Using the Zoho Books API to Create or Update Purchase Orders in PHP
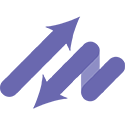
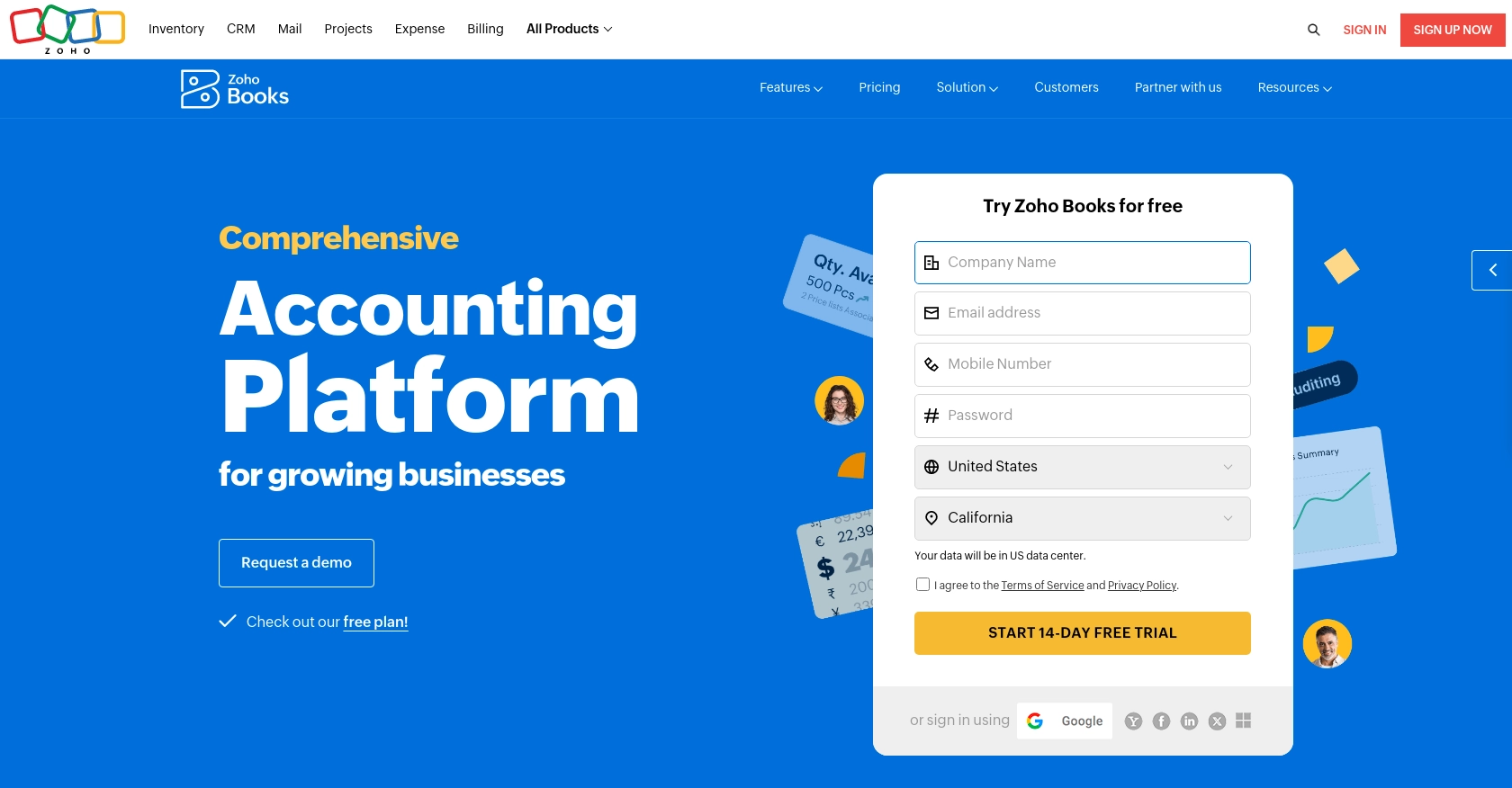
Introduction to Zoho Books API for Purchase Orders
Zoho Books is a comprehensive cloud-based accounting software designed to streamline financial operations for businesses. It offers a wide range of features, including invoicing, expense tracking, and inventory management, making it a popular choice for businesses looking to automate their accounting processes.
Integrating with the Zoho Books API allows developers to automate and manage purchase orders efficiently. By leveraging this API, developers can create or update purchase orders directly from their applications, ensuring seamless communication between systems. For example, a developer might want to automate the creation of purchase orders based on inventory levels, reducing manual entry and minimizing errors.
This article will guide you through using the Zoho Books API to create or update purchase orders using PHP, providing a step-by-step approach to streamline your business operations.
Setting Up Your Zoho Books Test/Sandbox Account for API Integration
Before you can start integrating with the Zoho Books API to create or update purchase orders, you'll need to set up a test or sandbox account. This allows you to safely test your API calls without affecting your live data.
Creating a Zoho Books Account
If you don't already have a Zoho Books account, you can sign up for a free trial on the Zoho Books website. Follow the instructions to create your account. Once your account is set up, you'll be able to access the Zoho Books dashboard.
Registering Your Application for OAuth Authentication
Zoho Books uses OAuth 2.0 for authentication, which requires you to register your application to obtain the necessary credentials.
- Go to the Zoho Developer Console.
- Click on Add Client ID to register your application.
- Fill in the required details, such as the client name, homepage URL, and authorized redirect URIs.
- Upon successful registration, you will receive a Client ID and Client Secret. Keep these credentials secure as they are essential for API authentication.
Generating the OAuth Access Token
To interact with the Zoho Books API, you need to generate an OAuth access token using your client credentials.
- Redirect users to the following authorization URL to obtain a grant token:
- After the user authorizes your application, Zoho will redirect to your specified redirect URI with a code parameter.
- Exchange this code for an access token by making a POST request to:
- The response will include an access_token and a refresh_token. Use the access token for API calls.
https://accounts.zoho.com/oauth/v2/auth?scope=ZohoBooks.purchaseorders.CREATE,ZohoBooks.purchaseorders.UPDATE&client_id=YOUR_CLIENT_ID&response_type=code&redirect_uri=YOUR_REDIRECT_URI
https://accounts.zoho.com/oauth/v2/token?code=YOUR_CODE&client_id=YOUR_CLIENT_ID&client_secret=YOUR_CLIENT_SECRET&redirect_uri=YOUR_REDIRECT_URI&grant_type=authorization_code
Setting Up Your Organization ID
Every API request requires an organization ID to identify your business within Zoho Books. You can find your organization ID by navigating to the Manage Organizations section in the Zoho Books admin console.
Alternatively, you can retrieve it programmatically using the following API call:
$ curl -X GET 'https://www.zohoapis.com/books/v3/organizations' -H 'Authorization: Zoho-oauthtoken YOUR_ACCESS_TOKEN'
With your test account and OAuth setup complete, you're ready to start integrating with the Zoho Books API to create or update purchase orders in PHP.
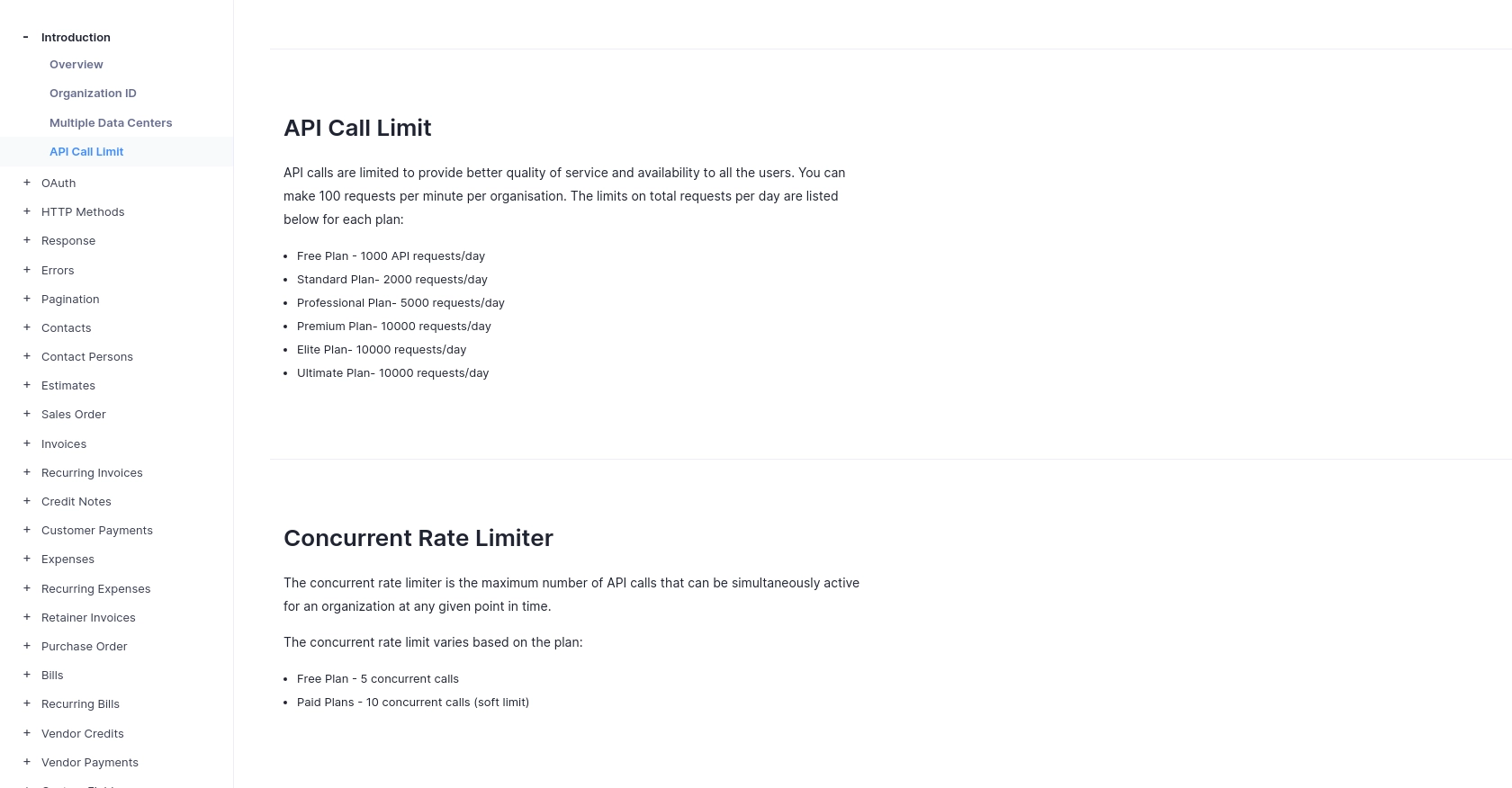
sbb-itb-96038d7
Making API Calls to Zoho Books for Creating or Updating Purchase Orders in PHP
To interact with the Zoho Books API for creating or updating purchase orders, you'll need to use PHP. This section will guide you through the process, including setting up your environment, writing the code, and handling responses.
Setting Up Your PHP Environment for Zoho Books API Integration
Before making API calls, ensure your PHP environment is ready. You'll need:
- PHP 7.4 or later
- cURL extension enabled
To install the cURL extension, you can use the following command:
sudo apt-get install php-curl
Creating a Purchase Order with Zoho Books API in PHP
Here's how to create a purchase order using the Zoho Books API:
$curl = curl_init();
$data = [
"vendor_id" => "460000000026049",
"currency_id" => "460000000000099",
"line_items" => [
[
"item_id" => "460000000027009",
"rate" => 112,
"quantity" => 1
]
]
];
curl_setopt_array($curl, [
CURLOPT_URL => "https://www.zohoapis.com/books/v3/purchaseorders?organization_id=YOUR_ORG_ID",
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => "",
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 30,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => "POST",
CURLOPT_POSTFIELDS => json_encode($data),
CURLOPT_HTTPHEADER => [
"Authorization: Zoho-oauthtoken YOUR_ACCESS_TOKEN",
"Content-Type: application/json"
],
]);
$response = curl_exec($curl);
$err = curl_error($curl);
curl_close($curl);
if ($err) {
echo "cURL Error #:" . $err;
} else {
echo $response;
}
Replace YOUR_ORG_ID
and YOUR_ACCESS_TOKEN
with your actual organization ID and access token.
Updating a Purchase Order with Zoho Books API in PHP
To update an existing purchase order, modify the request method to PUT and include the purchase order ID:
$curl = curl_init();
$data = [
"vendor_id" => "460000000026049",
"currency_id" => "460000000000099",
"line_items" => [
[
"line_item_id" => "460000000074009",
"rate" => 120,
"quantity" => 2
]
]
];
curl_setopt_array($curl, [
CURLOPT_URL => "https://www.zohoapis.com/books/v3/purchaseorders/{purchase_order_id}?organization_id=YOUR_ORG_ID",
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => "",
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 30,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => "PUT",
CURLOPT_POSTFIELDS => json_encode($data),
CURLOPT_HTTPHEADER => [
"Authorization: Zoho-oauthtoken YOUR_ACCESS_TOKEN",
"Content-Type: application/json"
],
]);
$response = curl_exec($curl);
$err = curl_error($curl);
curl_close($curl);
if ($err) {
echo "cURL Error #:" . $err;
} else {
echo $response;
}
Ensure you replace {purchase_order_id}
with the actual ID of the purchase order you wish to update.
Handling API Responses and Errors
After making an API call, it's crucial to handle the response properly:
- Check for HTTP status codes. A 200 or 201 indicates success, while 4xx and 5xx indicate errors.
- Parse the JSON response to extract useful information.
For error handling, refer to the Zoho Books API error documentation for detailed error codes and messages.
Verifying API Call Success in Zoho Books
To verify the success of your API calls, log in to your Zoho Books account and check the purchase orders section. Newly created or updated purchase orders should reflect the changes made via the API.
For more details on API limits, refer to the API call limit documentation.
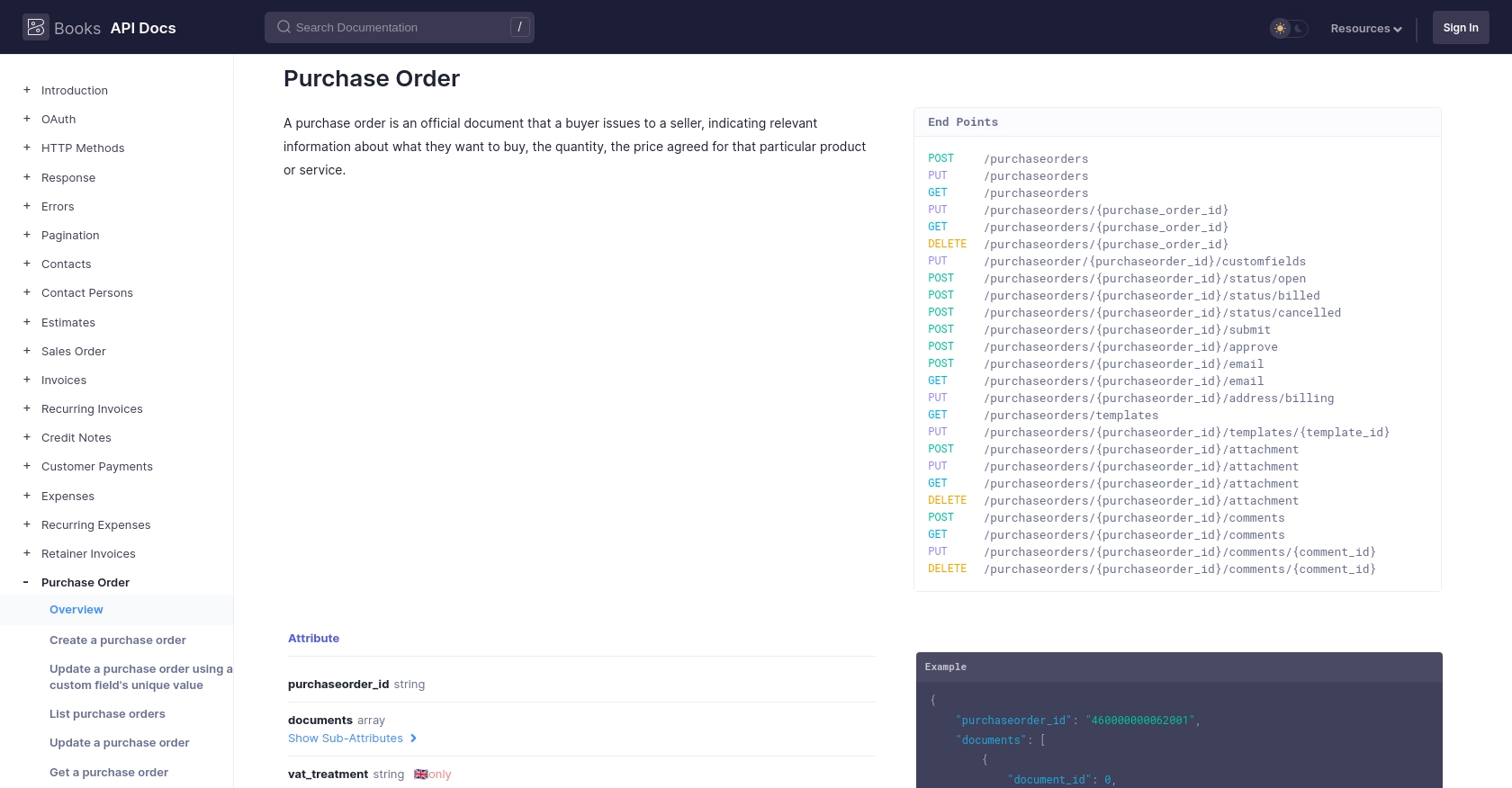
Conclusion and Best Practices for Zoho Books API Integration in PHP
Integrating with the Zoho Books API to create or update purchase orders in PHP can significantly enhance your business operations by automating routine tasks and reducing manual errors. By following the steps outlined in this guide, you can efficiently manage purchase orders directly from your application, ensuring seamless communication between systems.
Best Practices for Secure and Efficient Zoho Books API Integration
- Securely Store Credentials: Always store your OAuth credentials, such as the Client ID and Client Secret, securely. Avoid hardcoding them in your source code.
- Handle Rate Limiting: Zoho Books API has rate limits, with a maximum of 100 requests per minute per organization. Implement logic to handle rate limits gracefully to avoid disruptions. For more details, refer to the API call limit documentation.
- Manage Access Tokens: Access tokens have limited validity. Use refresh tokens to obtain new access tokens when needed, ensuring uninterrupted API access.
- Standardize Data Fields: Ensure that data fields are standardized across your application to maintain consistency and avoid errors during API interactions.
- Implement Error Handling: Properly handle API errors by checking HTTP status codes and parsing error messages. Refer to the Zoho Books API error documentation for guidance.
Streamline Your Integration Process with Endgrate
While integrating with Zoho Books API can be straightforward, managing multiple integrations can become complex. Endgrate offers a unified API solution that simplifies the integration process, allowing you to focus on your core product. With Endgrate, you can build once for each use case and leverage an intuitive integration experience for your customers.
Explore how Endgrate can help you save time and resources by outsourcing integrations. Visit Endgrate to learn more about our solutions and how we can support your business needs.
Read More
- https://endgrate.com/provider/zohobooks
- https://www.zoho.com/books/api/v3/introduction/#api-call-limit
- https://www.zoho.com/books/api/v3/oauth/#overview
- https://www.zoho.com/books/api/v3/errors/#overview
- https://www.zoho.com/books/api/v3/pagination/#overview
- https://www.zoho.com/books/api/v3/purchase-order/#overview
Ready to get started?