How to Create Opportunities with the Outreach API in Javascript
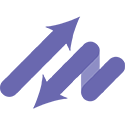
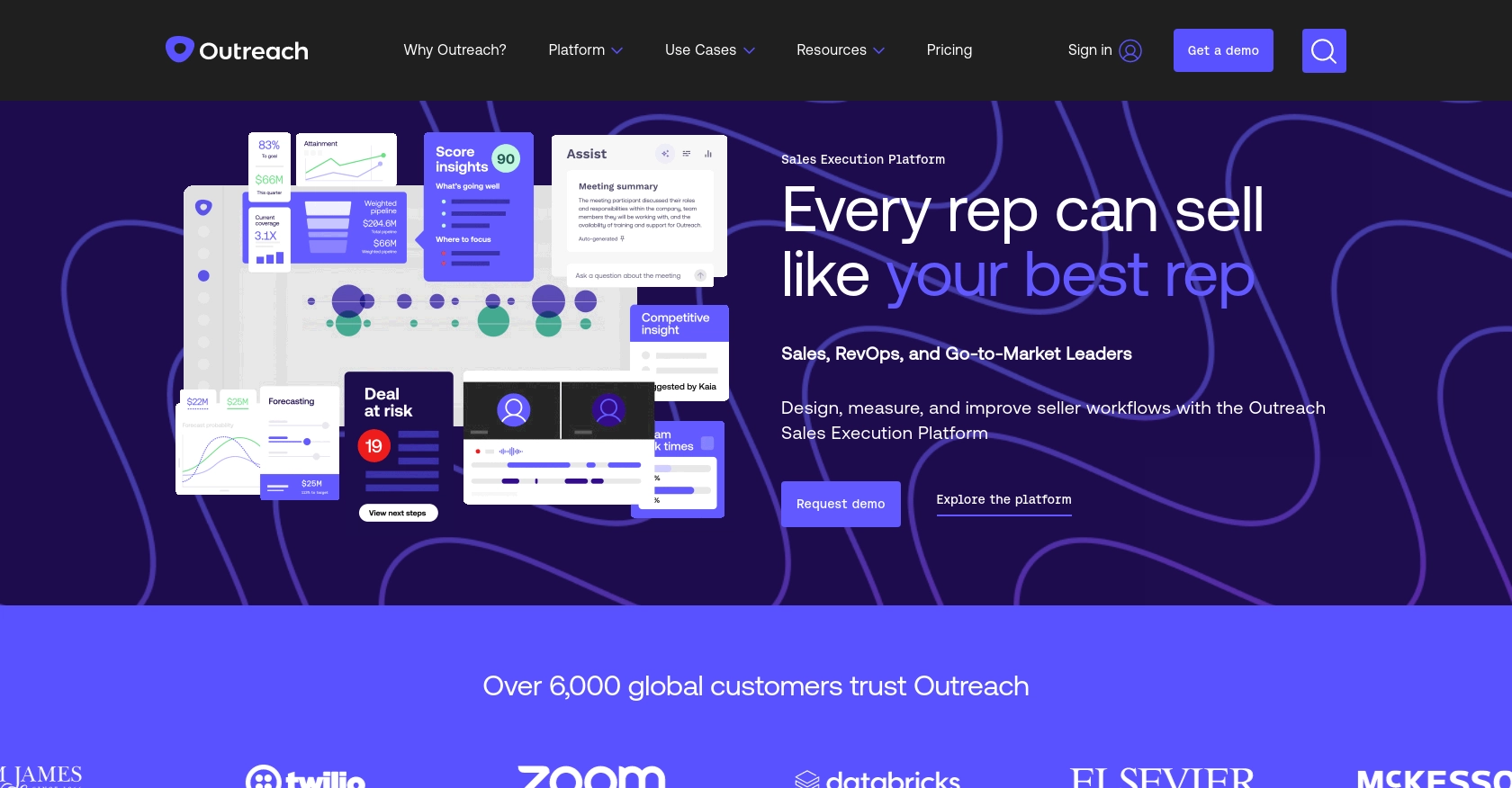
Introduction to Outreach API for Opportunity Management
Outreach is a powerful sales engagement platform that helps businesses streamline their sales processes and improve productivity. With its robust set of tools, Outreach enables sales teams to manage customer interactions, automate workflows, and track performance metrics effectively.
Integrating with the Outreach API allows developers to enhance their applications by automating sales processes and managing opportunities seamlessly. For example, a developer might use the Outreach API to create new sales opportunities directly from their application, ensuring that sales teams can quickly act on potential deals without manual data entry.
This article will guide you through the process of creating opportunities using the Outreach API with JavaScript, providing step-by-step instructions and code examples to help you get started efficiently.
Setting Up Your Outreach Test or Sandbox Account
Before you can start creating opportunities with the Outreach API, you'll need to set up a test or sandbox account. This allows you to safely experiment with the API without affecting live data. Follow these steps to get started:
Create an Outreach Developer Account
If you don't already have an Outreach account, you can sign up for a developer account on the Outreach Developer Portal. This account will provide you with access to the necessary tools and resources for API integration.
Configure OAuth for API Access
The Outreach API uses OAuth 2.0 for authentication, which requires you to create an app within your developer account. Follow these steps to configure OAuth:
- Log in to your Outreach developer account.
- Navigate to the "My Apps" section and click on "Create App."
- Fill in the required details, such as the app name and description.
- Go to the "API Access" tab and configure your OAuth settings:
- Specify one or more redirect URIs.
- Select the necessary OAuth scopes for your application.
- Save your changes to generate your client ID and client secret. Note that these credentials will only be displayed once, so make sure to store them securely.
For more detailed information on setting up OAuth, refer to the Outreach OAuth Documentation.
Generate OAuth Tokens
Once your app is set up, you'll need to generate OAuth tokens to authenticate API requests:
- Redirect users to the following URL to obtain an authorization code:
- After user authorization, use the authorization code to request an access token:
- Store the access token securely and use it in your API requests. Remember that access tokens are short-lived and need to be refreshed periodically using the refresh token provided in the response.
https://api.outreach.io/oauth/authorize?client_id=<Your_Client_ID>&redirect_uri=<Your_Redirect_URI>&response_type=code&scope=<Your_Scopes>
curl https://api.outreach.io/oauth/token \
-X POST \
-d client_id=<Your_Client_ID> \
-d client_secret=<Your_Client_Secret> \
-d redirect_uri=<Your_Redirect_URI> \
-d grant_type=authorization_code \
-d code=<Authorization_Code>
For more information on requesting and refreshing tokens, visit the Outreach Getting Started Guide.
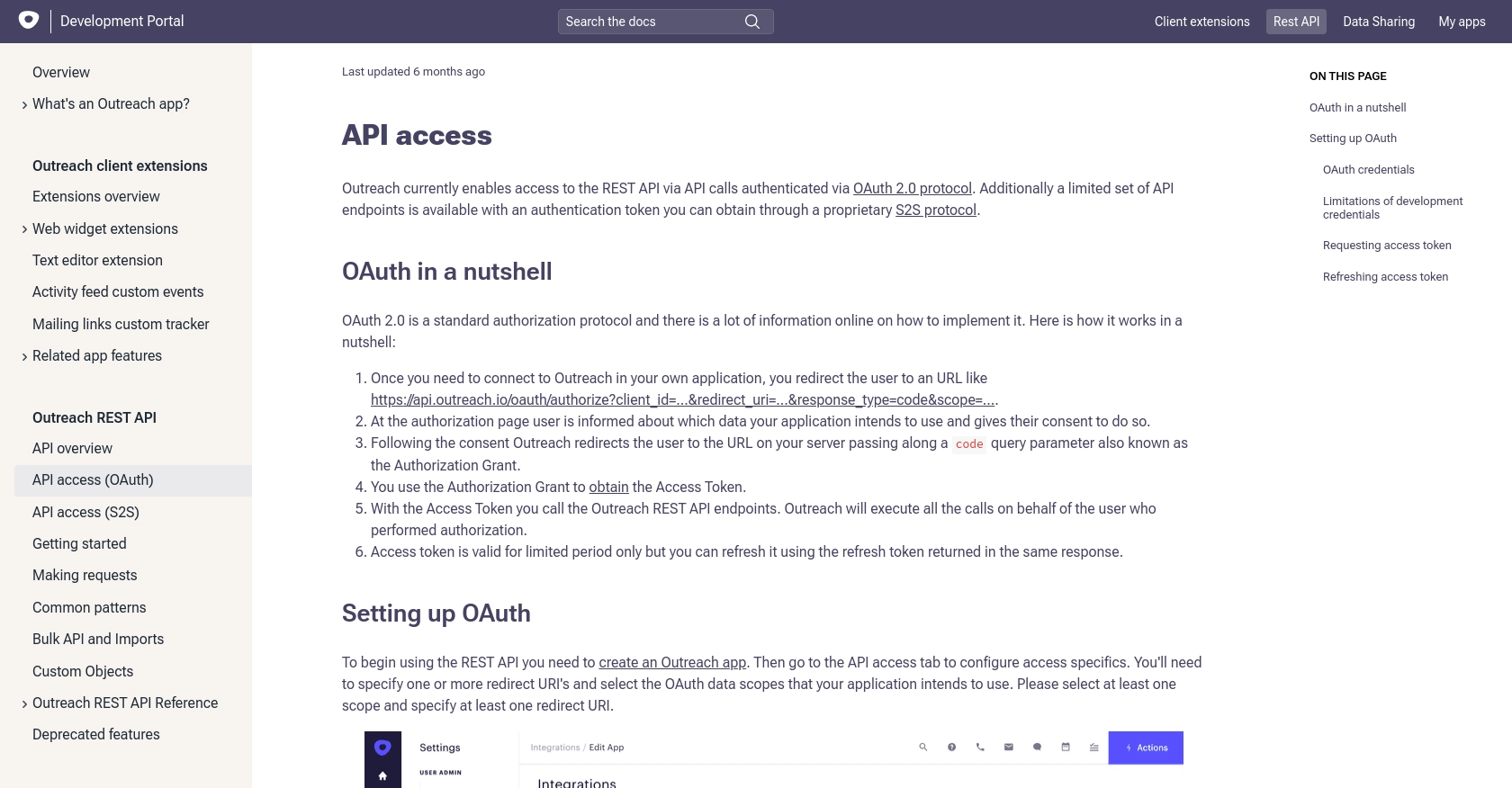
sbb-itb-96038d7
Making API Calls to Create Opportunities with Outreach in JavaScript
To create opportunities using the Outreach API in JavaScript, you'll need to set up your development environment and make HTTP requests to the API endpoints. This section will guide you through the necessary steps, including setting up your JavaScript environment, writing the code to make API calls, and handling responses and errors.
Setting Up Your JavaScript Environment for Outreach API Integration
Before you begin coding, ensure you have the following prerequisites installed on your machine:
- Node.js (version 14 or later)
- NPM (Node Package Manager)
Once you have Node.js and NPM installed, create a new project directory and initialize it with the following command:
npm init -y
Next, install the Axios library to handle HTTP requests:
npm install axios
Writing JavaScript Code to Create Opportunities with Outreach API
Now that your environment is set up, you can write the JavaScript code to create opportunities using the Outreach API. Create a new file named createOpportunity.js
and add the following code:
const axios = require('axios');
// Set the API endpoint and headers
const endpoint = 'https://api.outreach.io/api/v2/opportunities';
const headers = {
'Authorization': 'Bearer Your_Access_Token',
'Content-Type': 'application/vnd.api+json'
};
// Define the opportunity data
const opportunityData = {
data: {
type: 'opportunity',
attributes: {
name: 'New Sales Opportunity',
amount: 10000,
closeDate: '2023-12-31',
probability: 75
}
}
};
// Make a POST request to create a new opportunity
axios.post(endpoint, opportunityData, { headers })
.then(response => {
console.log('Opportunity Created Successfully:', response.data);
})
.catch(error => {
console.error('Error Creating Opportunity:', error.response.data);
});
Replace Your_Access_Token
with the access token you obtained during the OAuth setup. This code sets up the API endpoint, headers, and opportunity data, then makes a POST request to create a new opportunity.
Verifying Successful API Requests and Handling Errors
After running the code, you should see a success message in the console if the opportunity is created successfully. You can verify this by checking your Outreach sandbox account for the new opportunity.
In case of errors, the catch block will log the error details. Common error codes include:
- 401 Unauthorized: Check if your access token is valid and not expired.
- 403 Forbidden: Ensure your OAuth scopes are correctly set.
- 429 Too Many Requests: You have exceeded the rate limit of 10,000 requests per hour. Wait for the rate limit to reset before making more requests.
For more information on error handling, refer to the Outreach API Reference.
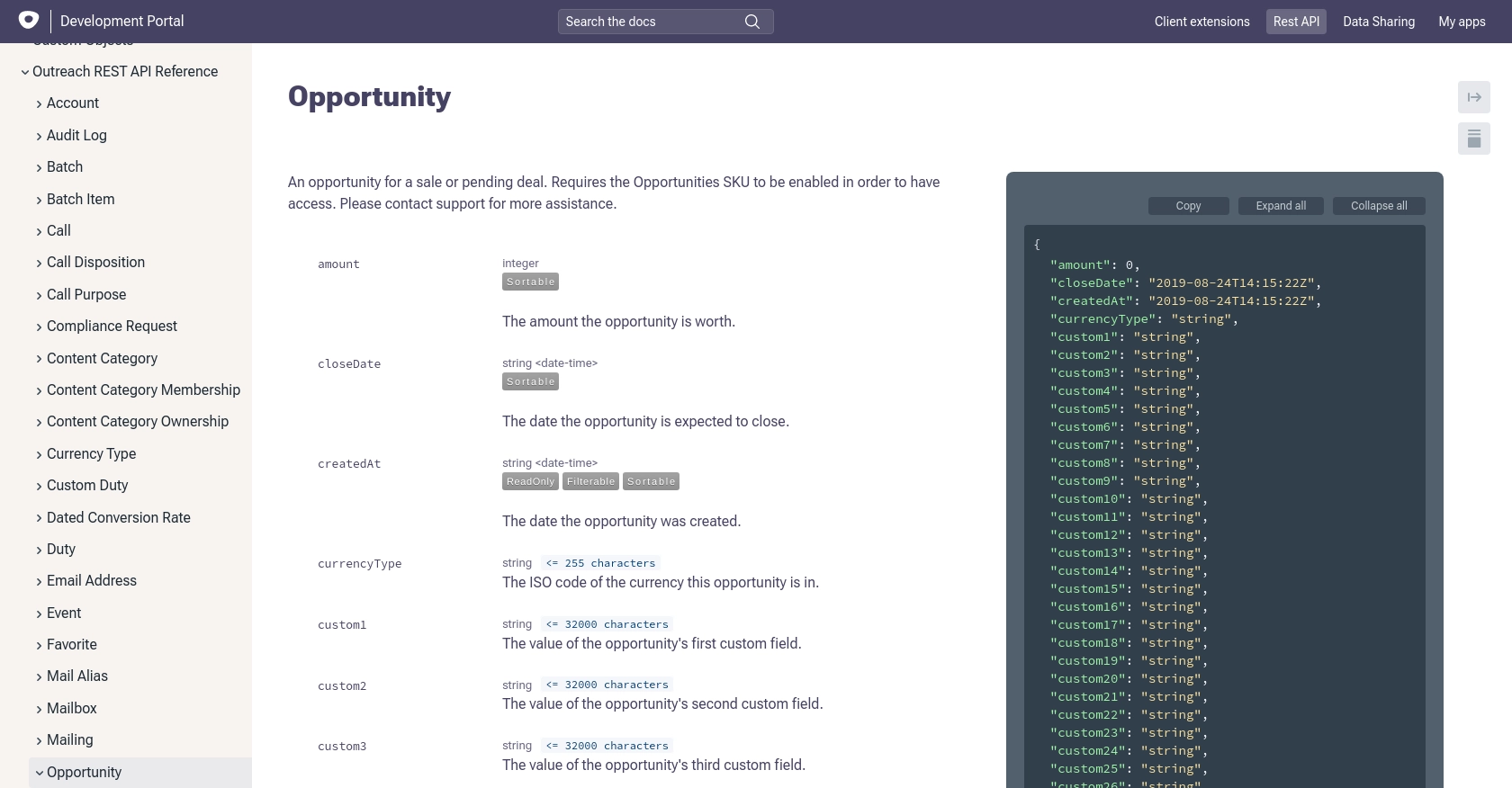
Conclusion and Best Practices for Using the Outreach API in JavaScript
Integrating with the Outreach API to create opportunities in JavaScript can significantly enhance your application's capabilities by automating sales processes and improving efficiency. By following the steps outlined in this guide, you can seamlessly connect your application to Outreach and manage opportunities effectively.
Best Practices for Secure and Efficient API Integration with Outreach
- Securely Store Credentials: Always store your OAuth client ID, client secret, and access tokens securely. Avoid hardcoding them in your source code and consider using environment variables or secure vaults.
- Handle Rate Limiting: Be mindful of the Outreach API's rate limit of 10,000 requests per hour. Implement logic to handle 429 errors and retry requests after the rate limit resets.
- Refresh Tokens Periodically: Access tokens are short-lived. Use the refresh token to obtain new access tokens before they expire to maintain uninterrupted API access.
- Optimize Data Handling: Standardize and transform data fields as needed to ensure consistency across your application and the Outreach platform.
Enhance Your Integration Strategy with Endgrate
While integrating with the Outreach API can be straightforward, managing multiple integrations across different platforms can become complex. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Outreach. By leveraging Endgrate, you can save time and resources, allowing your team to focus on core product development while offering an intuitive integration experience for your customers.
Explore how Endgrate can streamline your integration efforts by visiting Endgrate today.
Read More
Ready to get started?