Using the Sap Business One API to Create or Update Invoices in PHP
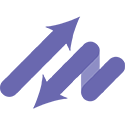
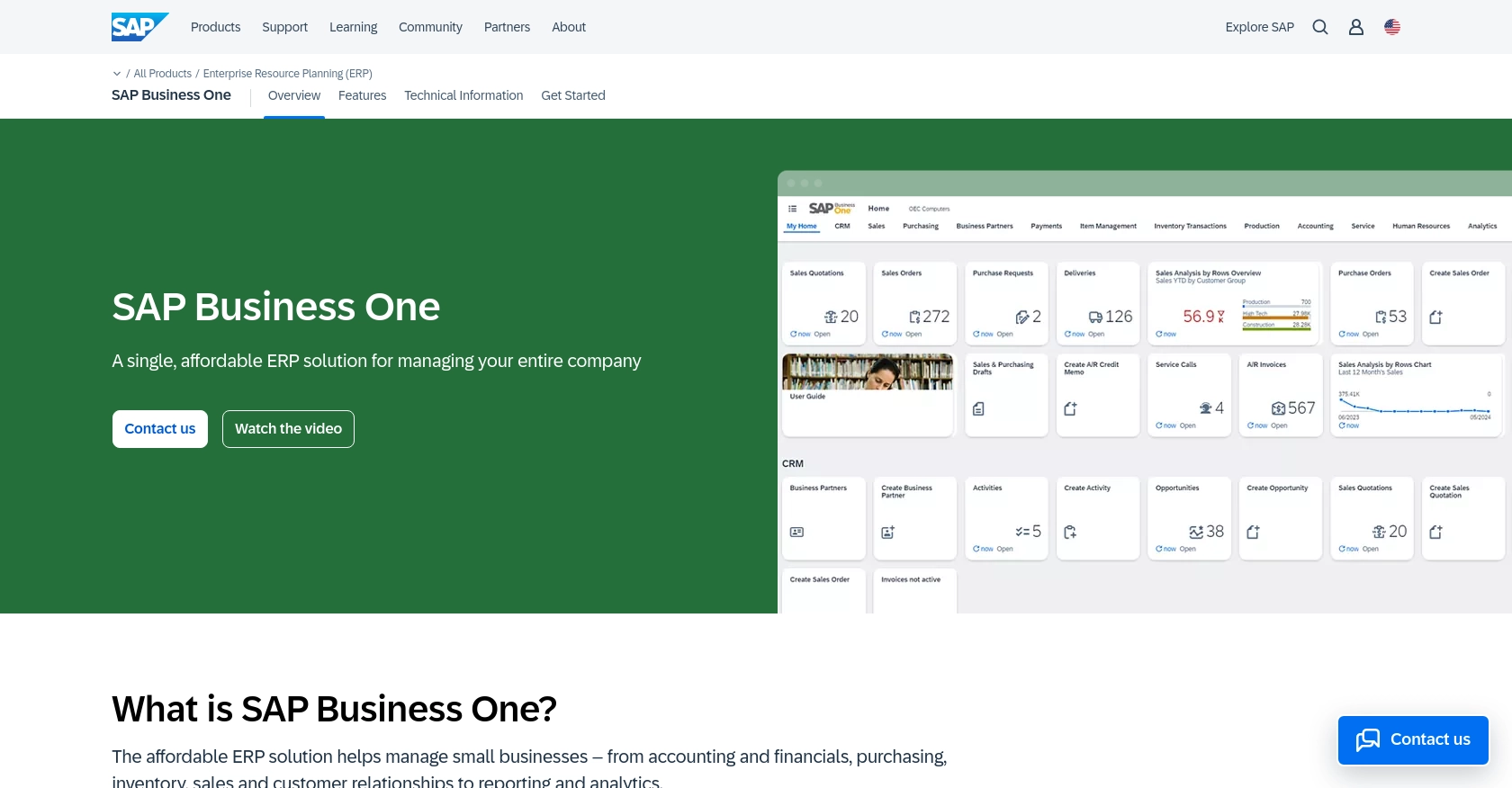
Introduction to SAP Business One API
SAP Business One is a comprehensive enterprise resource planning (ERP) solution designed for small to medium-sized businesses. It offers a wide range of functionalities, including financial management, sales, customer relationship management, and inventory control, all within a single platform.
Integrating with the SAP Business One API allows developers to automate and streamline business processes, such as creating or updating invoices. For example, a developer might use the API to automatically generate invoices from sales data collected in an external application, ensuring accurate and timely billing.
Setting Up a Test or Sandbox Account for SAP Business One API
Before you can start integrating with the SAP Business One API, you need to set up a test or sandbox account. This environment allows you to safely test your API calls without affecting live data. Follow these steps to get started:
Step 1: Accessing the SAP Business One Sandbox Environment
To begin, you need access to the SAP Business One sandbox environment. If you don't have an account, you can request access through the SAP Business One website or contact your SAP representative for assistance. This environment mimics the live system, providing a safe space for testing.
Step 2: Creating an SAP Business One App for API Access
Once you have access to the sandbox, the next step is to create an application that will allow you to interact with the SAP Business One API. Follow these instructions:
- Log in to your SAP Business One sandbox account.
- Navigate to the Administration section and select Integration Framework.
- Under Applications, click on Create New App.
- Fill in the required details, such as the app name and description.
Step 3: Generating API Credentials
After creating your app, you'll need to generate the necessary credentials to authenticate your API requests. SAP Business One uses a custom authentication method. Here's how to obtain your credentials:
- In your app settings, navigate to the API Credentials section.
- Generate a Client ID and Client Secret. Make sure to store these securely, as they will be used to authenticate your API calls.
Step 4: Configuring API Access Permissions
To ensure your app can interact with invoices, configure the necessary permissions:
- Go to the Permissions tab in your app settings.
- Select the required scopes for creating and updating invoices, such as Read and Write access to financial documents.
- Save your changes to apply the permissions.
With your sandbox account set up and your app configured, you're now ready to start making API calls to create or update invoices in SAP Business One using PHP.
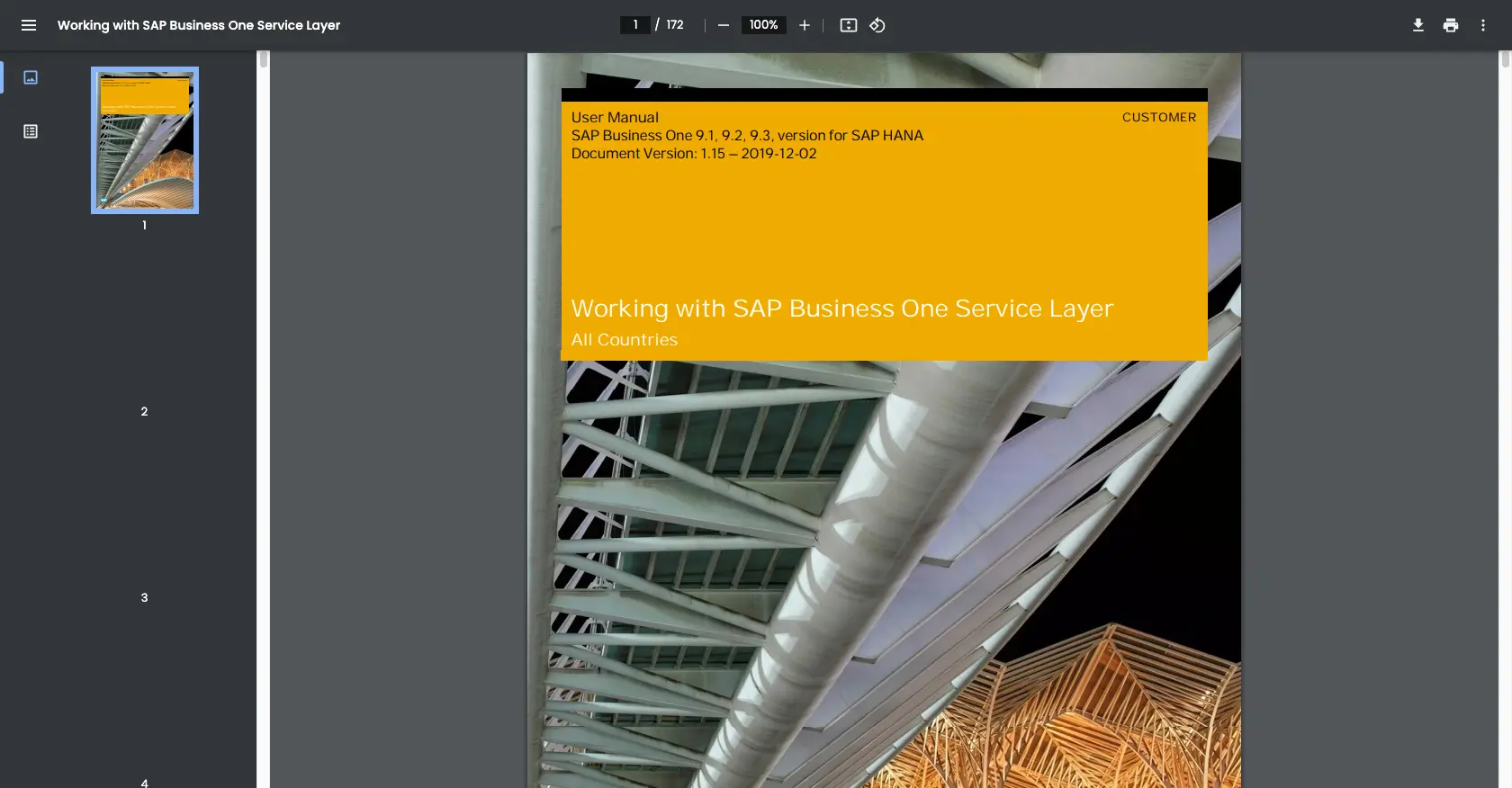
sbb-itb-96038d7
Making API Calls to Create or Update Invoices in SAP Business One Using PHP
To interact with the SAP Business One API for creating or updating invoices, you'll need to use PHP, a popular server-side scripting language. This section will guide you through setting up your PHP environment, making the necessary API calls, and handling responses effectively.
Setting Up Your PHP Environment for SAP Business One API Integration
Before making API calls, ensure your PHP environment is correctly configured. Follow these steps:
- Install PHP version 7.4 or higher on your server or local machine.
- Ensure the
cURL
extension is enabled in yourphp.ini
file, as it is essential for making HTTP requests. - Install Composer, a dependency manager for PHP, to manage any additional libraries you might need.
Installing Required PHP Libraries for SAP Business One API
To simplify API interactions, you can use libraries like Guzzle, a PHP HTTP client. Install it using Composer:
composer require guzzlehttp/guzzle
Creating or Updating Invoices with SAP Business One API in PHP
With your environment set up, you can now create or update invoices using the SAP Business One API. Below is a sample PHP script demonstrating how to make an API call:
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
// Set the API endpoint and headers
$endpoint = 'https://your-sap-business-one-instance.com/b1s/v1/Invoices';
$headers = [
'Content-Type' => 'application/json',
'Authorization' => 'Bearer Your_Access_Token'
];
// Define the invoice data
$invoiceData = [
'CardCode' => 'C20000',
'DocDate' => '2023-10-01',
'DocDueDate' => '2023-10-15',
'DocumentLines' => [
[
'ItemCode' => 'A00001',
'Quantity' => 10,
'Price' => 100
]
]
];
try {
// Make a POST request to create an invoice
$response = $client->post($endpoint, [
'headers' => $headers,
'json' => $invoiceData
]);
if ($response->getStatusCode() == 201) {
echo "Invoice created successfully.";
} else {
echo "Failed to create invoice.";
}
} catch (Exception $e) {
echo 'Error: ' . $e->getMessage();
}
Replace Your_Access_Token
with the token obtained from your SAP Business One app credentials. The script uses Guzzle to send a POST request to the SAP Business One API endpoint, creating an invoice with the specified data.
Verifying API Call Success in SAP Business One Sandbox
After executing the script, verify the success of your API call by checking the SAP Business One sandbox environment. Navigate to the invoices section to ensure the newly created or updated invoice appears as expected.
Handling Errors and Error Codes in SAP Business One API
When making API calls, it's crucial to handle potential errors. The SAP Business One API may return various error codes, such as 400 for bad requests or 401 for unauthorized access. Implement error handling in your script to manage these scenarios gracefully:
catch (GuzzleHttp\Exception\ClientException $e) {
$response = $e->getResponse();
$statusCode = $response->getStatusCode();
$errorBody = $response->getBody();
echo "Error $statusCode: $errorBody";
}
This code snippet captures client exceptions and outputs the error code and message, helping you diagnose and resolve issues efficiently.
Conclusion and Best Practices for SAP Business One API Integration
Integrating with the SAP Business One API using PHP allows developers to automate invoice management, enhancing business efficiency and accuracy. By following the steps outlined in this guide, you can effectively create or update invoices within the SAP Business One environment.
Best Practices for Secure and Efficient SAP Business One API Usage
- Securely Store API Credentials: Always store your Client ID and Client Secret securely, using environment variables or a secure vault, to prevent unauthorized access.
- Handle Rate Limiting: Be aware of any rate limits imposed by the SAP Business One API. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Standardize Data Fields: Ensure that data fields are standardized and validated before making API calls to prevent errors and maintain data integrity.
- Implement Robust Error Handling: Use comprehensive error handling to manage API response errors, ensuring that your application can recover gracefully from failures.
Streamlining Integrations with Endgrate
While integrating with SAP Business One can be highly beneficial, managing multiple integrations can be complex and time-consuming. Endgrate offers a unified API solution that simplifies the integration process, allowing you to focus on your core product development.
With Endgrate, you can build once for each use case and seamlessly connect to various platforms, including SAP Business One. This not only saves time and resources but also provides an intuitive integration experience for your customers.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate today.
Read More
Ready to get started?