How to Create Products with the Stamped API in PHP
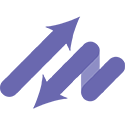
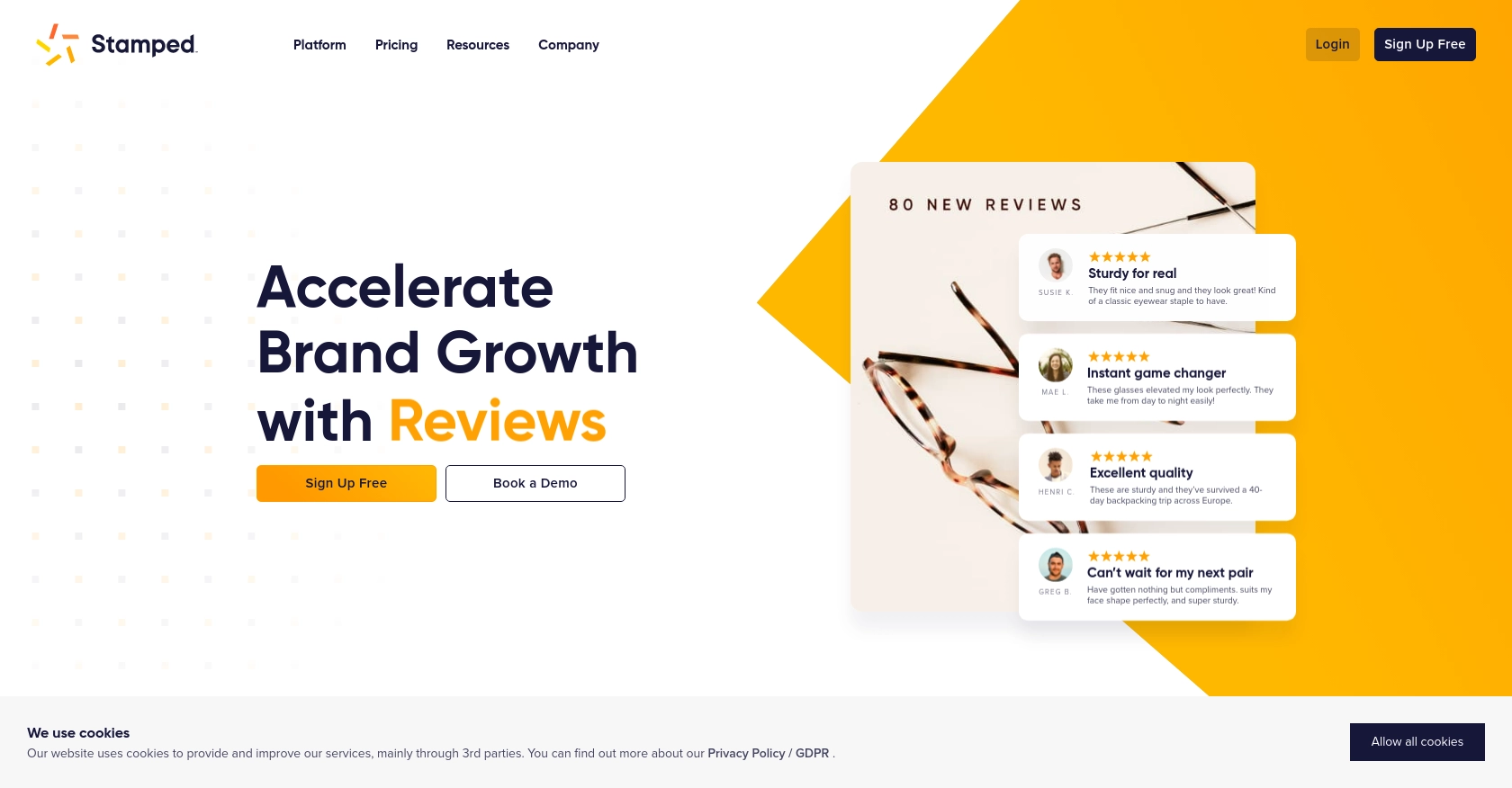
Introduction to Stamped API for Product Management
Stamped is a powerful platform that empowers over 75,000 brands to enhance their growth through customer reviews, loyalty programs, and more. It offers a comprehensive suite of tools designed to help businesses engage with their customers and drive sales.
For developers working with e-commerce platforms, integrating with the Stamped API can significantly streamline product management tasks. By leveraging the API, developers can automate the creation and management of product listings, ensuring that product data is consistently up-to-date across platforms.
In this article, we'll explore how to create products using the Stamped API with PHP. This integration can be particularly useful for businesses looking to automate their product catalog updates, ensuring seamless synchronization between their e-commerce store and Stamped's platform.
Setting Up Your Stamped Test Account for API Integration
Before you can start creating products with the Stamped API using PHP, you'll need to set up a test account. This will allow you to safely experiment with the API without affecting your live data.
Creating a Stamped Account
To begin, you'll need to sign up for a Stamped account. Please note that access to the API requires a Professional or Enterprise plan. Visit the Stamped website to create your account.
Generating API Keys for Authentication
Once your account is set up, you'll need to generate API keys to authenticate your requests. Follow these steps to obtain your keys:
- Log in to your Stamped account and navigate to the Control Panel.
- Go to the API Keys section.
- Generate a new set of API keys. You will receive a public API key and a private API key.
- Store these keys securely, as they will be used for authenticating your API requests.
Understanding Stamped API Authentication
The Stamped API uses HTTP Basic Auth for authentication. When making requests to private endpoints, you will need to provide your public API key as the username and your private API key as the password. Public endpoints may only require the store hash or public key.
Configuring Your PHP Environment
Ensure your PHP environment is ready for making API calls. You will need to have PHP installed on your system, along with any necessary extensions for handling HTTP requests.
With your test account and API keys ready, you're all set to start integrating with the Stamped API. In the next section, we'll dive into the PHP code required to create products using the API.
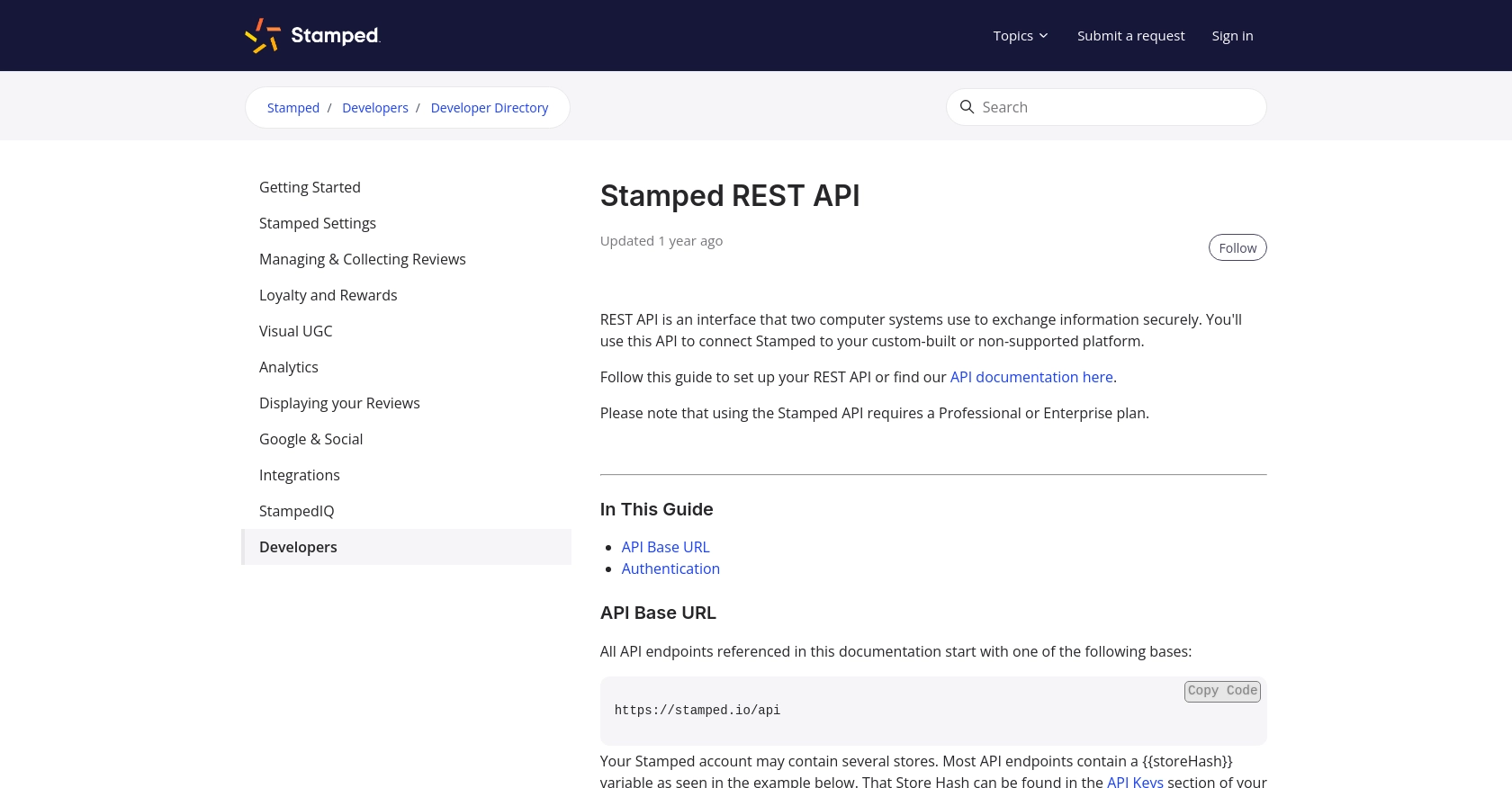
sbb-itb-96038d7
Making API Calls to Create Products with Stamped API in PHP
To effectively create products using the Stamped API in PHP, you'll need to ensure your PHP environment is properly configured and ready to handle HTTP requests. This section will guide you through the necessary steps and provide sample code to help you make successful API calls.
Setting Up Your PHP Environment for Stamped API Integration
Before diving into the code, make sure you have PHP installed on your system. Additionally, you'll need the cURL
extension enabled to handle HTTP requests. You can verify this by running the following command in your terminal:
php -m | grep curl
If cURL
is not listed, you may need to install or enable it in your PHP configuration.
Creating a Product with Stamped API Using PHP
Once your environment is set up, you can proceed with writing the PHP code to create a product using the Stamped API. Below is an example script that demonstrates how to make a POST request to the Stamped API to create a product.
<?php
// Set your API credentials
$publicApiKey = 'your_public_api_key';
$privateApiKey = 'your_private_api_key';
$storeHash = 'your_store_hash';
// Define the API endpoint
$url = "https://stamped.io/api/v3/merchant/shops/{$storeHash}/products";
// Prepare the product data
$productData = [
'title' => 'Sample Product',
'description' => 'This is a sample product description.',
'price' => 29.99,
'sku' => 'SAMPLE123'
];
// Initialize cURL session
$ch = curl_init($url);
// Set cURL options
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_HTTPAUTH, CURLAUTH_BASIC);
curl_setopt($ch, CURLOPT_USERPWD, "{$publicApiKey}:{$privateApiKey}");
curl_setopt($ch, CURLOPT_POST, true);
curl_setopt($ch, CURLOPT_POSTFIELDS, json_encode($productData));
curl_setopt($ch, CURLOPT_HTTPHEADER, [
'Content-Type: application/json'
]);
// Execute the request
$response = curl_exec($ch);
// Check for errors
if (curl_errno($ch)) {
echo 'Request Error:' . curl_error($ch);
} else {
// Parse and display the response
$responseData = json_decode($response, true);
echo 'Product Created: ' . print_r($responseData, true);
}
// Close cURL session
curl_close($ch);
?>
In this script, replace your_public_api_key
, your_private_api_key
, and your_store_hash
with your actual Stamped API credentials. The $productData
array contains the product details you wish to create.
Verifying the API Request Success
After running the script, you should see a response indicating the product was created successfully. You can verify this by checking your Stamped dashboard to ensure the new product appears in your product listings.
Handling Errors and Troubleshooting
If you encounter any errors, ensure that your API credentials are correct and that your PHP environment is configured properly. Common error codes and their meanings can be found in the Stamped API documentation.
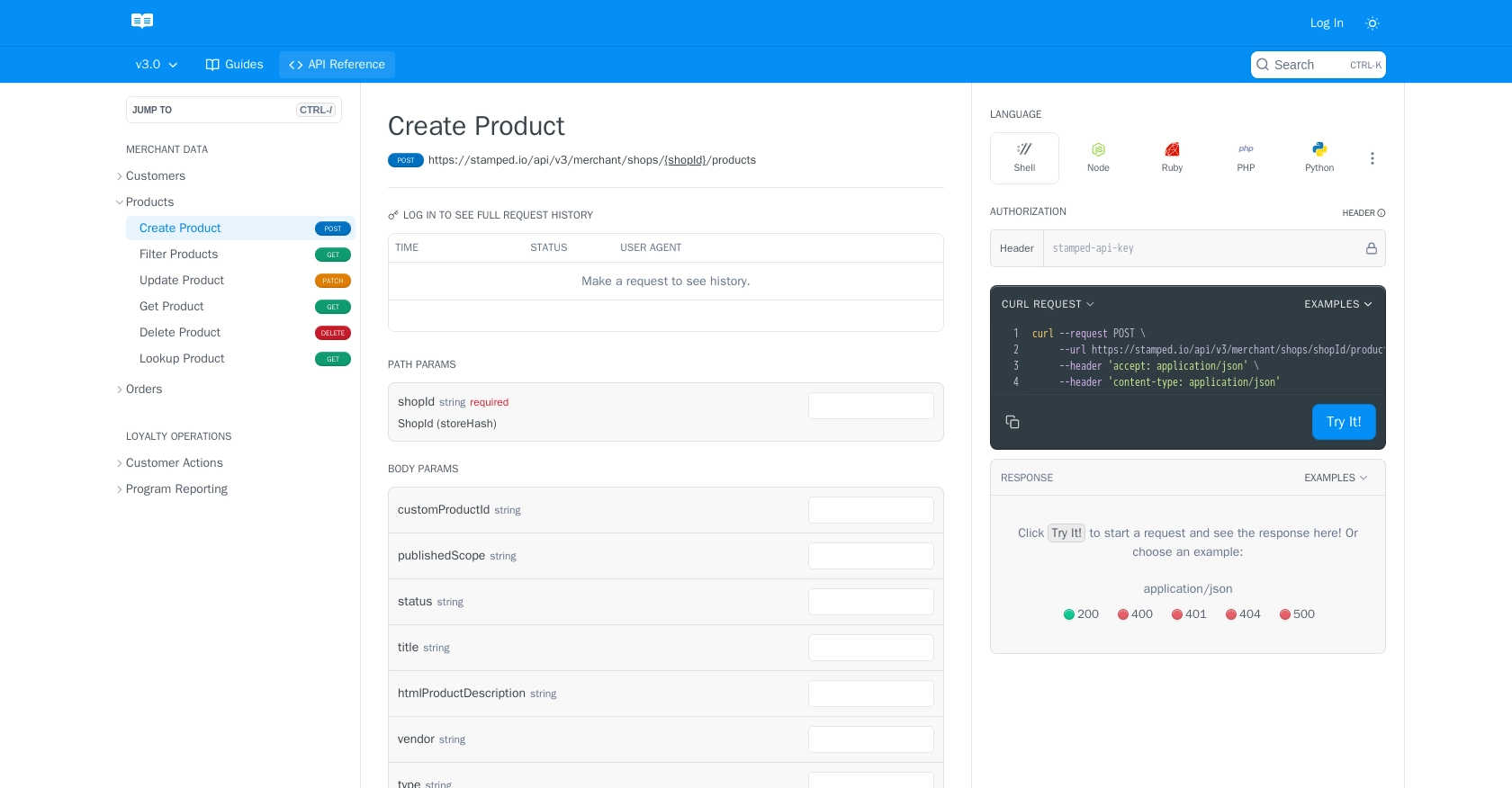
Conclusion and Best Practices for Using Stamped API in PHP
Integrating with the Stamped API using PHP can significantly enhance your e-commerce platform by automating product management tasks. By following the steps outlined in this article, you can efficiently create products and ensure your product data is synchronized across platforms.
Best Practices for Secure and Efficient Stamped API Integration
- Secure Storage of API Credentials: Always store your API keys securely. Consider using environment variables or secure vaults to prevent unauthorized access.
- Handle Rate Limiting: Be mindful of the API rate limits to avoid throttling. Implement exponential backoff strategies to handle rate limit responses gracefully.
- Data Standardization: Ensure that your product data is consistently formatted before sending it to the API. This helps maintain data integrity across systems.
- Error Handling: Implement robust error handling to manage API response errors effectively. Refer to the Stamped API documentation for common error codes and solutions.
Streamlining Integrations with Endgrate
If managing multiple integrations becomes overwhelming, consider using Endgrate to simplify the process. Endgrate allows you to build once for each use case and connect with multiple platforms through a single API endpoint. This not only saves time and resources but also provides a seamless integration experience for your customers.
Explore how Endgrate can help you focus on your core product while efficiently managing integrations. Visit Endgrate to learn more about how it can benefit your business.
Read More
Ready to get started?