How to Get Users with the Zendesk Support API in PHP
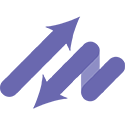
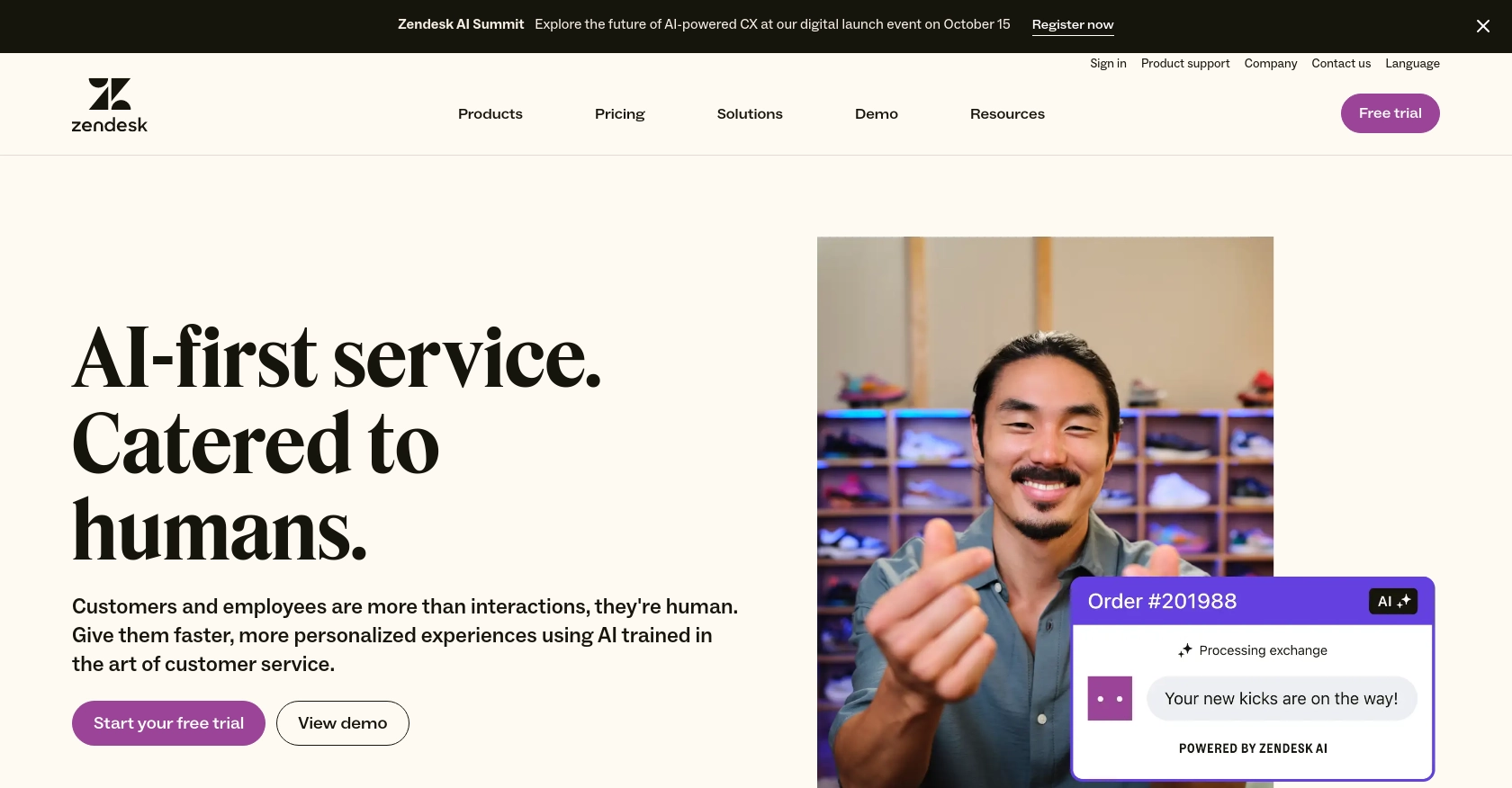
Introduction to Zendesk Support
Zendesk Support is a powerful customer service platform that enables businesses to manage and improve their customer interactions. With features like ticketing, self-service, and customer engagement tools, Zendesk Support helps organizations deliver exceptional customer experiences.
Developers may want to integrate with the Zendesk Support API to access and manage user data efficiently. For example, you can retrieve user information to personalize support interactions or automate user management tasks. This integration can streamline operations and enhance customer satisfaction.
Setting Up Your Zendesk Support Test/Sandbox Account
Before you can start integrating with the Zendesk Support API, you need to set up a test or sandbox account. This will allow you to safely experiment with API calls without affecting your live data.
Creating a Zendesk Support Sandbox Account
If you don't already have a Zendesk account, you can sign up for a free trial or a sandbox account. Follow these steps to get started:
- Visit the Zendesk registration page and fill out the required information to create your account.
- Once your account is created, log in to the Zendesk Admin Center.
- Navigate to Admin Center > Apps and integrations > APIs > Zendesk API.
Registering Your Application for OAuth Authentication
Since the Zendesk Support API uses OAuth for authentication, you'll need to register your application to obtain the necessary credentials:
- In the Admin Center, click on the OAuth Clients tab.
- Click Add OAuth client to create a new client.
- Fill in the following fields:
- Client Name: Enter a name for your application.
- Description: Optionally, provide a short description.
- Company: Optionally, enter your company name.
- Redirect URLs: Enter the URL where Zendesk should redirect users after authorization.
- Click Save to generate your OAuth credentials.
- Copy the Client ID and Client Secret and store them securely. You will need these to authenticate API requests.
Generating an OAuth Access Token
With your OAuth client set up, you can now generate an access token to authenticate your API requests:
- Direct users to the Zendesk authorization page using the following URL format:
https://{subdomain}.zendesk.com/oauth/authorizations/new?response_type=code&client_id={your_client_id}&redirect_uri={your_redirect_url}&scope=read
- Once the user authorizes the application, Zendesk will redirect them to your specified redirect URL with an authorization code.
- Exchange the authorization code for an access token by making a POST request to:
https://{subdomain}.zendesk.com/oauth/tokens
Include the following parameters in the request body:
{ "grant_type": "authorization_code", "code": "{authorization_code}", "client_id": "{your_client_id}", "client_secret": "{your_client_secret}", "redirect_uri": "{your_redirect_url}" }
- Zendesk will respond with an access token, which you can use to authenticate your API requests.
For more detailed information on OAuth authentication, refer to the Zendesk OAuth documentation.
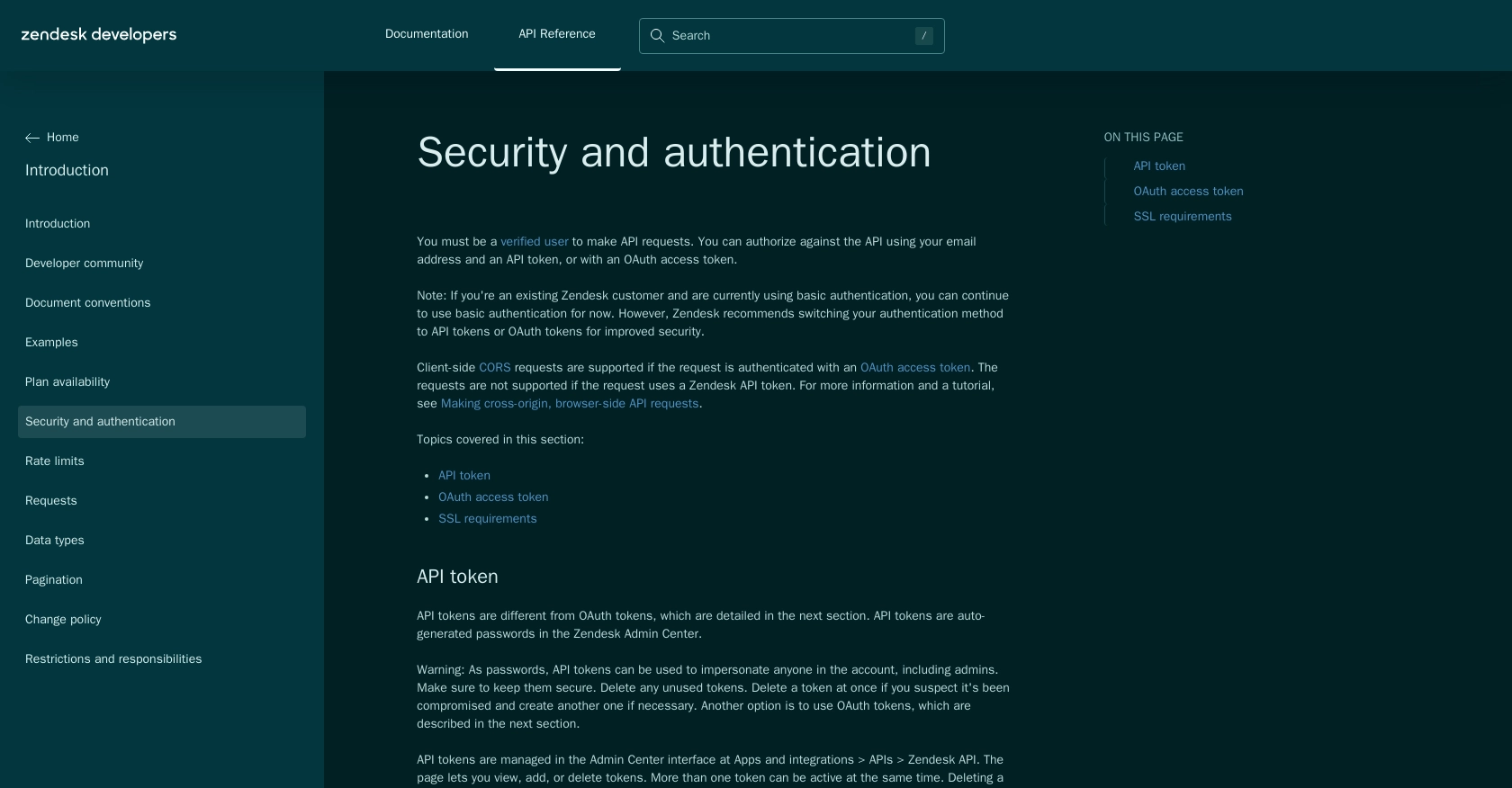
sbb-itb-96038d7
Making API Calls to Retrieve Users with Zendesk Support API in PHP
To interact with the Zendesk Support API and retrieve user data, you'll need to use PHP to make HTTP requests. This section will guide you through setting up your PHP environment, making the API call, and handling the response.
Setting Up Your PHP Environment for Zendesk Support API
Before making API calls, ensure you have the following prerequisites:
- PHP 7.4 or later installed on your machine.
- Composer for managing dependencies.
Install the Guzzle HTTP client, which simplifies making HTTP requests in PHP:
composer require guzzlehttp/guzzle
Writing PHP Code to Retrieve Users from Zendesk Support
Create a new PHP file named get_zendesk_users.php
and add the following code:
request('GET', "https://{$subdomain}.zendesk.com/api/v2/users.json", [
'headers' => [
'Authorization' => "Bearer {$accessToken}",
'Content-Type' => 'application/json',
]
]);
if ($response->getStatusCode() === 200) {
$users = json_decode($response->getBody(), true);
foreach ($users['users'] as $user) {
echo "ID: " . $user['id'] . " - Name: " . $user['name'] . "\n";
}
} else {
echo "Failed to retrieve users. Status code: " . $response->getStatusCode();
}
?>
Replace your_subdomain
and your_access_token
with your actual Zendesk subdomain and OAuth access token.
Running the PHP Script and Verifying the Output
Execute the script from the command line:
php get_zendesk_users.php
If successful, the script will output a list of users with their IDs and names. If there are any errors, ensure your access token is valid and check the response status code for more information.
Handling Errors and Rate Limits with Zendesk Support API
Zendesk API has a rate limit of 700 requests per minute. If you exceed this limit, you'll receive a 429 Too Many Requests status code. Handle this by checking the response and implementing a retry mechanism:
if ($response->getStatusCode() === 429) {
$retryAfter = $response->getHeader('Retry-After')[0];
sleep($retryAfter);
// Retry the request
}
For more details on error handling and rate limits, refer to the Zendesk API documentation.
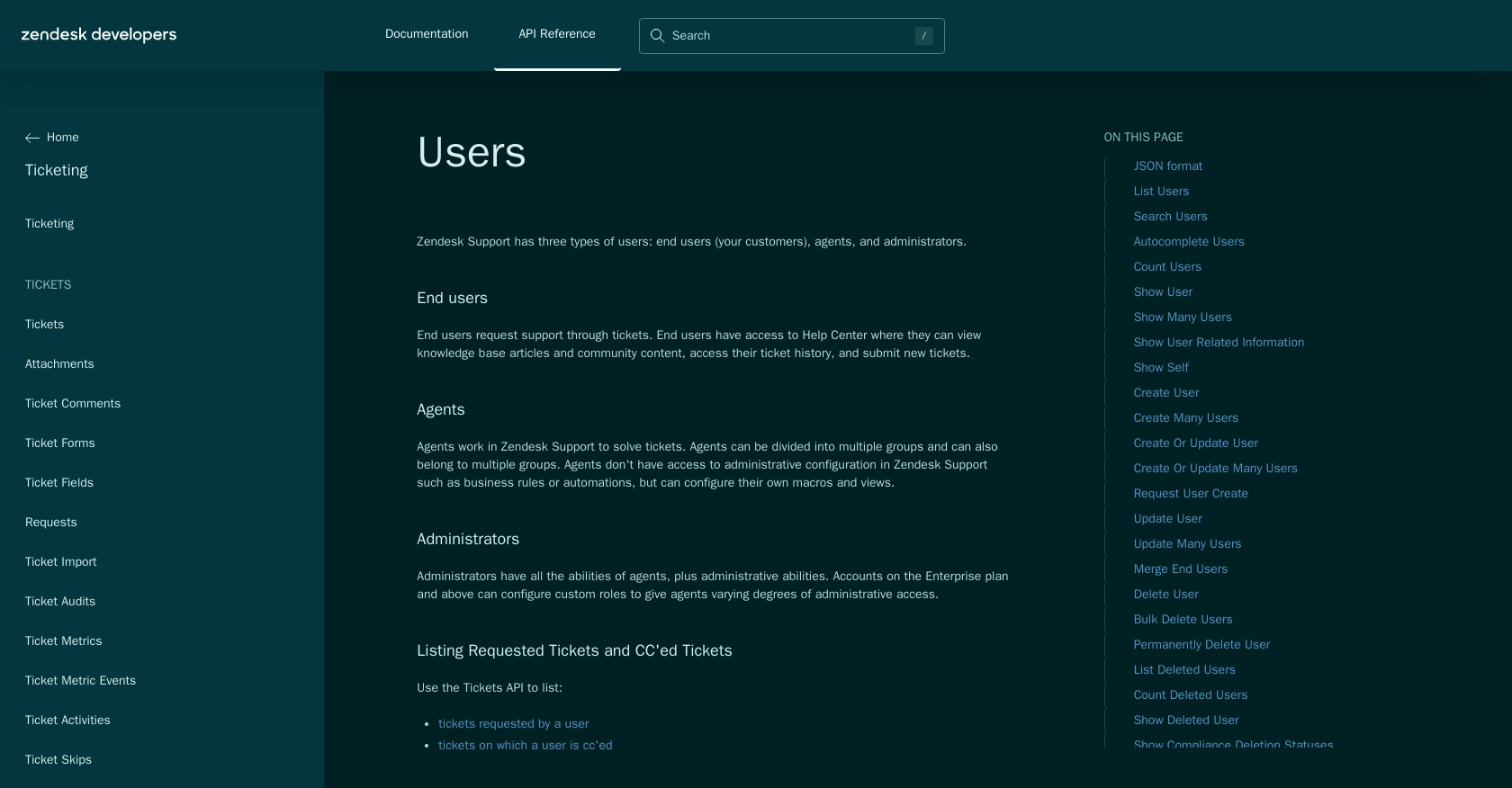
Conclusion and Best Practices for Integrating with Zendesk Support API in PHP
Integrating with the Zendesk Support API using PHP can significantly enhance your ability to manage user data and automate customer support processes. By following the steps outlined in this guide, you can efficiently retrieve user information and handle API interactions with ease.
Best Practices for Secure and Efficient Zendesk Support API Integration
- Securely Store Credentials: Always store your OAuth credentials and access tokens securely. Avoid hardcoding them in your scripts and consider using environment variables or secure vaults.
- Handle Rate Limits Gracefully: Implement logic to handle the 429 Too Many Requests status code by respecting the Retry-After header and retrying requests after the specified interval.
- Optimize API Calls: Use pagination to efficiently handle large datasets and reduce the number of API calls. Refer to the Zendesk pagination documentation for more details.
- Monitor API Usage: Regularly monitor your API usage to ensure you stay within the rate limits and optimize your integration for performance.
Enhance Your Integration Strategy with Endgrate
While integrating with Zendesk Support API can be straightforward, managing multiple integrations across different platforms can become complex. Endgrate offers a unified API solution that simplifies integration management, allowing you to focus on your core product development.
By leveraging Endgrate, you can streamline your integration processes, reduce development time, and provide a seamless experience for your users. Visit Endgrate to learn more about how you can optimize your integration strategy.
Read More
- https://endgrate.com/provider/zendesksupport
- https://developer.zendesk.com/api-reference/introduction/security-and-auth/
- https://support.zendesk.com/hc/en-us/articles/4408845965210-Using-OAuth-authentication-with-your-application
- https://developer.zendesk.com/api-reference/introduction/rate-limits/
- https://developer.zendesk.com/api-reference/introduction/pagination/
- https://developer.zendesk.com/api-reference/ticketing/users/users/
Ready to get started?