Using the Zoho CRM API to Get Users in PHP
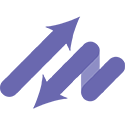
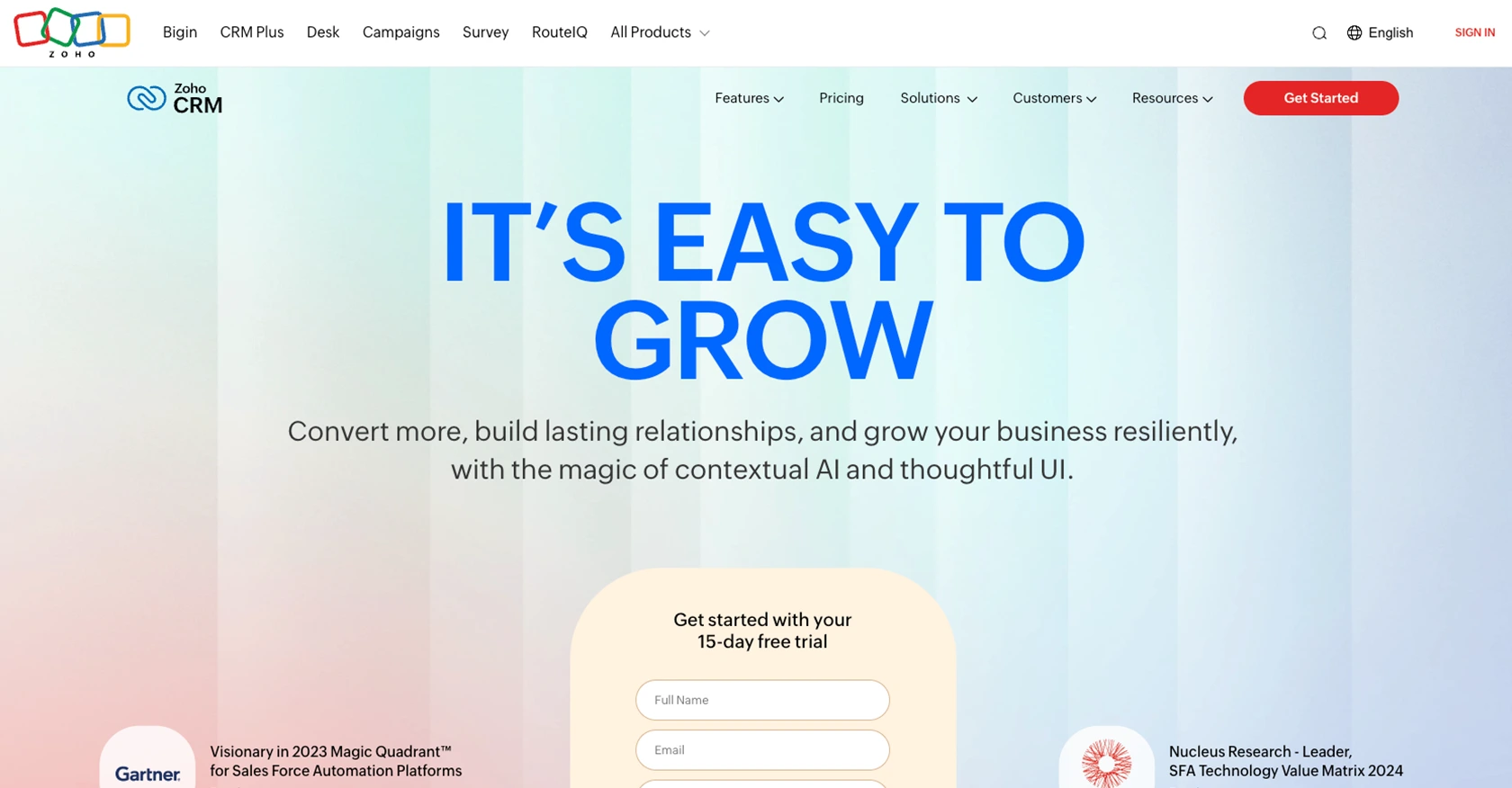
Introduction to Zoho CRM
Zoho CRM is a comprehensive customer relationship management platform that empowers businesses to manage their sales, marketing, and support in a single system. Known for its flexibility and scalability, Zoho CRM is a popular choice for organizations looking to enhance their customer interactions and streamline their business processes.
Developers often integrate with Zoho CRM's API to automate and enhance various CRM functionalities. For example, using the Zoho CRM API, a developer can retrieve user data to analyze team performance or manage user roles programmatically. This capability is particularly useful for businesses that need to synchronize user information across multiple platforms or applications.
Setting Up Your Zoho CRM Test/Sandbox Account
Before you can start integrating with the Zoho CRM API, you need to set up a test or sandbox account. This allows you to safely experiment with API calls without affecting live data.
Creating a Zoho CRM Account
If you don't already have a Zoho CRM account, you can sign up for a free trial on the Zoho CRM website. Follow the instructions to complete the registration process. Once your account is created, log in to access the CRM dashboard.
Registering a Zoho CRM App for OAuth Authentication
Zoho CRM uses OAuth 2.0 for authentication, which requires you to register your application to obtain the necessary credentials. Follow these steps to register your app:
- Navigate to the Zoho Developer Console.
- Select "Add Client" and choose the client type that suits your application (e.g., Web Based, Self Client).
- Fill in the required details:
- Client Name: Enter a name for your application.
- Homepage URL: Provide the URL of your website.
- Authorized Redirect URIs: Enter a valid URL where Zoho will redirect after authentication.
- Click "Create" to register your application.
Upon successful registration, you will receive a Client ID and Client Secret. Keep these credentials secure as they are essential for authenticating API requests.
Generating OAuth Tokens
To interact with the Zoho CRM API, you need to generate access and refresh tokens using the OAuth 2.0 protocol. Follow these steps:
- Direct users to the Zoho authorization URL to obtain an authorization code:
https://accounts.zoho.com/oauth/v2/auth?scope=ZohoCRM.users.ALL&client_id=YOUR_CLIENT_ID&response_type=code&redirect_uri=YOUR_REDIRECT_URI
- Exchange the authorization code for access and refresh tokens by making a POST request:
POST https://accounts.zoho.com/oauth/v2/token Content-Type: application/x-www-form-urlencoded client_id=YOUR_CLIENT_ID &client_secret=YOUR_CLIENT_SECRET &grant_type=authorization_code &code=AUTHORIZATION_CODE &redirect_uri=YOUR_REDIRECT_URI
Store the access and refresh tokens securely. The access token is valid for an hour, while the refresh token can be used to obtain new access tokens.
For more detailed information on OAuth setup, refer to the Zoho CRM OAuth documentation.
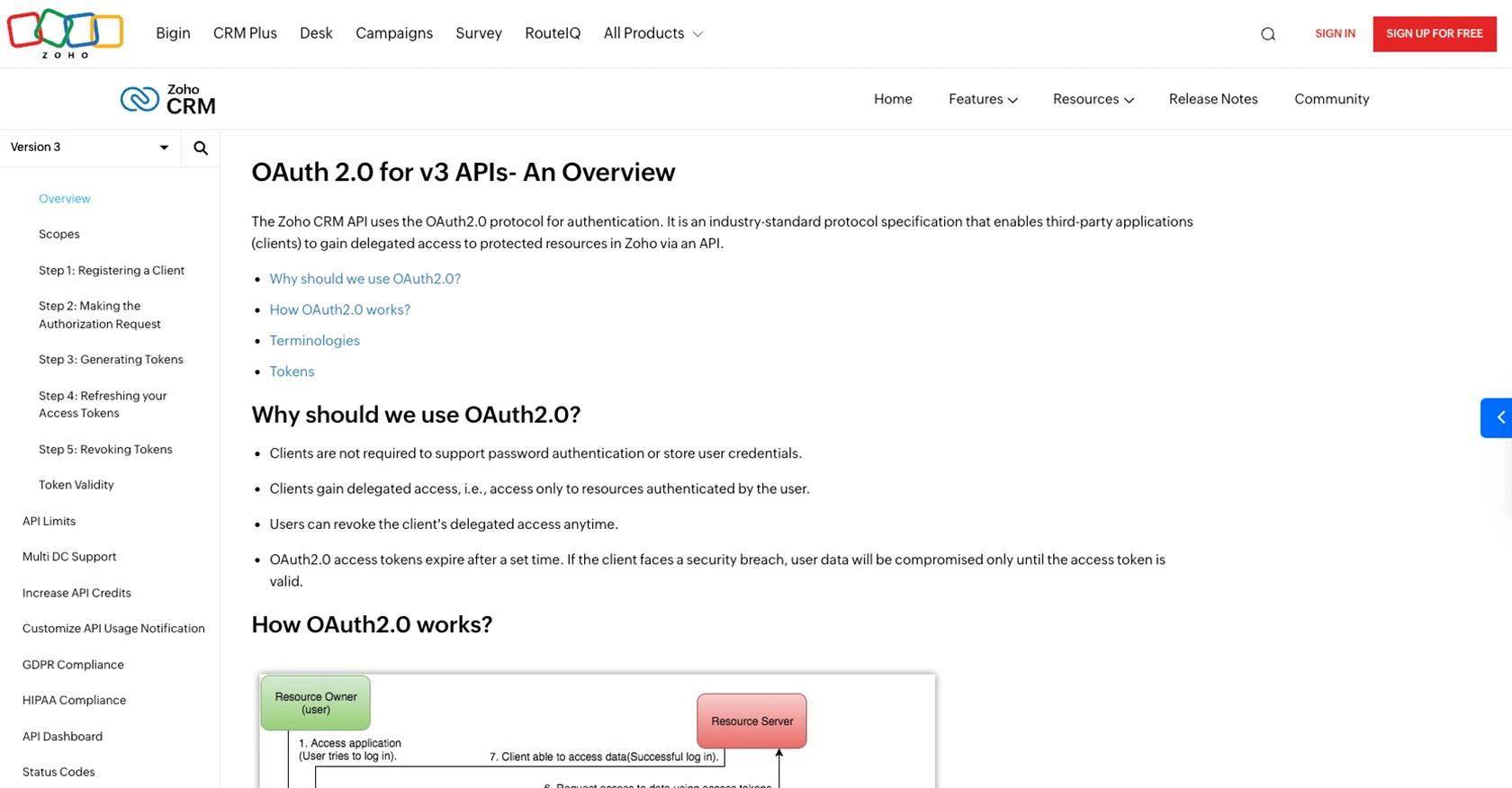
sbb-itb-96038d7
Making API Calls to Retrieve Users from Zoho CRM Using PHP
To interact with the Zoho CRM API and retrieve user data, you'll need to make HTTP requests using PHP. This section will guide you through the necessary steps, including setting up your environment, writing the code, and handling responses.
Setting Up Your PHP Environment for Zoho CRM API Integration
Before making API calls, ensure your PHP environment is properly configured. You'll need:
- PHP 7.4 or later
- cURL extension enabled
- Composer for managing dependencies
Install the guzzlehttp/guzzle
package using Composer to simplify HTTP requests:
composer require guzzlehttp/guzzle
Writing PHP Code to Retrieve Users from Zoho CRM
With your environment set up, you can now write the PHP code to make an API call to Zoho CRM and retrieve user data. Create a file named get_zoho_users.php
and add the following code:
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$accessToken = 'YOUR_ACCESS_TOKEN';
$response = $client->request('GET', 'https://www.zohoapis.com/crm/v3/users', [
'headers' => [
'Authorization' => 'Zoho-oauthtoken ' . $accessToken
]
]);
$data = json_decode($response->getBody(), true);
foreach ($data['users'] as $user) {
echo 'Name: ' . $user['full_name'] . ', Email: ' . $user['email'] . "\n";
}
Replace YOUR_ACCESS_TOKEN
with the access token you obtained during the OAuth setup.
Understanding the API Response and Handling Errors
The API response will contain user data in JSON format. The code above decodes this JSON and prints each user's name and email. If the request fails, handle errors gracefully by checking the response status code:
if ($response->getStatusCode() !== 200) {
echo 'Error: ' . $response->getReasonPhrase();
}
Refer to the Zoho CRM API status codes documentation for more details on handling specific error codes.
Verifying Successful API Calls in Zoho CRM
To ensure your API call was successful, log in to your Zoho CRM account and verify that the retrieved user data matches the records in your CRM. This step is crucial for confirming that your integration is working as expected.
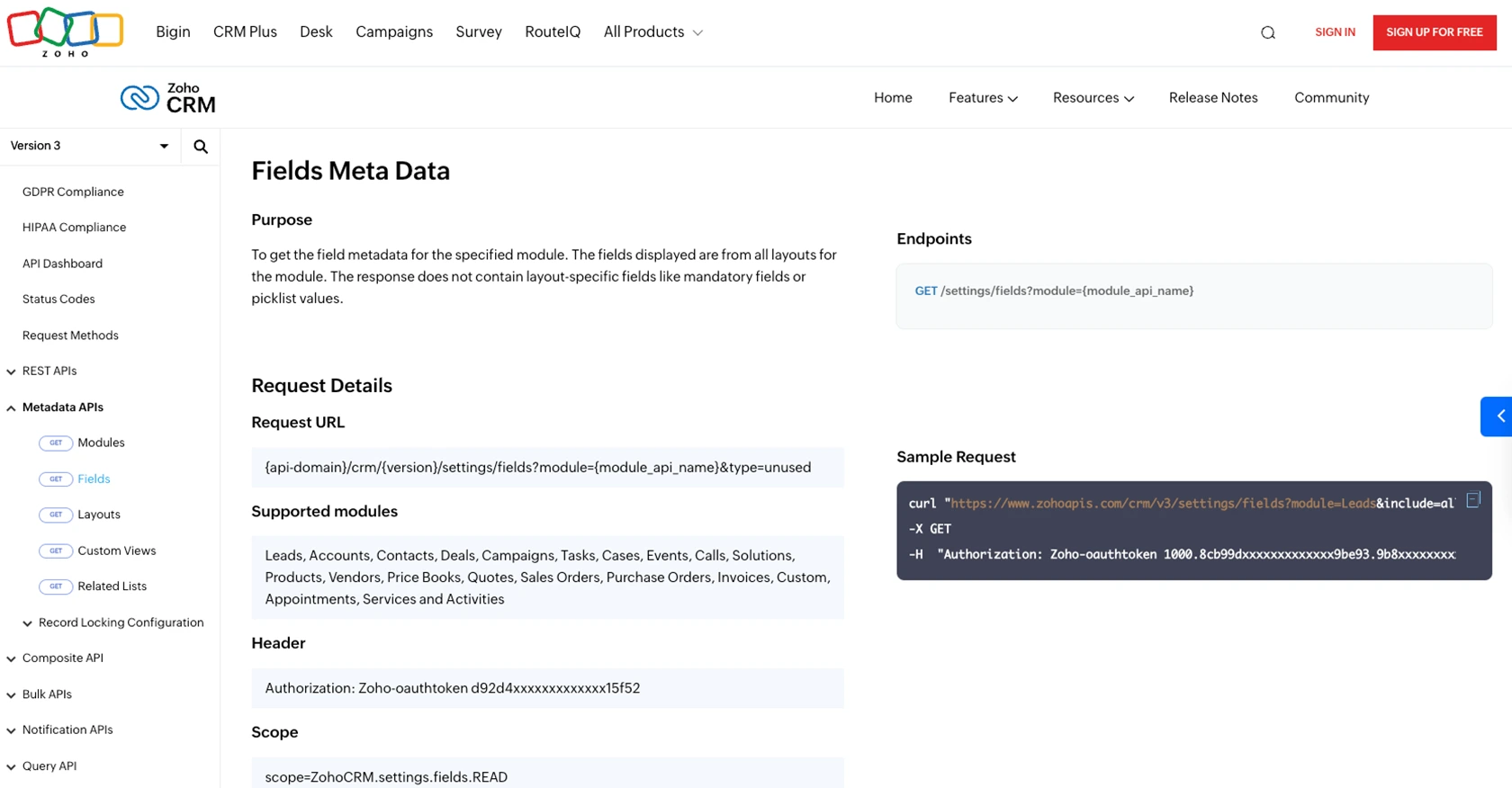
Conclusion and Best Practices for Zoho CRM API Integration
Integrating with the Zoho CRM API using PHP offers a powerful way to automate and enhance your CRM functionalities. By following the steps outlined in this guide, you can efficiently retrieve user data and manage CRM operations programmatically.
Best Practices for Secure and Efficient Zoho CRM API Usage
- Secure Storage of Credentials: Always store your OAuth credentials, including the Client ID, Client Secret, and tokens, securely. Avoid exposing them in public repositories or client-side code.
- Handling Rate Limits: Zoho CRM imposes API rate limits based on your subscription plan. Monitor your API usage and implement logic to handle rate limit errors gracefully. Refer to the Zoho CRM API limits documentation for more details.
- Data Standardization: Ensure that data retrieved from Zoho CRM is standardized and transformed as needed to maintain consistency across your applications.
- Error Handling: Implement robust error handling by checking response status codes and handling exceptions. This will help you manage unexpected issues effectively.
Streamlining Integrations with Endgrate
If managing multiple integrations becomes overwhelming, consider using Endgrate to simplify the process. Endgrate provides a unified API endpoint that connects to multiple platforms, including Zoho CRM, allowing you to focus on your core product while outsourcing integration complexities.
With Endgrate, you can build once for each use case and enjoy an intuitive integration experience for your customers. Visit Endgrate to learn more about how it can enhance your integration strategy.
Read More
- https://endgrate.com/provider/zohocrm
- https://www.zoho.com/crm/developer/docs/api/v3/oauth-overview.html
- https://www.zoho.com/crm/developer/docs/api/v3/scopes.html
- https://www.zoho.com/crm/developer/docs/api/v3/register-client.html
- https://www.zoho.com/crm/developer/docs/api/v3/api-limits.html
- https://www.zoho.com/crm/developer/docs/api/v3/status-codes.html
- https://www.zoho.com/crm/developer/docs/api/v3/field-meta.html
- https://www.zoho.com/crm/developer/docs/api/v3/get-users.html
Ready to get started?