Using the Outlook API to Get Messages (with PHP examples)
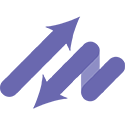
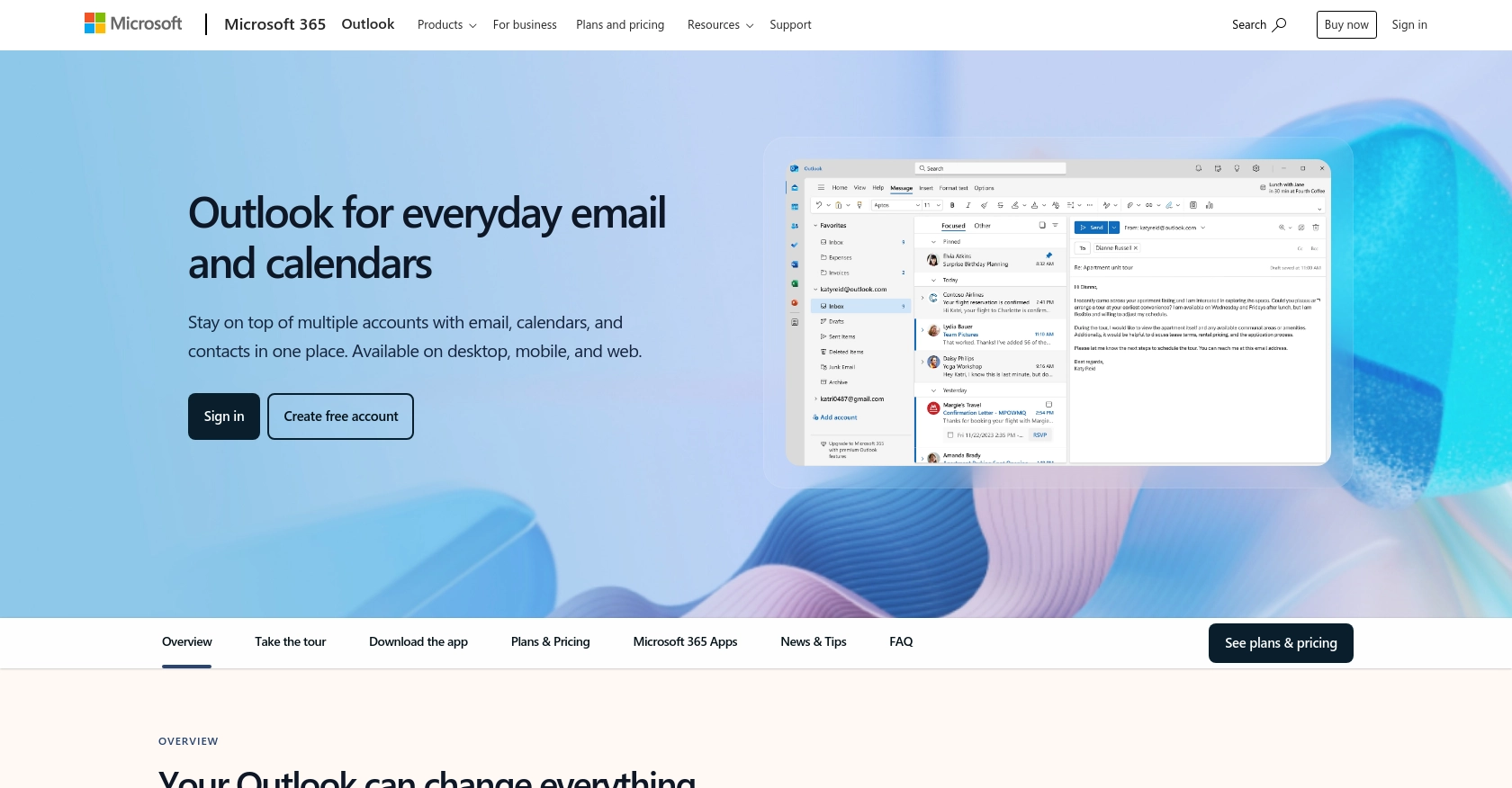
Introduction to the Outlook API
The Outlook API, part of the Microsoft Graph suite, provides developers with access to Outlook mail data, enabling seamless integration with Microsoft 365 services. This powerful API allows developers to interact with emails, calendars, and contacts, offering a unified approach to accessing Microsoft cloud data.
Connecting with the Outlook API is essential for developers looking to enhance productivity applications by integrating email functionalities. For example, a developer might use the Outlook API to retrieve messages and automate email sorting or create custom notifications based on specific email criteria.
In this article, we will explore how to use PHP to interact with the Outlook API, focusing on retrieving messages. This guide will provide step-by-step instructions, making it easier for developers to integrate Outlook's email capabilities into their applications.
Setting Up Your Outlook API Test Account
Before you can start using the Outlook API with PHP, you'll need to set up a test account. This involves creating a Microsoft Entra ID account and registering your application to obtain the necessary credentials for OAuth-based authentication.
Create a Microsoft Entra ID Account
If you don't already have a Microsoft Entra ID account, you can create one by signing up for a free Azure account. This will give you access to the Microsoft Entra admin center, where you can manage your applications and permissions.
- Visit the Azure free account page and sign up.
- Follow the on-screen instructions to complete the registration process.
Register Your Application in Microsoft Entra
Once you have your Microsoft Entra ID account, you need to register your application to interact with the Outlook API. This process will provide you with the client ID and client secret required for OAuth authentication.
- Sign in to the Microsoft Entra admin center.
- Navigate to Identity > Applications > App registrations and click on New registration.
- Enter a name for your application and select the appropriate account type.
- Click Register to complete the registration.
Configure Authentication Settings
After registering your application, configure the authentication settings to enable OAuth-based access.
- In the app registration overview, select Authentication under Manage.
- Click on Add a platform and select Web.
- Enter a redirect URI where your app will receive authentication responses. This URI must match one of the URIs registered in your app settings.
- Ensure that the Access tokens and ID tokens options are checked under Implicit grant and hybrid flows.
- Click Configure to save your settings.
Generate Client Secret
To authenticate your application, you'll need a client secret.
- Navigate to Certificates & secrets under Manage.
- Click on New client secret.
- Add a description and select an expiration period.
- Click Add and copy the client secret value. Store it securely as it will not be displayed again.
With your application registered and authentication configured, you're ready to start making API calls to the Outlook API using PHP. In the next section, we'll cover how to use these credentials to authenticate and retrieve messages from Outlook.
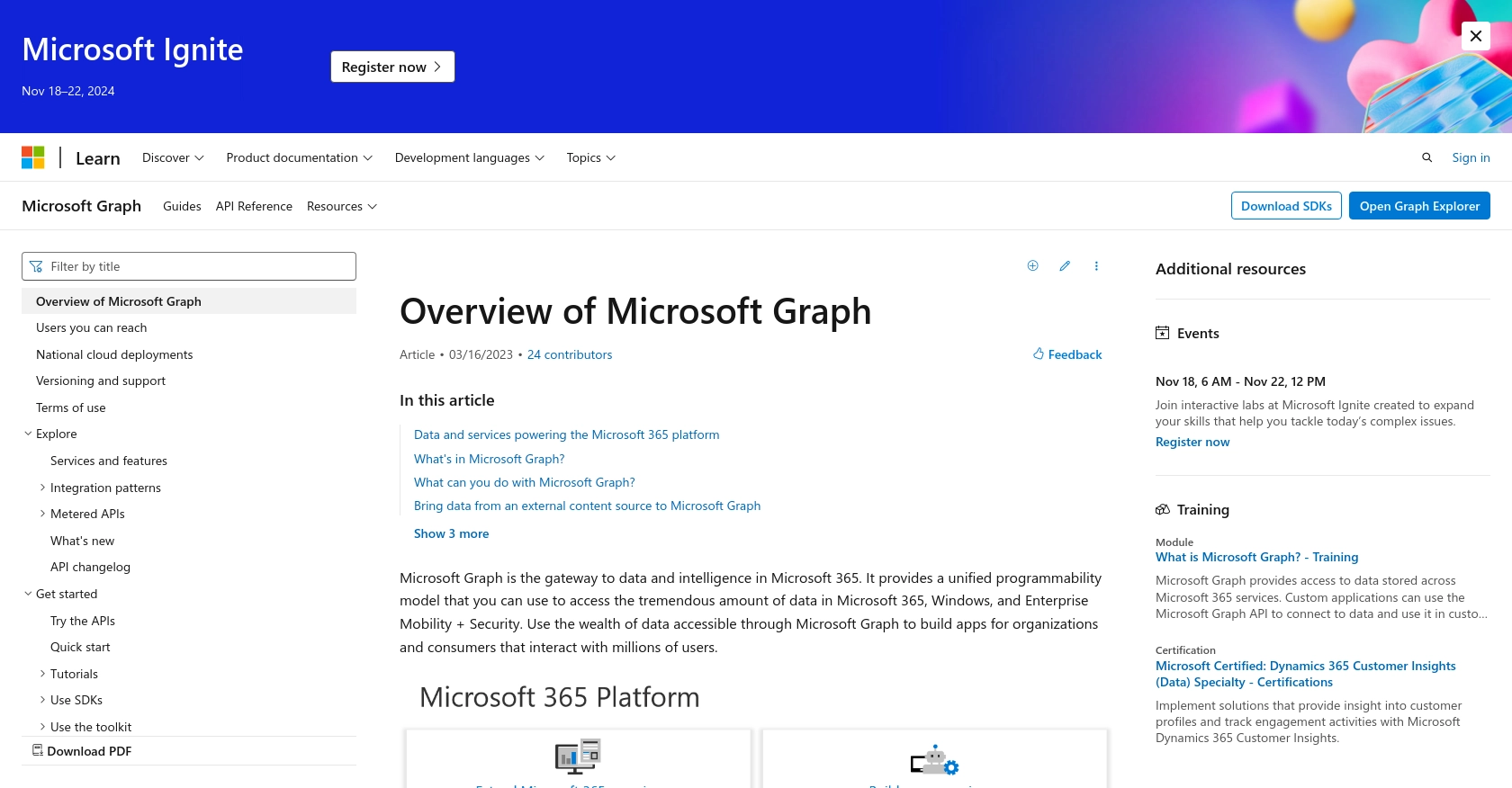
sbb-itb-96038d7
Making API Calls to Retrieve Outlook Messages Using PHP
To interact with the Outlook API and retrieve messages, you'll need to use PHP to make HTTP requests. This section will guide you through the process of setting up your PHP environment, installing necessary dependencies, and executing the API call to fetch messages from Outlook.
Setting Up Your PHP Environment for Outlook API Integration
Before making API calls, ensure your PHP environment is properly configured. You'll need PHP 7.4 or later and the Composer package manager to handle dependencies.
- Ensure PHP is installed on your machine. You can download it from the official PHP website.
- Install Composer by following the instructions on the Composer website.
Installing Required PHP Packages for Outlook API
To interact with the Outlook API, you'll need the Guzzle HTTP client, which simplifies making HTTP requests in PHP. Install it using Composer:
composer require guzzlehttp/guzzle
Executing the Outlook API Call to Retrieve Messages
With your environment set up, you can now write PHP code to make an API call to Outlook and retrieve messages. Below is a sample script demonstrating how to achieve this:
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$accessToken = 'YOUR_ACCESS_TOKEN'; // Replace with your actual access token
$response = $client->request('GET', 'https://graph.microsoft.com/v1.0/me/messages', [
'headers' => [
'Authorization' => 'Bearer ' . $accessToken,
'Accept' => 'application/json',
],
'query' => [
'$select' => 'sender,subject',
'$top' => 10
]
]);
$messages = json_decode($response->getBody(), true);
foreach ($messages['value'] as $message) {
echo "Subject: " . $message['subject'] . "\n";
echo "Sender: " . $message['sender']['emailAddress']['name'] . "\n\n";
}
Replace YOUR_ACCESS_TOKEN
with the access token obtained during the authentication process. This script uses the Guzzle client to send a GET request to the Outlook API, retrieving the top 10 messages with their sender and subject details.
Verifying Successful API Requests and Handling Errors
After running the script, you should see the subjects and senders of your Outlook messages printed in the console. If the request fails, ensure your access token is valid and check for any error messages returned by the API.
Common error codes include:
- 401 Unauthorized: Invalid or expired access token.
- 403 Forbidden: Insufficient permissions to access the resource.
- 404 Not Found: Incorrect API endpoint or resource not available.
For more details on error handling, refer to the Microsoft Graph error documentation.
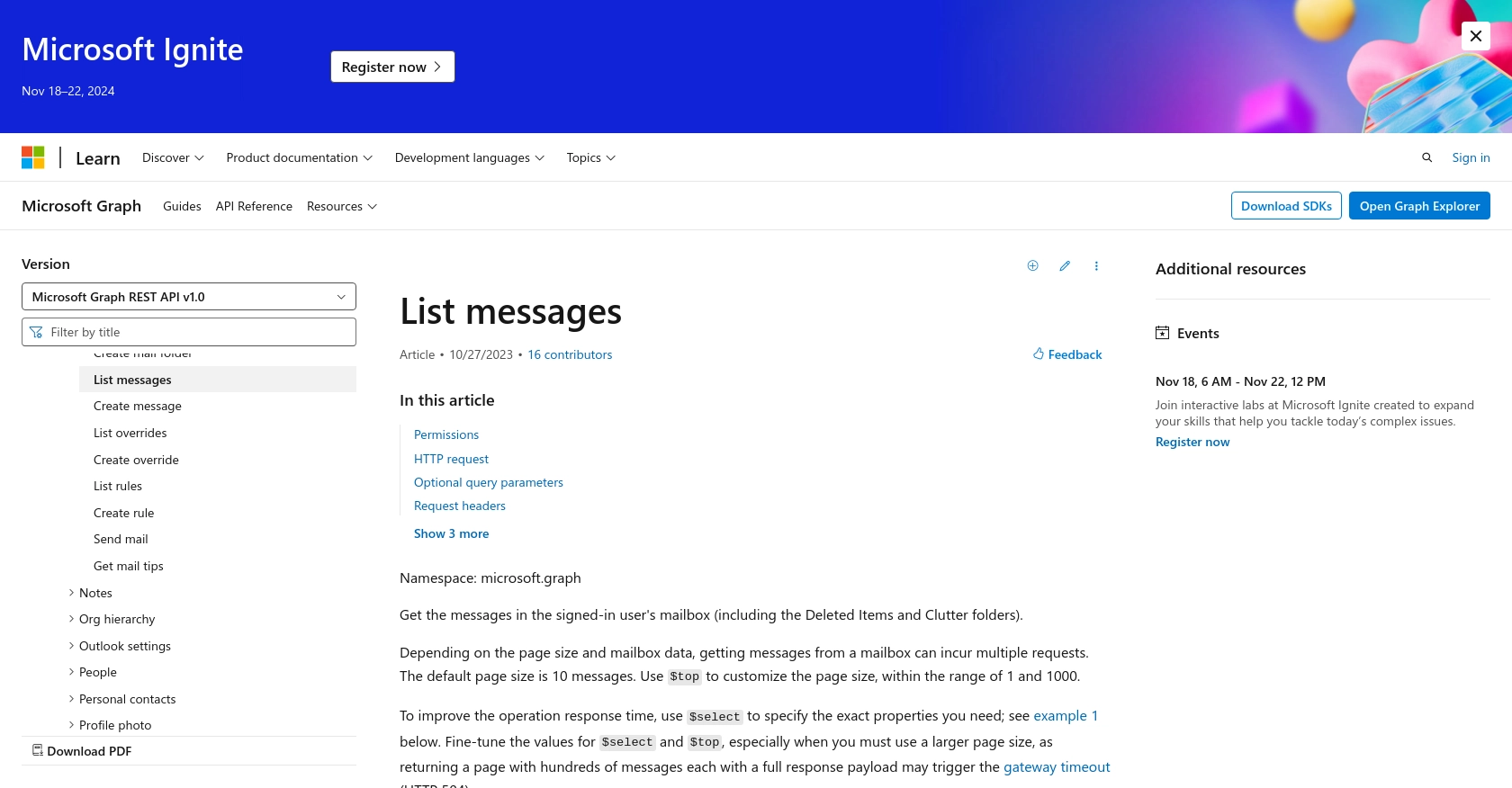
Best Practices for Secure and Efficient Outlook API Integration
When integrating with the Outlook API, it's crucial to follow best practices to ensure security and efficiency. Here are some recommendations:
- Securely Store Credentials: Always store your client ID, client secret, and access tokens securely. Use environment variables or secure vaults to prevent unauthorized access.
- Handle Rate Limiting: Be aware of the API's rate limits to avoid throttling. Implement exponential backoff strategies to handle retries gracefully. For more details, refer to the Microsoft Graph throttling documentation.
- Optimize Data Requests: Use query parameters like
$select
and$top
to limit the data returned by the API, reducing response times and improving performance. - Regularly Refresh Tokens: Access tokens are short-lived. Use refresh tokens to obtain new access tokens without user intervention, ensuring uninterrupted access.
Enhance Your Integration Strategy with Endgrate
Integrating multiple APIs can be complex and time-consuming. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Outlook. By using Endgrate, you can:
- Save time and resources by outsourcing integrations, allowing you to focus on your core product.
- Build once for each use case instead of multiple times for different integrations.
- Offer an intuitive integration experience for your customers, enhancing user satisfaction.
Explore how Endgrate can streamline your integration efforts by visiting Endgrate.
Read More
- https://endgrate.com/provider/outlook
- https://docs.microsoft.com/en-us/graph/overview
- https://learn.microsoft.com/en-us/graph/auth/auth-concepts
- https://learn.microsoft.com/en-us/graph/auth-register-app-v2
- https://learn.microsoft.com/en-us/graph/auth-v2-user?tabs=http
- https://learn.microsoft.com/en-us/graph/api/user-list-messages?view=graph-rest-1.0&tabs=http
Ready to get started?