How to Send Messages (Channel) with the Slack API in PHP
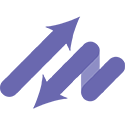
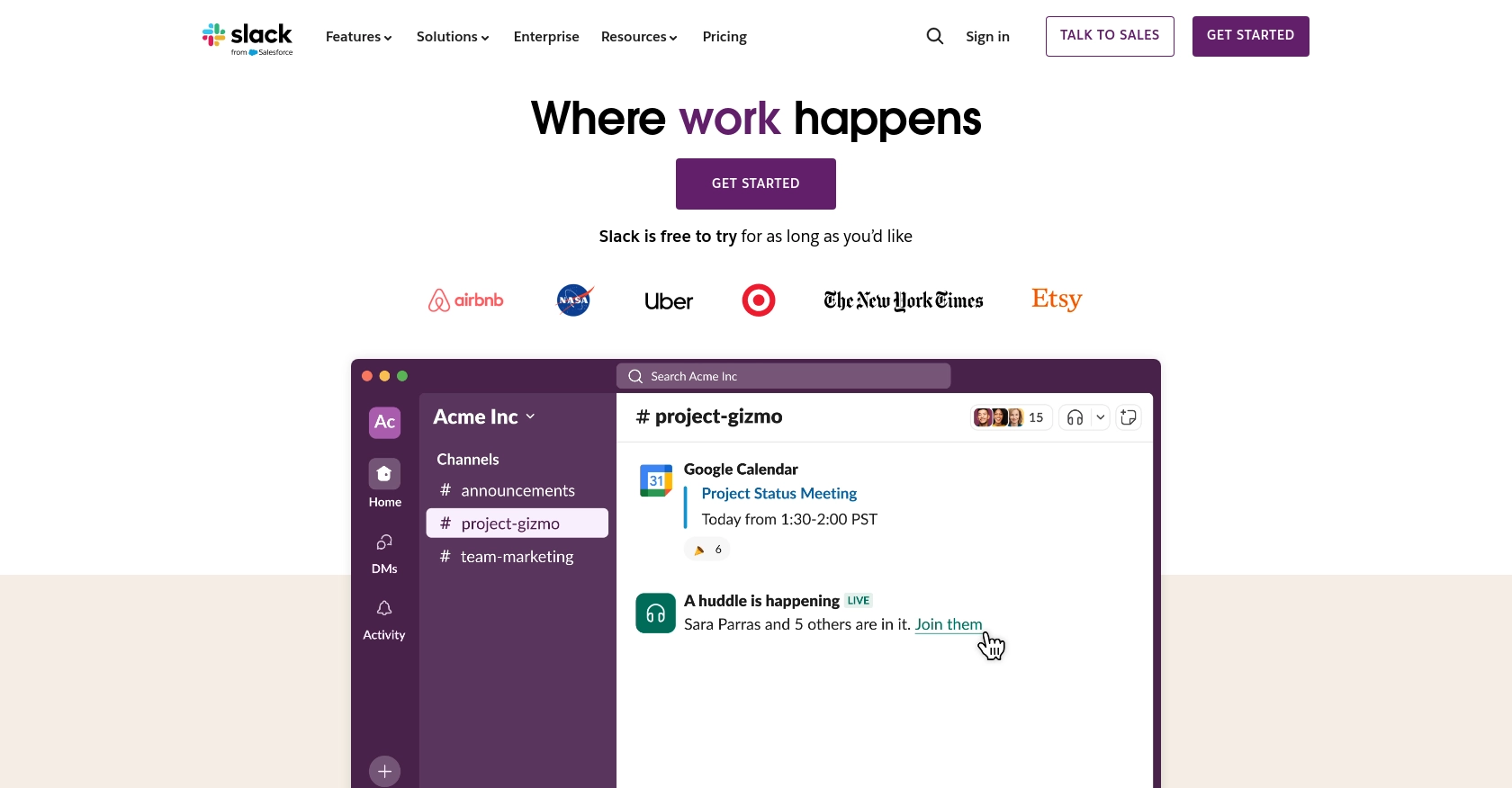
Introduction to Slack API for Messaging
Slack is a powerful collaboration platform that facilitates communication and teamwork through channels, direct messages, and integrations. It is widely used by businesses to enhance productivity and streamline workflows. With its robust API, developers can create custom applications that interact with Slack, enabling automated messaging, notifications, and more.
Integrating with Slack's API allows developers to send messages to channels programmatically, offering opportunities to automate communication processes. For example, a developer might use the Slack API to send automated alerts to a team channel whenever a critical event occurs in a monitoring system, ensuring that the team is promptly informed.
This article will guide you through the process of using PHP to send messages to a Slack channel via the Slack API. You'll learn how to set up your Slack app, authenticate using OAuth, and execute API calls to post messages, enhancing your application's interaction with Slack.
Setting Up Your Slack Test or Sandbox Account
Before you can start sending messages using the Slack API in PHP, you need to set up a Slack app and configure it for API access. This involves creating a test or sandbox account, which allows you to safely develop and test your integrations without affecting your live workspace.
Creating a Slack App for API Integration
To begin, you'll need to create a Slack app. Follow these steps to set up your app:
- Visit the Slack Apps page and click on "Create New App".
- Select "From scratch" and enter your app's name, such as "PHP Messaging Bot".
- Choose the workspace where you want to develop your app. You can distribute it to other workspaces later.
- Click "Create App" to proceed.
Configuring OAuth for Slack API Access
Slack uses OAuth 2.0 for authentication. You'll need to configure your app to use OAuth to interact with the Slack API:
- Navigate to the "OAuth & Permissions" section in your app's settings.
- Under "Scopes", add the following scopes to your app:
chat:write
- Allows your app to send messages.channels:read
- Allows your app to access public channels.
- Click "Save Changes" to apply the scopes.
- Scroll to the top and click "Install App to Workspace". Follow the prompts to authorize your app.
- After installation, you'll receive an OAuth access token. Store this token securely, as it will be used to authenticate your API requests.
Testing Your Slack App in a Sandbox Environment
With your Slack app set up and authorized, you can now test your API interactions in a sandbox environment:
- Create a new channel in your Slack workspace specifically for testing purposes.
- Invite your Slack app to this channel using the
/invite
command. - Use this channel to test sending messages and other interactions without affecting your main workspace.
By following these steps, you ensure that your Slack app is properly configured and ready for development and testing. This setup allows you to safely experiment with the Slack API and refine your integration before deploying it to a live environment.
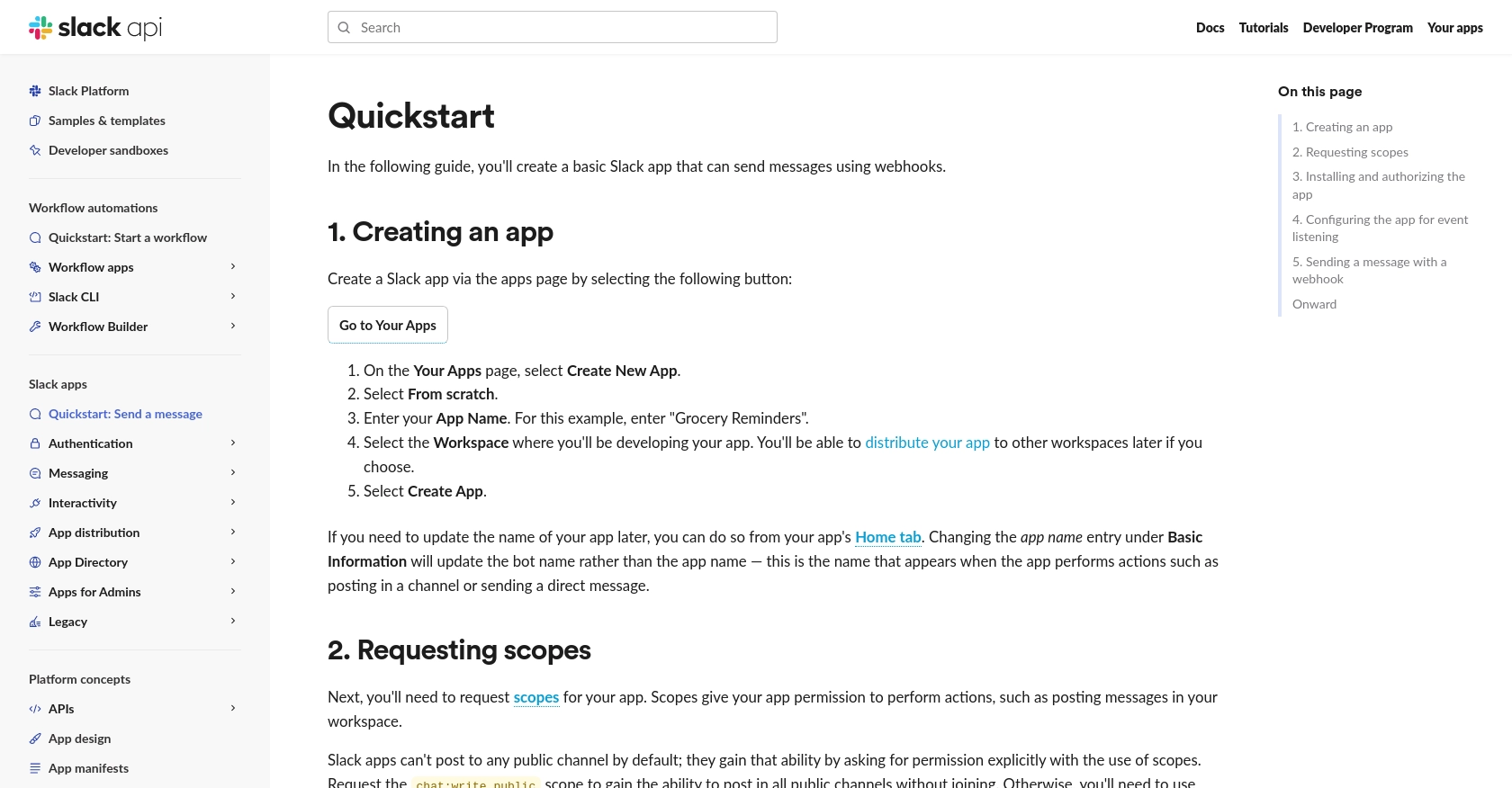
sbb-itb-96038d7
Executing Slack API Calls to Send Messages Using PHP
Once your Slack app is set up and authorized, you can begin sending messages to Slack channels using PHP. This section will guide you through the process of making API calls to post messages, ensuring seamless integration with Slack's messaging platform.
Prerequisites for Sending Messages with Slack API in PHP
Before diving into the code, ensure you have the following prerequisites:
- PHP 7.4 or higher installed on your machine.
- Composer for managing dependencies.
- The
guzzlehttp/guzzle
package for making HTTP requests. Install it using the command:
composer require guzzlehttp/guzzle
Composing a Slack Message with PHP
To send a message to a Slack channel, you'll need to construct a JSON payload containing the channel ID and the message text. Here's a basic example:
use GuzzleHttp\Client;
$client = new Client();
$channelId = 'YOUR_CHANNEL_ID';
$message = 'Hello, Slack!';
$response = $client->post('https://slack.com/api/chat.postMessage', [
'headers' => [
'Authorization' => 'Bearer YOUR_OAUTH_ACCESS_TOKEN',
'Content-Type' => 'application/json',
],
'json' => [
'channel' => $channelId,
'text' => $message,
],
]);
$result = json_decode($response->getBody(), true);
if ($result['ok']) {
echo "Message sent successfully!";
} else {
echo "Error: " . $result['error'];
}
Verifying Successful Message Delivery in Slack
After executing the API call, verify that the message appears in the specified Slack channel. You can do this by checking the channel directly in your Slack workspace. If the message is not visible, review the response from the API call for any error messages.
Handling Errors and Common Slack API Error Codes
When interacting with the Slack API, it's crucial to handle potential errors gracefully. Common error codes include:
invalid_auth
: The provided token is invalid.channel_not_found
: The specified channel ID does not exist.rate_limited
: The app has exceeded the rate limit for API requests.
Refer to the Slack API documentation for a comprehensive list of error codes and their meanings.
Testing and Debugging Your Slack API Integration
To ensure your integration works as expected, test it thoroughly in your sandbox environment. Use logging to capture API responses and errors, which can help in diagnosing issues. Adjust your code as needed based on the feedback from these tests.
By following these steps, you can effectively send messages to Slack channels using PHP, enhancing your application's communication capabilities with Slack's robust API.
.webp)
Best Practices for Using Slack API in PHP
When integrating with the Slack API using PHP, it's essential to follow best practices to ensure security, efficiency, and maintainability. Here are some recommendations:
- Secure Storage of OAuth Tokens: Store your OAuth tokens securely, using environment variables or secure vaults, to prevent unauthorized access.
- Handle Rate Limiting: Be aware of Slack's rate limits and implement retry logic with exponential backoff to handle
rate_limited
errors gracefully. Refer to the Slack API documentation for specific rate limit details. - Data Transformation and Standardization: Ensure that data sent to and received from Slack is properly formatted and standardized to maintain consistency across your application.
- Error Handling and Logging: Implement robust error handling and logging to capture and diagnose issues quickly, improving the reliability of your integration.
Enhancing Integration Efficiency with Endgrate
Building and maintaining integrations can be time-consuming and complex. Endgrate offers a solution by providing a unified API endpoint that connects to multiple platforms, including Slack. By using Endgrate, you can:
- Save time and resources by outsourcing integrations, allowing you to focus on your core product.
- Build once for each use case instead of multiple times for different integrations.
- Provide an intuitive integration experience for your customers, enhancing user satisfaction.
Explore how Endgrate can streamline your integration processes by visiting Endgrate.
Conclusion: Streamlining Communication with Slack API
Integrating with the Slack API using PHP empowers developers to automate and enhance communication workflows within their applications. By following the steps outlined in this guide, you can effectively send messages to Slack channels, ensuring timely and efficient communication.
Whether you're sending alerts, notifications, or updates, leveraging Slack's robust API capabilities can significantly improve your team's collaboration and productivity. Embrace these integrations to transform your application's interaction with Slack and unlock new possibilities for automation and efficiency.
Read More
Ready to get started?