Using the Endear API to Get Customers in Javascript
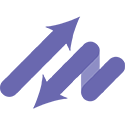
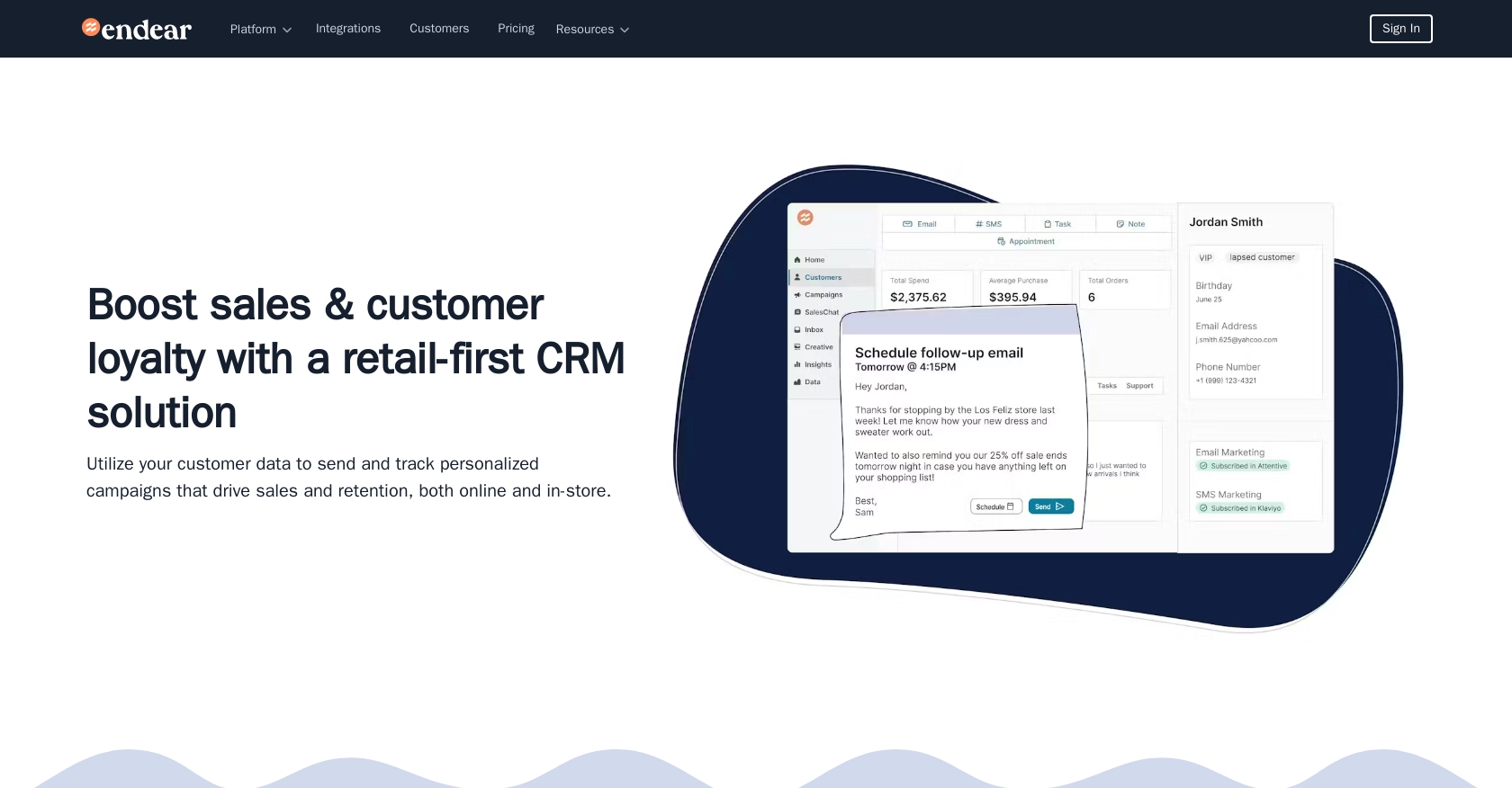
Introduction to Endear API Integration
Endear is a powerful CRM platform designed to enhance customer relationships and streamline communication for retail businesses. By providing a centralized hub for customer data, Endear enables businesses to personalize interactions and improve customer engagement.
Developers may want to integrate with Endear's API to access and manage customer data efficiently. For example, using the Endear API, a developer can retrieve customer information to tailor marketing campaigns or enhance customer service experiences.
This article will guide you through using JavaScript to interact with the Endear API, specifically focusing on retrieving customer data. By following this tutorial, you'll learn how to set up your environment, authenticate requests, and handle API responses effectively.
Setting Up Your Endear Test Account for API Integration
Before you can start interacting with the Endear API using JavaScript, you'll need to set up a test account and generate an API key. This key will allow you to authenticate your requests and access customer data securely.
Creating an Endear Integration and Generating an API Key
- Log in to your Endear account. If you don't have one, sign up for a free trial on the Endear website.
- Navigate to the Settings section in your Endear dashboard.
- Click on Integrations and then select Add Integration.
- Choose API from the options provided.
- Fill in the required details to create your integration. Once completed, you will receive an API key.
Ensure you store this API key securely, as it will be used to authenticate your API requests.
Authenticating API Requests with Endear
With your API key in hand, you can now authenticate your requests to the Endear API. All API requests should be sent to the following endpoint:
https://api.endearhq.com/graphql
Include the API key in the request headers as shown below:
{
"Content-Type": "application/json",
"X-Endear-Api-Key": "YOUR_API_KEY"
}
Replace YOUR_API_KEY
with the API key you generated earlier. This header is crucial for authenticating your requests and accessing the API.
For more detailed information on authentication, refer to the Endear Authentication Documentation.
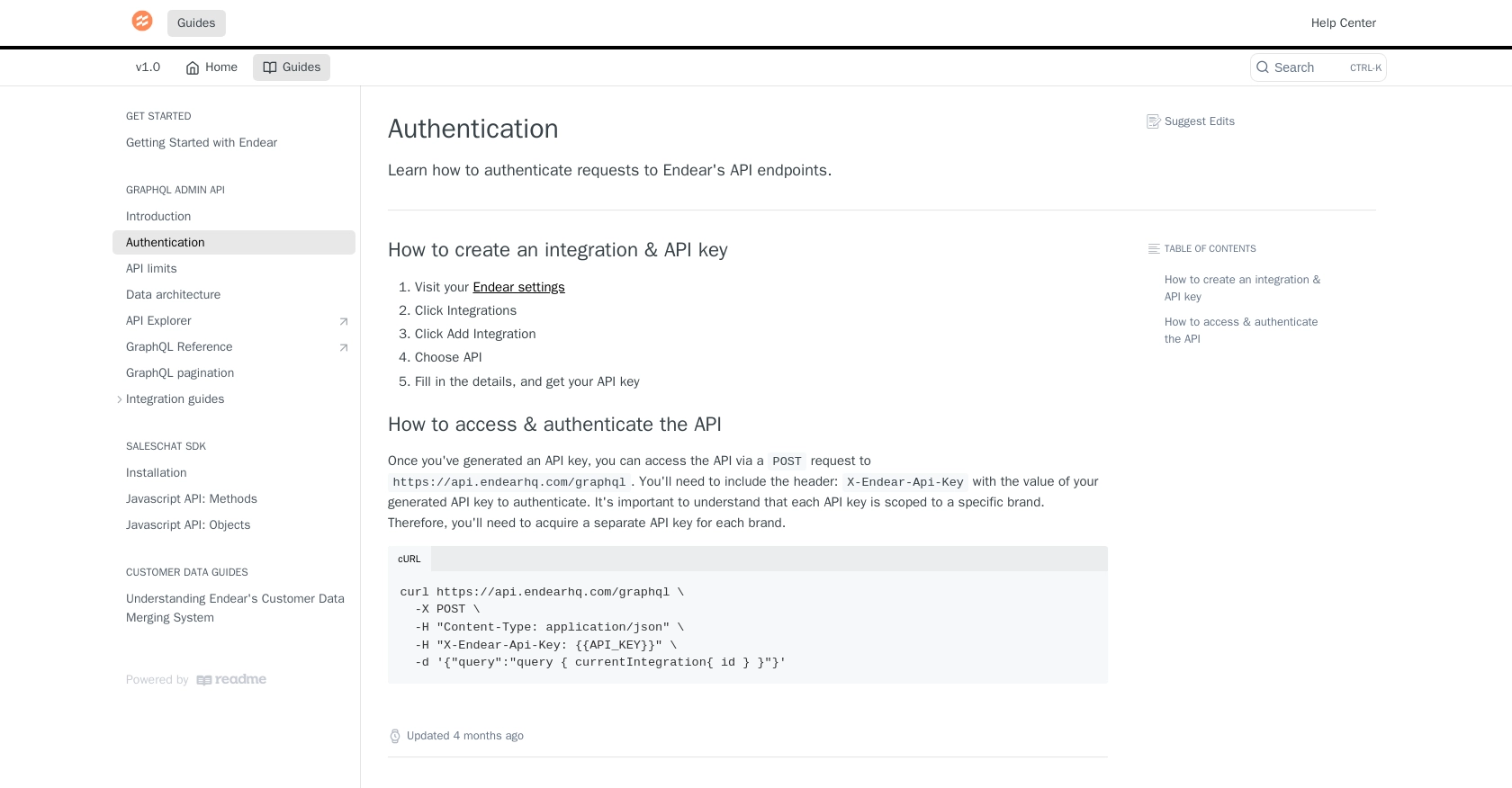
sbb-itb-96038d7
Making API Calls to Retrieve Customer Data from Endear Using JavaScript
To interact with the Endear API and retrieve customer data, you'll need to set up your JavaScript environment and make a POST request to the API endpoint. This section will guide you through the necessary steps, including setting up your environment, writing the code, and handling potential errors.
Setting Up Your JavaScript Environment for Endear API Integration
Before making API calls, ensure you have Node.js installed on your machine. You can download it from the official Node.js website. Once installed, you can use npm (Node Package Manager) to manage your dependencies.
To make HTTP requests, you'll need the axios
library. Install it using the following command:
npm install axios
Writing JavaScript Code to Retrieve Customers from Endear API
Create a new JavaScript file, for example, getCustomers.js
, and add the following code:
const axios = require('axios');
// Define the API endpoint and headers
const endpoint = 'https://api.endearhq.com/graphql';
const headers = {
'Content-Type': 'application/json',
'X-Endear-Api-Key': 'YOUR_API_KEY'
};
// Define the GraphQL query
const query = `
query {
searchCustomers {
edges {
node {
default_address
default_city
default_country
default_email_address
default_phone_number
default_postal_code
default_province
birthday
first_name
last_name
full_name
id
}
}
pageInfo {
endCursor
hasNextPage
}
}
}
`;
// Make the POST request to the API
axios.post(endpoint, { query }, { headers })
.then(response => {
console.log('Customer Data:', response.data.data.searchCustomers.edges);
})
.catch(error => {
console.error('Error fetching customer data:', error);
});
Replace YOUR_API_KEY
with the API key you generated earlier. This script sends a POST request to the Endear API endpoint, retrieves customer data, and logs it to the console.
Verifying Successful API Requests and Handling Errors
After running the script, you should see the customer data printed in the console. If the request is successful, verify the data by checking your Endear test account to ensure it matches the retrieved information.
If you encounter errors, the Endear API will return specific error codes. For example, an HTTP 429 error indicates that you've exceeded the rate limit of 120 requests per minute. For more information on error codes, refer to the Endear Rate Limits and Errors Documentation.
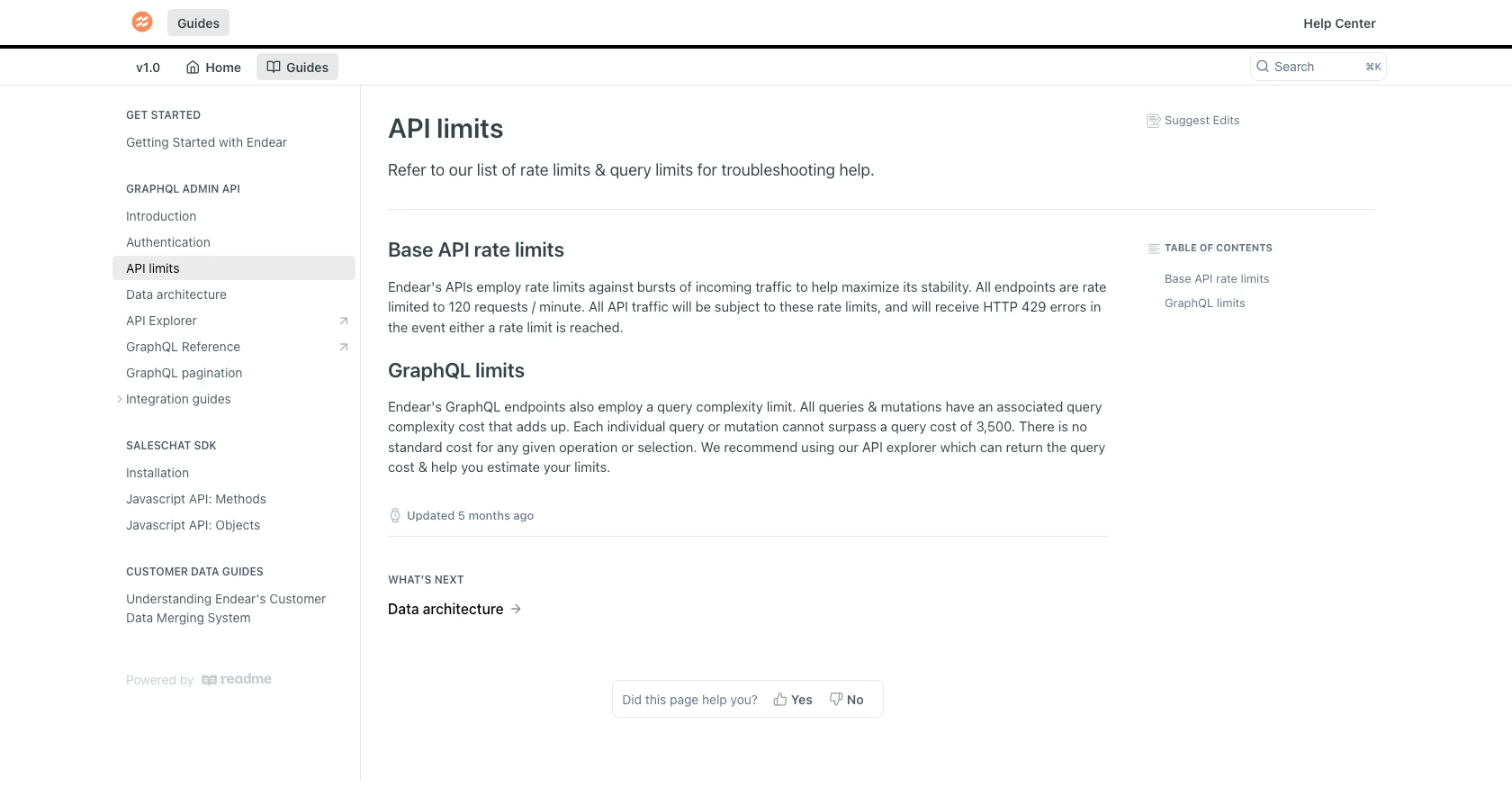
Conclusion and Best Practices for Using Endear API with JavaScript
Integrating with the Endear API using JavaScript allows developers to efficiently manage and retrieve customer data, enhancing the ability to personalize customer interactions and improve service delivery. By following the steps outlined in this guide, you can set up your environment, authenticate requests, and handle API responses effectively.
Best Practices for Secure and Efficient Endear API Integration
- Secure Storage of API Keys: Always store your API keys securely, using environment variables or a secure vault, to prevent unauthorized access.
- Handle Rate Limits: Be mindful of the rate limit of 120 requests per minute. Implement retry logic with exponential backoff to handle HTTP 429 errors gracefully. For more details, refer to the Endear Rate Limits Documentation.
- Data Standardization: Ensure that customer data retrieved from the API is standardized and transformed as needed to maintain consistency across your systems.
Enhancing Integration Capabilities with Endgrate
While integrating with Endear's API can be straightforward, managing multiple integrations across different platforms can become complex and time-consuming. This is where Endgrate can be a valuable asset.
Endgrate simplifies the integration process by providing a unified API endpoint that connects to multiple platforms, including Endear. This allows developers to build once for each use case and leverage a seamless integration experience, saving time and resources.
Explore how Endgrate can streamline your integration efforts by visiting Endgrate and discover how you can focus on your core product while outsourcing integration complexities.
Read More
- https://endgrate.com/provider/endear
- https://docs.endearhq.com/docs/authentication
- https://docs.endearhq.com/docs/rate-limits-status-codes-and-errors
- https://docs.endearhq.com/docs/disclaimers
- https://developers.endearhq.com/docs/graphql/objects/Customer
- https://docs.endearhq.com/docs/send-your-first-record
Ready to get started?