Using the Younium API to Get Payments in Python
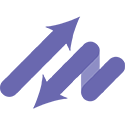
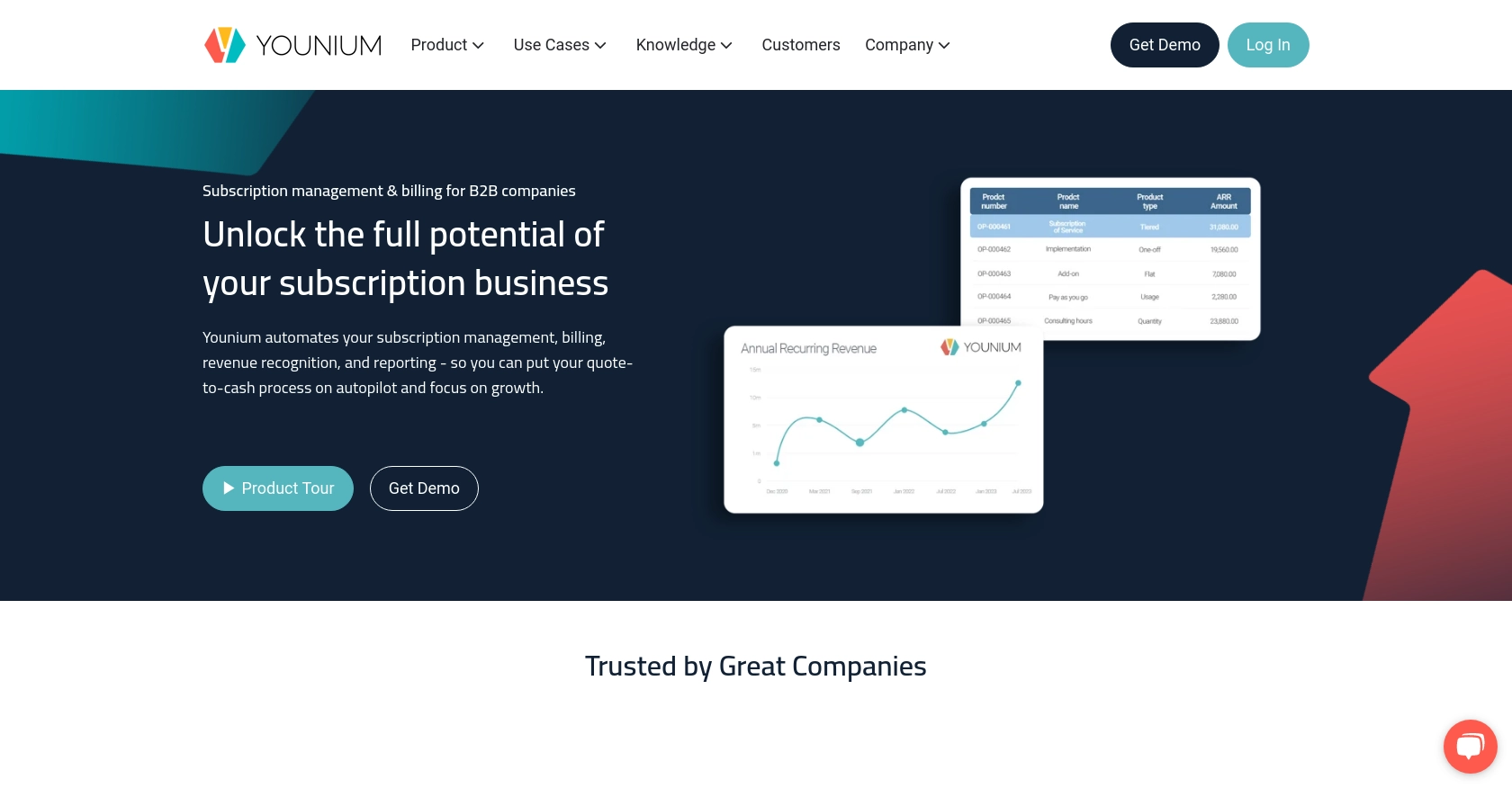
Introduction to Younium API Integration
Younium is a comprehensive subscription management platform designed to streamline billing and financial operations for B2B SaaS companies. It offers a robust API that allows developers to automate and manage various financial processes, including invoicing, payments, and subscription management.
Integrating with the Younium API can significantly enhance a developer's ability to manage payment data efficiently. For example, by using the Younium API, developers can retrieve payment information programmatically, enabling seamless integration with financial reporting tools or dashboards. This capability is particularly useful for businesses looking to automate their financial workflows and gain real-time insights into their payment operations.
Setting Up Your Younium Sandbox Account for API Integration
Before you can start using the Younium API to retrieve payment data, you need to set up a sandbox account. This environment allows you to test your integration without affecting live data, ensuring a smooth development process.
Creating a Younium Sandbox Account
To begin, you need to create a sandbox account with Younium. Follow these steps:
- Visit the Younium Developer Portal and sign up for a sandbox account.
- Once registered, log in to your account to access the sandbox environment.
Generating API Tokens and Client Credentials
Younium uses JWT access tokens for API authentication. Follow these steps to generate the necessary credentials:
- Navigate to your user profile by clicking your name in the top right corner and select “Privacy & Security.”
- In the left panel, click on “Personal Tokens” and then “Generate Token.”
- Provide a relevant description for your token and click “Create.”
- Copy the generated Client ID and Secret Key. These credentials are crucial for generating your JWT access token and will not be visible again.
Acquiring a JWT Access Token
With your client credentials ready, you can now generate a JWT access token:
- Make a POST request to the
/auth/token
endpoint using the following details:
import requests
url = "https://api.sandbox.younium.com/auth/token"
headers = {"Content-Type": "application/json"}
body = {
"clientId": "Your_Client_ID",
"secret": "Your_Secret_Key"
}
response = requests.post(url, headers=headers, json=body)
token_data = response.json()
access_token = token_data["accessToken"]
print("Access Token:", access_token)
Replace Your_Client_ID
and Your_Secret_Key
with the credentials you copied earlier. If successful, this request will return a JWT access token valid for 24 hours.
Handling Authentication Errors
If you encounter errors during authentication, check the following:
- A
400
or401
error indicates invalid credentials or an expired token. Ensure your Client ID and Secret Key are correct. - Refer to the Younium documentation for more details on handling authentication issues.
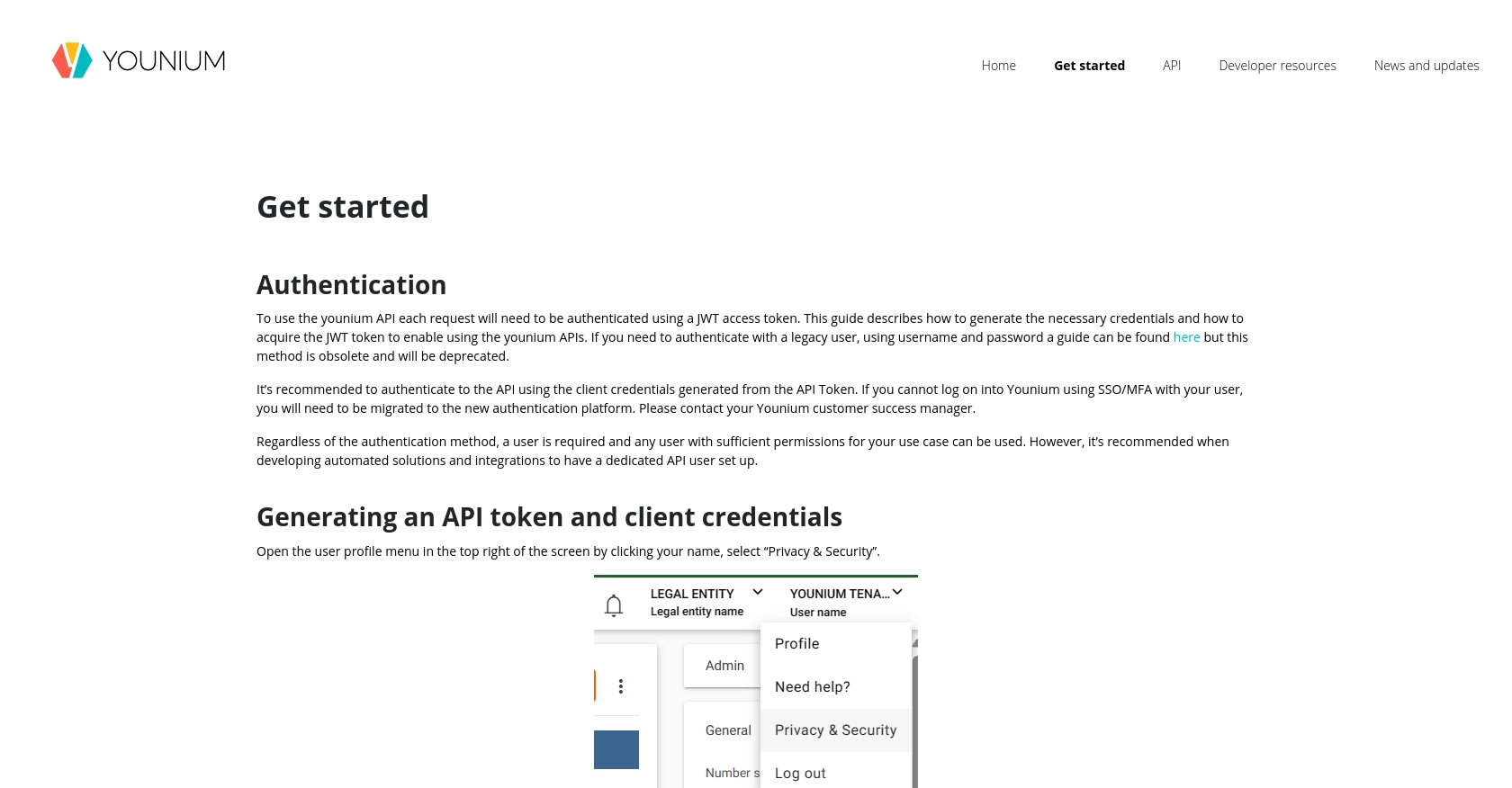
sbb-itb-96038d7
Making API Calls to Retrieve Payments Using Younium API in Python
Now that you have your JWT access token, you can proceed to make API calls to the Younium API to retrieve payment data. This section will guide you through the process of setting up your Python environment and executing the necessary API calls.
Setting Up Your Python Environment for Younium API Integration
To interact with the Younium API, ensure you have Python installed on your machine. This tutorial uses Python 3.11.1. Additionally, you'll need the requests
library to handle HTTP requests. Install it using pip:
pip install requests
Retrieving Payments from Younium API
With your environment ready, you can now write a Python script to retrieve payments. Create a file named get_younium_payments.py
and add the following code:
import requests
# Define the API endpoint and headers
endpoint = "https://api.sandbox.younium.com/payments"
headers = {
"Authorization": "Bearer Your_Access_Token",
"Content-Type": "application/json",
"api-version": "2.1"
}
# Make a GET request to the Younium API
response = requests.get(endpoint, headers=headers)
# Check if the request was successful
if response.status_code == 200:
payments_data = response.json()
print("Payments Data:", payments_data)
else:
print("Failed to retrieve payments:", response.status_code, response.text)
Replace Your_Access_Token
with the JWT access token you obtained earlier. This script sends a GET request to the Younium API to fetch payment data.
Verifying API Call Success and Handling Errors
After running the script, you should see the payment data printed in the console if the request is successful. If the request fails, the script will output the error status code and message. Common error codes include:
401 Unauthorized
: Indicates an expired or invalid access token. Ensure your token is correct and not expired.403 Forbidden
: Occurs if the legal entity specified is incorrect or if the user lacks necessary permissions.
For more details on error handling, refer to the Younium API documentation.
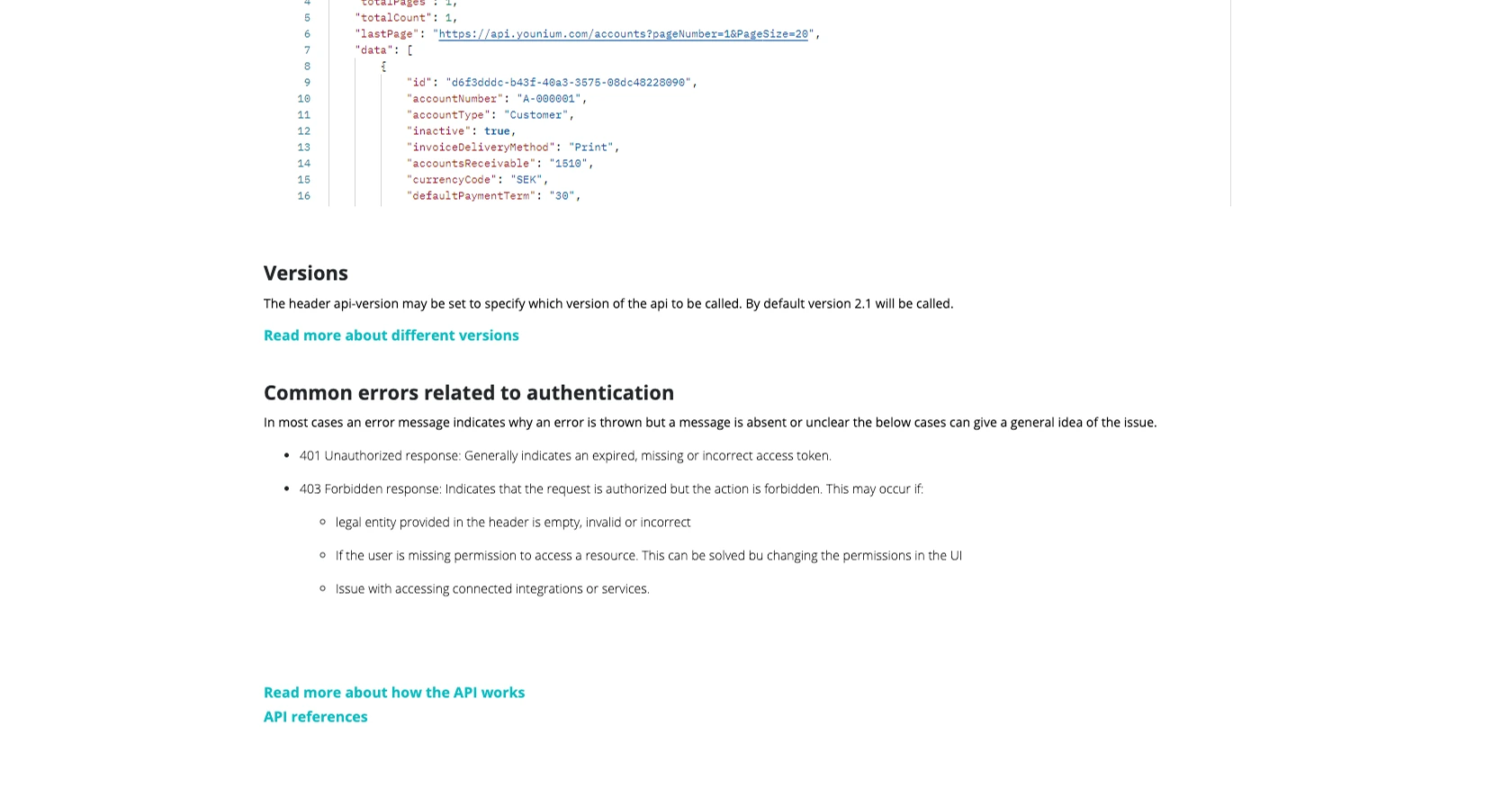
Conclusion and Best Practices for Younium API Integration
Integrating with the Younium API allows developers to efficiently manage and automate payment processes, providing seamless access to financial data. By following the steps outlined in this guide, you can successfully retrieve payment information using Python, enhancing your financial workflows and gaining valuable insights.
Best Practices for Secure and Efficient Younium API Usage
- Secure Storage of Credentials: Always store your Client ID, Secret Key, and JWT access tokens securely. Consider using environment variables or secure vaults to prevent unauthorized access.
- Handle Rate Limiting: Be mindful of the API's rate limits to avoid throttling. Implement retry mechanisms with exponential backoff to manage rate limit responses gracefully.
- Data Standardization: Ensure that the data retrieved from the Younium API is standardized and transformed as needed to integrate smoothly with your existing systems.
Streamline Your Integrations with Endgrate
While integrating with the Younium API can be straightforward, managing multiple integrations can become complex and time-consuming. Endgrate offers a unified API solution that simplifies the integration process, allowing you to focus on your core product development.
With Endgrate, you can build once for each use case and leverage an intuitive integration experience for your customers. Save time and resources by outsourcing your integrations and ensuring a seamless connection with platforms like Younium.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate today.
Read More
Ready to get started?