Using the Zoho CRM API to Create or Update Contacts (with Javascript examples)
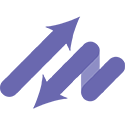
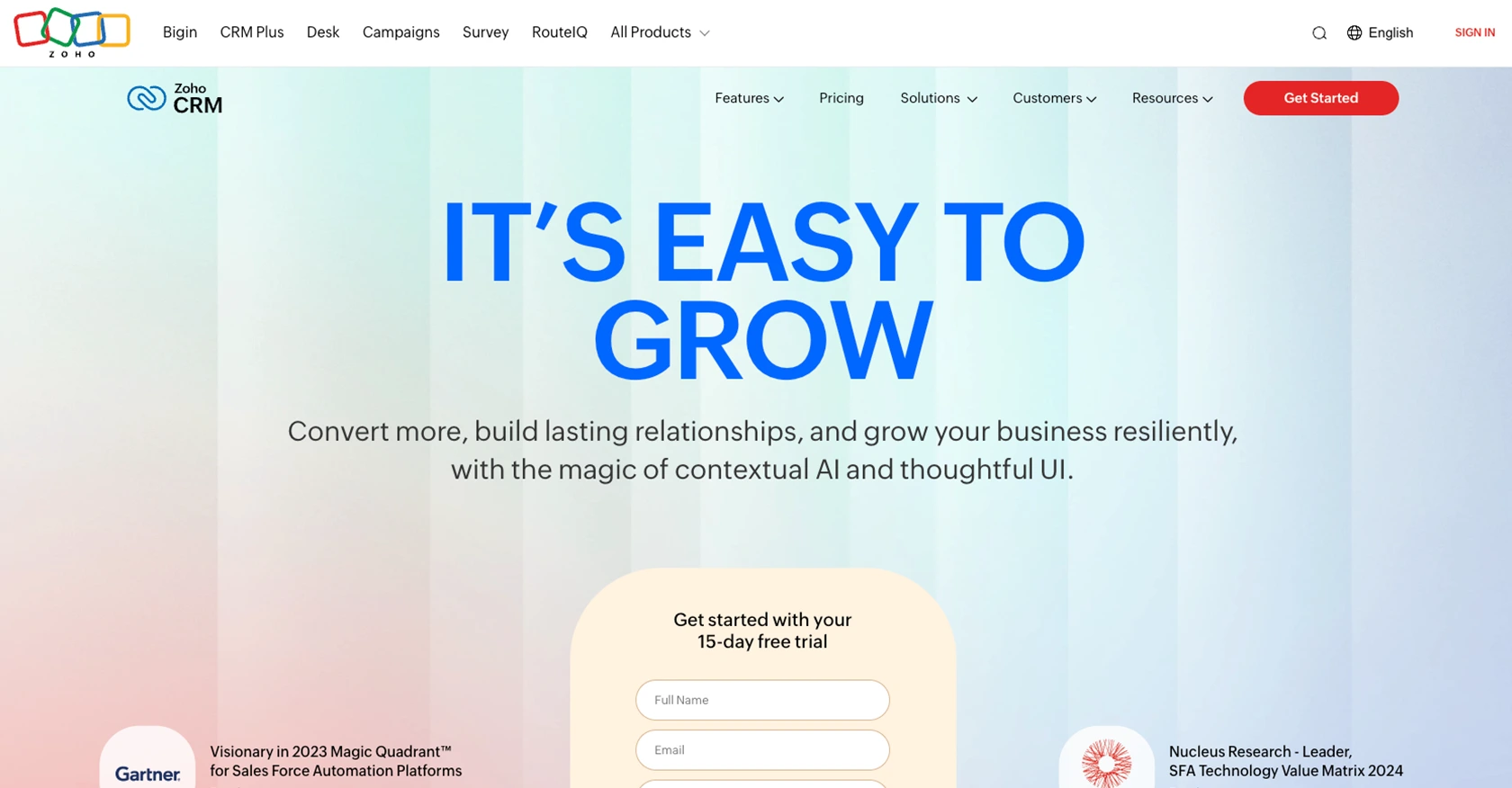
Introduction to Zoho CRM API Integration
Zoho CRM is a comprehensive customer relationship management platform that empowers businesses to manage their sales, marketing, and support in one unified system. Known for its flexibility and scalability, Zoho CRM is a popular choice for organizations looking to enhance their customer engagement and streamline operations.
Integrating with the Zoho CRM API allows developers to automate and optimize various CRM functions, such as managing contacts. By leveraging the API, developers can create or update contact records programmatically, ensuring data consistency and reducing manual effort.
For example, a developer might use the Zoho CRM API to automatically update contact information from an external database, ensuring that the CRM always reflects the most current data. This integration can significantly enhance productivity and data accuracy within the CRM system.
Setting Up Your Zoho CRM Test/Sandbox Account
Before you can start integrating with the Zoho CRM API, you'll need to set up a test or sandbox account. This will allow you to safely experiment with the API without affecting your live data.
Step 1: Sign Up for a Zoho CRM Developer Account
If you don't already have a Zoho CRM account, you can sign up for a free developer account. This account will give you access to the necessary tools and resources to start building your integration.
- Visit the Zoho CRM signup page.
- Fill out the registration form with your details.
- Verify your email address to activate your account.
Step 2: Create a Zoho CRM Sandbox Environment
The sandbox environment allows you to test your API integrations in a controlled setting. Follow these steps to set up your sandbox:
- Log in to your Zoho CRM account.
- Navigate to Setup > Developer Space > Sandbox.
- Click on Create Sandbox and follow the prompts to configure your sandbox environment.
Step 3: Register Your Application for OAuth Authentication
Zoho CRM uses OAuth 2.0 for authentication. You'll need to register your application to obtain the necessary credentials:
- Go to the Zoho Developer Console.
- Select Register Client and choose the client type as Web Based or JavaScript.
- Enter the required details such as Client Name, Homepage URL, and Authorized Redirect URIs.
- Click Create to generate your Client ID and Client Secret.
Step 4: Configure OAuth Scopes
Scopes define the level of access your application will have. Configure the necessary scopes for your integration:
- In the Developer Console, navigate to the Scopes section.
- Select the appropriate scopes for accessing and managing contacts, such as
ZohoCRM.modules.contacts.ALL
.
Step 5: Generate Access and Refresh Tokens
With your client credentials, you can now generate access and refresh tokens:
// Example code to generate tokens
const axios = require('axios');
async function getTokens() {
const response = await axios.post('https://accounts.zoho.com/oauth/v2/token', {
grant_type: 'authorization_code',
client_id: 'Your_Client_ID',
client_secret: 'Your_Client_Secret',
redirect_uri: 'Your_Redirect_URI',
code: 'Authorization_Code'
});
console.log(response.data);
}
getTokens();
Replace Your_Client_ID
, Your_Client_Secret
, Your_Redirect_URI
, and Authorization_Code
with your actual values.
By following these steps, you'll have a fully configured Zoho CRM sandbox environment ready for API integration testing.
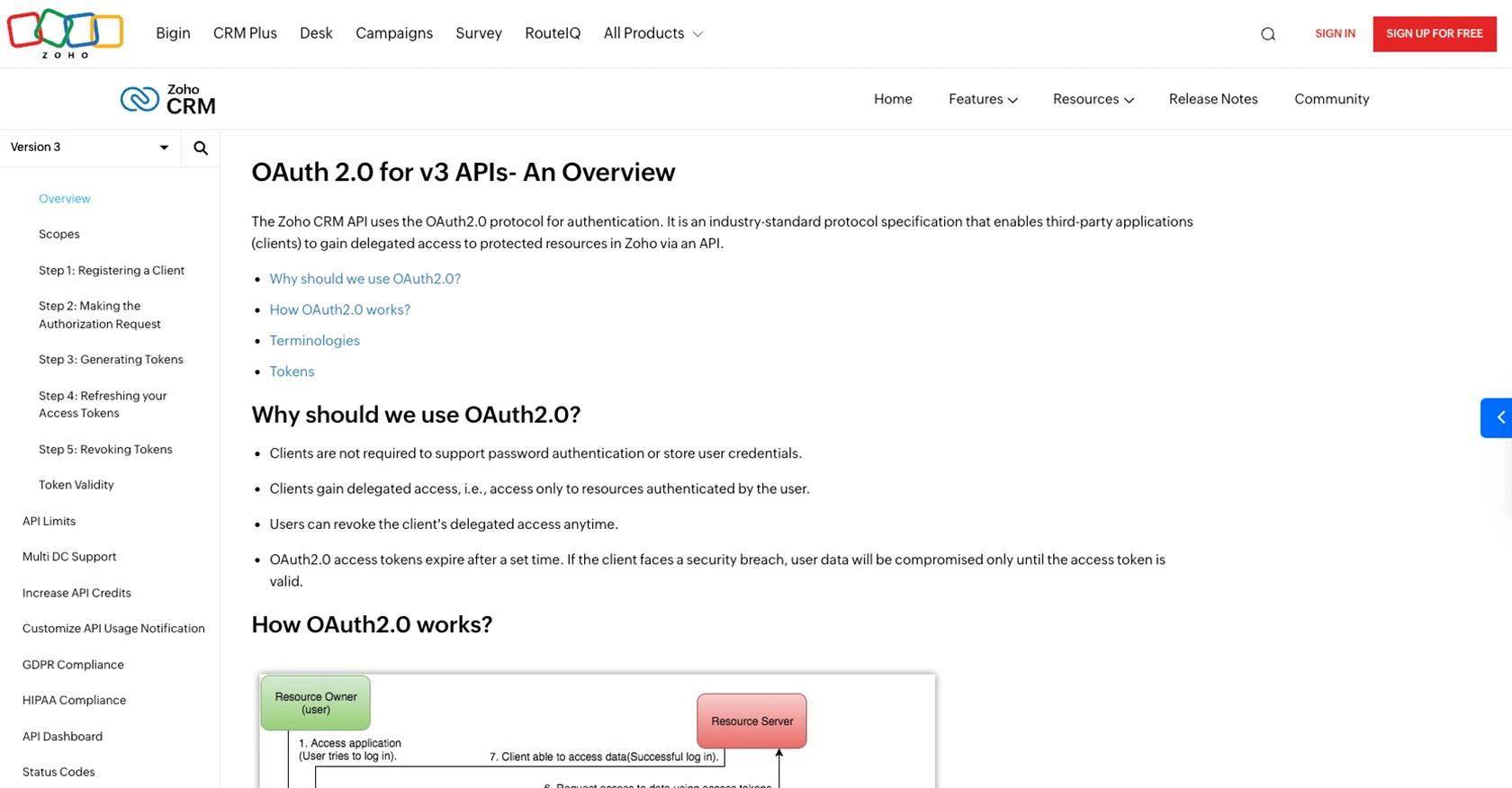
sbb-itb-96038d7
How to Make an API Call to Create or Update Contacts in Zoho CRM Using JavaScript
To interact with the Zoho CRM API for creating or updating contacts, you'll need to use JavaScript to make HTTP requests. This section will guide you through the process of setting up your environment and executing API calls effectively.
Prerequisites for Zoho CRM API Integration with JavaScript
Before you begin, ensure you have the following installed on your machine:
- Node.js and npm (Node Package Manager)
- Axios library for making HTTP requests
Install Axios by running the following command in your terminal:
npm install axios
Creating a New Contact in Zoho CRM Using JavaScript
To create a new contact in Zoho CRM, you'll need to send a POST request to the Zoho CRM API endpoint. Here's a sample code snippet to help you get started:
const axios = require('axios');
async function createContact() {
const accessToken = 'Your_Access_Token';
const contactData = {
data: [
{
Last_Name: 'Doe',
First_Name: 'John',
Email: 'john.doe@example.com'
}
]
};
try {
const response = await axios.post('https://www.zohoapis.com/crm/v3/Contacts', contactData, {
headers: {
Authorization: `Zoho-oauthtoken ${accessToken}`,
'Content-Type': 'application/json'
}
});
console.log('Contact Created:', response.data);
} catch (error) {
console.error('Error Creating Contact:', error.response.data);
}
}
createContact();
Replace Your_Access_Token
with the actual access token you obtained during the OAuth setup.
Updating an Existing Contact in Zoho CRM Using JavaScript
To update an existing contact, you'll need to know the contact's unique ID. Use the following code to update a contact:
async function updateContact(contactId) {
const accessToken = 'Your_Access_Token';
const updatedData = {
data: [
{
id: contactId,
Last_Name: 'Smith',
Email: 'john.smith@example.com'
}
]
};
try {
const response = await axios.put(`https://www.zohoapis.com/crm/v3/Contacts/${contactId}`, updatedData, {
headers: {
Authorization: `Zoho-oauthtoken ${accessToken}`,
'Content-Type': 'application/json'
}
});
console.log('Contact Updated:', response.data);
} catch (error) {
console.error('Error Updating Contact:', error.response.data);
}
}
updateContact('Contact_ID');
Replace Contact_ID
with the actual ID of the contact you wish to update.
Handling API Responses and Errors
After making an API call, it's crucial to handle the responses and any potential errors. Successful responses will contain the details of the created or updated contact, while errors will provide information on what went wrong.
Check the status
and message
fields in the response to verify the success of your API call. For error handling, inspect the error.response.data
to understand the issue and make necessary adjustments.
Verifying API Call Success in Zoho CRM Sandbox
To ensure your API call was successful, log in to your Zoho CRM sandbox environment and verify the contact details. If you created a new contact, it should appear in the contacts list. For updates, check that the contact's information reflects the changes you made.
By following these steps, you can efficiently create and update contacts in Zoho CRM using JavaScript, enhancing your CRM data management capabilities.
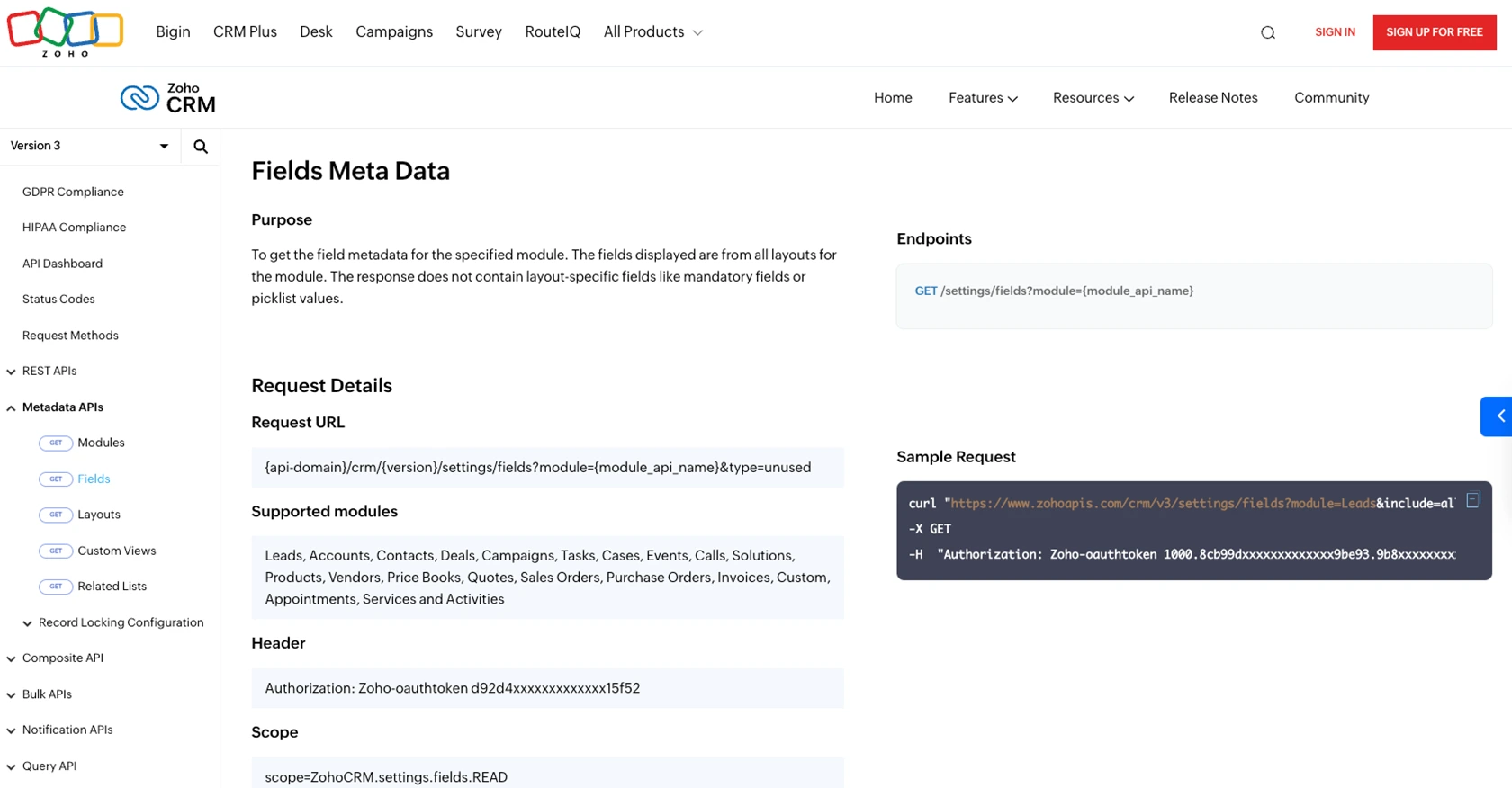
Conclusion: Best Practices for Zoho CRM API Integration and Next Steps
Integrating with the Zoho CRM API using JavaScript offers a powerful way to automate and enhance your CRM processes. By following the steps outlined in this guide, you can efficiently create and update contact records, ensuring your CRM data remains accurate and up-to-date.
Best Practices for Secure and Efficient Zoho CRM API Usage
- Secure Storage of Credentials: Always store your OAuth credentials, such as Client ID and Client Secret, securely. Avoid hardcoding them in your source code.
- Handle Rate Limiting: Zoho CRM imposes API rate limits. Monitor your API usage and implement retry logic to handle rate limit errors gracefully. For more details, refer to the Zoho CRM API Limits documentation.
- Data Standardization: Ensure consistent data formats when integrating with Zoho CRM to maintain data integrity across systems.
- Error Handling: Implement robust error handling to manage API call failures and log errors for troubleshooting.
Enhance Your Integration Strategy with Endgrate
For developers looking to streamline their integration processes further, consider leveraging Endgrate. With Endgrate, you can:
- Save time and resources by outsourcing complex integrations and focusing on your core product development.
- Build once for each use case and apply it across multiple integrations, reducing redundancy and effort.
- Provide an intuitive integration experience for your customers, enhancing user satisfaction and engagement.
Visit Endgrate to learn more about how you can optimize your integration efforts and deliver seamless experiences to your users.
Read More
- https://endgrate.com/provider/zohocrm
- https://www.zoho.com/crm/developer/docs/api/v3/oauth-overview.html
- https://www.zoho.com/crm/developer/docs/api/v3/scopes.html
- https://www.zoho.com/crm/developer/docs/api/v3/register-client.html
- https://www.zoho.com/crm/developer/docs/api/v3/api-limits.html
- https://www.zoho.com/crm/developer/docs/api/v3/status-codes.html
- https://www.zoho.com/crm/developer/docs/api/v3/field-meta.html
- https://www.zoho.com/crm/developer/docs/api/v3/search-records.html
- https://www.zoho.com/crm/developer/docs/api/v3/insert-records.html
- https://www.zoho.com/crm/developer/docs/api/v3/update-records.html
Ready to get started?