Using the Notion API to Create Records in Python
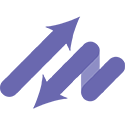
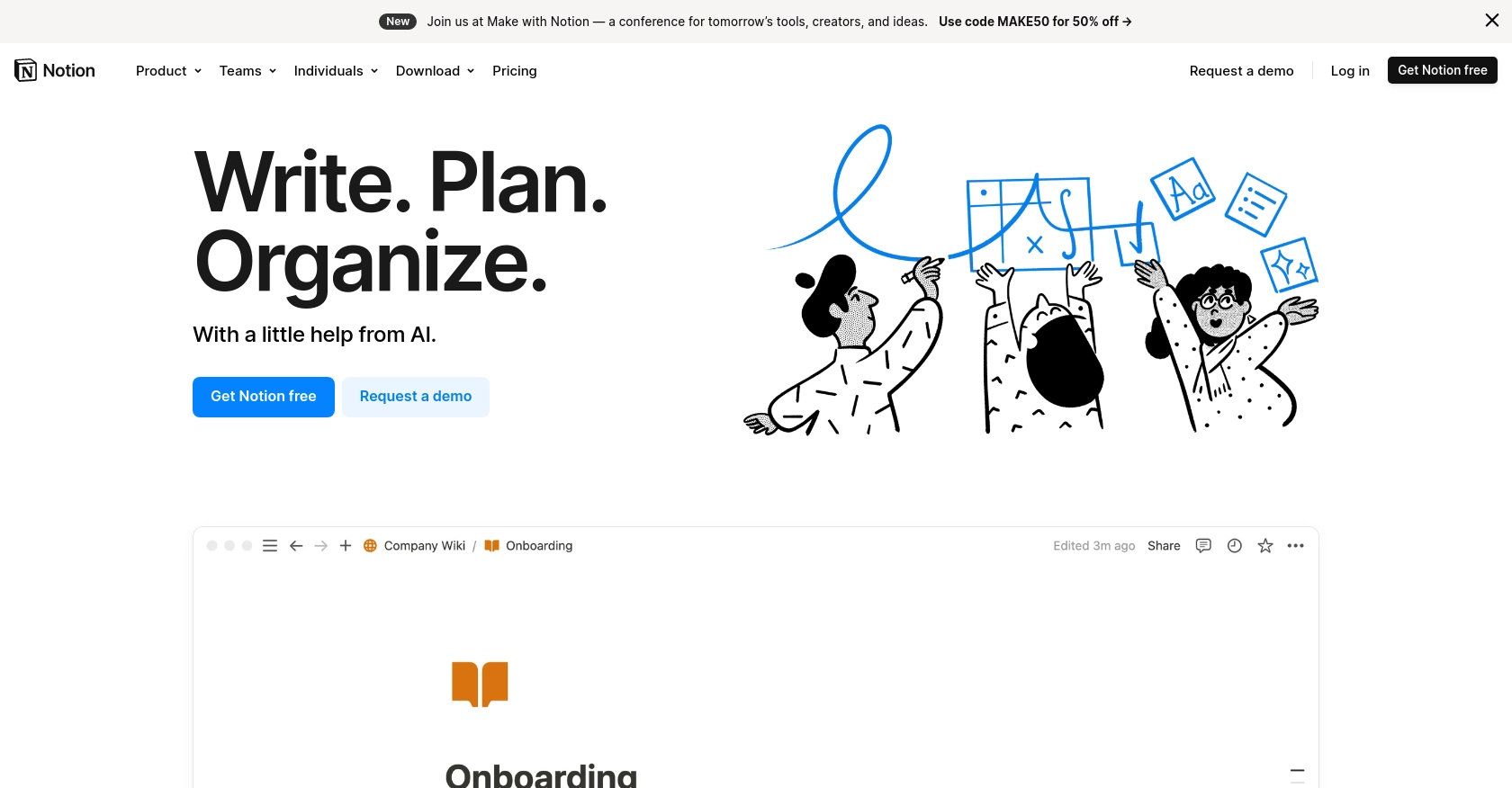
Introduction to Notion API for Creating Records
Notion is a versatile all-in-one workspace that allows users to manage notes, tasks, databases, and more in a highly customizable environment. Its flexibility and collaborative features make it a popular choice for teams and individuals looking to organize their work efficiently.
For developers, integrating with the Notion API opens up opportunities to automate and enhance workflows within Notion workspaces. By leveraging the API, developers can programmatically create, update, and manage records, enabling seamless data synchronization and automation across various applications.
In this article, we will explore how to use Python to interact with the Notion API, specifically focusing on creating records. This integration can be particularly useful for automating data entry tasks, such as adding new project tasks from an external system into a Notion database, thereby streamlining project management processes.
Setting Up a Notion Test or Sandbox Account for API Integration
Before diving into the Notion API, you'll need to set up a Notion account to test your integration. Notion offers a free version that is perfect for developers looking to experiment with API functionalities. Follow these steps to get started:
Create a Notion Account
- Visit the Notion website and sign up for a free account if you don't already have one.
- Once registered, you can create a personal workspace to test your integrations.
Set Up a Notion Integration
To interact with the Notion API, you'll need to create an integration within your Notion account. This involves generating an integration token that will allow your application to authenticate API requests.
- Navigate to the Settings & Members section in your Notion workspace.
- Go to the Integrations tab and click on Create New Integration.
- Fill in the required details such as the integration name and select the workspace you want to connect.
- Once created, you will receive an integration token. Keep this token secure as it will be used to authenticate your API requests.
Authorize the Integration
After creating the integration, you need to authorize it to access specific pages or databases within your workspace:
- Open the Notion page or database you want to share with the integration.
- Click on the Share button and add your integration by searching for its name.
- Ensure the integration has the necessary permissions to read or write data as required by your application.
OAuth Authentication for Notion API
The Notion API uses OAuth 2.0 for authentication. Here’s how to set it up:
- Direct users to the integration’s authorization URL to begin the OAuth flow.
- Once users authorize the integration, Notion will redirect them to your specified redirect URI with a temporary code.
- Exchange this code for an access token by making a POST request to the Notion API’s token endpoint.
- Store the access token securely for future API requests.
For more detailed instructions, refer to the Notion API Authorization Guide.
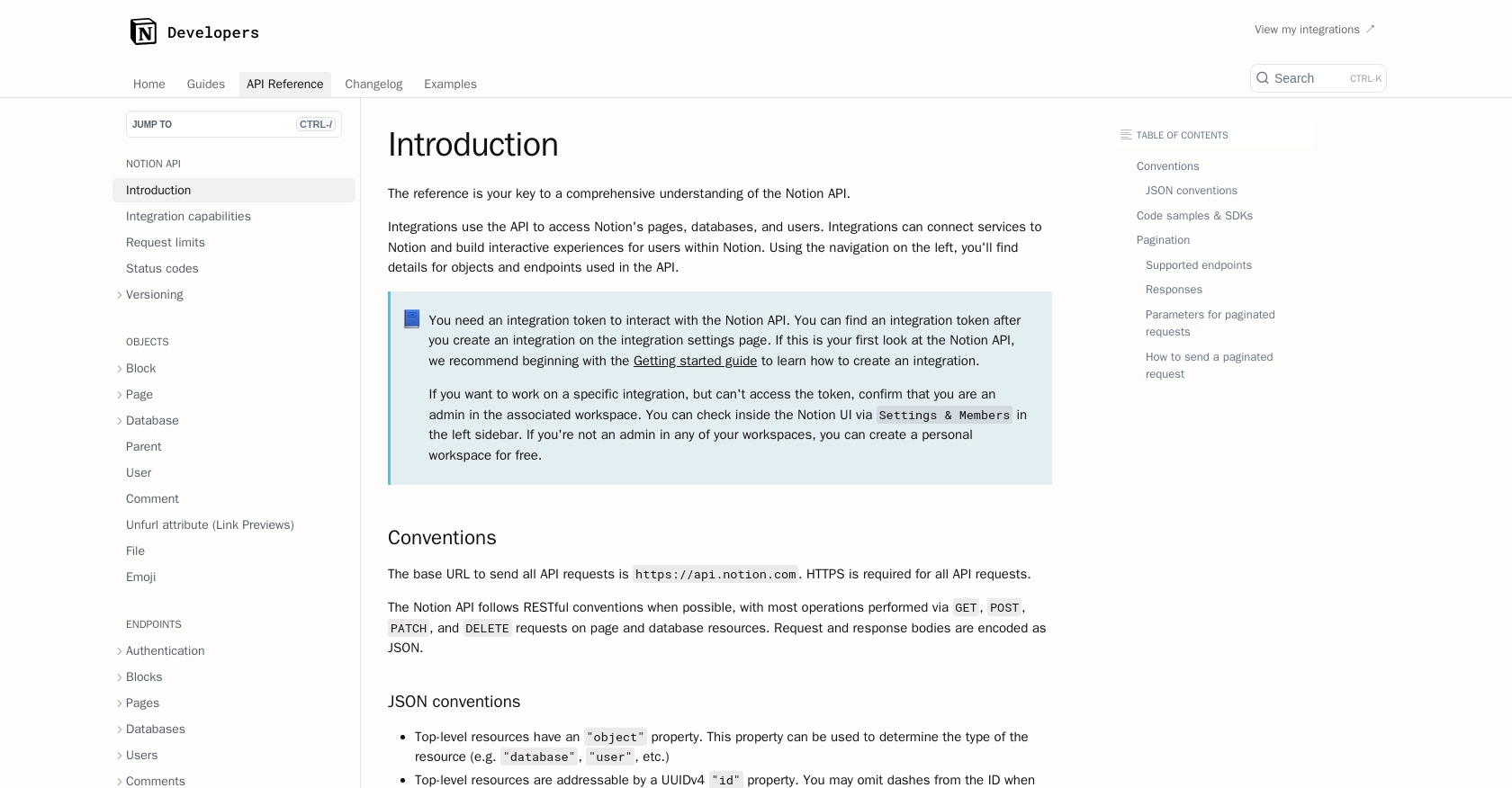
sbb-itb-96038d7
Making API Calls to Create Records in Notion Using Python
To interact with the Notion API and create records, you'll need to use Python, a versatile programming language known for its simplicity and readability. This section will guide you through the process of setting up your Python environment and making API calls to Notion.
Setting Up Python Environment for Notion API Integration
Before you begin, ensure you have Python installed on your machine. This tutorial uses Python 3.11.1. You will also need the requests
library to handle HTTP requests.
- Install Python from the official Python website if you haven't already.
- Open your terminal or command prompt and install the
requests
library using pip:
pip install requests
Creating a New Page in Notion Using Python
Now that your environment is set up, you can proceed to create a new page in Notion. Follow these steps to make the API call:
- Create a new Python file named
create_notion_page.py
and add the following code:
import requests
import json
# Set the API endpoint
url = "https://api.notion.com/v1/pages"
# Set the request headers
headers = {
"Authorization": "Bearer YOUR_ACCESS_TOKEN",
"Content-Type": "application/json",
"Notion-Version": "2022-06-28"
}
# Define the page properties
data = {
"parent": {"database_id": "YOUR_DATABASE_ID"},
"properties": {
"Name": {
"title": [
{
"text": {
"content": "New Task"
}
}
]
}
}
}
# Send the request
response = requests.post(url, headers=headers, data=json.dumps(data))
# Check the response
if response.status_code == 200:
print("Page created successfully:", response.json())
else:
print("Failed to create page:", response.status_code, response.json())
Replace YOUR_ACCESS_TOKEN
and YOUR_DATABASE_ID
with your actual Notion access token and database ID.
Verifying the API Request Success
After running the script, you should see a success message in the terminal if the page is created successfully. You can verify the creation by checking your Notion workspace for the new page.
Handling Errors and Understanding Notion API Response Codes
It's crucial to handle potential errors when making API requests. The Notion API may return various status codes indicating success or failure:
- 200: Request was successful.
- 400: Invalid request. Check your JSON structure.
- 401: Unauthorized. Verify your access token.
- 429: Rate limited. Slow down your requests.
For more details, refer to the Notion API Status Codes documentation.
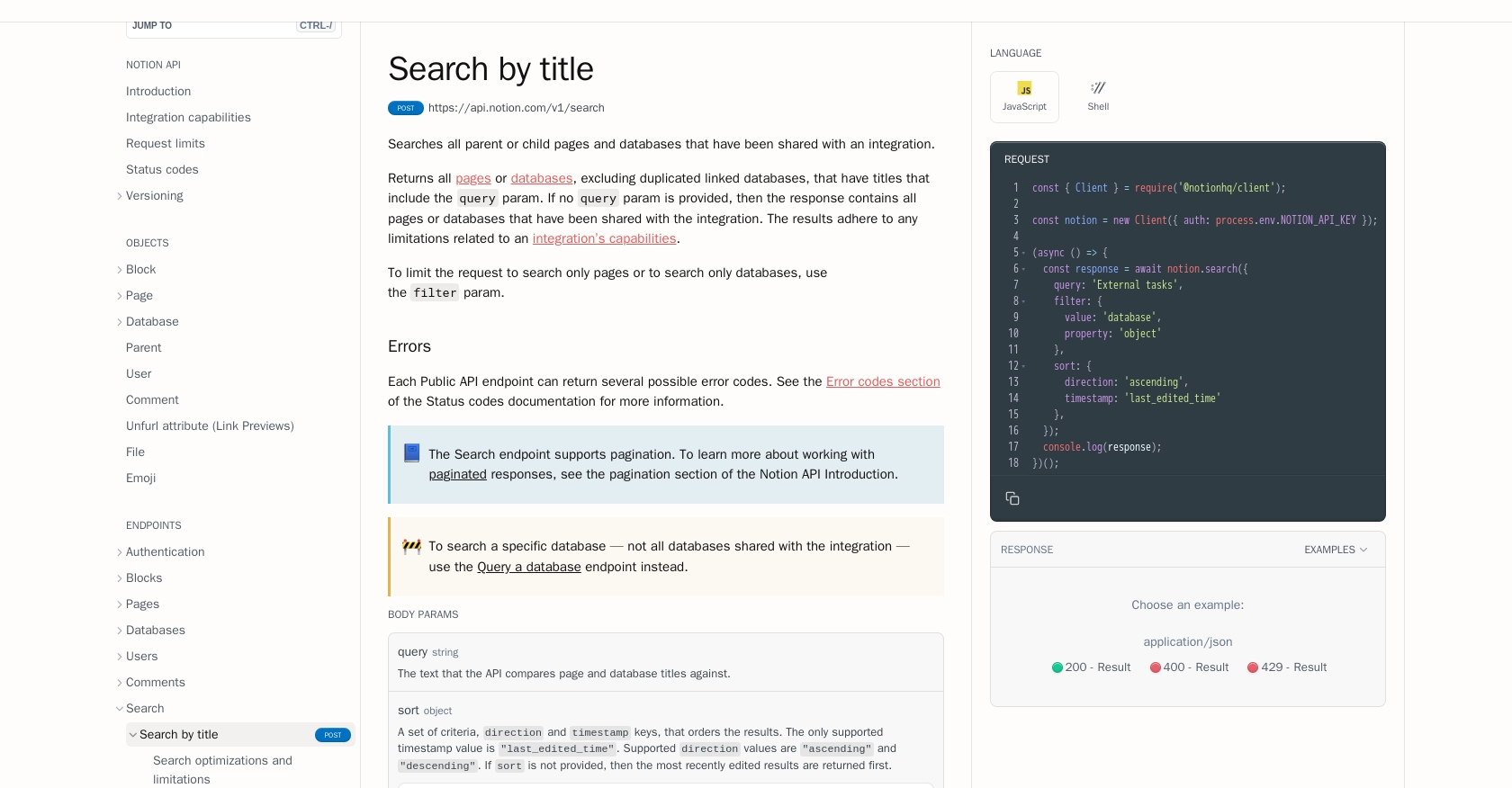
Conclusion and Best Practices for Using the Notion API in Python
Integrating with the Notion API using Python offers a powerful way to automate and enhance workflows within your Notion workspace. By following the steps outlined in this guide, you can efficiently create records and manage data, streamlining your project management and collaboration processes.
Best Practices for Secure and Efficient Notion API Integration
- Secure Storage of Access Tokens: Always store your access tokens securely, using environment variables or a secret manager. Never hard-code them into your source code.
- Handle Rate Limiting: The Notion API has a rate limit of three requests per second. Implement error handling for HTTP 429 responses and use the
Retry-After
header to manage request pacing. - Data Standardization: Ensure that data fields are standardized and consistent across your applications to avoid discrepancies and errors.
Enhance Your Integration Experience with Endgrate
While building integrations with the Notion API can be rewarding, it can also be time-consuming and complex. Endgrate simplifies this process by providing a unified API endpoint that connects to multiple platforms, including Notion. This allows you to focus on your core product while outsourcing integration efforts.
With Endgrate, you can build once for each use case and enjoy an intuitive integration experience for your customers. Visit Endgrate to learn more about how you can streamline your integration processes and enhance your product offerings.
Read More
- https://endgrate.com/provider/Notion
- https://developers.notion.com/reference/intro
- https://developers.notion.com/docs/getting-started
- https://developers.notion.com/docs/authorization
- https://developers.notion.com/reference/request-limits
- https://developers.notion.com/reference/status-codes
- https://developers.notion.com/reference/post-search
- https://developers.notion.com/reference/post-page
Ready to get started?