Using the Applied Epic API to Get People in Python
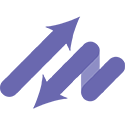
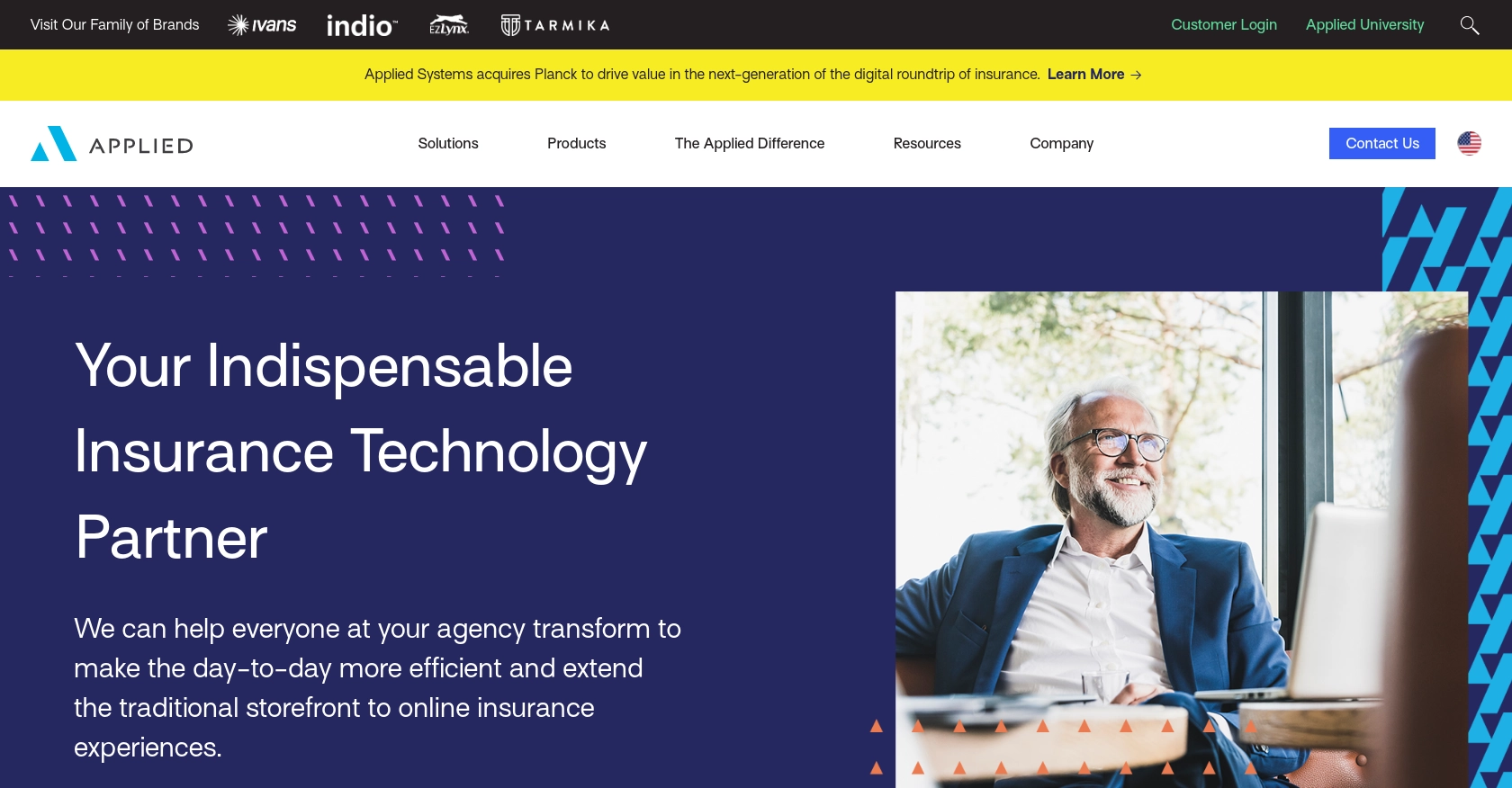
Introduction to Applied Epic API
Applied Epic is a powerful insurance management platform that offers comprehensive solutions for agencies and brokerages. It streamlines operations by integrating various aspects of insurance management, including client data, policy management, and accounting.
Developers may want to connect with the Applied Epic API to efficiently manage and retrieve client information. For example, using the API to access a list of people associated with an account can enhance customer relationship management by providing quick access to client details for personalized service.
Setting Up Your Applied Epic Test/Sandbox Account
Before you can start interacting with the Applied Epic API, you'll need to set up a test or sandbox account. This environment allows you to safely experiment with API calls without affecting live data.
Creating an Applied Epic Developer Account
To begin, you'll need to sign up for a developer account on the Applied Epic Developer Portal. Follow these steps:
- Visit the Applied Epic Developer Portal.
- Click on the "Sign Up" button and fill in the required details, including your name, email, and company information.
- Once registered, verify your email address to activate your account.
Accessing the Sandbox Environment
After setting up your developer account, you can access the sandbox environment:
- Log in to your account on the Applied Epic Developer Portal.
- Navigate to the "Sandbox" section to request access to the test environment.
- Follow the instructions provided to set up your sandbox account, which may include agreeing to terms and conditions.
Configuring OAuth Authentication for API Access
The Applied Epic API uses OAuth for authentication. To configure this, you'll need to create an application within your sandbox account:
- Go to the "Applications" section in your sandbox account.
- Click on "Create New Application" and provide the necessary details such as application name and description.
- Once created, note down the client ID and client secret, as these will be used for OAuth authentication.
Ensure that your application has the necessary scopes for accessing people data, specifically the epic/people:read
scope.
With your sandbox account and OAuth configuration in place, you're ready to start making API calls to the Applied Epic platform.
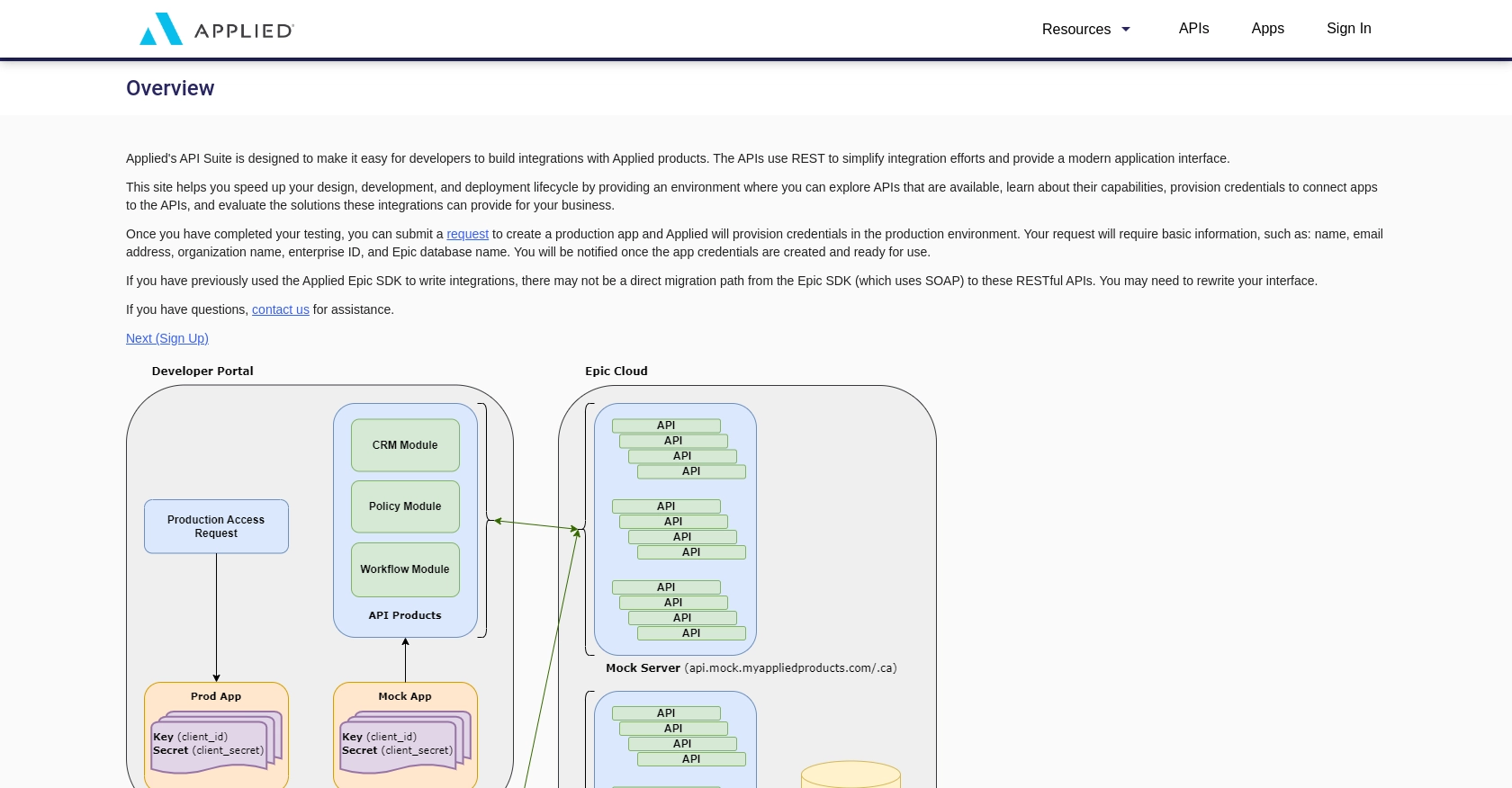
sbb-itb-96038d7
Making API Calls to Retrieve People Data from Applied Epic Using Python
To interact with the Applied Epic API and retrieve people data, you'll need to use Python, a versatile programming language widely used for API integrations. This section will guide you through the necessary steps to make successful API calls.
Setting Up Your Python Environment for Applied Epic API Integration
Before making API calls, ensure you have the following prerequisites installed on your machine:
- Python 3.11.1 or later
- The Python package installer
pip
Once these are installed, open your terminal or command prompt and install the requests
library, which is essential for making HTTP requests:
pip install requests
Writing Python Code to Retrieve People Data from Applied Epic API
Create a new Python file named get_applied_epic_people.py
and add the following code:
import requests
# Set the API endpoint and headers
endpoint = "https://api.myappliedproducts.com/crm/v1/people"
headers = {
"Authorization": "Bearer Your_Access_Token",
"Content-Type": "application/json"
}
# Make a GET request to the API
response = requests.get(endpoint, headers=headers)
# Check if the request was successful
if response.status_code == 200:
# Parse the JSON data from the response
data = response.json()
# Loop through the people and print their information
for person in data["_embedded"]["people"]:
print(f"ID: {person['id']}, Name: {person['firstName']} {person['lastName']}")
else:
print(f"Failed to retrieve people data: {response.status_code} - {response.text}")
Replace Your_Access_Token
with the OAuth token obtained from your sandbox account setup.
Running the Python Script to Fetch People Data from Applied Epic
Execute the script using the following command in your terminal or command prompt:
python get_applied_epic_people.py
If successful, you should see a list of people with their IDs and names printed in the console. This confirms that the API call was successful and the data was retrieved correctly.
Handling Errors and Verifying API Call Success
It's crucial to handle potential errors when making API calls. The Applied Epic API may return various error codes, such as:
- 400 Bad Request: The request was invalid. Check your parameters and try again.
- 401 Unauthorized: Authentication failed. Verify your OAuth token.
- 403 Forbidden: You do not have permission to access this resource.
Always check the response status code and handle errors appropriately to ensure robust integration.
Conclusion and Best Practices for Applied Epic API Integration
Integrating with the Applied Epic API can significantly enhance your ability to manage client data efficiently. By following the steps outlined in this guide, you can successfully retrieve people data using Python, streamlining your customer relationship management processes.
Best Practices for Secure and Efficient API Usage
- Securely Store Credentials: Always store your OAuth tokens and client secrets securely. Consider using environment variables or a secure vault to manage sensitive information.
- Handle Rate Limiting: Be mindful of any rate limits imposed by the API. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Data Standardization: Ensure that the data retrieved from the API is standardized and transformed as needed to fit your application's requirements.
Streamlining Integrations with Endgrate
If managing multiple integrations is becoming a challenge, consider using Endgrate to simplify the process. Endgrate allows you to build once for each use case and connect to various platforms through a single API endpoint. This can save you time and resources, allowing you to focus on your core product development.
Explore how Endgrate can enhance your integration experience by visiting Endgrate's website and discover the benefits of a unified API solution.
Read More
Ready to get started?