Using the Apollo API to Create Or Update Companies (with Python examples)
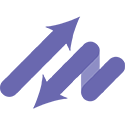
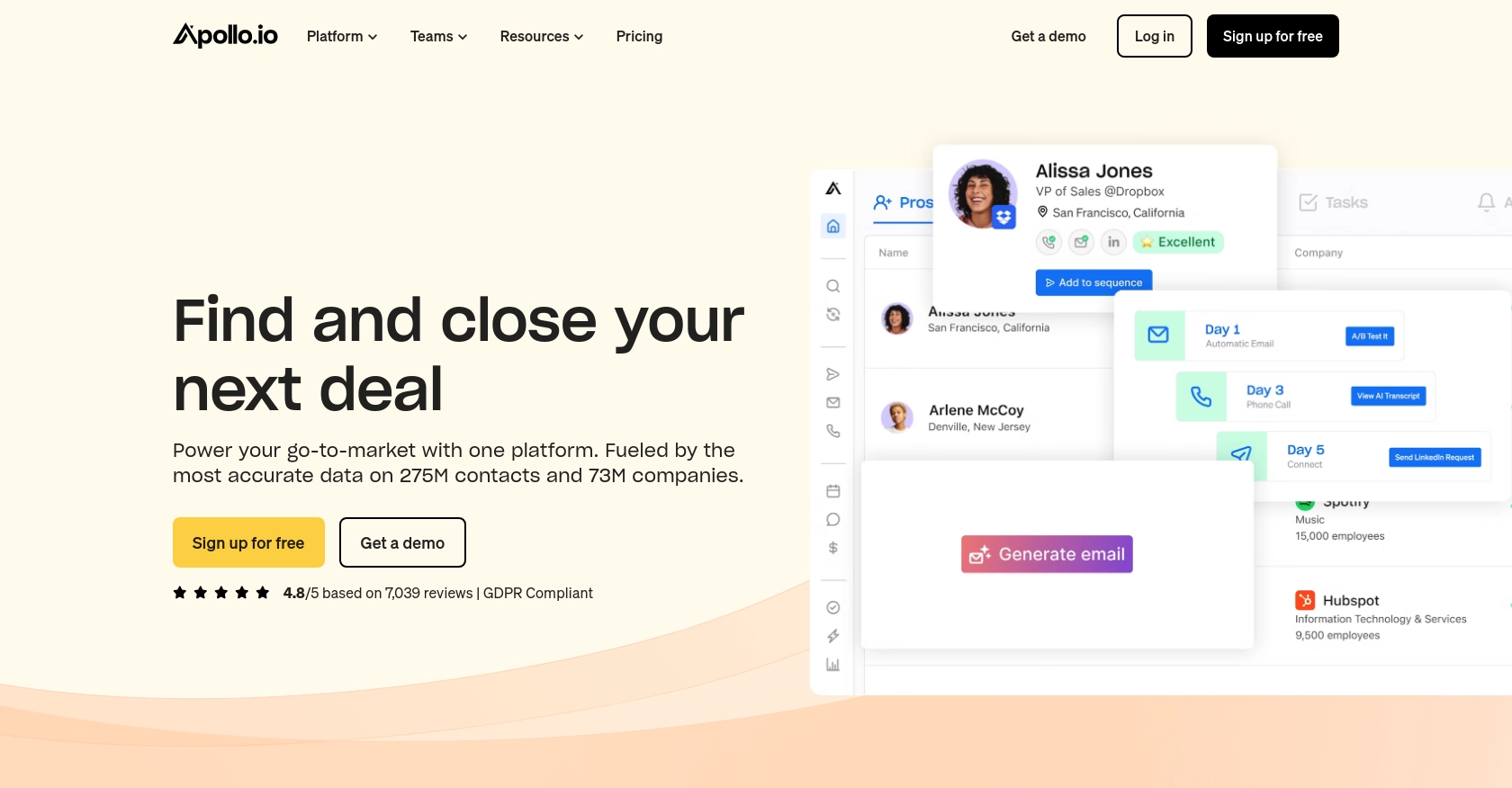
Introduction to Apollo API for Company Management
Apollo is a powerful sales intelligence and engagement platform that provides businesses with the tools they need to connect with potential customers and manage their sales processes effectively. With a rich database of contacts and companies, Apollo offers insights and automation capabilities to enhance sales strategies.
Developers may want to integrate with Apollo's API to streamline the management of company data, such as creating or updating company records. For example, a developer could use the Apollo API to automatically update company details in their CRM system, ensuring that sales teams have access to the most current information.
This article will guide you through using Python to interact with the Apollo API for creating or updating company records, providing practical examples and best practices for efficient integration.
Setting Up Your Apollo API Test or Sandbox Account
Before you can start integrating with the Apollo API, you need to set up a test or sandbox account. This will allow you to safely experiment with API calls without affecting live data. Apollo provides a straightforward process for developers to access their API using an API key.
Creating an Apollo Account
If you don't already have an Apollo account, you can sign up for a free trial or use the free version available on the Apollo website. Follow these steps to create your account:
- Visit the Apollo website and click on the "Sign Up" button.
- Fill in the required information, such as your name, email, and password.
- Verify your email address by clicking on the link sent to your inbox.
- Log in to your new Apollo account.
Generating an API Key for Apollo API Access
Once your account is set up, you need to generate an API key to authenticate your requests to the Apollo API. Follow these steps to obtain your API key:
- Log in to your Apollo account and navigate to the API settings section.
- Click on "Generate API Key" to create a new key.
- Copy the generated API key and store it securely, as you will need it for API requests.
Configuring Your Development Environment
With your API key ready, you can now configure your development environment to interact with the Apollo API using Python. Ensure you have the following prerequisites:
- Python 3.x installed on your machine.
- The
requests
library installed for making HTTP requests. You can install it using the following command:
pip install requests
With these steps completed, you are ready to start making API calls to Apollo and manage company data effectively.
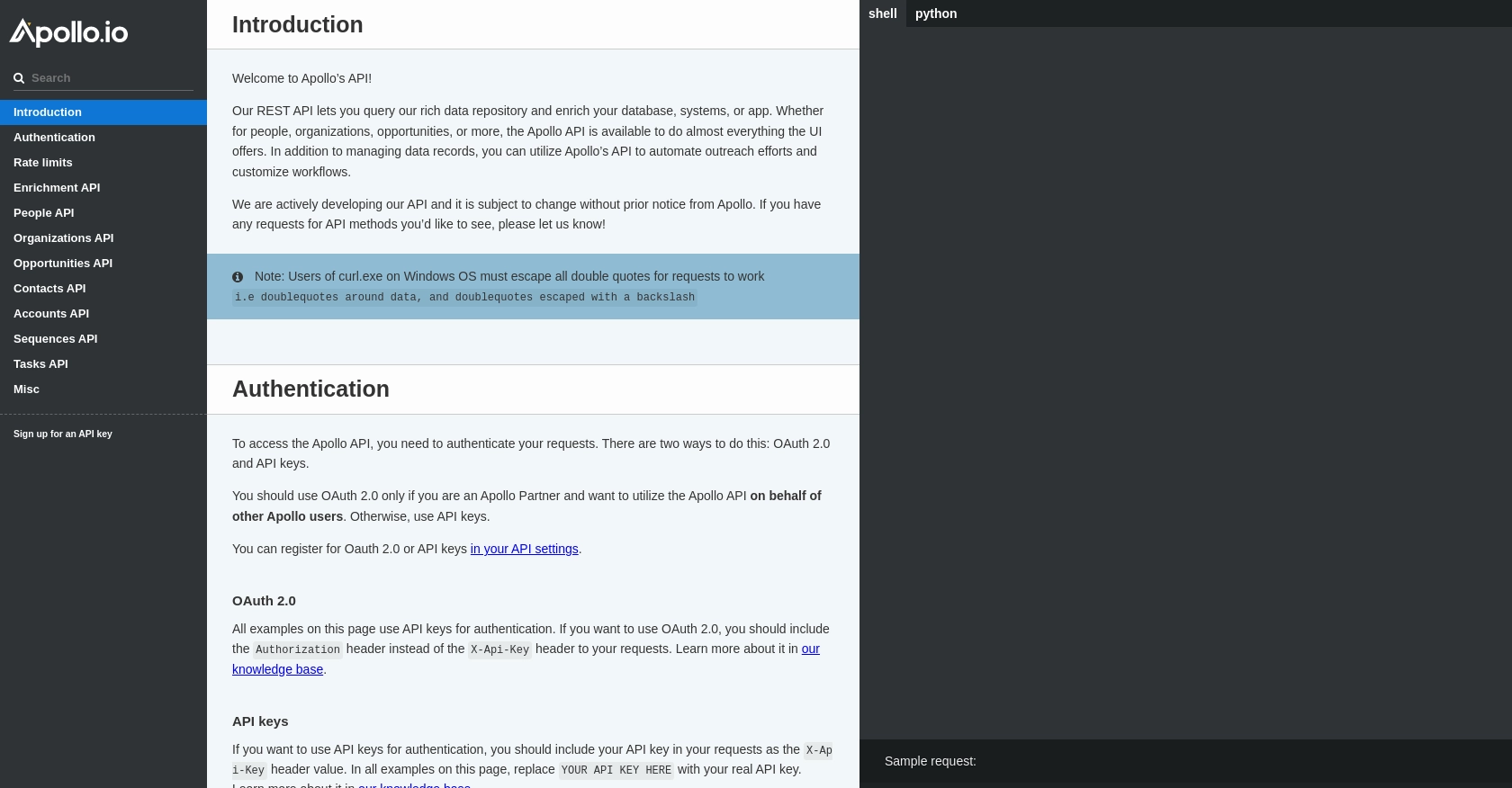
sbb-itb-96038d7
Making API Calls to Apollo for Creating or Updating Company Records Using Python
In this section, we will explore how to make API calls to the Apollo API to create or update company records using Python. This involves setting up the necessary environment, writing the code to interact with the API, and handling responses effectively.
Setting Up Your Python Environment for Apollo API Integration
Before making API calls, ensure your Python environment is correctly set up. You should have Python 3.x installed, along with the requests
library for handling HTTP requests. If you haven't installed the requests
library, do so using the following command:
pip install requests
Writing Python Code to Create or Update Companies in Apollo
With your environment ready, you can now write the Python code to interact with the Apollo API. Below is an example of how to create or update a company record:
import requests
# Define the API endpoint for creating or updating companies
url = "https://api.apollo.io/v1/accounts"
# Set the headers, including your API key
headers = {
'Content-Type': 'application/json',
'Cache-Control': 'no-cache',
'X-Api-Key': 'YOUR_API_KEY'
}
# Define the company data you want to create or update
data = {
"name": "New Company Name",
"domain": "newcompany.com",
"phone_number": "123-456-7890",
"raw_address": "123 New Street, New City, NC"
}
# Make a POST request to create or update the company
response = requests.post(url, headers=headers, json=data)
# Print the response from the server
print(response.text)
Replace YOUR_API_KEY
with the API key you generated earlier. This code snippet demonstrates how to send a POST request to the Apollo API to create or update a company record. The data
dictionary contains the company details you want to manage.
Handling API Responses and Errors from Apollo
After making the API call, it's crucial to handle the response correctly. Check the response status code to determine if the request was successful. Here’s how you can handle different scenarios:
if response.status_code == 200:
print("Company record created or updated successfully.")
else:
print(f"Failed to create or update company. Status code: {response.status_code}")
print(f"Error message: {response.json().get('error_message', 'No error message provided')}")
This code checks if the response status code is 200, indicating success. If not, it prints the status code and any error message returned by the API.
Verifying Successful API Requests in Apollo Sandbox
To ensure that your API requests are successful, verify the changes in your Apollo sandbox account. Check if the company record appears as expected. This step is crucial for confirming that your integration works correctly.
Best Practices for Error Handling and Rate Limiting with Apollo API
When working with the Apollo API, consider the following best practices:
- Store your API key securely and avoid hardcoding it in your scripts.
- Implement error handling to manage different response codes and error messages.
- Be mindful of rate limits. Apollo's API allows a certain number of requests per minute, hour, and day. Monitor your usage to avoid exceeding these limits.
By following these practices, you can ensure a robust and efficient integration with the Apollo API.
Conclusion and Best Practices for Apollo API Integration
Integrating with the Apollo API to manage company records can significantly enhance your sales and marketing strategies by ensuring your CRM is always up-to-date with the latest company information. By following the steps outlined in this guide, you can efficiently create or update company records using Python, leveraging Apollo's robust API capabilities.
Best Practices for Secure and Efficient Apollo API Usage
- Secure API Key Management: Store your API key securely, using environment variables or secure vaults, to prevent unauthorized access.
- Implement Comprehensive Error Handling: Always check response status codes and handle errors gracefully to ensure your application can recover from API failures.
- Monitor API Rate Limits: Be aware of Apollo's rate limits, which are 50 requests per minute, 100 requests per hour, and 300 requests per day. Implement logic to handle rate limit responses and avoid exceeding these limits.
- Data Validation and Transformation: Validate and transform data before sending it to the API to ensure consistency and accuracy in your records.
Enhance Your Integration Strategy with Endgrate
While integrating with the Apollo API can streamline your processes, managing multiple integrations can be complex and time-consuming. Endgrate offers a unified API solution that simplifies integration management across various platforms, including Apollo. By using Endgrate, you can focus on your core product development while ensuring seamless integration experiences for your customers.
Explore how Endgrate can help you save time and resources by visiting Endgrate today.
Read More
Ready to get started?