Using the Intercom API to Create or Update Contacts in Javascript
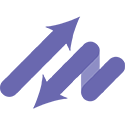
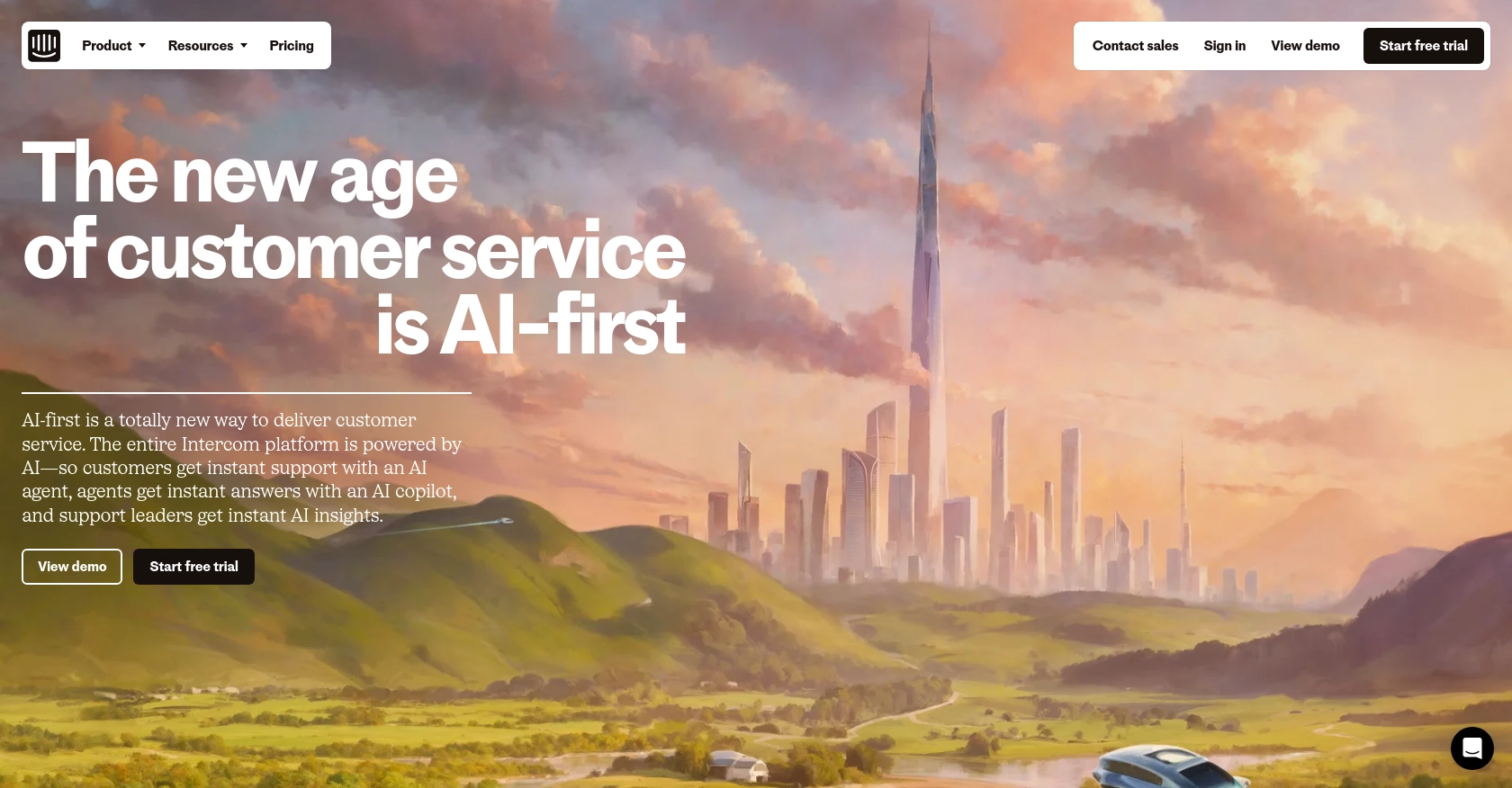
Introduction to Intercom API for Contact Management
Intercom is a powerful customer communication platform that enables businesses to engage with their customers through personalized messaging and support. It offers a suite of tools for managing customer interactions, including live chat, email marketing, and customer data management.
Developers often integrate with the Intercom API to streamline customer relationship management by automating tasks such as creating or updating contact information. For example, a developer might use the Intercom API to automatically update customer details in response to changes in a CRM system, ensuring that all customer data remains consistent and up-to-date across platforms.
This article will guide you through using JavaScript to interact with the Intercom API, focusing on creating and updating contacts. By following this tutorial, you'll learn how to efficiently manage customer data within the Intercom platform, enhancing your application's integration capabilities.
Setting Up Your Intercom Test or Sandbox Account
Before you can start interacting with the Intercom API using JavaScript, you'll need to set up a test or sandbox account. This will allow you to safely experiment with API calls without affecting live data.
Create an Intercom Developer Account
If you don't already have an Intercom account, you can sign up for a free developer account. This account will give you access to the Developer Hub, where you can manage your apps and integrations.
- Visit the Intercom Developer Portal and sign up for a new account.
- Once registered, log in to access your Developer Hub.
Set Up a Development Workspace
Intercom requires a development workspace to build and test integrations. Follow these steps to set up your workspace:
- In the Developer Hub, navigate to the "Workspaces" section.
- Create a new workspace by following the on-screen instructions.
- This workspace will be used for testing and development purposes.
Configure OAuth for Intercom API Access
Since the Intercom API uses OAuth for authentication, you'll need to configure it to obtain access tokens. Here's how:
- In your Developer Hub, go to the "Authentication" section.
- Enable the "Use OAuth" option.
- Provide the necessary redirect URLs, ensuring they use HTTPS.
- Select the permissions your application needs, such as reading and writing contacts.
For detailed instructions, refer to the Intercom OAuth Setup Guide.
Generate Client ID and Client Secret
After setting up OAuth, you'll receive a client ID and client secret. These credentials are essential for authorizing API requests:
- Find your client ID and client secret in the "Basic Information" section of your app in the Developer Hub.
- Store these credentials securely, as they will be used in your API calls.
Testing Your Intercom API Integration
With your sandbox account and OAuth configured, you're ready to test your Intercom API integration. Use the client ID and secret to authenticate and start making API calls to create or update contacts.
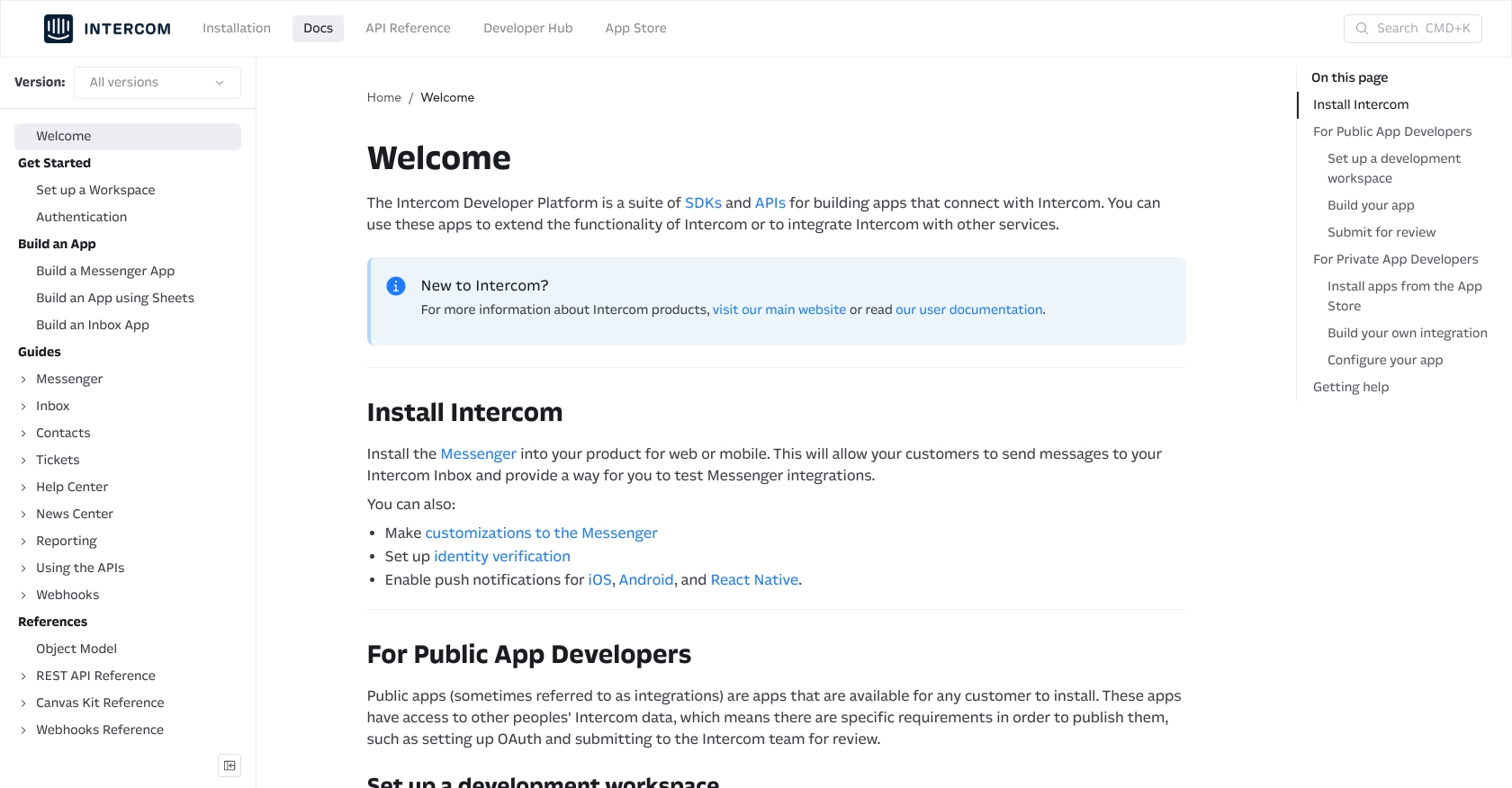
sbb-itb-96038d7
Making API Calls to Intercom for Contact Management Using JavaScript
To interact with the Intercom API for creating or updating contacts, you'll need to use JavaScript. This section will guide you through the necessary steps, including setting up your environment and writing the code to make API calls.
Setting Up Your JavaScript Environment
Before you begin, ensure you have Node.js installed on your machine. Node.js provides the runtime environment needed to execute JavaScript code outside of a browser.
- Download and install Node.js from the official website.
- Verify the installation by running
node -v
in your terminal to check the version.
Installing Required Dependencies
You'll need the axios
library to make HTTP requests to the Intercom API. Install it using npm:
npm install axios
Writing JavaScript Code to Create or Update Contacts in Intercom
With your environment set up, you can now write the JavaScript code to interact with the Intercom API. Below is an example of how to create or update a contact:
const axios = require('axios');
// Replace with your actual access token
const accessToken = 'YOUR_ACCESS_TOKEN';
// Function to create or update a contact
async function createOrUpdateContact(email, name) {
try {
const response = await axios.post('https://api.intercom.io/contacts', {
email: email,
name: name
}, {
headers: {
'Authorization': `Bearer ${accessToken}`,
'Content-Type': 'application/json',
'Intercom-Version': '2.11'
}
});
console.log('Contact created or updated:', response.data);
} catch (error) {
console.error('Error creating or updating contact:', error.response ? error.response.data : error.message);
}
}
// Example usage
createOrUpdateContact('johndoe@example.com', 'John Doe');
Understanding the API Response and Error Handling
Upon a successful API call, the response will contain the contact details. You can verify the creation or update by checking the response data or logging into your Intercom sandbox account.
In case of errors, the code handles them by logging the error message. Common error codes include:
- 401 Unauthorized: Check your access token.
- 404 Not Found: Ensure the endpoint URL is correct.
- 400 Bad Request: Verify the request payload and headers.
For more detailed information on error codes, refer to the Intercom API documentation.
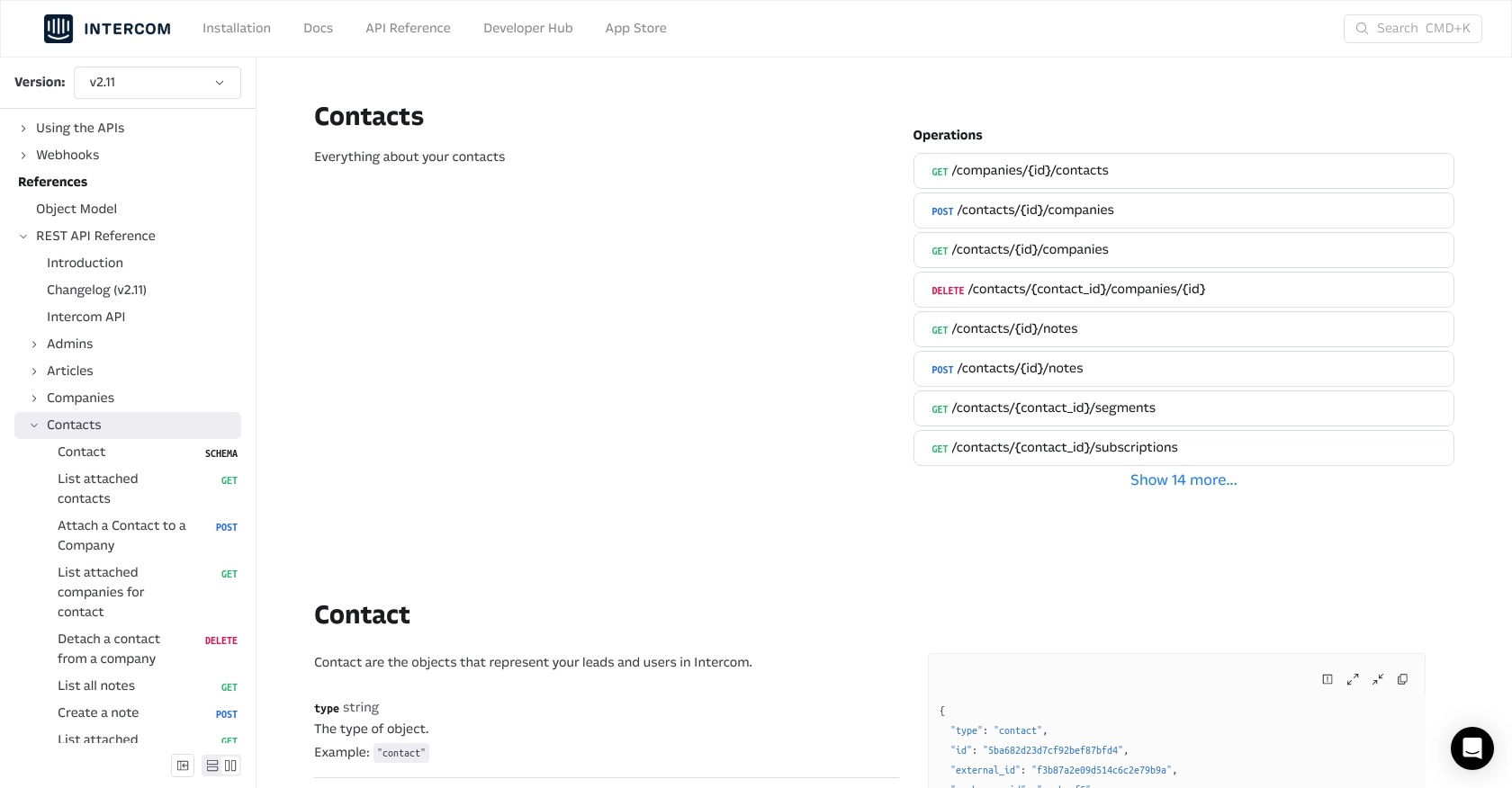
Conclusion: Best Practices for Intercom API Integration in JavaScript
Integrating with the Intercom API using JavaScript provides a powerful way to manage customer data efficiently. By automating the creation and updating of contacts, you can ensure that your customer information is always accurate and up-to-date.
Securely Storing Intercom API Credentials
It's crucial to store your Intercom API credentials, such as the client ID, client secret, and access token, securely. Avoid hardcoding them in your source code. Instead, use environment variables or a secure vault to manage these sensitive details.
Handling Intercom API Rate Limiting
Intercom imposes rate limits on API requests to ensure fair usage. Be mindful of these limits and implement retry logic with exponential backoff to handle rate limit errors gracefully. This approach helps maintain a smooth integration experience without exceeding the allowed request thresholds.
Transforming and Standardizing Data Fields
When integrating with Intercom, it's essential to standardize data fields to match your application's requirements. Transform data as needed to ensure consistency across platforms, which will enhance data integrity and usability.
Leverage Endgrate for Streamlined Integrations
Consider using Endgrate to simplify your integration processes. With Endgrate, you can save time and resources by outsourcing integrations, allowing you to focus on your core product. Build once for each use case and enjoy an intuitive integration experience for your customers.
Visit Endgrate to learn more about how it can enhance your integration capabilities.
Read More
Ready to get started?