Using the Xero API to Create or Update Items in Python
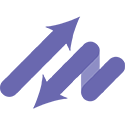
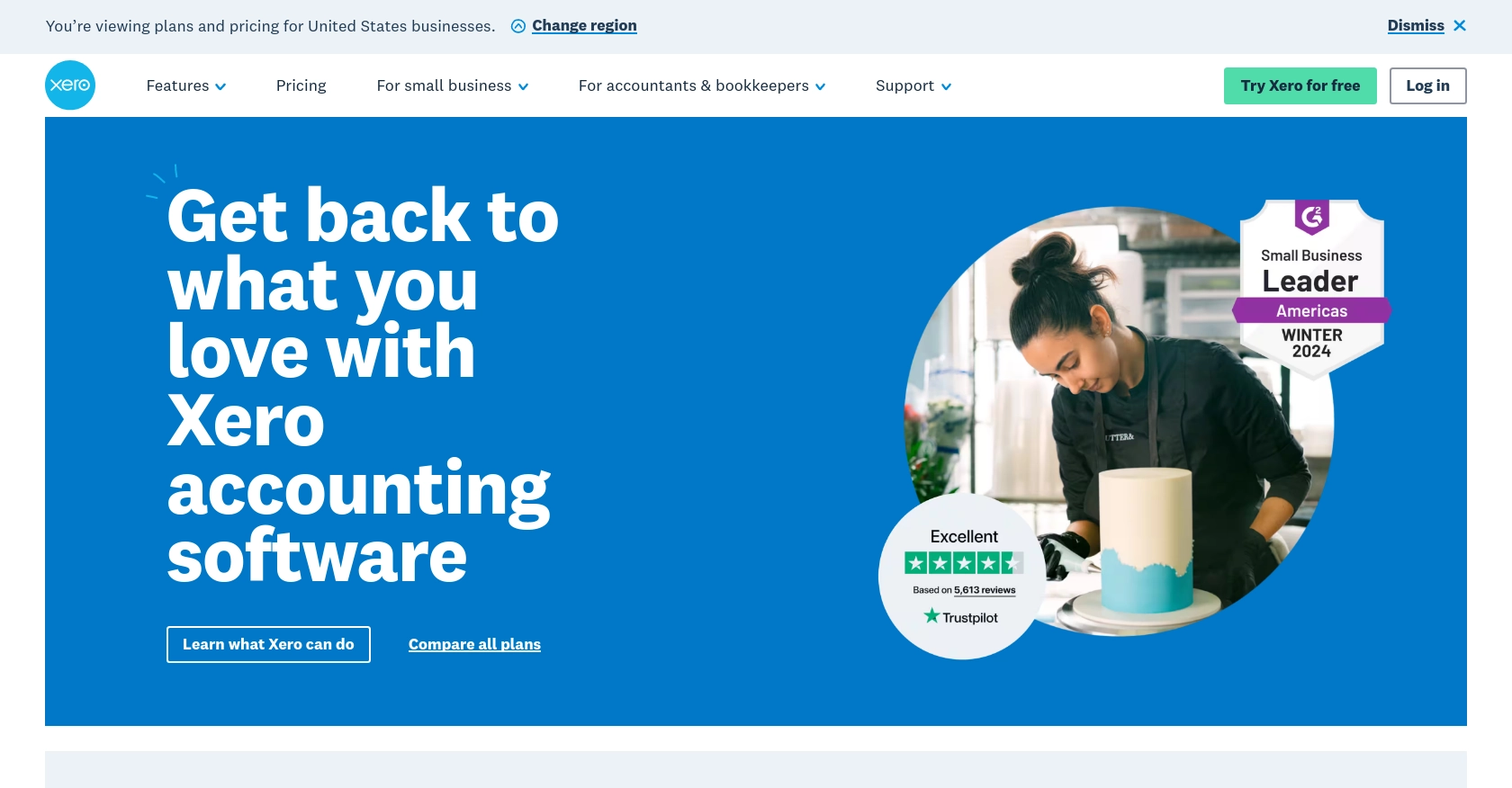
Introduction to Xero API for Item Management
Xero is a powerful cloud-based accounting software platform designed to help small and medium-sized businesses manage their finances with ease. It offers a comprehensive suite of tools for invoicing, expense tracking, payroll, and more, making it a popular choice for businesses looking to streamline their accounting processes.
For developers, integrating with Xero's API can unlock numerous possibilities for automating financial tasks. One common use case is managing inventory items. By using the Xero API, developers can create or update items in the inventory, ensuring that the data remains consistent and up-to-date across different platforms.
This article will guide you through the process of using Python to interact with the Xero API, specifically focusing on creating or updating inventory items. Whether you're building a custom application or integrating Xero with existing systems, this tutorial will provide you with the foundational knowledge needed to get started.
Setting Up Your Xero Sandbox Account for API Integration
Before diving into the Xero API to create or update items, you'll need to set up a sandbox account. This allows you to test your integration without affecting live data. Xero provides a demo company for developers to experiment with API calls.
Creating a Xero Developer Account
To begin, you'll need a Xero developer account. Follow these steps:
- Visit the Xero Developer Portal and sign up for a free developer account.
- Once registered, log in to your account and navigate to the My Apps section.
Setting Up a Xero Demo Company
After creating your developer account, you can access a demo company:
- Log in to your Xero account and select the Demo Company from the organization menu.
- This demo company will serve as your sandbox environment for testing API interactions.
Creating a Xero App for OAuth Authentication
The Xero API uses OAuth 2.0 for authentication. Follow these steps to create an app and obtain the necessary credentials:
- In the My Apps section, click on "New App" to create a new application.
- Fill in the required details, such as the app name and company URL.
- Set the redirect URI, which is used during the OAuth flow. This should be a valid URL where you can handle authentication responses.
- Once the app is created, note down the Client ID and Client Secret, as you'll need these for authentication.
For more details on OAuth 2.0 authentication, refer to the Xero OAuth 2.0 Overview.
Configuring OAuth Scopes for Xero API Access
To interact with the Xero API, you'll need to configure the appropriate scopes:
- Navigate to the Scopes section of your app settings.
- Select the scopes required for managing items, such as "accounting.transactions" and "accounting.settings".
For a complete list of scopes, visit the Xero OAuth 2.0 Scopes page.
With your sandbox account and app set up, you're now ready to start making API calls to create or update items in Xero using Python.
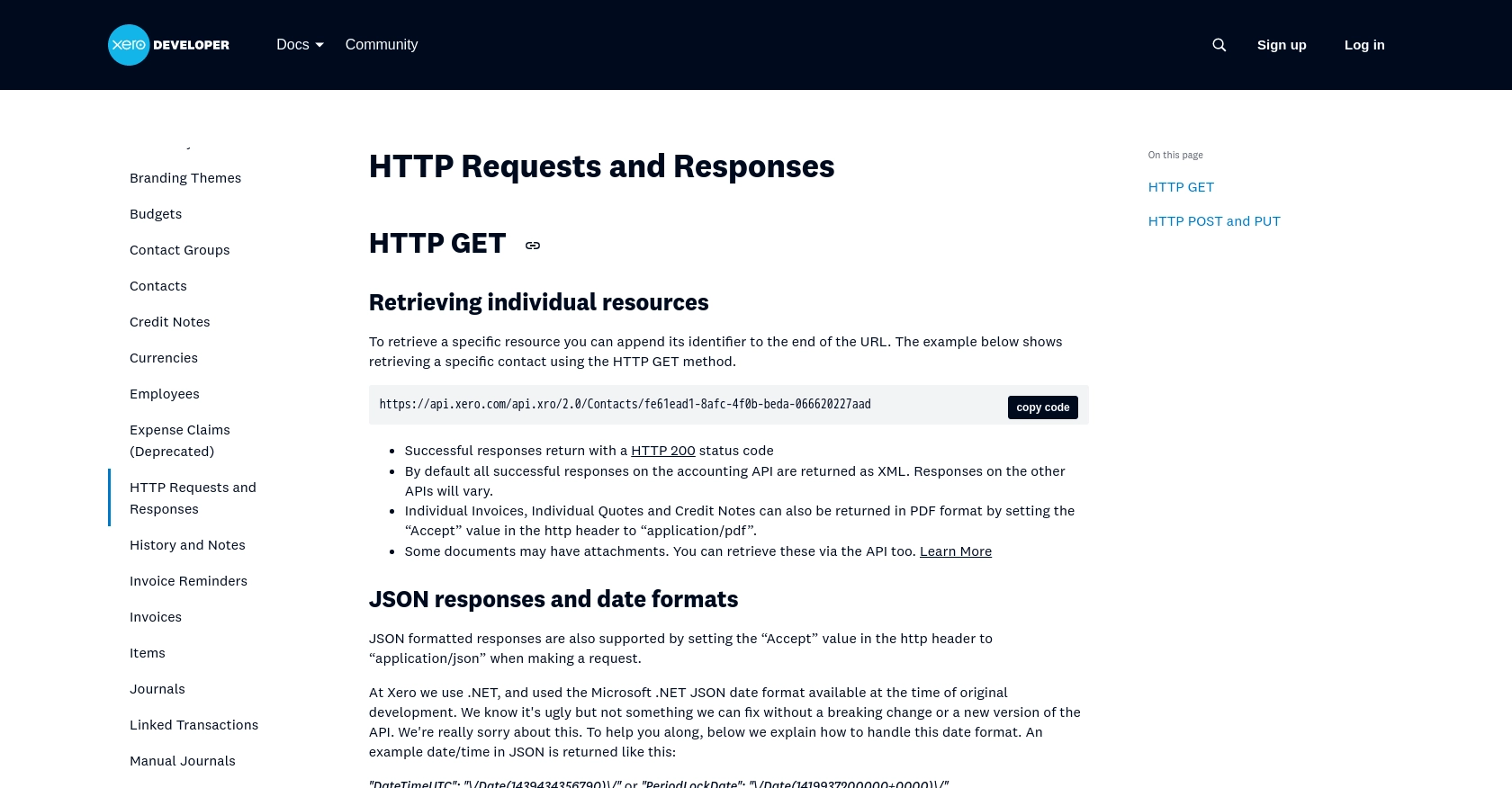
sbb-itb-96038d7
Making API Calls to Xero for Creating or Updating Items Using Python
With your Xero sandbox account and app configured, you're ready to make API calls to create or update items. This section will guide you through the process using Python, ensuring you have the right tools and code to interact with the Xero API effectively.
Setting Up Your Python Environment for Xero API Integration
Before you begin coding, ensure you have the following prerequisites installed on your machine:
- Python 3.11.1
- The Python package installer, pip
You'll also need to install the requests
library to handle HTTP requests. Run the following command in your terminal:
pip install requests
Authenticating with Xero API Using OAuth 2.0
To authenticate with the Xero API, you'll need to implement the OAuth 2.0 flow. Here's a basic example of how to obtain an access token using your Client ID and Client Secret:
import requests
# Define your credentials
client_id = 'Your_Client_ID'
client_secret = 'Your_Client_Secret'
redirect_uri = 'Your_Redirect_URI'
auth_url = 'https://login.xero.com/identity/connect/authorize'
token_url = 'https://identity.xero.com/connect/token'
# Step 1: Direct user to Xero's authorization URL
print(f"Visit the following URL to authorize the app: {auth_url}?response_type=code&client_id={client_id}&redirect_uri={redirect_uri}&scope=offline_access accounting.transactions accounting.settings")
# Step 2: Exchange authorization code for access token
auth_code = input('Enter the authorization code: ')
response = requests.post(token_url, data={
'grant_type': 'authorization_code',
'code': auth_code,
'redirect_uri': redirect_uri,
'client_id': client_id,
'client_secret': client_secret
})
# Parse the access token
token_data = response.json()
access_token = token_data['access_token']
print(f"Access Token: {access_token}")
Creating or Updating Items in Xero Using Python
Once authenticated, you can proceed to create or update items in Xero. Here's a sample code snippet to create an item:
import requests
# Define the API endpoint and headers
endpoint = 'https://api.xero.com/api.xro/2.0/Items'
headers = {
'Authorization': f'Bearer {access_token}',
'Content-Type': 'application/json'
}
# Define the item data
item_data = {
"Code": "ITEM001",
"Description": "Sample Item",
"PurchaseDetails": {
"UnitPrice": 10.00,
"AccountCode": "400"
},
"SalesDetails": {
"UnitPrice": 15.00,
"AccountCode": "200"
}
}
# Make a POST request to create the item
response = requests.post(endpoint, json=item_data, headers=headers)
# Check the response
if response.status_code == 200:
print("Item created successfully.")
else:
print(f"Failed to create item: {response.status_code} - {response.text}")
Replace Your_Client_ID
, Your_Client_Secret
, and Your_Redirect_URI
with your actual credentials. The item_data
dictionary contains the details of the item you wish to create or update.
Verifying API Call Success in Xero Sandbox
After executing the code, verify the success of your API call by checking the Xero sandbox environment. Navigate to the Items section in your demo company to confirm that the item has been created or updated as expected.
Handling Errors and Error Codes in Xero API
It's crucial to handle potential errors when interacting with the Xero API. Common error codes include:
- 400 Bad Request: The request was invalid or cannot be served.
- 401 Unauthorized: Authentication failed or user does not have permissions.
- 403 Forbidden: The request is understood, but it has been refused.
- 404 Not Found: The requested resource could not be found.
For more detailed information on error handling, refer to the Xero API Requests and Responses documentation.
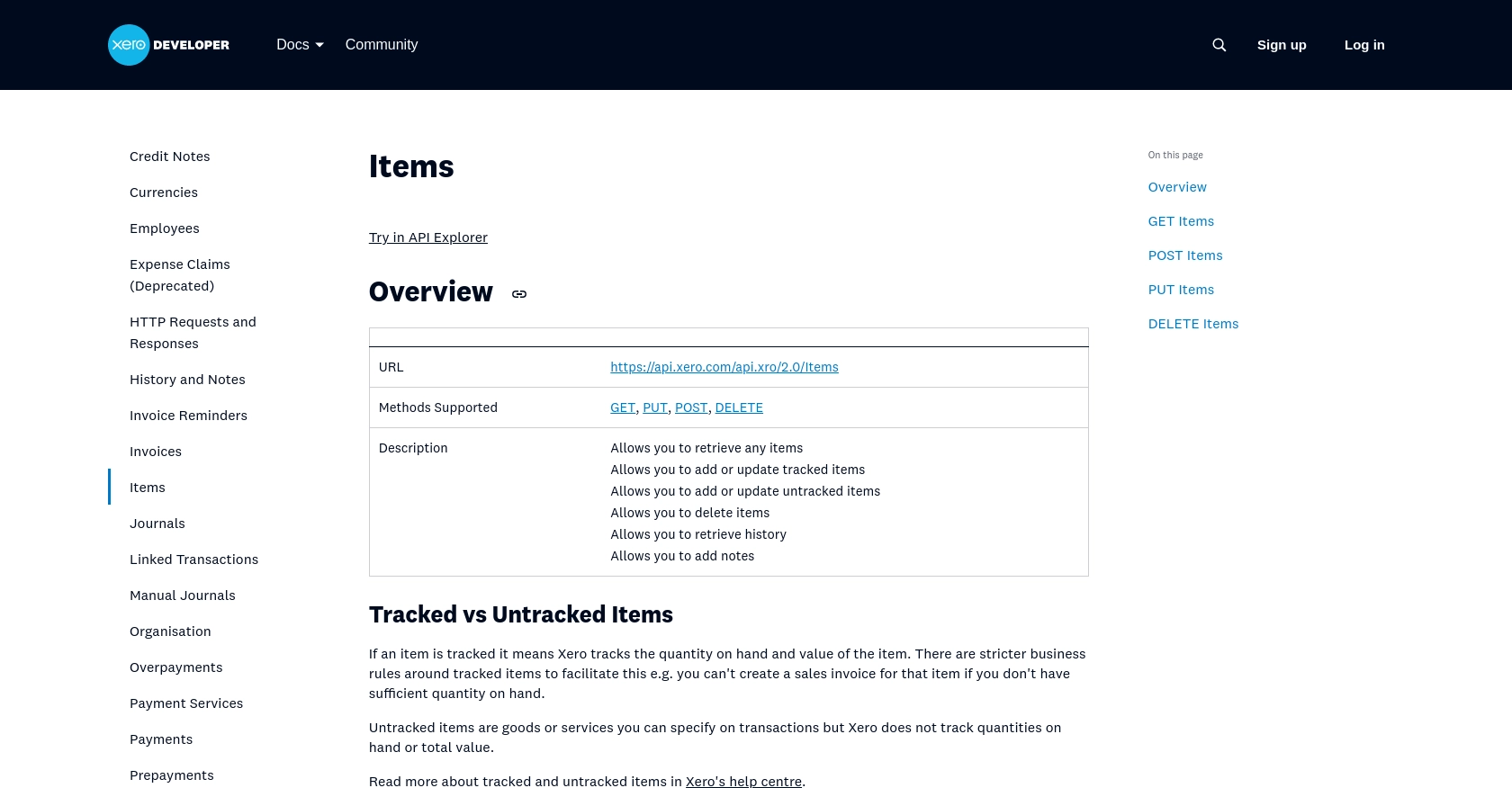
Conclusion and Best Practices for Xero API Integration
Integrating with the Xero API to create or update items using Python can significantly enhance your application's ability to manage inventory efficiently. By following the steps outlined in this guide, you can ensure a seamless interaction with Xero's robust accounting platform.
Best Practices for Secure and Efficient Xero API Usage
- Securely Store Credentials: Always store your Client ID, Client Secret, and access tokens securely. Consider using environment variables or a secure vault to manage sensitive information.
- Handle Rate Limiting: Xero imposes rate limits on API requests. Be mindful of these limits to avoid disruptions. For more details, refer to the Xero API Rate Limits documentation.
- Implement Error Handling: Properly handle errors by checking response codes and implementing retry logic where appropriate. This ensures your application can gracefully recover from temporary issues.
- Data Standardization: Ensure that data fields are standardized across your application to maintain consistency and accuracy when interacting with Xero.
Streamlining Integration with Endgrate
While building integrations with Xero can be rewarding, it can also be time-consuming and complex. Endgrate offers a solution by providing a unified API endpoint that simplifies the integration process across multiple platforms, including Xero.
By leveraging Endgrate, you can save time and resources, allowing you to focus on your core product development. Whether you need to build once for each use case or provide an intuitive integration experience for your customers, Endgrate can help streamline your efforts.
Explore how Endgrate can transform your integration strategy by visiting Endgrate's website and discover the benefits of outsourcing integrations to enhance your application's capabilities.
Read More
- https://endgrate.com/provider/xero
- https://developer.xero.com/documentation/api/accounting/requests-and-responses
- https://developer.xero.com/documentation/guides/oauth2/limits/
- https://developer.xero.com/documentation/guides/oauth2/overview
- https://developer.xero.com/documentation/guides/oauth2/scopes
- https://developer.xero.com/documentation/guides/oauth2/auth-flow
- https://developer.xero.com/documentation/guides/oauth2/tenants
- https://developer.xero.com/documentation/api/accounting/items
Ready to get started?