Using the Airtable API to Create Or Update Records in Python
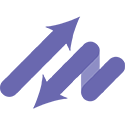
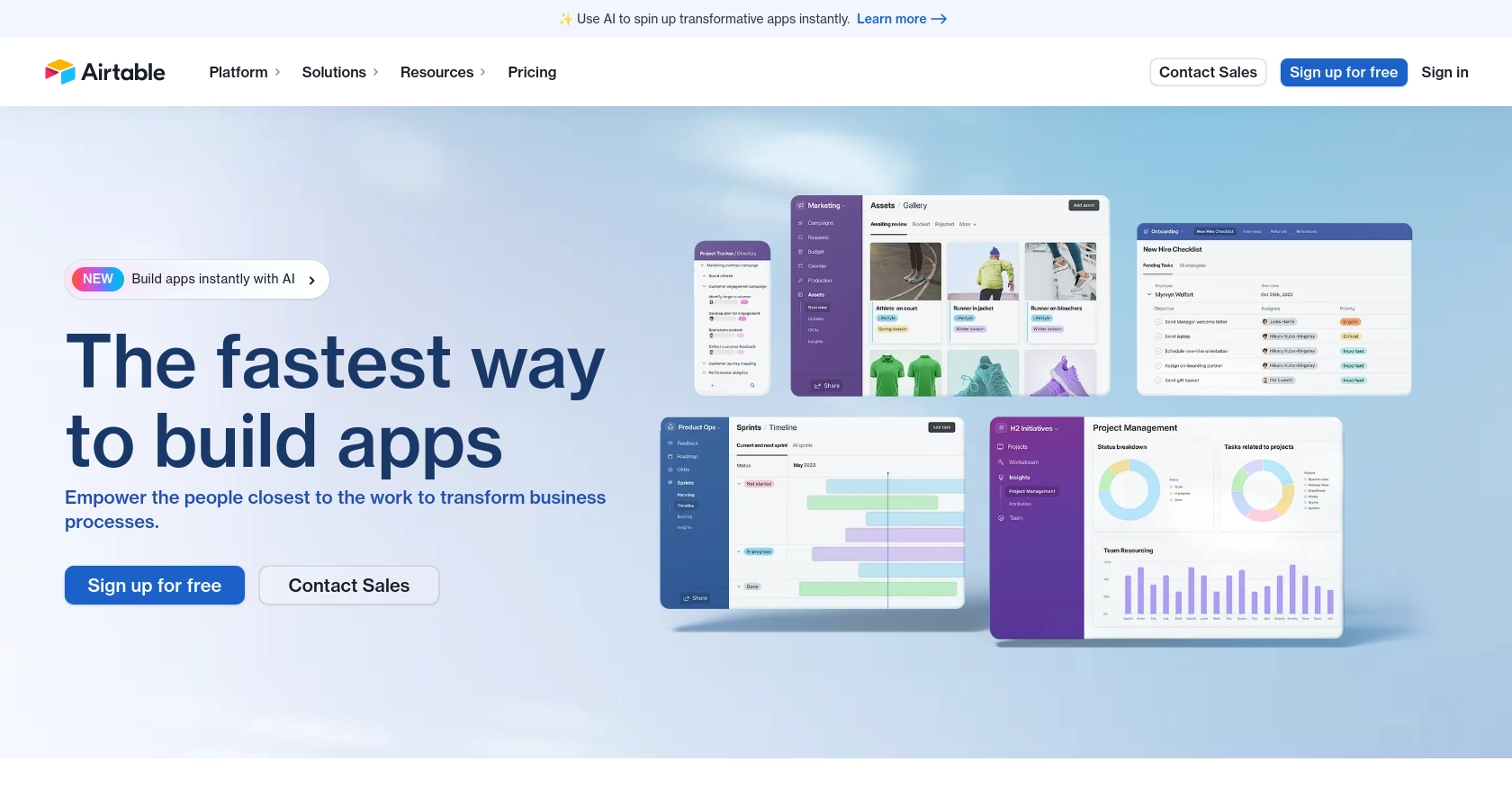
Introduction to Airtable API Integration
Airtable is a versatile cloud-based platform that combines the simplicity of a spreadsheet with the power of a database. It is widely used by businesses and developers to organize and manage data efficiently. With its intuitive interface and robust API, Airtable allows users to create custom applications tailored to their specific needs.
Integrating with the Airtable API enables developers to automate data management tasks, such as creating or updating records programmatically. For example, a developer might use the Airtable API to automatically update inventory records in a retail application, ensuring that stock levels are always current and accurate.
Setting Up Your Airtable Test or Sandbox Account
Before diving into the Airtable API integration, it's essential to set up a test or sandbox account. This allows you to experiment with API calls without affecting your live data. Airtable offers a straightforward process to get started with their API using OAuth authentication.
Creating an Airtable Account
If you don't already have an Airtable account, you can sign up for a free account on the Airtable website. Follow the instructions to create your account and log in.
Setting Up a Base for Testing
Once logged in, create a new base to use for testing your API calls:
- Click on the "Add a base" button from your Airtable dashboard.
- Select "Start from scratch" and give your base a name, such as "API Test Base".
- Add a table with some sample fields and records to interact with during testing.
Registering an OAuth Integration with Airtable
To authenticate API requests, you'll need to register an OAuth integration:
- Navigate to the OAuth integration page.
- Click on "Create an OAuth integration" and fill in the required details, including the integration name and redirect URI.
- Set the necessary scopes, such as
data.records:write
, to allow creating and updating records. - Save your integration to generate a client ID and client secret.
Obtaining OAuth Access Tokens
With your OAuth integration set up, you can now obtain access tokens to authenticate API requests:
- Direct users to the authorization URL to grant access to your integration.
- Exchange the authorization code for an access token using the token endpoint.
- Store the access token securely, as it will be used in the authorization header for API requests.
For detailed steps on obtaining OAuth tokens, refer to the Airtable OAuth reference.
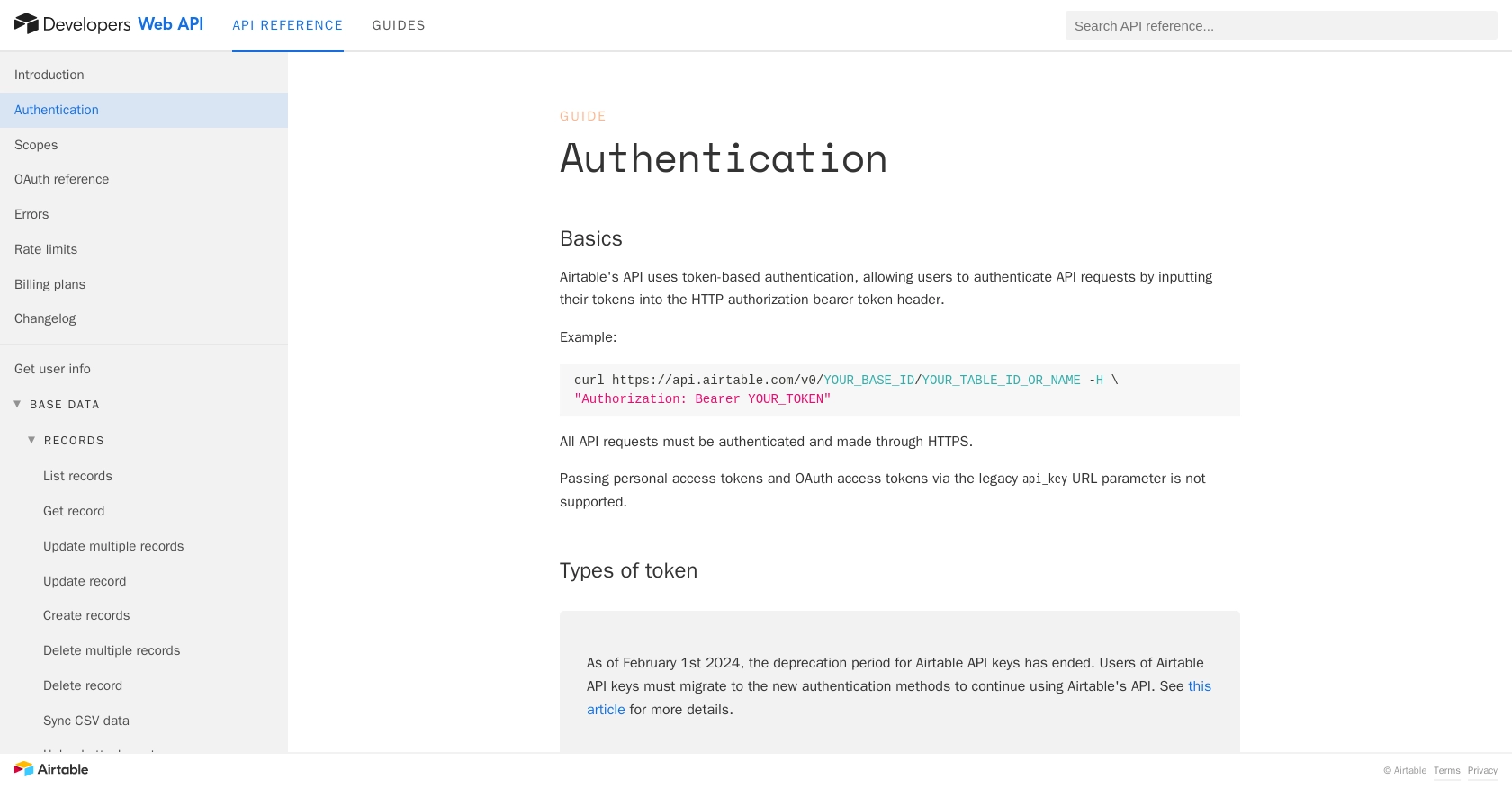
sbb-itb-96038d7
Making API Calls to Create or Update Records in Airtable Using Python
To interact with the Airtable API for creating or updating records, you'll need to use Python, a versatile and widely-used programming language. This section will guide you through the process of setting up your Python environment and making API calls to Airtable.
Setting Up Your Python Environment for Airtable API Integration
Before making API calls, ensure you have Python installed on your machine. This tutorial uses Python 3.11.1. Additionally, you'll need the requests
library to handle HTTP requests.
- Install Python from the official website if you haven't already.
- Install the
requests
library using pip:
pip install requests
Creating Records in Airtable Using Python
To create records in Airtable, you'll need to make a POST request to the Airtable API endpoint. Here's how you can do it:
import requests
# Define the API endpoint and headers
url = "https://api.airtable.com/v0/YOUR_BASE_ID/YOUR_TABLE_NAME"
headers = {
"Authorization": "Bearer YOUR_ACCESS_TOKEN",
"Content-Type": "application/json"
}
# Define the data for the new record
data = {
"records": [
{
"fields": {
"Name": "New Record",
"Description": "This is a new record"
}
}
]
}
# Make the POST request to create the record
response = requests.post(url, json=data, headers=headers)
# Check if the request was successful
if response.status_code == 200:
print("Record created successfully:", response.json())
else:
print("Failed to create record:", response.json())
Replace YOUR_BASE_ID
, YOUR_TABLE_NAME
, and YOUR_ACCESS_TOKEN
with your actual base ID, table name, and access token. Upon successful execution, you should see the details of the newly created record.
Updating Records in Airtable Using Python
To update existing records, you'll need to make a PATCH request. Here's an example:
import requests
# Define the API endpoint and headers
url = "https://api.airtable.com/v0/YOUR_BASE_ID/YOUR_TABLE_NAME/YOUR_RECORD_ID"
headers = {
"Authorization": "Bearer YOUR_ACCESS_TOKEN",
"Content-Type": "application/json"
}
# Define the updated data for the record
data = {
"fields": {
"Description": "Updated description"
}
}
# Make the PATCH request to update the record
response = requests.patch(url, json=data, headers=headers)
# Check if the request was successful
if response.status_code == 200:
print("Record updated successfully:", response.json())
else:
print("Failed to update record:", response.json())
Replace YOUR_BASE_ID
, YOUR_TABLE_NAME
, YOUR_RECORD_ID
, and YOUR_ACCESS_TOKEN
with your actual base ID, table name, record ID, and access token. This will update the specified fields of the record.
Handling Errors and Verifying API Requests in Airtable
When making API calls, it's crucial to handle potential errors. Airtable's API uses standard HTTP status codes to indicate success or failure:
- 200 OK: Request completed successfully.
- 400 Bad Request: Invalid request encoding.
- 401 Unauthorized: Invalid credentials.
- 429 Too Many Requests: Rate limit exceeded. Wait 30 seconds before retrying.
For more detailed error information, refer to the Airtable API error documentation.
To verify that your requests have succeeded, check the Airtable base to see if the records have been created or updated as expected.
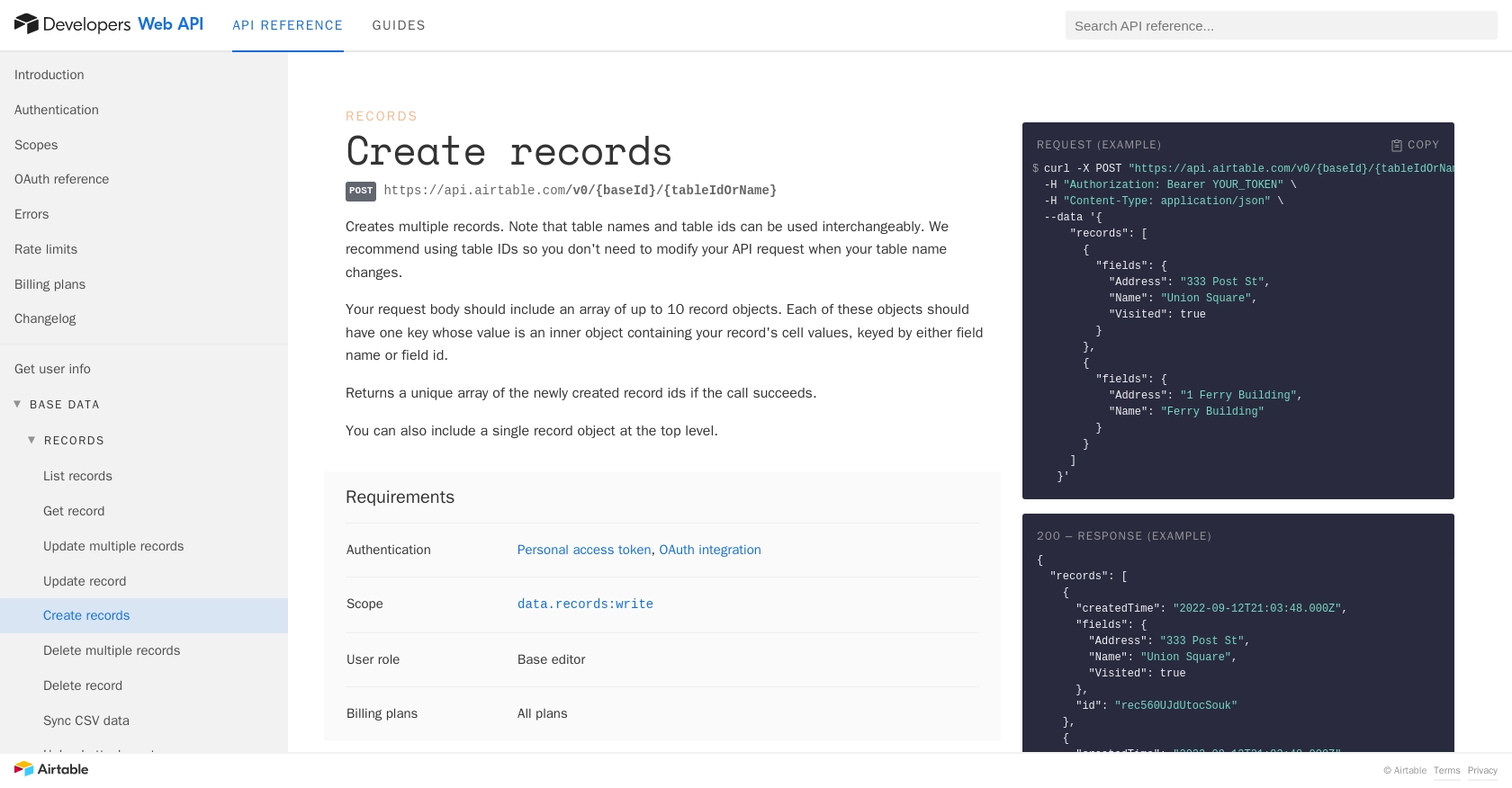
Best Practices for Airtable API Integration
When integrating with the Airtable API, it's important to follow best practices to ensure security, efficiency, and maintainability of your application.
- Securely Store Credentials: Always store your OAuth access tokens securely. Avoid hardcoding them in your source code. Consider using environment variables or secure vaults to manage sensitive information.
- Handle Rate Limiting: Airtable imposes a rate limit of 5 requests per second per base. Implement back-off and retry logic to handle 429 status codes gracefully. For more details, refer to the Airtable rate limits documentation.
- Data Transformation: Ensure that data fields are standardized and transformed as needed before making API calls. This helps maintain data integrity and consistency across your applications.
Streamlining Integrations with Endgrate
Building and maintaining integrations can be time-consuming and complex. Endgrate simplifies this process by providing a unified API endpoint that connects to multiple platforms, including Airtable. With Endgrate, you can:
- Save time and resources by outsourcing integrations and focusing on your core product.
- Build once for each use case instead of multiple times for different integrations.
- Offer an easy, intuitive integration experience for your customers.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate.
Read More
- https://endgrate.com/provider/airtable
- https://airtable.com/developers/web/api/authentication
- https://airtable.com/developers/web/api/scopes
- https://airtable.com/developers/web/api/oauth-reference
- https://airtable.com/developers/web/api/errors
- https://airtable.com/developers/web/api/rate-limits
- https://airtable.com/developers/web/api/create-records
- https://airtable.com/developers/web/api/update-record
Ready to get started?