Using the Chargebee API to Create or Update Payments in Javascript
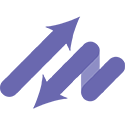
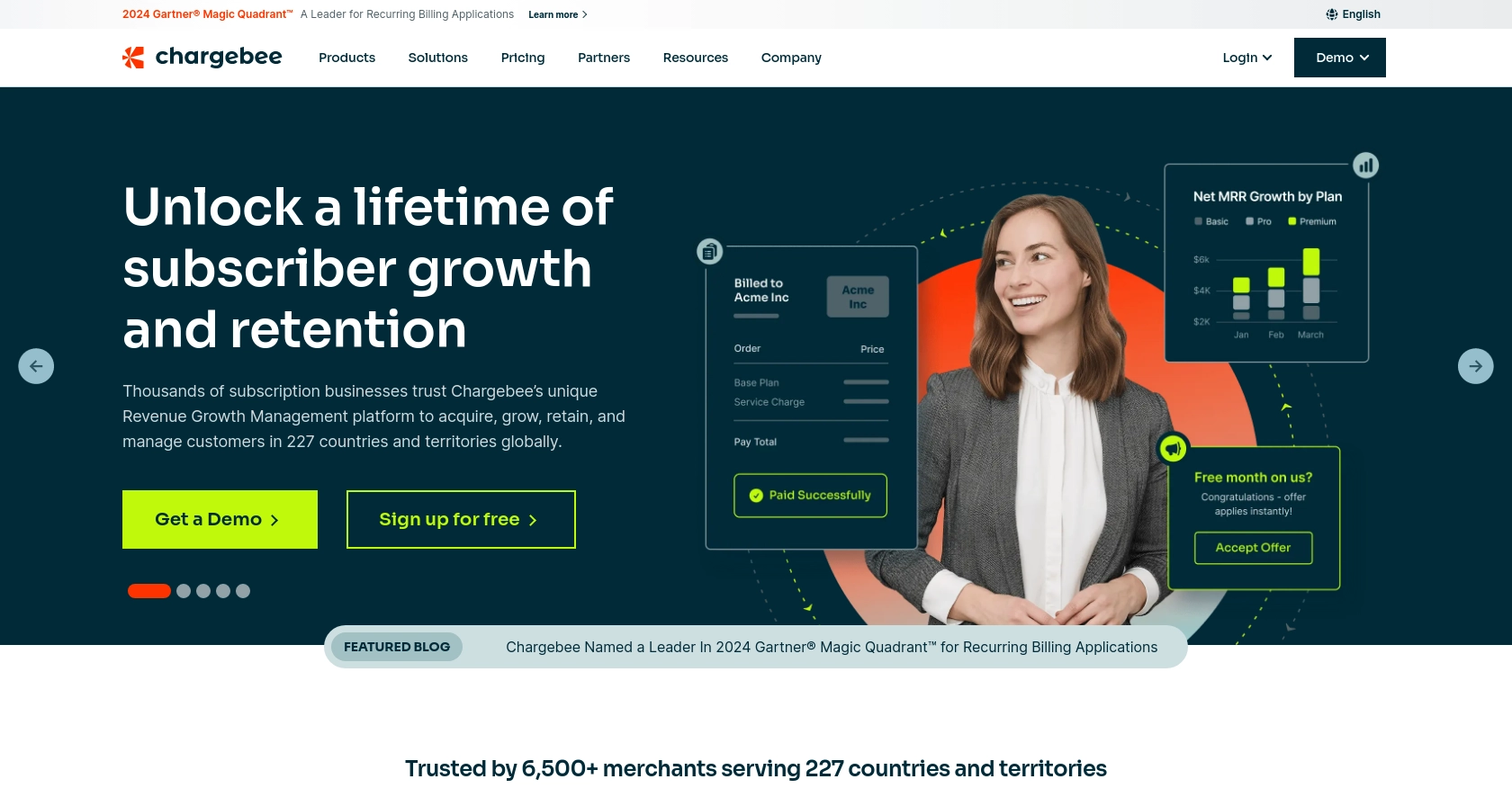
Introduction to Chargebee API for Payment Management
Chargebee is a robust subscription management platform that simplifies billing and revenue operations for businesses. It offers a comprehensive suite of tools to manage subscriptions, invoicing, and payments, making it a popular choice for SaaS companies looking to streamline their financial processes.
Integrating with Chargebee's API allows developers to automate payment workflows, such as creating or updating payments, directly from their applications. For example, a developer might use the Chargebee API to automate the process of capturing payments from customers, ensuring a seamless billing experience.
This article will guide you through using JavaScript to interact with the Chargebee API, focusing on creating or updating payments. You'll learn how to set up your environment, authenticate API requests, and handle transactions efficiently.
Setting Up Your Chargebee Test or Sandbox Account for API Integration
Before you can start integrating with the Chargebee API using JavaScript, you'll need to set up a Chargebee test or sandbox account. This environment allows you to safely test API calls without affecting live data, ensuring that your integration works seamlessly before going live.
Creating a Chargebee Test Account
If you don't already have a Chargebee account, you can sign up for a free trial on the Chargebee website. This trial account will serve as your sandbox environment for testing API interactions.
- Visit the Chargebee Signup Page.
- Fill in the required details to create your account.
- Once your account is set up, log in to access the Chargebee dashboard.
Generating API Keys for Chargebee Authentication
Chargebee uses HTTP Basic authentication for API calls, where your API key serves as the username. You can generate API keys from the Chargebee admin console.
- Navigate to the Settings section in the Chargebee dashboard.
- Select API Keys under the Configure Chargebee menu.
- Click on Create a Key to generate a new API key for your test environment.
- Copy the API key and store it securely, as it will be used for authenticating your API requests.
Configuring Chargebee API Authentication in JavaScript
With your API key ready, you can now configure your JavaScript application to authenticate API requests to Chargebee. Below is a sample code snippet demonstrating how to set up authentication:
// Import the necessary modules
const axios = require('axios');
// Set up the Chargebee API base URL
const chargebeeBaseUrl = 'https://{your-site}.chargebee.com/api/v2';
// Configure the API key for authentication
const apiKey = 'your_api_key_here';
// Example function to make an authenticated API call
async function getTransactions() {
try {
const response = await axios.get(`${chargebeeBaseUrl}/transactions`, {
auth: {
username: apiKey,
password: ''
}
});
console.log(response.data);
} catch (error) {
console.error('Error fetching transactions:', error);
}
}
// Call the function to test the setup
getTransactions();
Replace your_api_key_here
with the API key you generated earlier. This setup ensures that your API calls are authenticated and can interact with the Chargebee test environment.
For more detailed information on Chargebee authentication, refer to the Chargebee API Authentication Documentation.
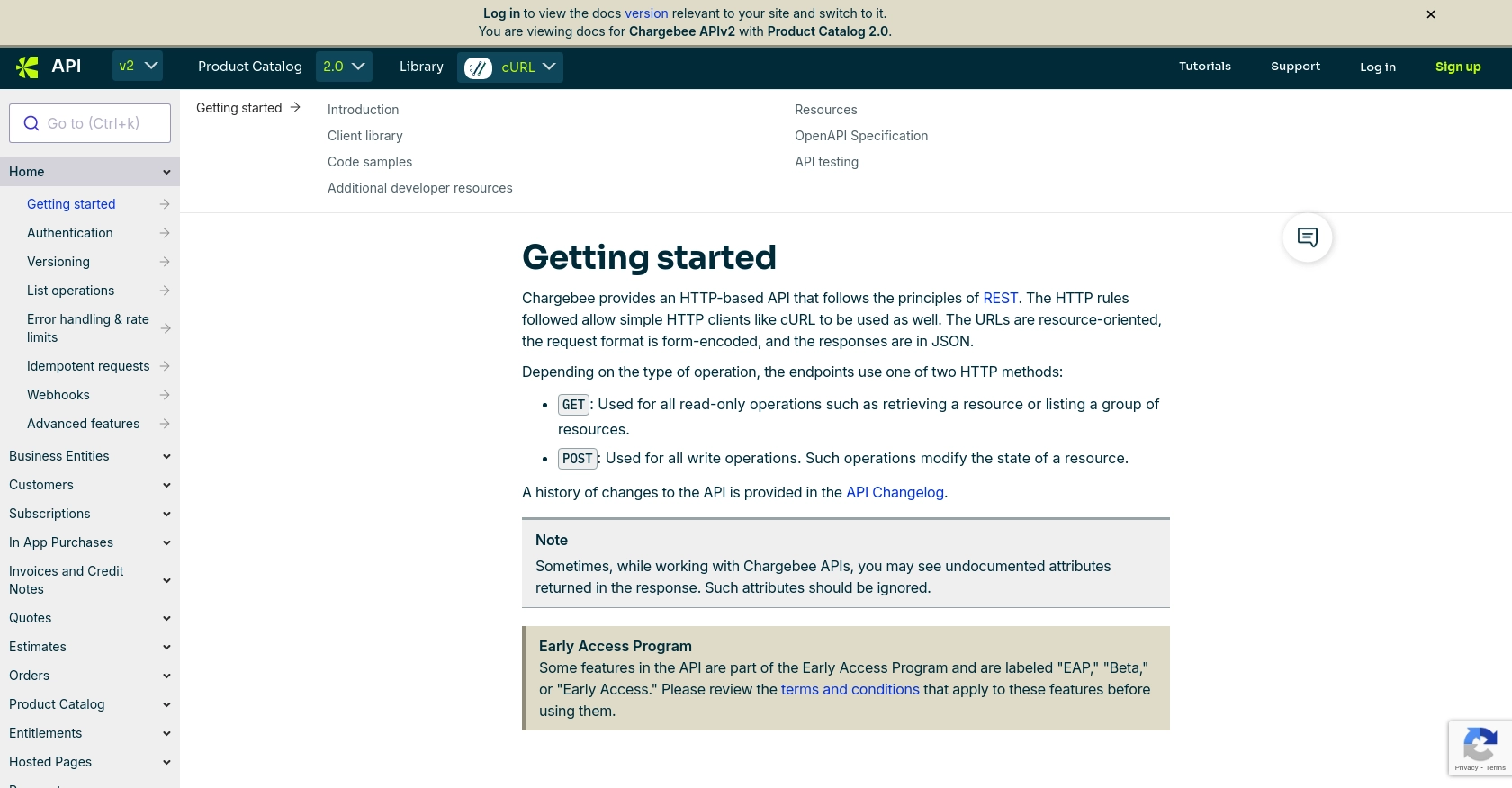
sbb-itb-96038d7
Making API Calls to Chargebee for Payment Transactions Using JavaScript
To interact with the Chargebee API for creating or updating payments, you'll need to make HTTP requests from your JavaScript application. This section will guide you through the process of setting up your environment, making API calls, and handling responses effectively.
Setting Up JavaScript Environment for Chargebee API Integration
Before making API calls, ensure your JavaScript environment is properly configured. You'll need Node.js and the Axios library to handle HTTP requests.
- Ensure Node.js is installed on your machine. You can download it from the official Node.js website.
- Install Axios by running the following command in your terminal:
npm install axios
Creating a Payment Transaction with Chargebee API
To create a payment transaction, you'll need to send a POST request to the Chargebee API endpoint. Below is a sample code snippet demonstrating how to create a payment transaction:
// Import Axios
const axios = require('axios');
// Set up the Chargebee API base URL
const chargebeeBaseUrl = 'https://{your-site}.chargebee.com/api/v2';
// Configure the API key for authentication
const apiKey = 'your_api_key_here';
// Function to create a payment transaction
async function createPaymentTransaction(customerId, amount) {
try {
const response = await axios.post(`${chargebeeBaseUrl}/transactions/create_authorization`, {
customer_id: customerId,
amount: amount
}, {
auth: {
username: apiKey,
password: ''
}
});
console.log('Transaction created successfully:', response.data);
} catch (error) {
console.error('Error creating transaction:', error.response.data);
}
}
// Call the function with sample data
createPaymentTransaction('__test__KyVnHhSBWltgF2p6', 1000);
Replace your_api_key_here
with your actual API key and {your-site}
with your Chargebee site identifier. This code will create a payment transaction for the specified customer ID and amount.
Handling API Responses and Errors
After making an API call, it's crucial to handle the response and any potential errors. The Chargebee API returns responses in JSON format, and you should check the status code to determine if the request was successful.
- If the request is successful, the status code will be in the 2XX range, and you can process the response data accordingly.
- If an error occurs, the status code will be in the 4XX or 5XX range. You should log the error details for troubleshooting.
For more information on error handling, refer to the Chargebee API Error Handling Documentation.
Verifying Transactions in Chargebee Dashboard
Once you've created a transaction, you can verify it by checking the Chargebee dashboard. Navigate to the Transactions section to view the details of the transaction you just created.
This verification step ensures that your API integration is functioning correctly and that transactions are being recorded as expected.
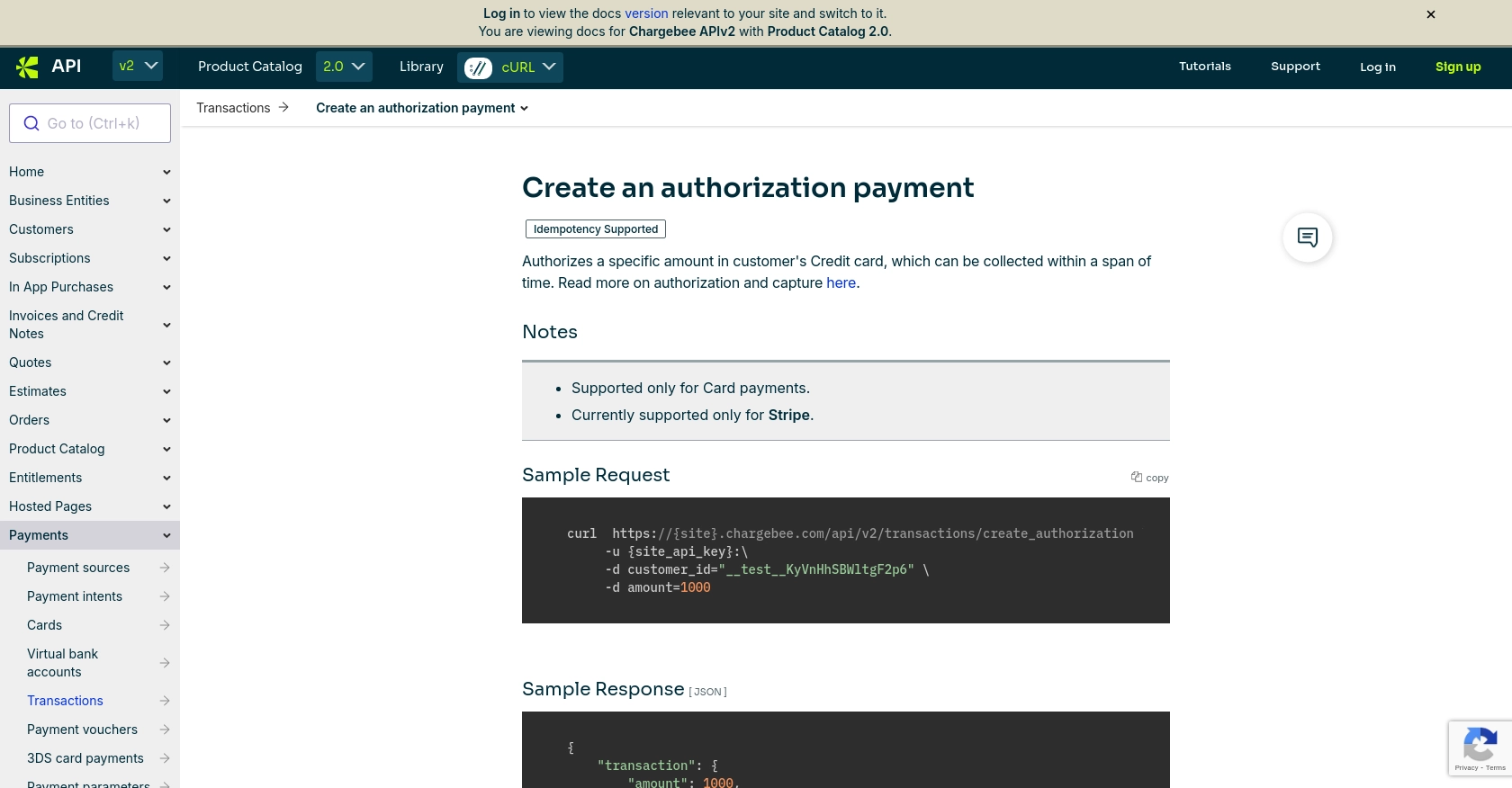
Best Practices for Chargebee API Integration in JavaScript
When integrating with the Chargebee API, it's essential to follow best practices to ensure a secure and efficient implementation. Here are some recommendations:
- Secure API Keys: Store your API keys securely and avoid hardcoding them in your application. Use environment variables or secure vaults to manage sensitive information.
- Handle Rate Limiting: Chargebee imposes rate limits on API requests. For test sites, the limit is approximately 750 API calls every 5 minutes, and for live sites, it's 150 calls per minute. Implement exponential backoff and retry mechanisms to handle HTTP 429 errors gracefully. For more details, refer to the Chargebee API Rate Limits Documentation.
- Data Standardization: Ensure that data fields are standardized and consistent across your application to avoid discrepancies during API interactions.
- Error Handling: Implement robust error handling by checking HTTP status codes and parsing error messages. This will help in diagnosing issues quickly and improving user experience.
Streamlining Integration with Endgrate
Building and maintaining multiple integrations can be time-consuming and complex. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Chargebee. By using Endgrate, you can:
- Save Time and Resources: Focus on your core product while Endgrate handles the integration complexities.
- Build Once, Use Everywhere: Develop a single integration for each use case, reducing redundancy and maintenance efforts.
- Enhance User Experience: Offer your customers a seamless and intuitive integration experience.
Explore how Endgrate can streamline your integration efforts by visiting Endgrate.
Read More
Ready to get started?