Using the Brevo API to Create or Update Contacts in PHP
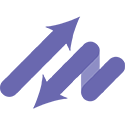
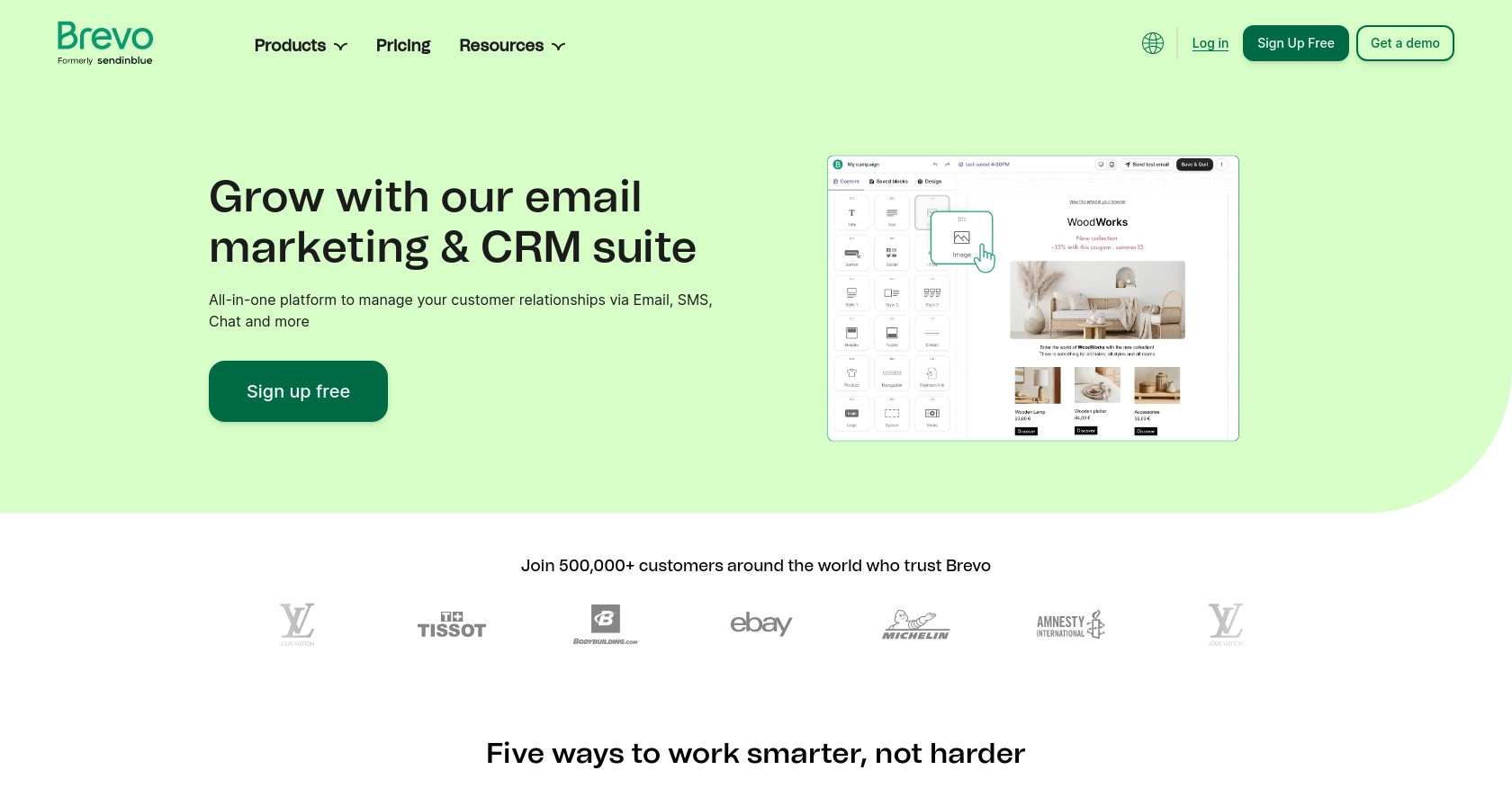
Introduction to Brevo API for Contact Management
Brevo is a powerful platform that offers a comprehensive suite of tools for managing customer relationships and communication. With its robust API, Brevo enables developers to seamlessly integrate contact management functionalities into their applications, enhancing the ability to create, update, and manage contacts efficiently.
Connecting with Brevo's API can significantly streamline your contact management processes. For example, developers can automate the creation or updating of contact information directly from their applications, ensuring that customer data is always up-to-date and accessible. This capability is particularly useful for businesses looking to maintain accurate customer records and improve their communication strategies.
In this article, we will explore how to use PHP to interact with the Brevo API, focusing on creating or updating contacts. By following this guide, developers can leverage Brevo's API to enhance their applications' contact management capabilities.
Setting Up Your Brevo Account for API Access
Before you can start using the Brevo API to manage contacts, you'll need to set up a Brevo account and obtain an API key. This key will allow you to authenticate your requests and interact with the Brevo platform securely.
Creating a Brevo Account
If you don't already have a Brevo account, follow these steps to create one:
- Visit the Brevo signup page.
- Fill out the registration form with your details and submit it.
- Check your email for a confirmation message and follow the instructions to verify your account.
Generating an API Key in Brevo
Once your account is set up, you can generate an API key to authenticate your API requests:
- Log in to your Brevo account.
- Click on your profile name in the top-right corner and select SMTP & API from the dropdown menu.
- Navigate to the API keys tab.
- Click on Generate a new API key.
- Enter a name for your API key to help you identify it later.
- Click Generate and copy the API key. Store it securely as you will need it for API requests.
For more details on setting up your API key, refer to the Brevo API documentation.
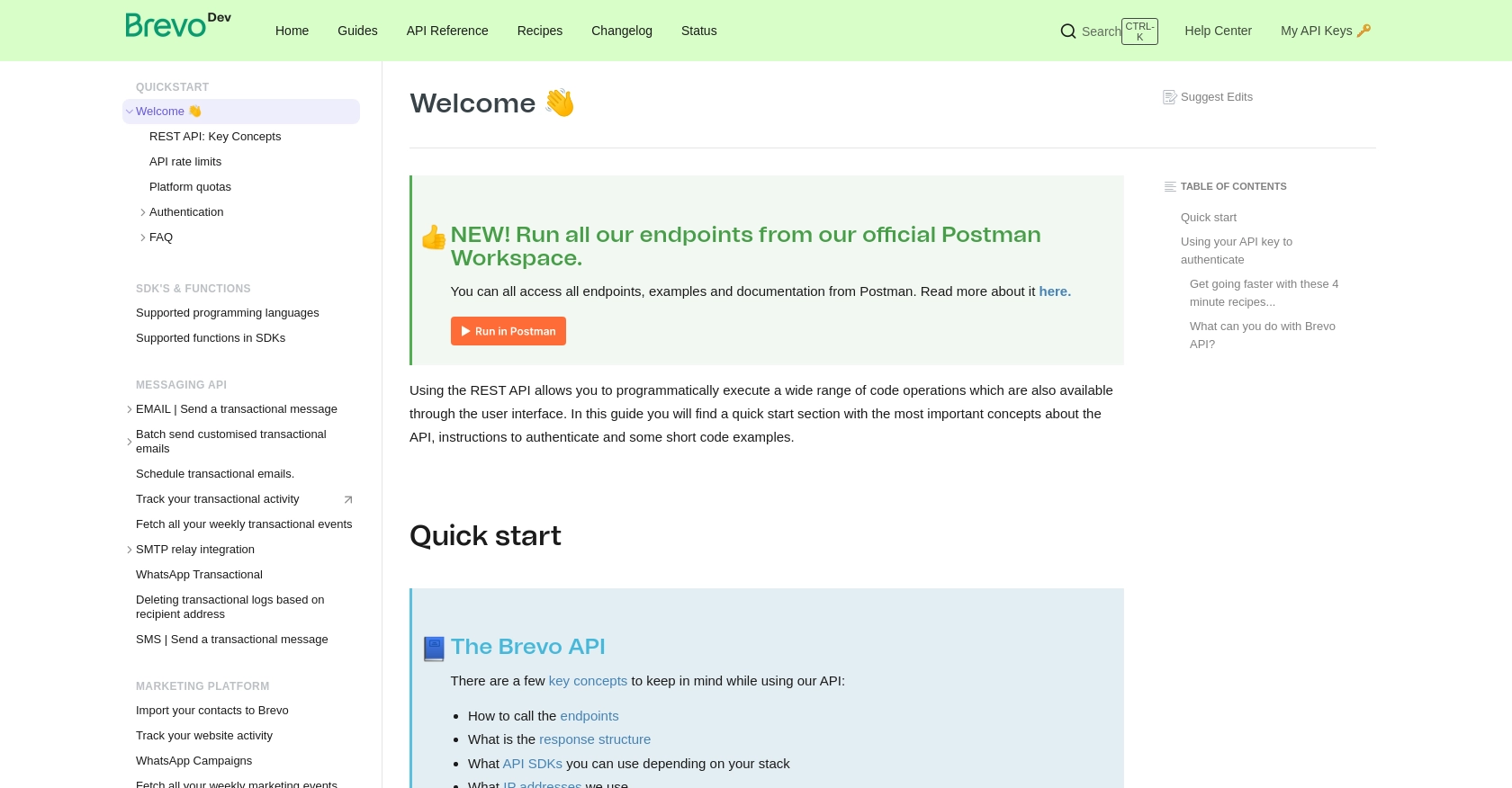
sbb-itb-96038d7
Making API Calls to Brevo for Contact Management Using PHP
To interact with the Brevo API for creating or updating contacts, you'll need to write PHP code that sends HTTP requests to the Brevo API endpoints. This section will guide you through the process of setting up your PHP environment, making the API call, and handling the response effectively.
Setting Up Your PHP Environment for Brevo API Integration
Before making API calls, ensure your PHP environment is correctly configured. You will need:
- PHP 7.4 or later
- Composer for dependency management
- The Guzzle HTTP client library
Install Guzzle using Composer with the following command:
composer require guzzlehttp/guzzle
Creating or Updating Contacts with Brevo API in PHP
Once your environment is set up, you can proceed to write the PHP code to create or update contacts in Brevo. Below is a sample script demonstrating how to achieve this:
require_once(__DIR__ . '/vendor/autoload.php');
use GuzzleHttp\Client;
use SendinBlue\Client\Configuration;
use SendinBlue\Client\Api\ContactsApi;
use SendinBlue\Client\Model\CreateContact;
// Configure API key authorization
$config = Configuration::getDefaultConfiguration()->setApiKey('api-key', 'YOUR_API_KEY');
// Create an instance of the Contacts API
$apiInstance = new ContactsApi(new Client(), $config);
// Define the contact details
$createContact = new CreateContact();
$createContact['email'] = 'example@example.com';
$createContact['listIds'] = [1];
try {
// Make the API call to create or update a contact
$result = $apiInstance->createContact($createContact);
print_r($result);
} catch (Exception $e) {
echo 'Exception when calling ContactsApi->createContact: ', $e->getMessage(), PHP_EOL;
}
Replace YOUR_API_KEY
with the API key you generated from your Brevo account. This script uses the Guzzle HTTP client to send a request to the Brevo API, creating or updating a contact with the specified email and list ID.
Verifying Successful API Requests and Handling Errors
After executing the script, check the response to verify the success of the API call. A successful request will return the contact details. If an error occurs, the exception block will capture and display the error message.
To ensure robust error handling, consider implementing additional checks for specific HTTP status codes and error messages. This will help you diagnose issues more effectively and improve the reliability of your integration.
For more information on the API call structure and error handling, refer to the Brevo API documentation.
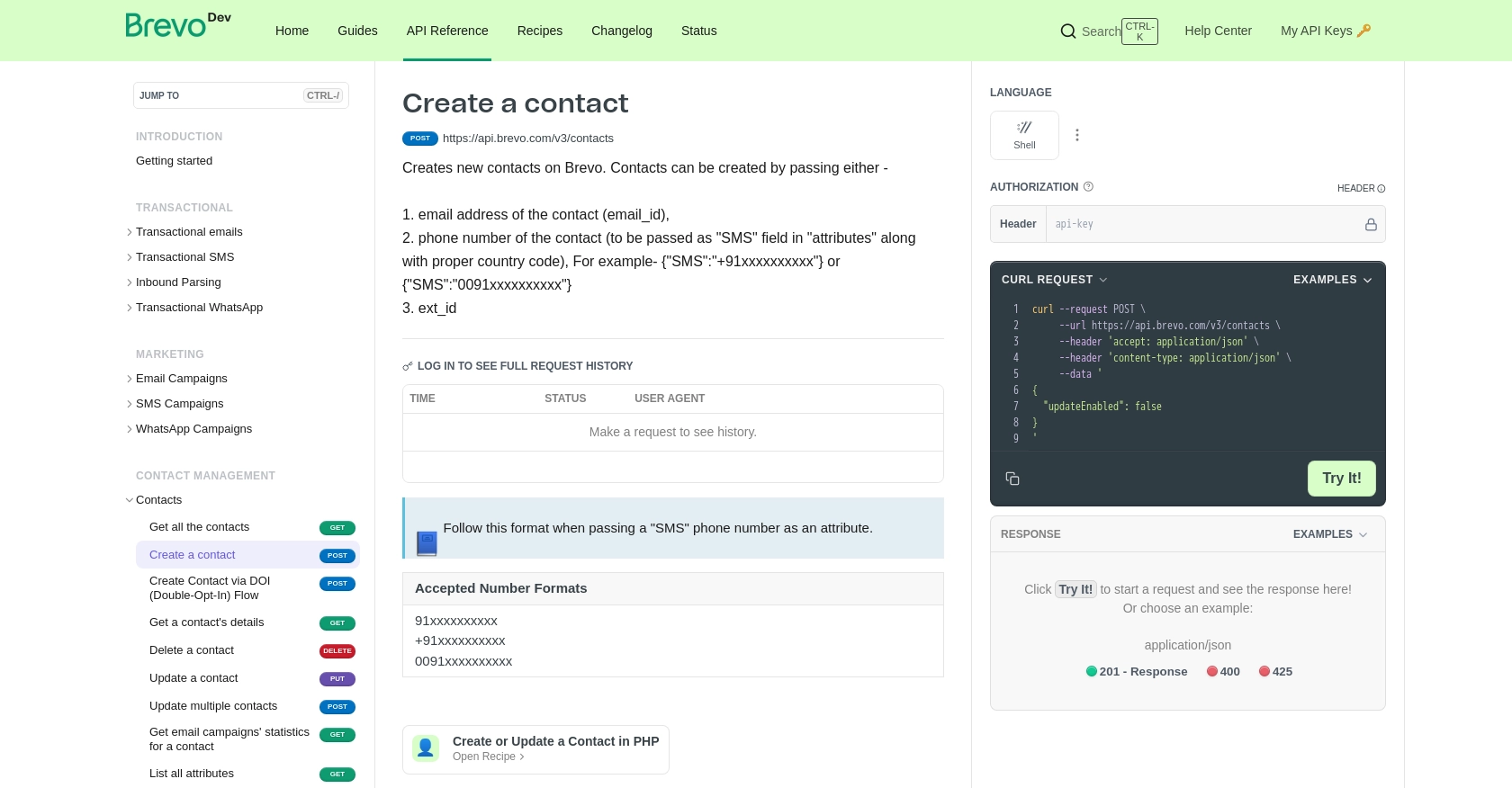
Conclusion: Best Practices for Using Brevo API in PHP
Integrating Brevo's API into your PHP applications can greatly enhance your contact management capabilities, allowing for seamless automation of creating and updating contacts. By following the steps outlined in this guide, you can ensure that your integration is both efficient and secure.
Best Practices for Secure API Integration with Brevo
- Secure Storage of API Keys: Always store your API keys securely, using environment variables or secure vaults, to prevent unauthorized access.
- Implement Error Handling: Use robust error handling to manage API call failures gracefully, ensuring your application can recover or notify users appropriately.
- Monitor API Usage: Regularly monitor your API usage to stay within Brevo's rate limits and avoid service disruptions. Refer to the Brevo documentation for details on rate limits.
Enhancing Your Application with Brevo API
By leveraging Brevo's API, you can automate contact management processes, improve data accuracy, and enhance communication strategies. This integration allows your application to maintain up-to-date customer records effortlessly.
Streamlining Integrations with Endgrate
If managing multiple integrations is becoming a challenge, consider using Endgrate to simplify your processes. Endgrate provides a unified API endpoint that connects to various platforms, including Brevo, allowing you to focus on your core product while outsourcing complex integrations.
Visit Endgrate to learn how you can save time and resources by streamlining your integration efforts.
Read More
Ready to get started?