How to Get Records with the Sugar Sell API in Python
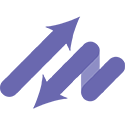
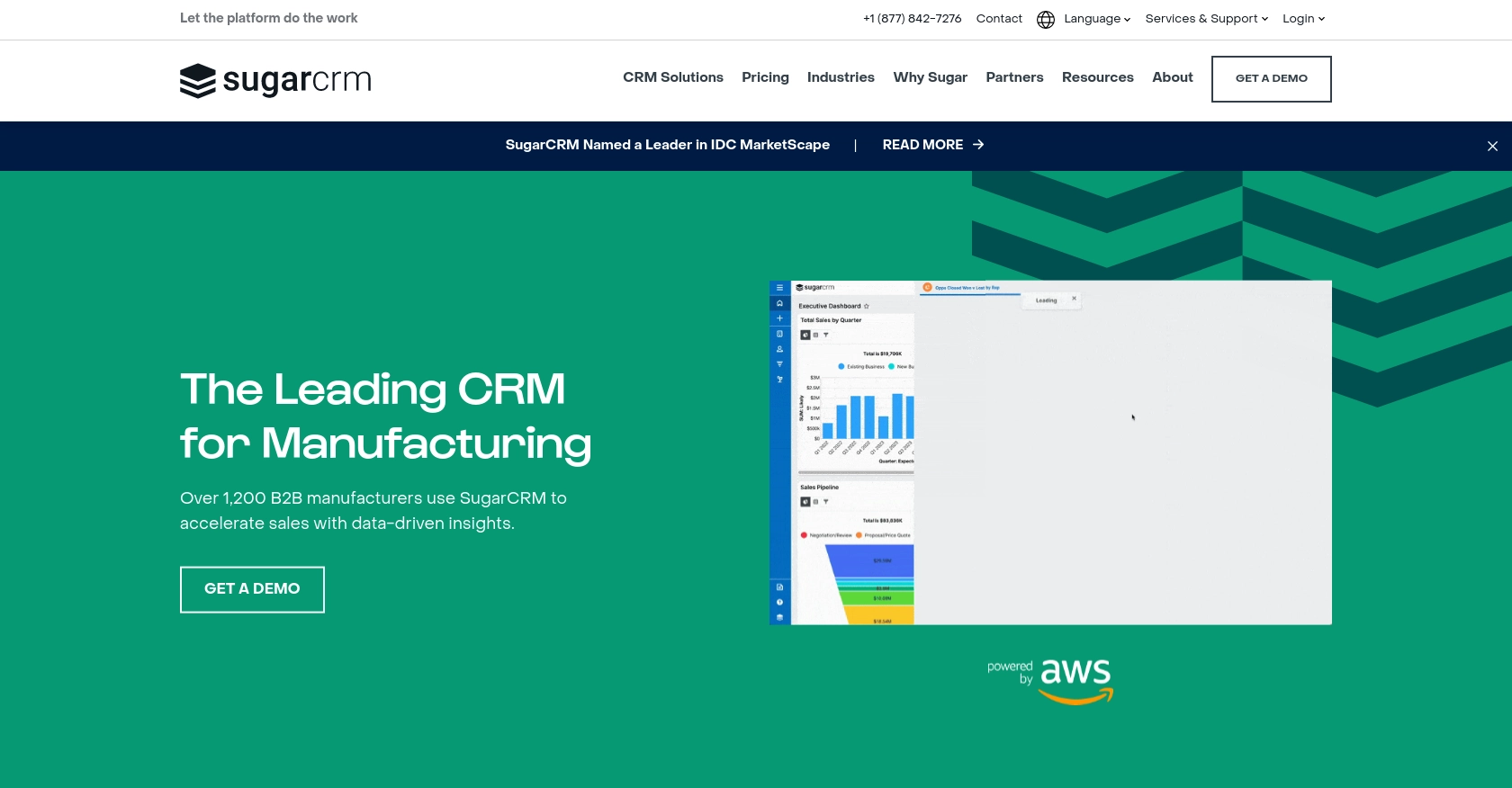
Introduction to Sugar Sell API
Sugar Sell is a robust CRM platform that empowers businesses to enhance their sales processes through comprehensive customer relationship management tools. It offers features such as lead management, sales forecasting, and customer insights, making it a popular choice for businesses aiming to improve their sales efficiency.
Integrating with the Sugar Sell API allows developers to access and manage sales data programmatically, enabling automation and customization of sales workflows. For example, a developer might use the Sugar Sell API to retrieve customer records and integrate them with other business systems, streamlining data management and enhancing customer interactions.
Setting Up Your Sugar Sell Test/Sandbox Account
Before you can start integrating with the Sugar Sell API, you need to set up a test or sandbox account. This environment allows you to safely experiment with API calls without affecting live data.
Create a Sugar Sell Sandbox Account
If you don't already have a Sugar Sell account, you can sign up for a free trial or request access to a sandbox environment through the SugarCRM website. Follow these steps to get started:
- Visit the SugarCRM website and navigate to the sign-up section.
- Choose the option for a free trial or sandbox account.
- Fill out the necessary information and submit your request.
- Once your account is created, you will receive an email with login details.
Generate API Credentials for Sugar Sell
To interact with the Sugar Sell API, you'll need to generate API credentials. Follow these steps to obtain the necessary client ID and client secret:
- Log in to your Sugar Sell account.
- Navigate to the Admin section and select OAuth Keys.
- Click on Create OAuth Key and fill in the required fields.
- Set the Client ID to "sugar" and leave the Client Secret blank for default settings.
- Save the OAuth Key and note down the Client ID and Client Secret for future use.
Authenticate Using Sugar Sell API
Sugar Sell uses two-legged OAuth2 for authentication. You will need to perform a POST request to obtain an access token. Here's how you can authenticate:
import requests
url = "https://<site_url>/rest/v13.2/oauth2/token"
payload = {
"grant_type": "password",
"client_id": "sugar",
"client_secret": "",
"username": "<your_username>",
"password": "<your_password>",
"platform": "custom"
}
headers = {
"Content-Type": "application/json"
}
response = requests.post(url, json=payload, headers=headers)
tokens = response.json()
access_token = tokens['access_token']
refresh_token = tokens['refresh_token']
Replace <site_url>
, <your_username>
, and <your_password>
with your actual Sugar Sell site URL, username, and password. Store the access_token
and refresh_token
securely for future API calls.
For more details on authentication, refer to the SugarCRM documentation.
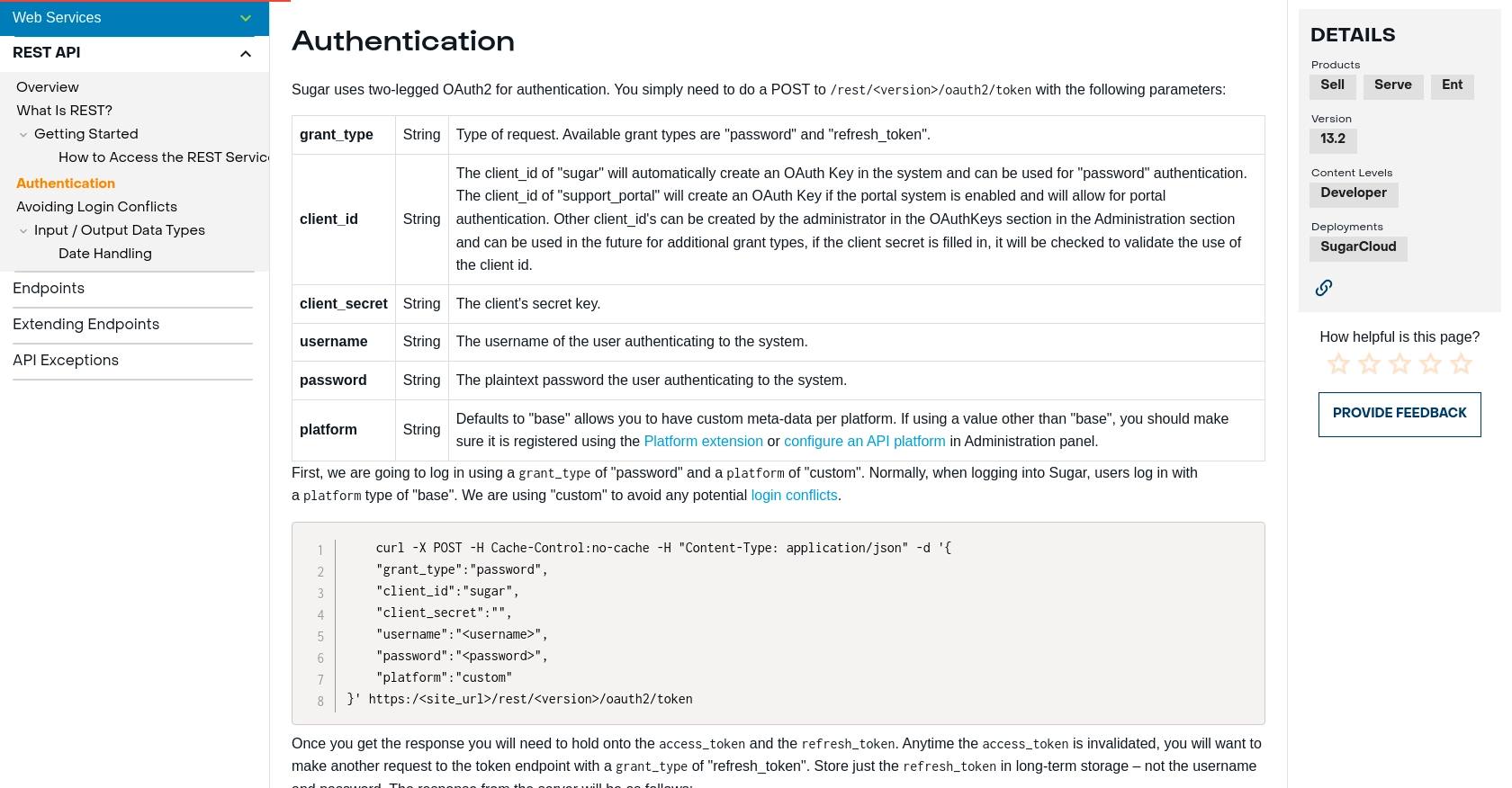
sbb-itb-96038d7
Making API Calls to Retrieve Records with Sugar Sell API in Python
To interact with the Sugar Sell API and retrieve records, you'll need to make HTTP requests using Python. This section will guide you through the process of setting up your environment, writing the necessary code, and handling the responses effectively.
Setting Up Your Python Environment for Sugar Sell API
Before making API calls, ensure you have Python installed on your machine. This tutorial uses Python 3.11.1. Additionally, you'll need the requests
library to handle HTTP requests. Install it using the following command:
pip install requests
Writing Python Code to Retrieve Records from Sugar Sell API
Once your environment is ready, you can write a Python script to retrieve records from the Sugar Sell API. Create a file named get_sugar_sell_records.py
and add the following code:
import requests
# Define the API endpoint and headers
url = "https://<site_url>/rest/v13.2/your_endpoint"
headers = {
"Authorization": "Bearer <access_token>",
"Content-Type": "application/json"
}
# Make a GET request to the API
response = requests.get(url, headers=headers)
# Check if the request was successful
if response.status_code == 200:
data = response.json()
# Process the retrieved records
for record in data['records']:
print(record)
else:
print(f"Failed to retrieve records: {response.status_code} - {response.text}")
Replace <site_url>
with your Sugar Sell site URL and <access_token>
with the access token obtained during authentication. Specify the correct endpoint for the records you wish to retrieve.
Verifying Successful API Requests in Sugar Sell
After running your script, verify that the records are retrieved successfully. You should see the records printed in your terminal. If the request fails, the error message will provide details on the issue.
Handling Errors and Common Error Codes in Sugar Sell API
When making API calls, you may encounter errors. Common HTTP status codes include:
- 400 Bad Request: The request was malformed. Check your syntax and parameters.
- 401 Unauthorized: Authentication failed. Verify your access token.
- 404 Not Found: The endpoint does not exist. Check the URL.
- 500 Internal Server Error: An error occurred on the server. Try again later.
For more details on error handling, refer to the SugarCRM documentation.
Conclusion and Best Practices for Using Sugar Sell API in Python
Integrating with the Sugar Sell API using Python can significantly enhance your ability to manage sales data efficiently. By automating data retrieval and processing, you can streamline workflows and improve customer interactions. However, to ensure a smooth integration experience, it's essential to follow best practices.
Best Practices for Securely Storing Sugar Sell API Credentials
- Secure Storage: Always store your
access_token
andrefresh_token
securely. Consider using environment variables or secure vaults to keep them safe. - Token Refresh: Regularly refresh your tokens to maintain uninterrupted access to the API. Use the
refresh_token
to obtain a newaccess_token
when needed.
Handling Sugar Sell API Rate Limiting and Error Responses
- Rate Limiting: Be mindful of the API's rate limits to avoid throttling. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Error Handling: Implement robust error handling to manage different HTTP status codes effectively. Log errors for further analysis and troubleshooting.
Data Transformation and Standardization with Sugar Sell API
- Data Consistency: Ensure that the data retrieved from the API is transformed and standardized to match your application's requirements.
- Data Validation: Validate the data before processing to prevent errors and ensure data integrity.
By following these best practices, you can create a reliable and efficient integration with the Sugar Sell API, enhancing your sales processes and customer relationship management.
Streamline Your Integrations with Endgrate
If you're looking to simplify your integration efforts, consider using Endgrate. With Endgrate, you can save time and resources by outsourcing integrations, allowing you to focus on your core product. Build once for each use case and enjoy an intuitive integration experience for your customers. Visit Endgrate to learn more about how it can support your integration needs.
Read More
- https://endgrate.com/provider/sugarsell
- https://support.sugarcrm.com/documentation/sugar_developer/sugar_developer_guide_13.2/integration/web_services/rest_api/#Authentication
- https://support.sugarcrm.com/documentation/sugar_developer/sugar_developer_guide_13.2/integration/web_services/rest_api/endpoints/
Ready to get started?