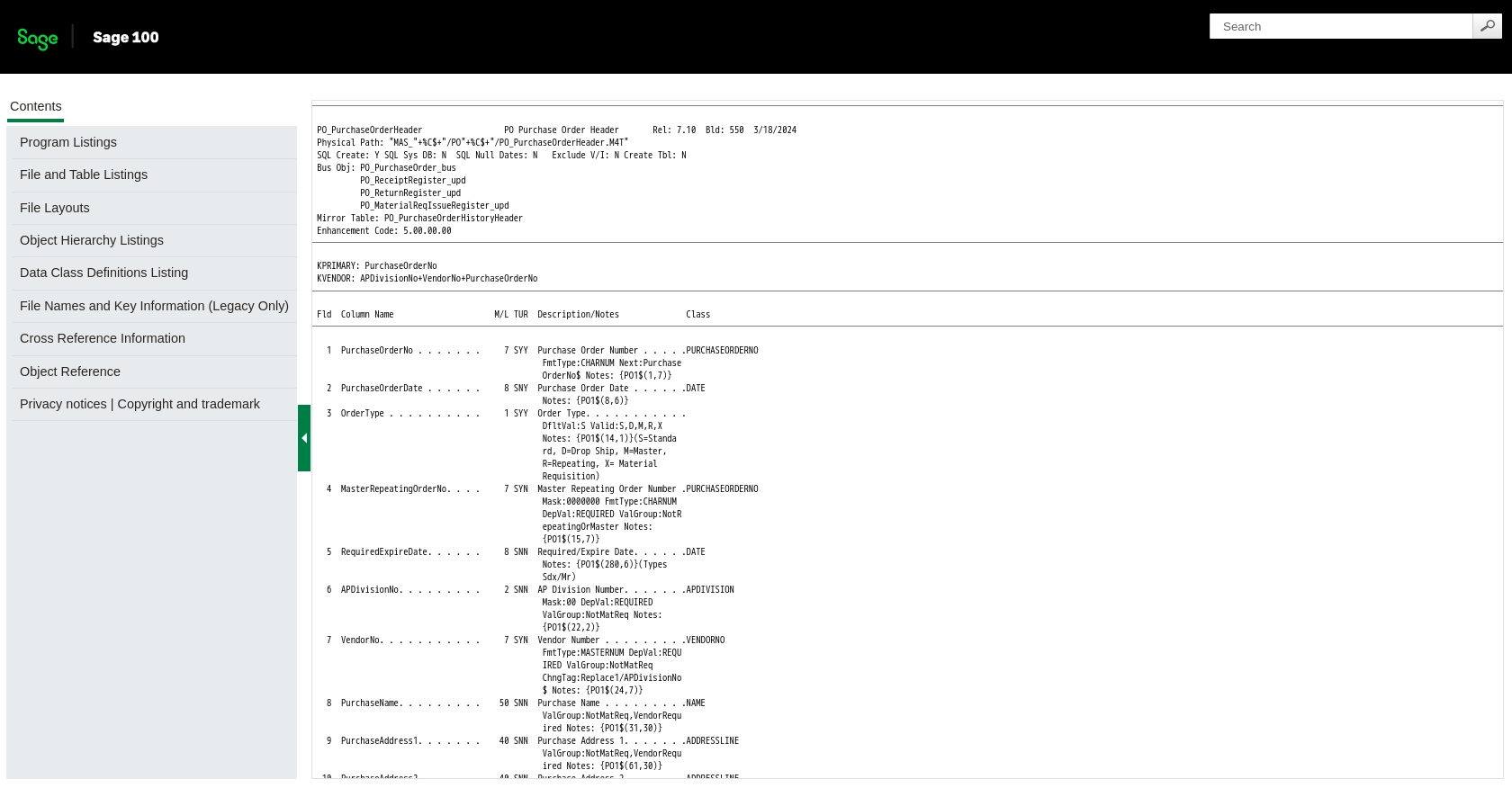
Conclusion and Best Practices for Integrating Sage 100 API with JavaScript
Integrating with the Sage 100 API using JavaScript provides a powerful way to access and manage purchase order data directly from your applications. By following the steps outlined in this guide, you can streamline your business operations and automate key processes.
Best Practices for Secure and Efficient Sage 100 API Integration
- Securely Store Credentials: Ensure that your DSN and any sensitive information are stored securely, using environment variables or secure vaults to prevent unauthorized access.
- Handle Rate Limiting: Be aware of any rate limits imposed by the Sage 100 API and implement retry logic or backoff strategies to handle potential throttling.
- Data Transformation: Consider transforming and standardizing data fields to match your application's requirements, ensuring consistency and compatibility.
- Error Handling: Implement robust error handling using try-catch blocks to manage exceptions and provide meaningful error messages for troubleshooting.
Streamlining Integrations with Endgrate
While integrating with Sage 100 API can be highly beneficial, managing multiple integrations can become complex and time-consuming. Endgrate offers a solution by providing a unified API endpoint that connects to various platforms, including Sage 100.
With Endgrate, you can save time and resources by outsourcing integrations, allowing you to focus on your core product. Build once for each use case and enjoy an intuitive integration experience for your customers. Explore how Endgrate can simplify your integration needs by visiting Endgrate.
Read More
- https://endgrate.com/provider/sage100
- https://help-sage100.na.sage.com/InstallGuide/2022/Content/InstallGuide/Config_ODBC_Driver.htm
- https://help-sage100.na.sage.com/2022/FLOR/index.htm#File_Layouts/Purchase_Order/PO_PurchaseOrderHeader.htm?Highlight=PO_PurchaseOrderHeader
- https://help-sage100.na.sage.com/2022/FLOR/index.htm#File_Layouts/Purchase_Order/PO_PurchaseOrderDetail.htm?Highlight=PO_PurchaseOrderDetail
Ready to get started?