How to Get Users with the Brevo API in PHP
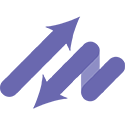
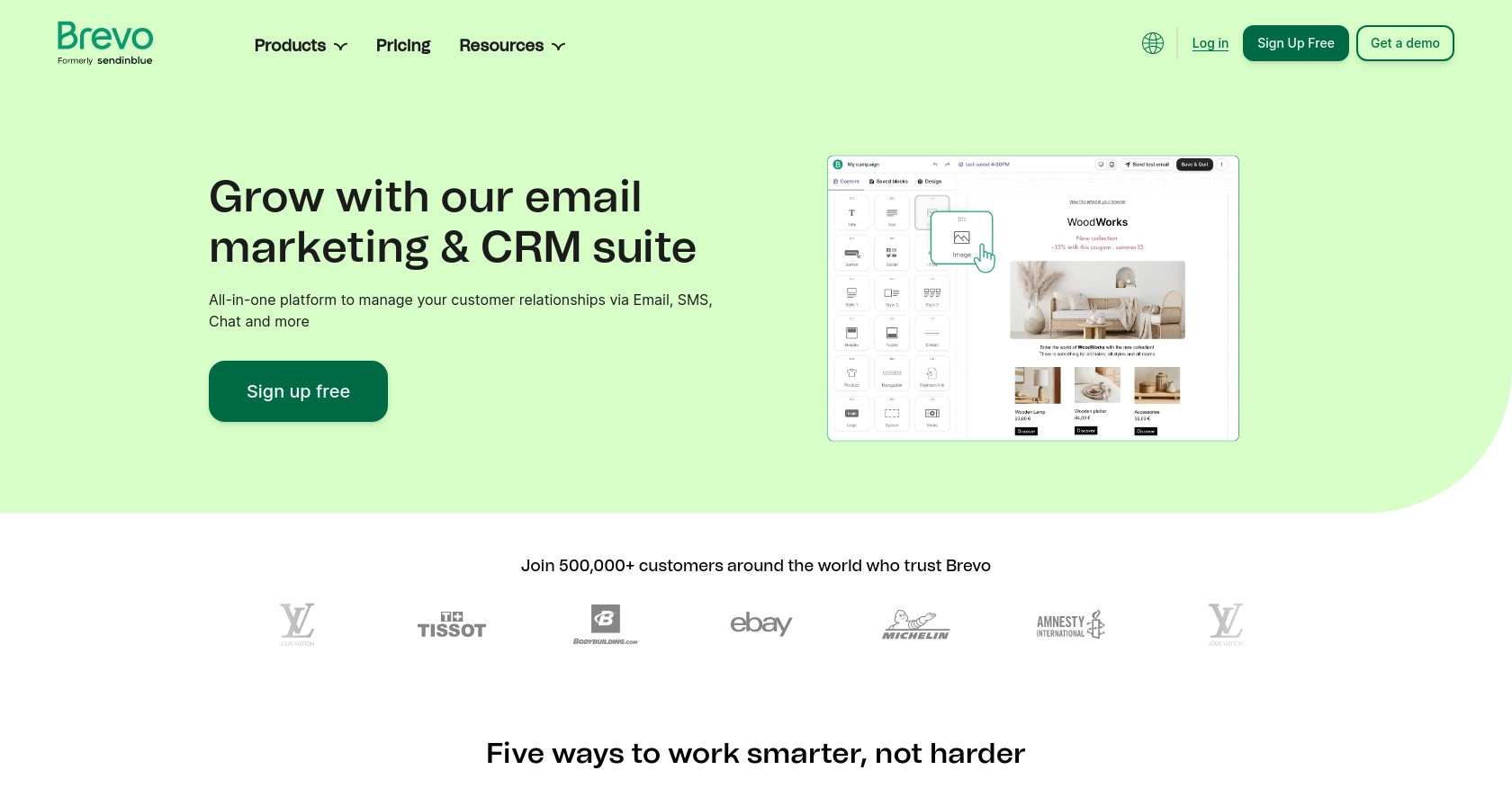
Introduction to Brevo API
Brevo is a versatile platform offering a suite of tools for managing communications and marketing efforts. It provides robust APIs that allow developers to integrate and automate various functionalities, such as email campaigns, SMS marketing, and contact management.
Connecting with Brevo's API can significantly enhance a developer's ability to manage user data and communications efficiently. For example, using the Brevo API, developers can retrieve a list of users to personalize marketing campaigns or manage user permissions within their application.
This article will guide you through the process of using PHP to interact with the Brevo API, specifically focusing on retrieving user information. By following this tutorial, you'll learn how to set up your environment, authenticate requests, and handle the data returned by the API.
Setting Up Your Brevo Account for API Access
Before you can start interacting with the Brevo API using PHP, you need to set up your Brevo account and obtain the necessary API key. This key will allow you to authenticate your requests and access the API's functionalities.
Creating a Brevo Account
If you don't already have a Brevo account, you can sign up for free on the Brevo website. Follow these steps to create your account:
- Visit the Brevo signup page.
- Fill in the required information, such as your email address and password.
- Confirm your email address by clicking the verification link sent to your inbox.
Generating an API Key in Brevo
Once your account is set up, you'll need to generate an API key to authenticate your requests. Follow these steps to obtain your API key:
- Log in to your Brevo account.
- Click on your profile name in the top-right corner and select SMTP & API from the dropdown menu.
- Navigate to the API keys tab.
- Click on Generate a new API key.
- Provide a name for your API key to help you identify it later.
- Click Generate and copy the API key provided. Store it securely, as you'll need it for making API requests.
For more detailed instructions, you can refer to the Brevo API documentation.
Understanding API Key Authentication
Brevo uses API key authentication to verify the identity of the user making the request. This means that every request you send to the Brevo API must include your API key in the headers. This key acts as a unique identifier for your account, ensuring that only authorized users can access your data.
With your API key ready, you can now proceed to make API calls to Brevo and start retrieving user information using PHP.
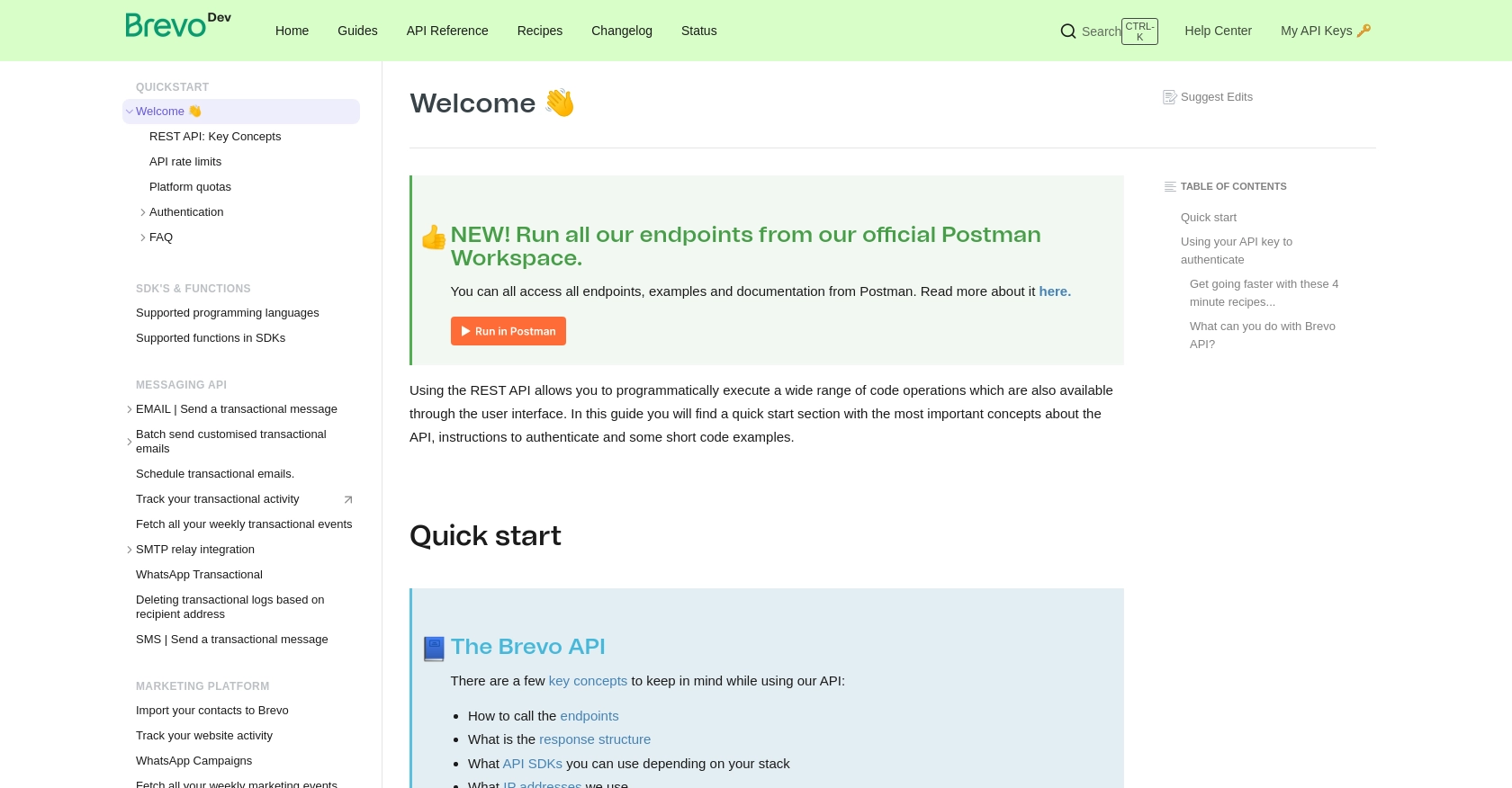
sbb-itb-96038d7
Making API Calls to Retrieve Users with Brevo API in PHP
To interact with the Brevo API using PHP, you'll need to set up your environment and write code to make API requests. This section will guide you through the necessary steps, including setting up PHP, installing dependencies, and writing the code to retrieve user information from Brevo.
Setting Up Your PHP Environment for Brevo API Integration
Before making API calls, ensure you have the following prerequisites installed on your machine:
- PHP 7.4 or higher
- Composer, the PHP package manager
Once you have these installed, open your terminal and navigate to your project directory. Use Composer to install the Guzzle HTTP client, which will help you make HTTP requests:
composer require guzzlehttp/guzzle
Writing PHP Code to Retrieve Users from Brevo API
With your environment set up, you can now write the PHP code to interact with the Brevo API. Create a new PHP file named get_brevo_users.php
and add the following code:
<?php
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$apiKey = 'Your_API_Key'; // Replace with your actual API key
$client = new Client();
try {
$response = $client->request('GET', 'https://api.brevo.com/v3/organization/invited/users', [
'headers' => [
'accept' => 'application/json',
'api-key' => $apiKey
]
]);
$data = json_decode($response->getBody(), true);
foreach ($data['users'] as $user) {
echo 'Email: ' . $user['email'] . '<br>';
echo 'Is Owner: ' . $user['is_owner'] . '<br>';
echo 'Status: ' . $user['status'] . '<br><br>';
}
} catch (Exception $e) {
echo 'Error: ' . $e->getMessage();
}
Replace Your_API_Key
with the API key you generated earlier. This code initializes a Guzzle client, makes a GET request to the Brevo API to retrieve the list of users, and then iterates through the response to display user details.
Verifying API Call Success and Handling Errors
After running the script, you should see the list of users printed on your screen. If the request is successful, the user data will match the information in your Brevo account. If there's an error, the script will catch it and display an error message.
Common error codes include:
- 400 Bad Request: Invalid parameters or missing required fields.
- 401 Unauthorized: Incorrect or missing API key.
- 403 Forbidden: Insufficient permissions to access the resource.
For more details on error codes, refer to the Brevo API documentation.
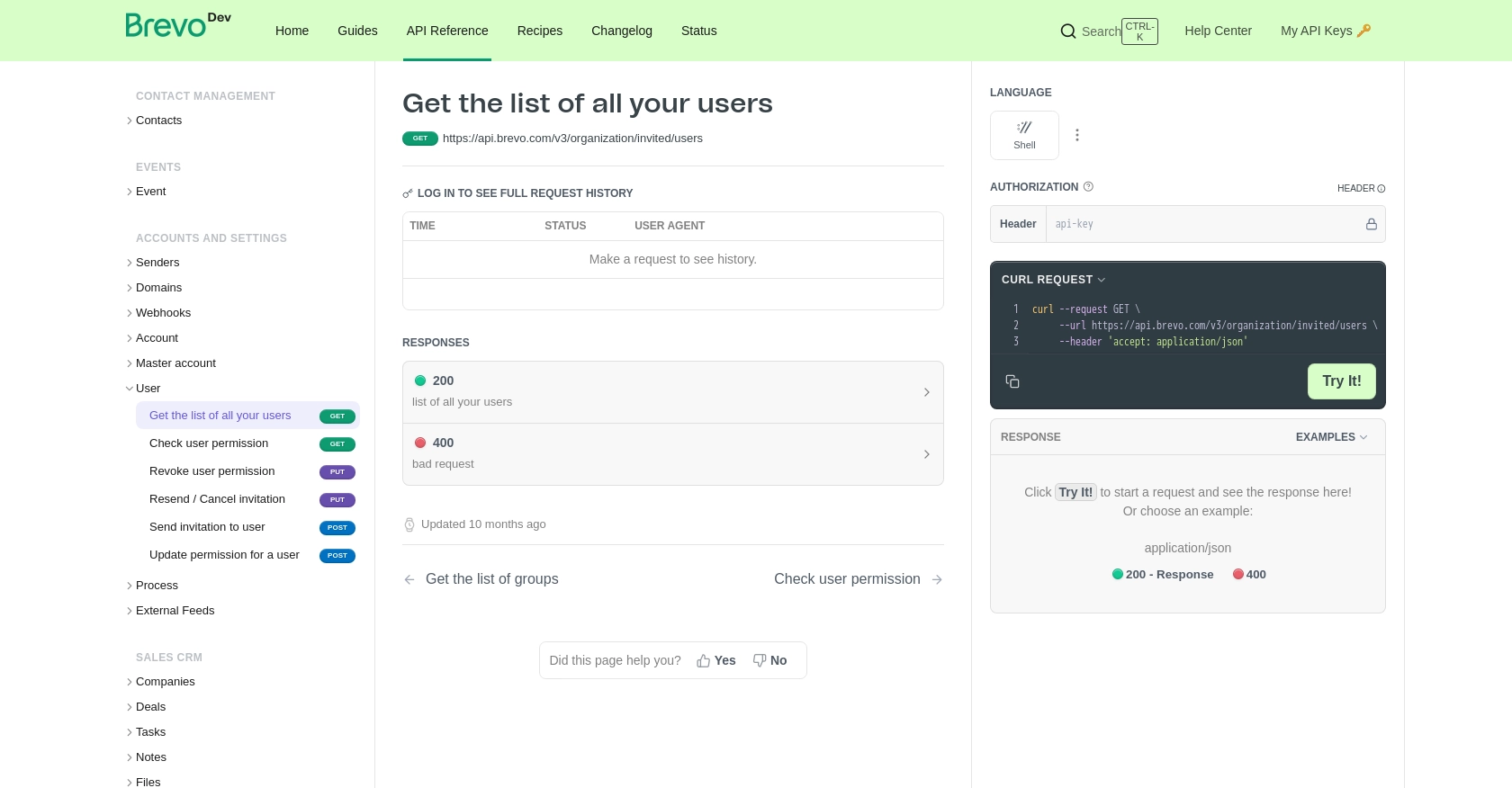
Conclusion and Best Practices for Using Brevo API with PHP
Integrating with the Brevo API using PHP allows developers to efficiently manage user data and enhance communication strategies. By following the steps outlined in this guide, you can set up your environment, authenticate requests, and retrieve user information seamlessly.
Best Practices for Secure and Efficient Brevo API Integration
- Secure API Key Storage: Always store your API keys securely, using environment variables or secure vaults, to prevent unauthorized access.
- Handle API Rate Limits: Be mindful of Brevo's API rate limits to avoid throttling. Implement retry logic with exponential backoff to manage rate limit errors gracefully.
- Data Standardization: Standardize and transform data fields as needed to ensure consistency across your application and Brevo's platform.
- Error Handling: Implement robust error handling to manage different HTTP status codes and provide meaningful feedback to users.
Enhance Your Integration Strategy with Endgrate
While integrating with Brevo is a powerful way to manage communications, handling multiple integrations can be complex and time-consuming. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Brevo.
With Endgrate, you can streamline your integration efforts, allowing you to focus on your core product while offering a seamless experience to your customers. Explore how Endgrate can help you save time and resources by visiting Endgrate's website.
Read More
Ready to get started?