Using the Pipeliner CRM API to Get Contacts (with PHP examples)
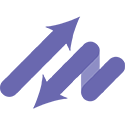
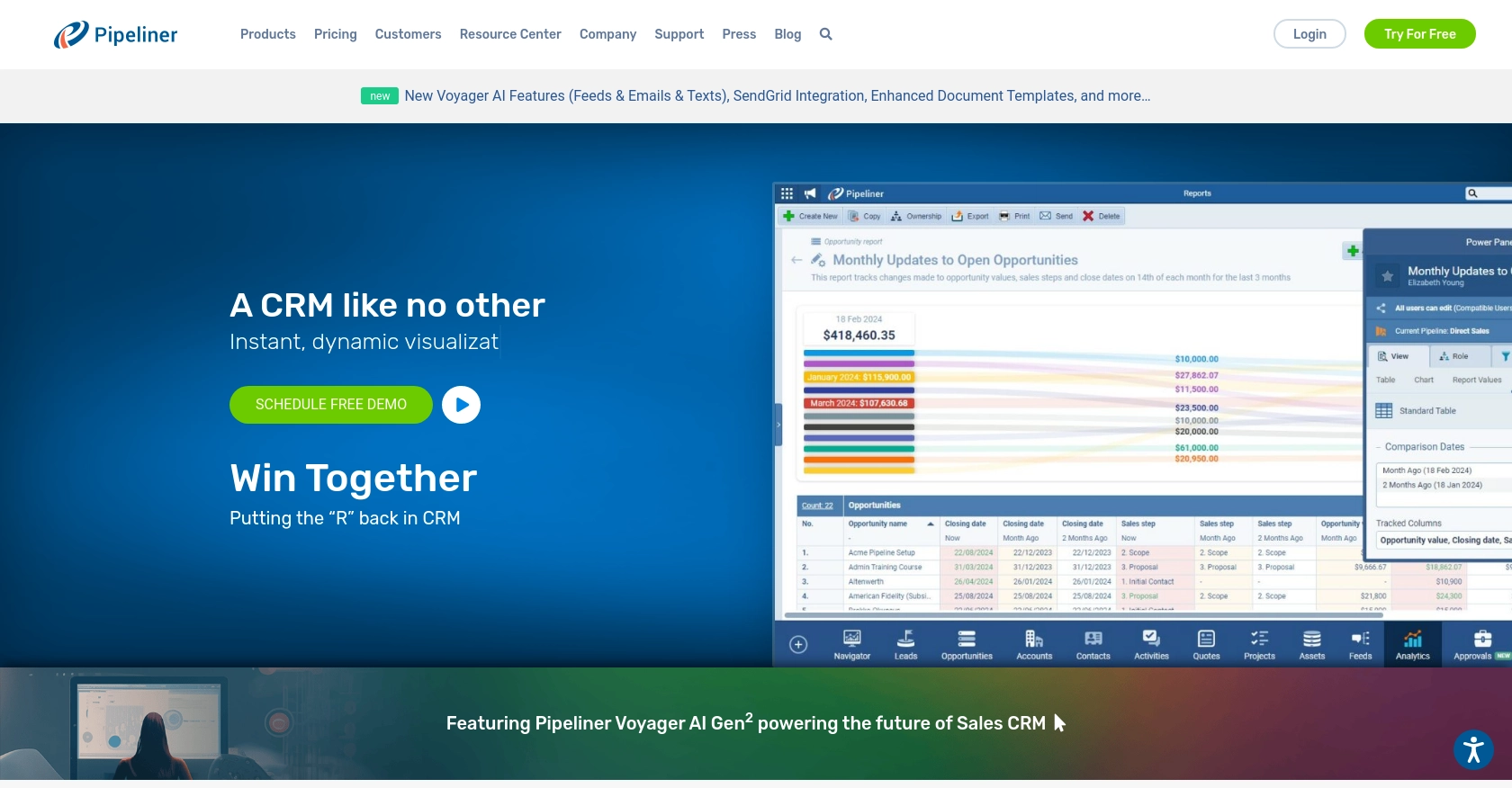
Introduction to Pipeliner CRM
Pipeliner CRM is a dynamic customer relationship management platform designed to enhance sales processes and improve customer interactions for businesses. With its intuitive interface and robust features, Pipeliner CRM helps sales teams manage leads, track opportunities, and optimize their sales pipelines efficiently.
Developers may want to integrate with Pipeliner CRM to access and manage contact data, enabling seamless synchronization of customer information across various platforms. For example, using the Pipeliner CRM API, a developer can retrieve contact details to update a centralized database or trigger personalized marketing campaigns based on customer interactions.
Setting Up Your Pipeliner CRM Test Account
Before you can start interacting with the Pipeliner CRM API, you need to set up a test account. This will allow you to safely experiment with API calls without affecting live data. Follow these steps to create and configure your Pipeliner CRM environment.
Creating a Pipeliner CRM Account
If you don't already have a Pipeliner CRM account, you can sign up for one by visiting the Pipeliner CRM website. Follow the on-screen instructions to create your account. Once your account is set up, log in to access your CRM space.
Generating API Keys for Pipeliner CRM
Pipeliner CRM uses Basic authentication, which requires a username and password. These credentials are generated as API keys specific to your team space. Here's how to obtain them:
- Log in to your Pipeliner CRM account and select your space.
- Navigate to the administration section.
- Under the "Unit, Users & Roles" tab, open "Applications."
- Create a new application and click "Show API Access" to generate your API keys.
- Store the generated username and password securely, as they will be used for API authentication.
Note: These credentials are generated only once, so ensure they are stored safely.
Understanding Pipeliner CRM API Authentication
With your API keys in hand, you can authenticate your API requests using Basic authentication. This involves including your username and password in the request headers. Ensure you keep these credentials confidential to maintain the security of your data.
For more information on authentication, refer to the Pipeliner CRM API Authentication Documentation.
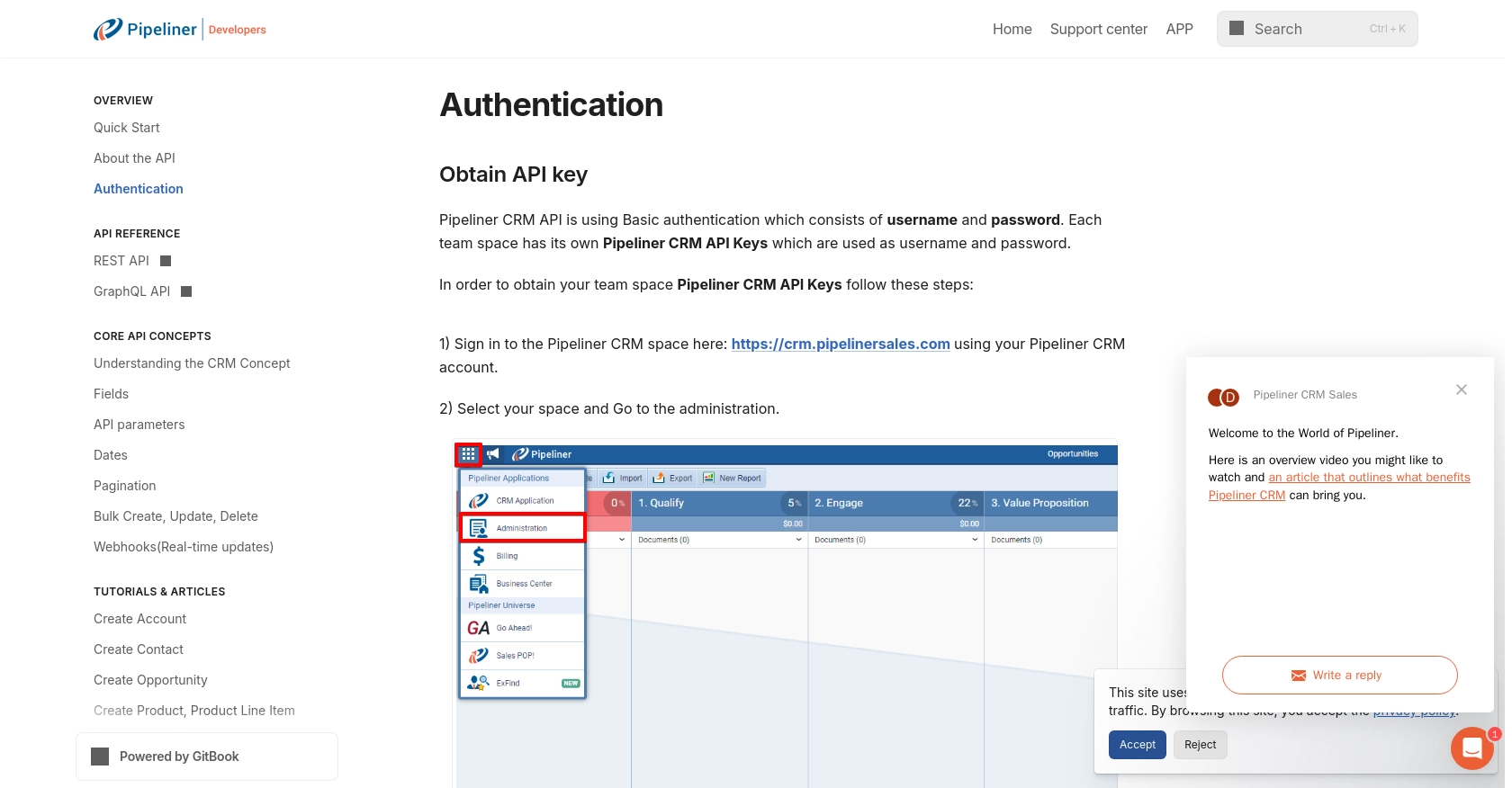
sbb-itb-96038d7
Making API Calls to Retrieve Contacts from Pipeliner CRM Using PHP
To interact with the Pipeliner CRM API and retrieve contact data, you'll need to make HTTP requests using PHP. This section will guide you through the process of setting up your PHP environment, making the API call, and handling the response.
Setting Up Your PHP Environment for Pipeliner CRM API Integration
Before you begin, ensure that you have PHP installed on your machine. You can download the latest version from the official PHP website. Additionally, you'll need the cURL
extension enabled, which is commonly used for making HTTP requests in PHP.
To verify that cURL
is enabled, you can create a PHP file with the following content and run it:
<?php
phpinfo();
?>
Look for the cURL
section in the output to ensure it's enabled.
Example Code to Retrieve Contacts from Pipeliner CRM
Now that your environment is ready, you can proceed with making an API call to retrieve contacts from Pipeliner CRM. Below is a sample PHP script that demonstrates how to do this:
<?php
// Pipeliner CRM API credentials
$username = 'your_api_username';
$password = 'your_api_password';
$spaceId = 'your_space_id';
$serviceUrl = 'your_service_url';
// API endpoint for retrieving contacts
$url = $serviceUrl . '/entities/Contacts';
// Initialize cURL session
$ch = curl_init($url);
// Set cURL options
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_HTTPAUTH, CURLAUTH_BASIC);
curl_setopt($ch, CURLOPT_USERPWD, "$username:$password");
// Execute cURL request
$response = curl_exec($ch);
// Check for errors
if (curl_errno($ch)) {
echo 'Request Error:' . curl_error($ch);
} else {
// Decode the JSON response
$data = json_decode($response, true);
// Display the contacts
foreach ($data['data'] as $contact) {
echo 'Contact Name: ' . $contact['name'] . '<br>';
echo 'Email: ' . $contact['email'] . '<br><br>';
}
}
// Close cURL session
curl_close($ch);
?>
Replace your_api_username
, your_api_password
, your_space_id
, and your_service_url
with your actual Pipeliner CRM API credentials and service URL.
Verifying Successful API Requests and Handling Errors
After executing the script, you should see a list of contacts displayed. If the request is successful, the contacts will be retrieved from your Pipeliner CRM space. You can verify this by checking the contact data in your Pipeliner CRM account.
In case of errors, the script will output the error message. Common issues might include incorrect credentials or network connectivity problems. Ensure your API keys are correct and that your network allows outbound HTTP requests.
For more details on handling errors and understanding response codes, refer to the Pipeliner CRM API Documentation.
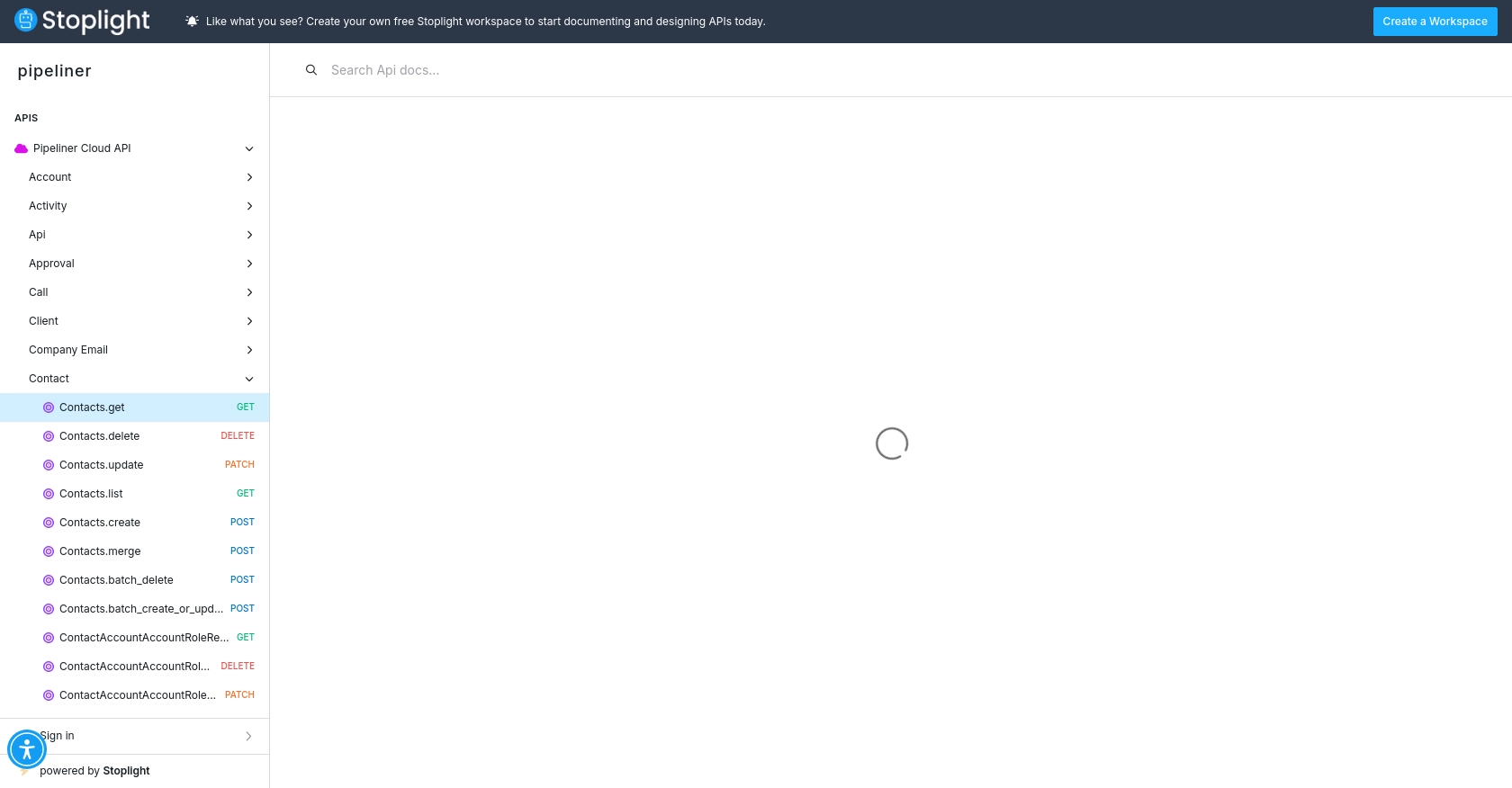
Best Practices for Using Pipeliner CRM API with PHP
When working with the Pipeliner CRM API, it's essential to follow best practices to ensure efficient and secure integration. Here are some recommendations:
- Securely Store Credentials: Always store your API credentials securely. Avoid hardcoding them in your scripts. Consider using environment variables or secure vaults to manage sensitive information.
- Handle Rate Limiting: Be mindful of the API's rate limits to avoid throttling. Implement exponential backoff strategies to handle rate limit errors gracefully.
- Paginate API Requests: Use pagination to efficiently handle large datasets. Pipeliner CRM supports cursor-based pagination, allowing you to retrieve data in manageable chunks.
- Implement Error Handling: Always check for errors in API responses and handle them appropriately. This includes checking HTTP status codes and parsing error messages.
- Data Transformation: Standardize and transform data fields as needed to ensure compatibility with your systems. This can help maintain data consistency across platforms.
Streamlining Integrations with Endgrate
Integrating with multiple platforms can be time-consuming and complex. Endgrate simplifies this process by offering a unified API endpoint that connects to various services, including Pipeliner CRM. By using Endgrate, you can:
- Save Time and Resources: Focus on your core product while Endgrate handles the integration complexities.
- Build Once, Deploy Everywhere: Create a single integration for multiple platforms, reducing development overhead.
- Enhance Customer Experience: Provide a seamless and intuitive integration experience for your users.
Explore how Endgrate can transform your integration strategy by visiting Endgrate today.
Read More
Ready to get started?