Using the VTiger API to Get Records (with PHP examples)
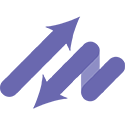
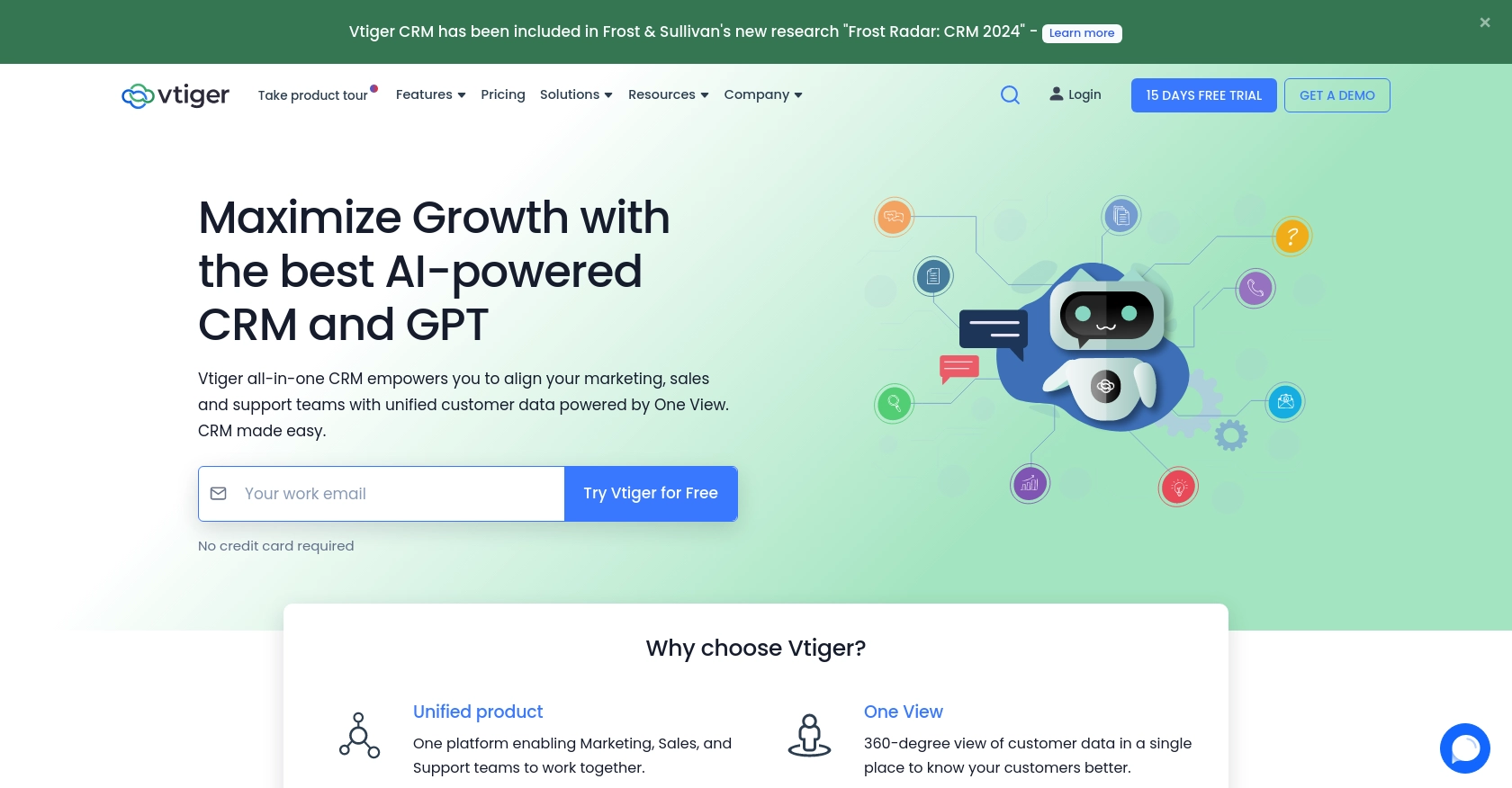
Introduction to VTiger CRM API Integration
VTiger CRM is a robust customer relationship management platform that offers a wide range of tools to help businesses manage their customer interactions, sales, and marketing efforts. Known for its flexibility and comprehensive features, VTiger is a popular choice for organizations looking to streamline their CRM processes.
Integrating with the VTiger API allows developers to automate and enhance CRM functionalities, such as retrieving and managing records. For example, a developer might use the VTiger API to extract customer data and integrate it with other business applications, enabling seamless data flow and improved decision-making.
Setting Up Your VTiger CRM Test/Sandbox Account
Before you can start interacting with the VTiger API, you need to set up a test or sandbox account. This will allow you to safely experiment with API calls without affecting live data. Follow these steps to get started:
Creating a VTiger CRM Account
If you don't already have a VTiger account, you can sign up for a free trial or use the demo version available on the VTiger website. This will give you access to the CRM features and the ability to test API integrations.
- Visit the VTiger website and click on the "Free Trial" button.
- Fill out the registration form with your details and submit it.
- Check your email for a confirmation link and follow the instructions to activate your account.
Generating API Access Key for VTiger
VTiger uses HTTP Basic Auth for API authentication, which requires a username and an access key. Here's how to generate your access key:
- Log in to your VTiger CRM account.
- Navigate to "My Preferences" from the user menu.
- Locate the "Access Key" section and copy the access key provided. This key is unique to your user account and will be used for API authentication.
Configuring VTiger API Authentication
With your access key in hand, you can now set up authentication for your API requests. VTiger's API expects the username and access key to be included in the HTTP headers:
// Set the API endpoint and authentication details
$endpoint = "https://your_instance.odx.vtiger.com/restapi/v1/vtiger/default";
$username = "your_username";
$accessKey = "your_access_key";
// Create a stream context with HTTP Basic Auth
$options = [
"http" => [
"header" => "Authorization: Basic " . base64_encode("$username:$accessKey")
]
];
$context = stream_context_create($options);
Replace your_instance
, your_username
, and your_access_key
with your actual VTiger instance URL, username, and access key.
Testing Your VTiger API Connection
To ensure that your setup is correct, you can make a simple API call to verify the connection:
// Example API call to list available modules
$response = file_get_contents("$endpoint/listtypes?fieldTypeList=null", false, $context);
$data = json_decode($response, true);
if ($data['success']) {
echo "Connection successful!";
} else {
echo "Connection failed: " . $data['error']['message'];
}
This code snippet makes a request to the VTiger API to list available modules, confirming that your authentication is working correctly.
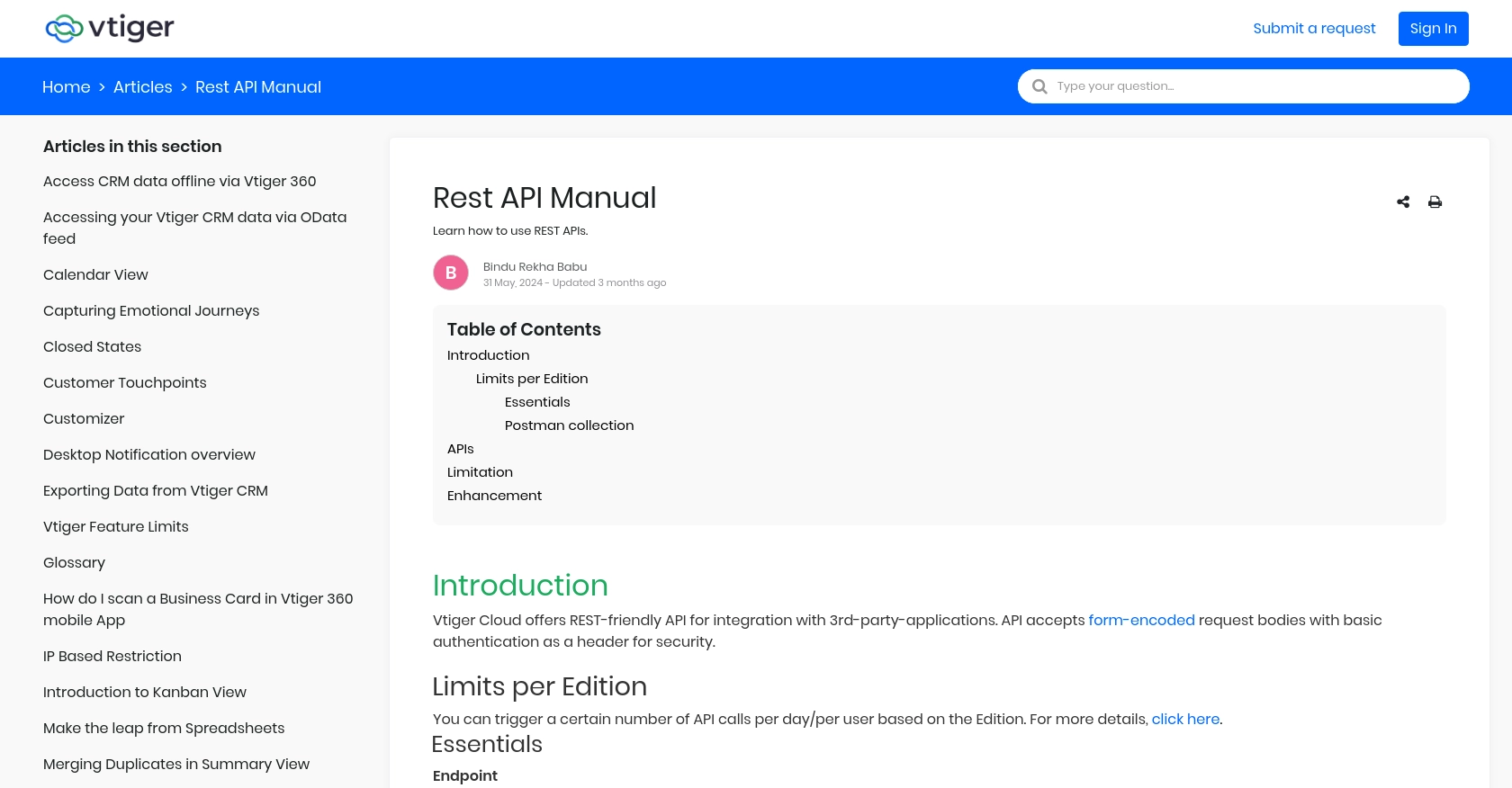
sbb-itb-96038d7
Making API Calls to Retrieve VTiger Records Using PHP
In this section, we'll explore how to interact with the VTiger API to retrieve records using PHP. This involves setting up your PHP environment, making API requests, and handling responses effectively.
Setting Up PHP Environment for VTiger API Integration
Before making API calls, ensure your PHP environment is correctly configured. You'll need PHP 7.4 or later and the cURL extension enabled. You can verify your PHP version and extensions using the following command:
php -v
php -m | grep curl
If cURL is not enabled, you can enable it by modifying your php.ini
file and restarting your web server.
Installing Required PHP Dependencies
To simplify HTTP requests, we'll use the Guzzle HTTP client. Install it via Composer:
composer require guzzlehttp/guzzle
Retrieving VTiger Records with PHP
Now, let's write a PHP script to retrieve records from VTiger. We'll use the "query" API to fetch records from a specific module. Here's a step-by-step guide:
require 'vendor/autoload.php';
use GuzzleHttp\Client;
// Set up API endpoint and authentication details
$endpoint = "https://your_instance.odx.vtiger.com/restapi/v1/vtiger/default/query";
$username = "your_username";
$accessKey = "your_access_key";
// Create a new Guzzle client
$client = new Client();
// Define the query to retrieve records
$objectType = "Contacts"; // Change this to your desired module
$query = "select * from $objectType limit 10";
try {
// Make the API request
$response = $client->request('GET', $endpoint, [
'headers' => [
'Authorization' => 'Basic ' . base64_encode("$username:$accessKey")
],
'query' => ['query' => $query]
]);
// Parse the JSON response
$data = json_decode($response->getBody(), true);
if ($data['success']) {
// Output the retrieved records
foreach ($data['result'] as $record) {
echo "Record ID: " . $record['id'] . "\n";
echo "Name: " . $record['firstname'] . " " . $record['lastname'] . "\n\n";
}
} else {
echo "Error: " . $data['error']['message'];
}
} catch (Exception $e) {
echo "Request failed: " . $e->getMessage();
}
Replace your_instance
, your_username
, and your_access_key
with your actual VTiger instance URL, username, and access key. Adjust the $objectType
variable to specify the module you wish to query.
Handling API Response and Errors
Upon executing the script, you should see a list of records from the specified module. If the request fails, the script will output an error message. Common HTTP response codes include:
- 200: Success
- 400: Bad Request
- 500: Internal Server Error
For more detailed error handling, refer to the VTiger API documentation.
Conclusion and Best Practices for VTiger API Integration with PHP
Integrating with the VTiger API using PHP offers a powerful way to automate CRM processes and enhance business operations. By following the steps outlined in this guide, developers can efficiently retrieve and manage records, enabling seamless data integration with other business applications.
Best Practices for Secure and Efficient VTiger API Usage
- Secure Storage of Credentials: Always store your VTiger username and access key securely, using environment variables or secure vaults to prevent unauthorized access.
- Handle API Rate Limits: Be mindful of VTiger's API rate limits to avoid service disruptions. Implement retry logic and exponential backoff strategies to manage rate limiting effectively.
- Data Standardization: Ensure that data retrieved from VTiger is standardized and transformed as needed to maintain consistency across integrated systems.
- Error Handling: Implement robust error handling to manage API response codes and exceptions, ensuring that your application can gracefully recover from failures.
Streamlining Integrations with Endgrate
While integrating with VTiger API directly can be effective, using a tool like Endgrate can further simplify the process. Endgrate provides a unified API endpoint that connects to multiple platforms, including VTiger, allowing developers to manage integrations more efficiently.
By leveraging Endgrate, you can save time and resources, focus on your core product, and provide an intuitive integration experience for your customers. Explore how Endgrate can enhance your integration strategy by visiting Endgrate's website.
Read More
Ready to get started?