Using the Salesforce API to Get Records in PHP
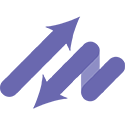
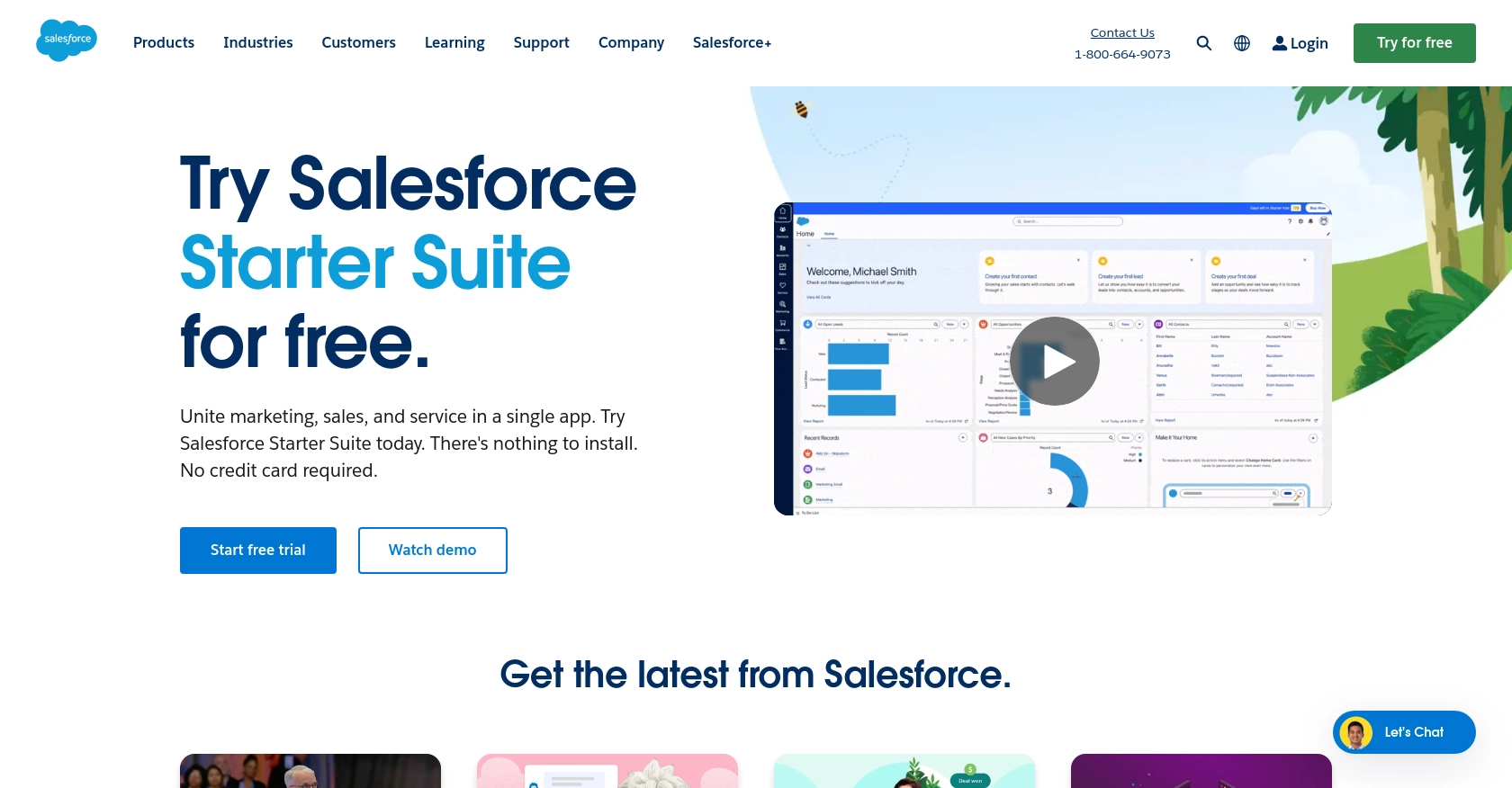
Introduction to Salesforce API Integration
Salesforce is a leading customer relationship management (CRM) platform that empowers businesses to manage their sales, marketing, and customer service operations effectively. With its robust suite of tools, Salesforce enables organizations to streamline their processes and enhance customer engagement.
For developers, integrating with Salesforce's API offers the opportunity to access and manipulate a wealth of customer data. This can be particularly useful for automating tasks such as retrieving records, updating customer information, or generating reports. For example, a developer might use the Salesforce API to fetch customer records and analyze sales trends, thereby providing valuable insights to the sales team.
Setting Up Your Salesforce Developer Account for API Integration
Before you can start interacting with the Salesforce API using PHP, you'll need to set up a Salesforce Developer account. This account will provide you with access to a sandbox environment where you can safely test your API calls without affecting live data.
Step-by-Step Guide to Creating a Salesforce Developer Account
-
Sign Up for Salesforce Developer Edition:
Visit the Salesforce Developer Signup Page and complete the registration form. Once registered, you'll receive an email to verify your account. Follow the instructions in the email to activate your account.
-
Log In to Salesforce:
After activating your account, log in to Salesforce using your credentials. This will take you to the Salesforce Developer Console, where you can manage your applications and data.
Creating a Connected App for OAuth Authentication
To interact with the Salesforce API, you'll need to create a Connected App. This app will enable OAuth authentication, allowing you to securely access Salesforce data.
-
Navigate to Setup:
In the Salesforce Developer Console, click on the gear icon in the top right corner and select "Setup."
-
Create a New Connected App:
In the Quick Find box, type "App Manager" and click on it. Then, click "New Connected App" in the top right corner.
-
Configure the Connected App:
Fill in the required fields such as "Connected App Name," "API Name," and "Contact Email." Under "API (Enable OAuth Settings)," check the box for "Enable OAuth Settings."
-
Set OAuth Scopes:
In the "Selected OAuth Scopes" section, add the necessary scopes such as "Full access (full)" and "Access and manage your data (api)."
-
Save and Retrieve Credentials:
Click "Save" to create the app. After saving, you'll be provided with a "Consumer Key" and "Consumer Secret." Make sure to copy these credentials as you'll need them for authentication.
With your Salesforce Developer account and Connected App set up, you're now ready to start making API calls using PHP. This setup ensures that you have the necessary environment and credentials to authenticate and interact with Salesforce data securely.
For more detailed information on setting up a Salesforce Developer account and creating a Connected App, refer to the official Salesforce documentation: Authorization Through Connected Apps and OAuth 2.0.
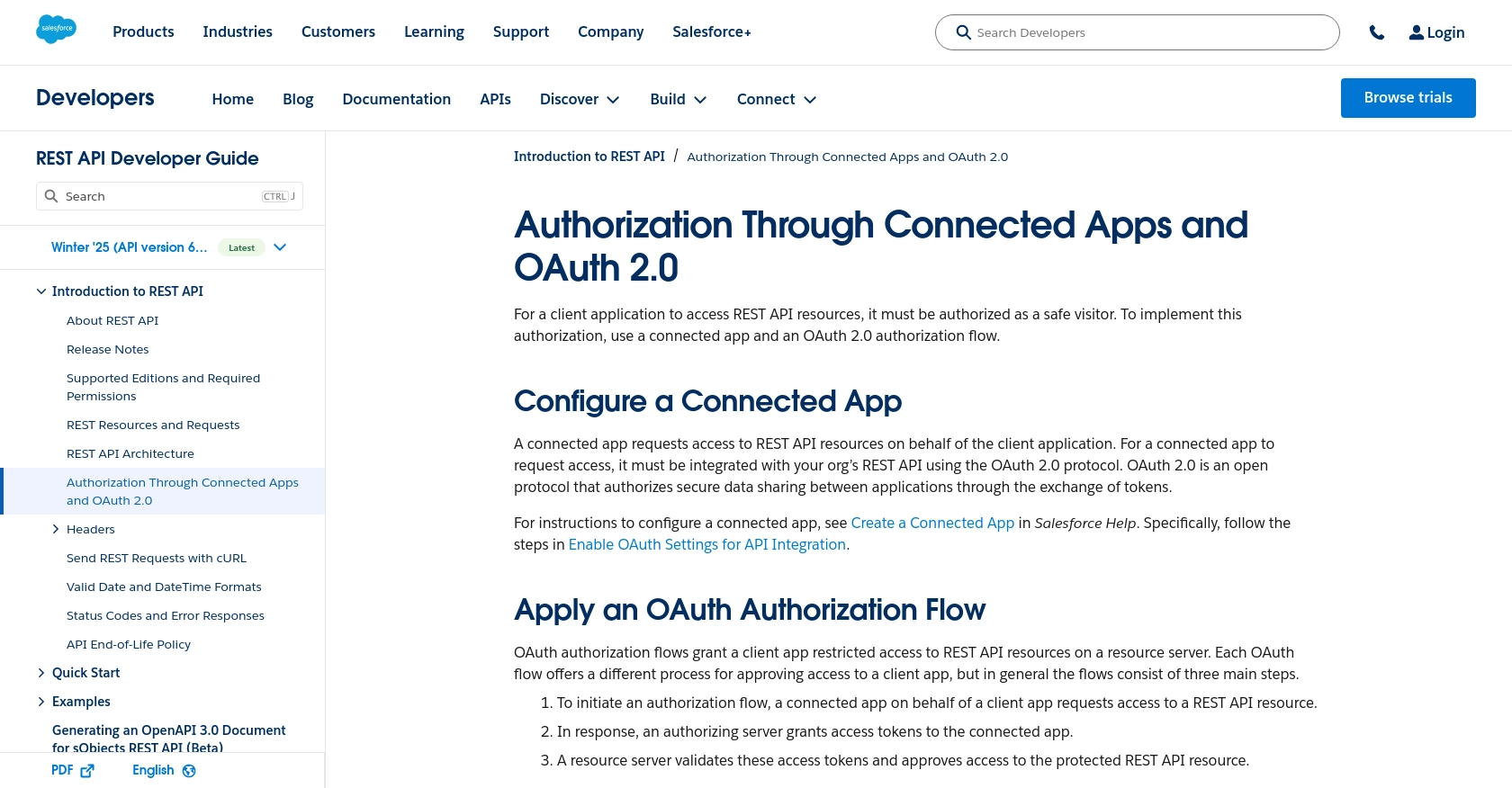
sbb-itb-96038d7
Making API Calls to Salesforce Using PHP
With your Salesforce Developer account and Connected App ready, you can now proceed to make API calls using PHP. This section will guide you through the process of setting up your PHP environment, writing the code to authenticate with Salesforce, and retrieving records from the Salesforce API.
Setting Up Your PHP Environment for Salesforce API Integration
Before making API calls, ensure that your PHP environment is properly configured. You'll need PHP 7.4 or later and the cURL
extension enabled. You can verify your PHP version and extensions by running the following command:
php -v
php -m | grep curl
Installing Required PHP Dependencies
To interact with the Salesforce API, you'll need to install the guzzlehttp/guzzle
library, which simplifies HTTP requests in PHP. Use Composer to install it:
composer require guzzlehttp/guzzle
Authenticating with Salesforce Using OAuth 2.0
To authenticate with Salesforce, you'll need to obtain an access token using the OAuth 2.0 protocol. Here's how you can do it in PHP:
use GuzzleHttp\Client;
$client = new Client();
$response = $client->post('https://login.salesforce.com/services/oauth2/token', [
'form_params' => [
'grant_type' => 'password',
'client_id' => 'Your_Consumer_Key',
'client_secret' => 'Your_Consumer_Secret',
'username' => 'Your_Salesforce_Username',
'password' => 'Your_Salesforce_Password' . 'Your_Security_Token'
]
]);
$accessToken = json_decode($response->getBody(), true)['access_token'];
Replace Your_Consumer_Key
, Your_Consumer_Secret
, Your_Salesforce_Username
, Your_Salesforce_Password
, and Your_Security_Token
with your actual Salesforce credentials and security token.
Retrieving Records from Salesforce API
Once authenticated, you can use the access token to make API calls to Salesforce. Here's an example of how to retrieve records:
$response = $client->get('https://your_instance.salesforce.com/services/data/v52.0/query', [
'headers' => [
'Authorization' => 'Bearer ' . $accessToken
],
'query' => [
'q' => 'SELECT Id, Name FROM Account LIMIT 10'
]
]);
$records = json_decode($response->getBody(), true);
foreach ($records['records'] as $record) {
echo 'ID: ' . $record['Id'] . ' Name: ' . $record['Name'] . "\n";
}
Replace your_instance
with your Salesforce instance URL. This code retrieves the first 10 account records and prints their IDs and names.
Handling Errors and Verifying API Call Success
It's important to handle potential errors when making API calls. You can check the response status code to ensure the request was successful:
if ($response->getStatusCode() === 200) {
echo "API call successful.";
} else {
echo "Error: " . $response->getStatusCode();
}
Verify the retrieved data by checking your Salesforce sandbox environment to ensure the records match the expected output.
For more detailed information on Salesforce API calls, refer to the official Salesforce documentation.
Conclusion and Best Practices for Salesforce API Integration Using PHP
Integrating with the Salesforce API using PHP offers developers a powerful way to access and manage customer data, automate processes, and generate valuable insights. By following the steps outlined in this guide, you can efficiently set up your Salesforce Developer account, authenticate using OAuth 2.0, and retrieve records seamlessly.
Best Practices for Secure and Efficient Salesforce API Integration
- Securely Store Credentials: Always store your Salesforce credentials, including the Consumer Key and Secret, securely. Consider using environment variables or secure vaults to protect sensitive information.
- Handle Rate Limiting: Salesforce imposes API rate limits to ensure fair usage. Be mindful of these limits and implement retry logic or backoff strategies to handle rate limit errors gracefully.
- Standardize Data Fields: When retrieving or updating records, ensure that data fields are standardized to maintain consistency across your application and Salesforce.
- Monitor API Usage: Regularly monitor your API usage and performance to identify any bottlenecks or issues. This will help you optimize your integration and ensure smooth operation.
Streamline Your Integration Process with Endgrate
While integrating with Salesforce is a powerful way to enhance your business operations, managing multiple integrations can be complex and time-consuming. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms and services, including Salesforce.
With Endgrate, you can save time and resources by building once for each use case instead of multiple times for different integrations. Focus on your core product while Endgrate handles the complexities of integration, offering an easy and intuitive experience for your customers.
Explore how Endgrate can transform your integration strategy by visiting Endgrate today.
Read More
- https://endgrate.com/provider/salesforce
- https://developer.salesforce.com/docs/atlas.en-us.api_rest.meta/api_rest/intro_oauth_and_connected_apps.htm
- https://developer.salesforce.com/docs/atlas.en-us.api_rest.meta/api_rest/quickstart_dev_org.htm
- https://developer.salesforce.com/docs/atlas.en-us.api_rest.meta/api_rest/resources_list.htm
Ready to get started?