Using the Sugar Market API to Create Or Update Accounts (with Javascript examples)
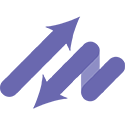
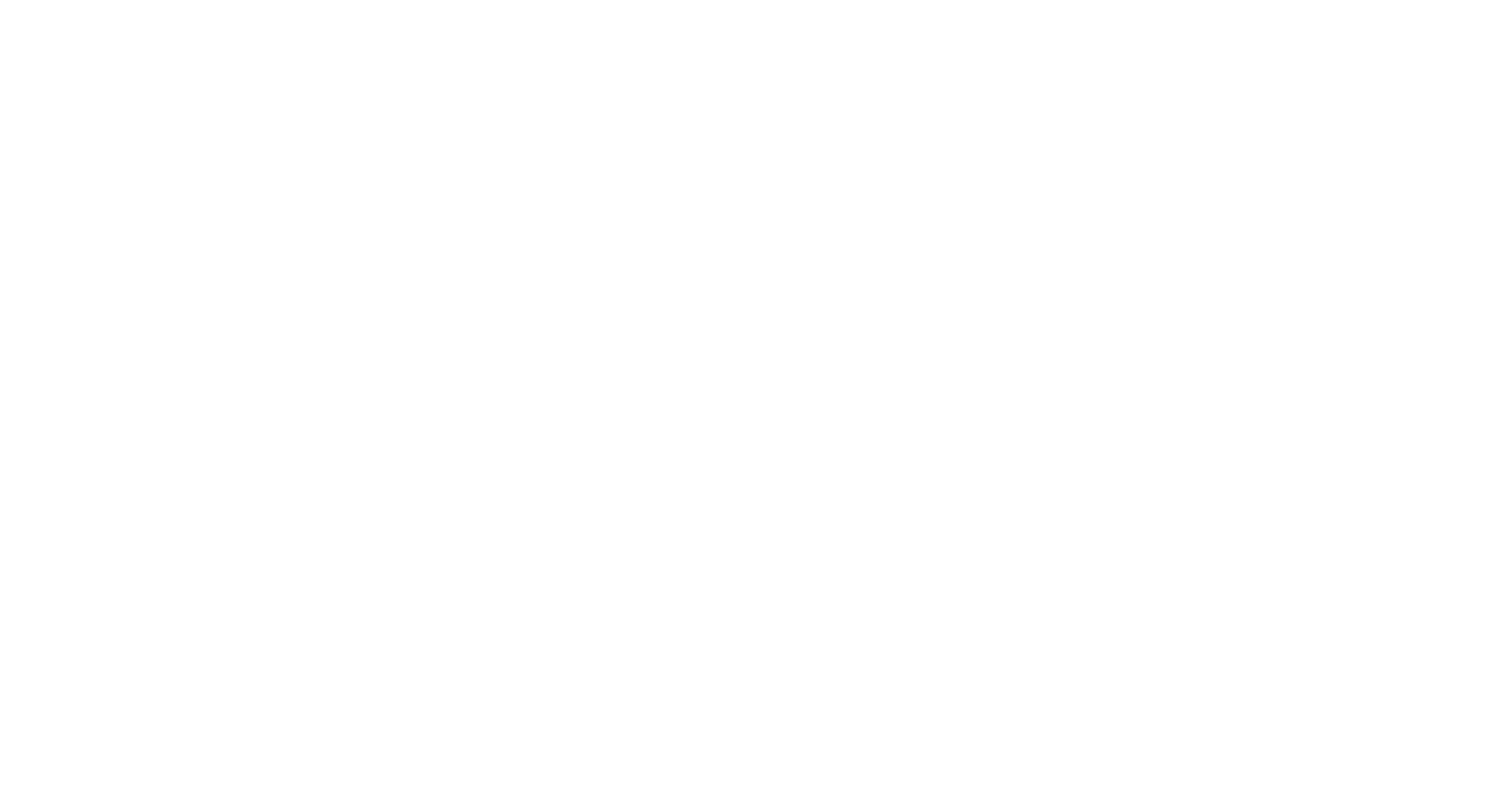
Introduction to Sugar Market API
Sugar Market, a part of the SugarCRM suite, is a powerful marketing automation platform designed to enhance marketing efforts through advanced analytics, email marketing, and lead nurturing. It provides businesses with the tools needed to create personalized marketing campaigns and track their effectiveness.
Developers may want to integrate with the Sugar Market API to streamline account management processes, such as creating or updating account information directly from their applications. For example, a developer could use the Sugar Market API to automatically update account details when changes occur in a connected CRM system, ensuring data consistency across platforms.
Setting Up a Sugar Market Test or Sandbox Account
Before you can start integrating with the Sugar Market API, it's essential to set up a test or sandbox account. This allows you to safely experiment with API calls without affecting live data. Follow these steps to get started:
Creating a Sugar Market Account
If you don't already have a Sugar Market account, you'll need to sign up for one. Visit the Sugar Market website and follow the instructions to create a new account. Look for options to access a free trial or a sandbox environment specifically for developers.
Generating API Credentials for Sugar Market
Once your account is set up, you'll need to generate API credentials to authenticate your requests. Sugar Market uses a custom authentication method, so follow these steps to obtain the necessary credentials:
- Log in to your Sugar Market account.
- Navigate to the API settings section, typically found under developer or integration settings.
- Create a new API application, providing any required details such as application name and description.
- Once the application is created, you'll receive a client ID and client secret. Keep these credentials secure, as they will be used to authenticate your API requests.
Configuring OAuth for Sugar Market API
To authenticate using OAuth, you will need to configure your application to use the client ID and client secret obtained earlier. Follow these steps to set up OAuth authentication:
- Use the client ID and client secret to request an access token from the Sugar Market authorization server.
- Include the access token in the header of your API requests to authenticate them.
- Ensure that your application can handle token expiration by refreshing the token as needed.
With your test account and API credentials set up, you're ready to start making API calls to Sugar Market. In the next section, we'll explore how to create or update accounts using JavaScript.
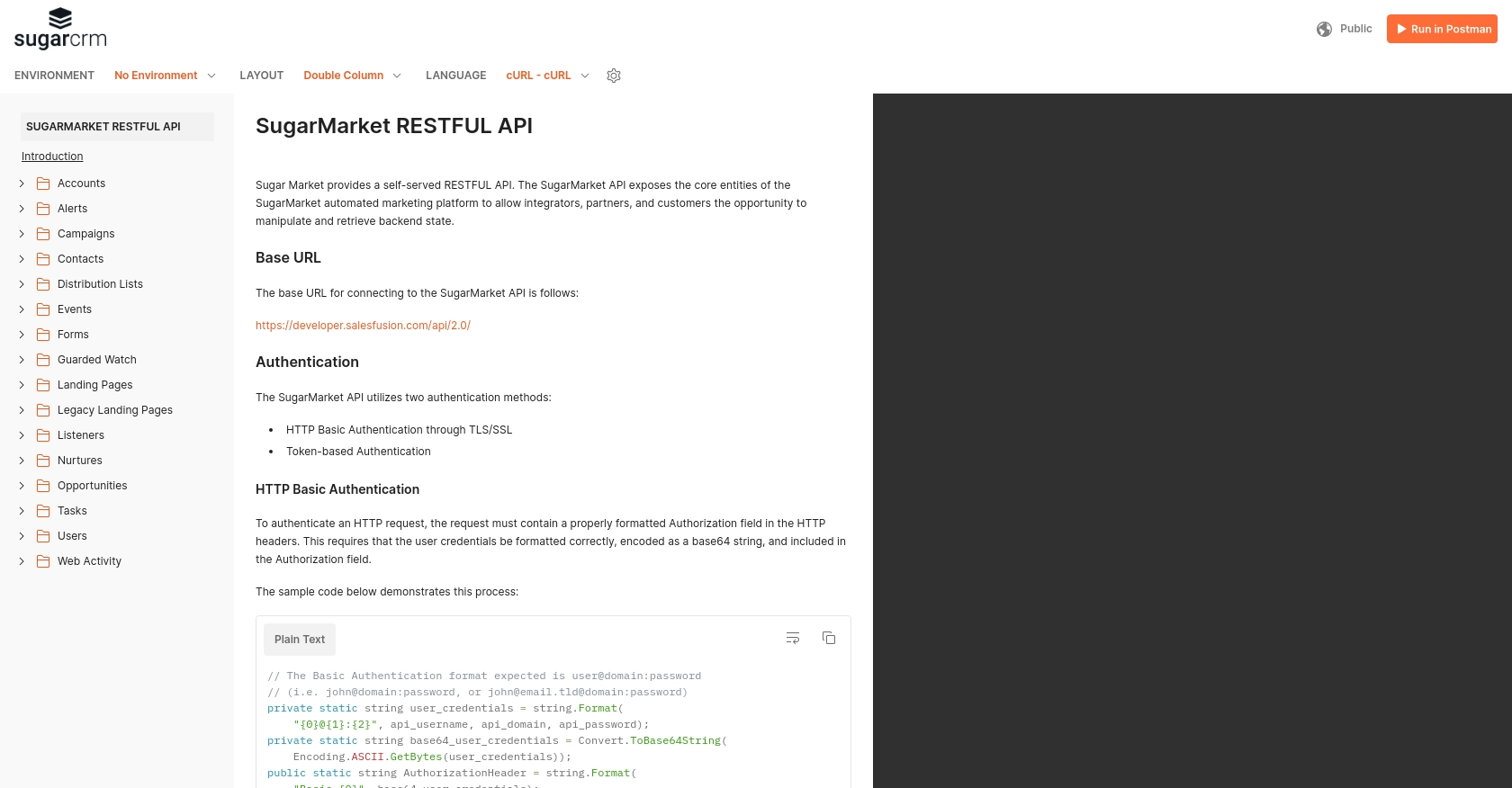
sbb-itb-96038d7
Making API Calls to Sugar Market with JavaScript
To interact with the Sugar Market API using JavaScript, you'll need to ensure you have the right environment set up. This includes having Node.js installed, which allows you to run JavaScript code outside of a browser. Additionally, you'll need to install the axios
library, a popular HTTP client for making requests.
Setting Up Your JavaScript Environment for Sugar Market API
First, ensure you have Node.js installed. You can download it from the official Node.js website. Once installed, you can use npm (Node Package Manager) to install the axios
library by running the following command in your terminal:
npm install axios
Creating or Updating Accounts with Sugar Market API
With your environment ready, you can now create or update accounts in Sugar Market using JavaScript. Below is an example of how to make a POST request to the Sugar Market API to create or update an account.
const axios = require('axios');
// Define the API endpoint and headers
const endpoint = 'https://api.sugarmarket.com/v1/accounts';
const headers = {
'Content-Type': 'application/json',
'Authorization': 'Bearer YOUR_ACCESS_TOKEN'
};
// Define the account data
const accountData = {
name: 'New Account Name',
industry: 'Technology',
website: 'https://example.com'
};
// Function to create or update an account
async function createOrUpdateAccount() {
try {
const response = await axios.post(endpoint, accountData, { headers });
console.log('Account created or updated successfully:', response.data);
} catch (error) {
console.error('Error creating or updating account:', error.response ? error.response.data : error.message);
}
}
// Call the function
createOrUpdateAccount();
Replace YOUR_ACCESS_TOKEN
with the access token obtained during the authentication setup. The accountData
object contains the details of the account you wish to create or update.
Verifying API Call Success in Sugar Market
After executing the code, you can verify the success of your API call by checking the response data in the console. Additionally, log in to your Sugar Market sandbox account to confirm that the account has been created or updated as expected.
Handling Errors and Error Codes from Sugar Market API
When making API calls, it's crucial to handle potential errors gracefully. The example code above includes error handling that logs the error message or response data if the request fails. Refer to the Sugar Market API documentation for detailed information on error codes and their meanings.
Best Practices for Using Sugar Market API
When working with the Sugar Market API, it's important to follow best practices to ensure efficient and secure integration. Here are some key recommendations:
- Securely Store Credentials: Always store your client ID, client secret, and access tokens securely. Avoid hardcoding them in your source code. Instead, use environment variables or secure vaults to manage sensitive information.
- Handle Rate Limiting: Be aware of any rate limits imposed by the Sugar Market API. Implement logic to handle rate limit responses gracefully, such as retrying requests after a delay. Refer to the Sugar Market API documentation for specific rate limit details.
- Data Transformation and Standardization: Ensure that data being sent to or received from the Sugar Market API is properly transformed and standardized to match your application's data structures. This helps maintain data consistency across systems.
- Error Handling: Implement robust error handling to manage API call failures. Log errors for debugging and provide meaningful feedback to users when issues occur.
Streamlining Integrations with Endgrate
Building and maintaining integrations with multiple platforms can be time-consuming and complex. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Sugar Market. By using Endgrate, you can:
- Save Time and Resources: Focus on your core product development while outsourcing integration complexities to Endgrate.
- Build Once, Use Everywhere: Develop a single integration for each use case, rather than multiple integrations for different platforms.
- Enhance User Experience: Offer your customers an intuitive and seamless integration experience across platforms.
Explore how Endgrate can transform your integration strategy by visiting Endgrate's website and discover the benefits of a streamlined integration process.
Read More
Ready to get started?