How to Create Deals with the PipelineCRM API in PHP
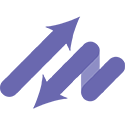
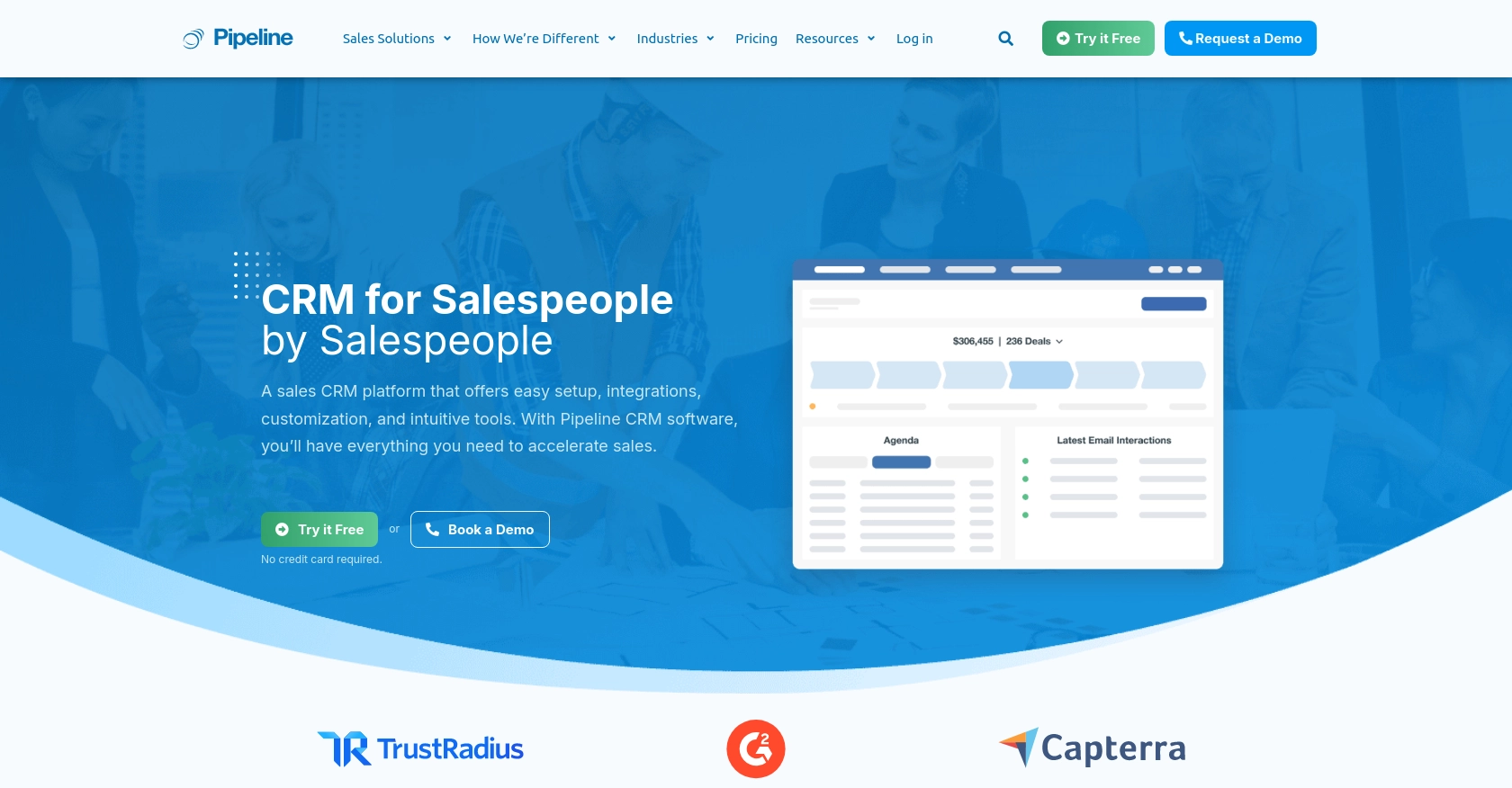
Introduction to PipelineCRM
PipelineCRM is a robust customer relationship management platform designed to help businesses streamline their sales processes and manage customer interactions effectively. With features like deal tracking, contact management, and sales reporting, PipelineCRM is a valuable tool for sales teams looking to enhance their productivity and close deals faster.
Integrating with the PipelineCRM API allows developers to automate and optimize sales workflows by programmatically creating and managing deals. For example, a developer might use the PipelineCRM API to automatically create new deals when a potential customer fills out a form on the company's website, ensuring that no sales opportunity is missed.
Setting Up Your PipelineCRM Test Account
Before you can start creating deals using the PipelineCRM API, you'll need to set up a test account. This will allow you to safely experiment with the API without affecting any live data.
Creating a PipelineCRM Account
If you don't already have a PipelineCRM account, you can sign up for a free trial on their website. This trial will give you access to the necessary features to test the API integration.
- Visit the PipelineCRM website and click on the "Sign Up" button.
- Fill out the registration form with your details and submit it to create your account.
- Once your account is created, log in to access the PipelineCRM dashboard.
Generating Your API Key for PipelineCRM
PipelineCRM uses API key-based authentication to authorize requests. Follow these steps to generate your API key:
- Log in to your PipelineCRM account and navigate to the "Settings" section.
- Under "API Access," click on "Generate API Key."
- Copy the API key provided and store it securely, as you will need it to authenticate your API requests.
For more detailed information on authentication, refer to the PipelineCRM API documentation.
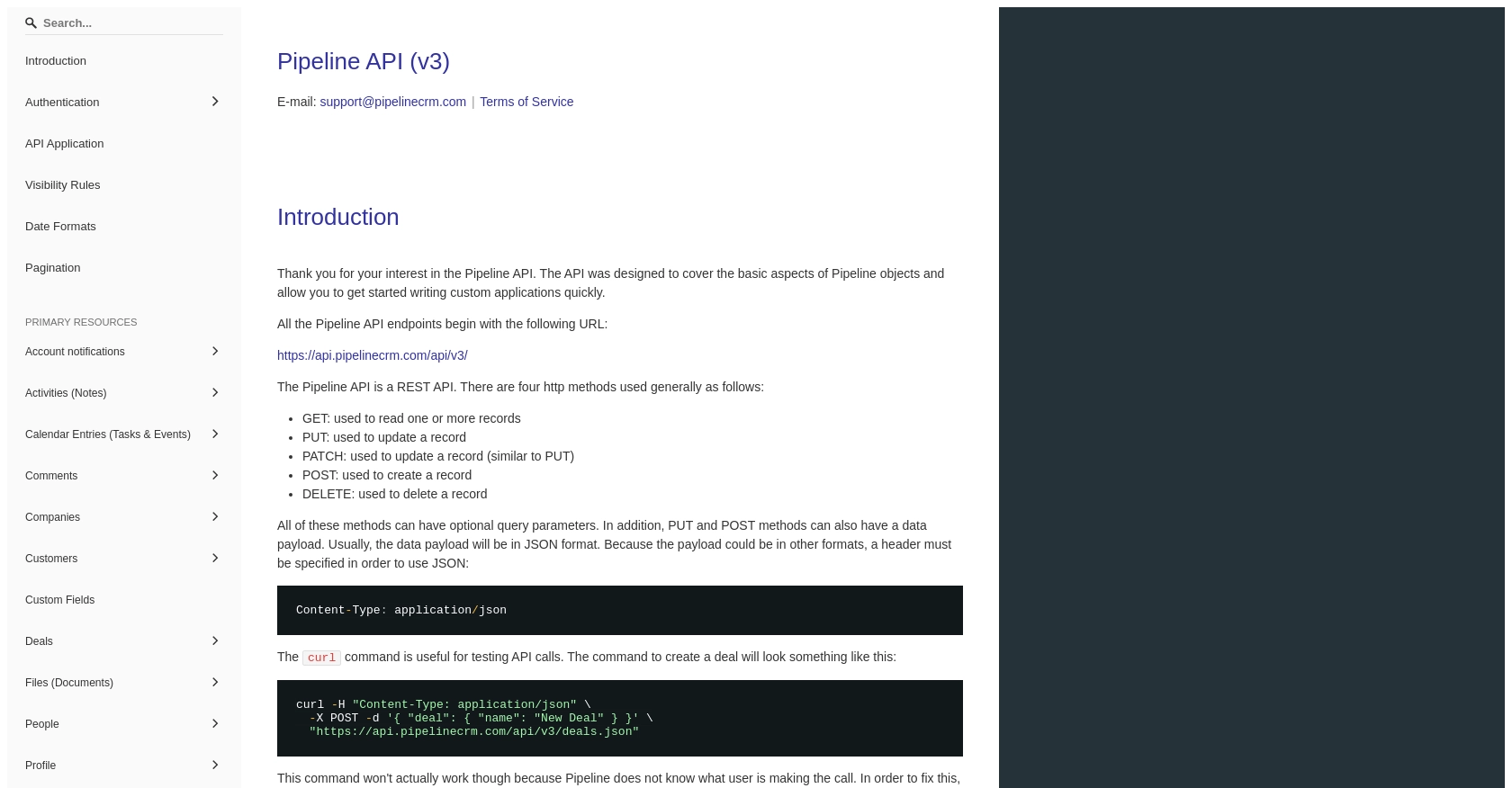
sbb-itb-96038d7
Making API Calls to Create Deals in PipelineCRM Using PHP
To interact with the PipelineCRM API and create deals programmatically, you'll need to use PHP, a popular server-side scripting language. This section will guide you through the necessary steps, including setting up your environment, writing the PHP code, and handling responses and errors effectively.
Setting Up Your PHP Environment for PipelineCRM API Integration
Before you begin coding, ensure that your development environment is ready:
- Install PHP version 7.4 or higher on your machine.
- Ensure you have access to a code editor like VSCode or PHPStorm.
- Install the
cURL
extension for PHP, which is essential for making HTTP requests.
Writing PHP Code to Create Deals with PipelineCRM API
Once your environment is set up, you can start writing the PHP code to create deals in PipelineCRM. Below is a sample script:
<?php
// Your PipelineCRM API key
$apiKey = 'Your_API_Key';
// API endpoint for creating deals
$url = 'https://api.pipelinecrm.com/api/v3/deals';
// Deal data to be sent in the request
$data = [
'name' => 'New Deal',
'value' => 1000,
'currency' => 'USD',
'stage_id' => 1
];
// Initialize cURL session
$ch = curl_init($url);
// Set cURL options
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_HTTPHEADER, [
'Content-Type: application/json',
'Authorization: Bearer ' . $apiKey
]);
curl_setopt($ch, CURLOPT_POST, true);
curl_setopt($ch, CURLOPT_POSTFIELDS, json_encode($data));
// Execute cURL request
$response = curl_exec($ch);
// Check for errors
if (curl_errno($ch)) {
echo 'Request Error:' . curl_error($ch);
} else {
// Decode the response
$responseData = json_decode($response, true);
if (isset($responseData['id'])) {
echo 'Deal Created Successfully. Deal ID: ' . $responseData['id'];
} else {
echo 'Failed to Create Deal. Response: ' . $response;
}
}
// Close cURL session
curl_close($ch);
?>
Replace Your_API_Key
with the API key you generated earlier. This script initializes a cURL session, sets the necessary headers, and sends a POST request to the PipelineCRM API to create a new deal.
Verifying API Call Success and Handling Errors
After executing the script, you should verify that the deal was created successfully:
- Check the response for a deal ID, which indicates success.
- Log in to your PipelineCRM dashboard and confirm that the new deal appears in the list.
If there are errors, the script will output the error message. Common issues include incorrect API keys or malformed request data.
For more information on error codes and handling, refer to the PipelineCRM API documentation.
Conclusion and Best Practices for Integrating with PipelineCRM API Using PHP
Integrating with the PipelineCRM API using PHP allows developers to automate sales processes and enhance productivity by programmatically managing deals. By following the steps outlined in this guide, you can efficiently create deals and ensure seamless integration with your sales workflows.
Best Practices for Secure and Efficient API Integration with PipelineCRM
- Securely Store API Keys: Always store your API keys securely and avoid hardcoding them in your scripts. Consider using environment variables or secure vaults to manage sensitive information.
- Handle Rate Limiting: Be mindful of any rate limits imposed by the PipelineCRM API. Implement retry logic and exponential backoff strategies to handle rate limit errors gracefully.
- Standardize Data Fields: Ensure that the data fields you send to the API are standardized and validated to prevent errors and maintain data integrity.
- Error Handling: Implement robust error handling to capture and log errors effectively. This will help in diagnosing issues and improving the reliability of your integration.
Streamline Your Integrations with Endgrate
While integrating with PipelineCRM API can significantly enhance your sales processes, managing multiple integrations can become complex and time-consuming. Endgrate offers a unified API solution that simplifies the integration process across various platforms, including PipelineCRM.
By leveraging Endgrate, you can save time and resources, allowing your team to focus on core product development. Build once for each use case and enjoy an intuitive integration experience for your customers. Explore how Endgrate can transform your integration strategy by visiting Endgrate today.
Read More
Ready to get started?