How to Create Or Update Companies with the Apollo API in PHP
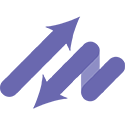
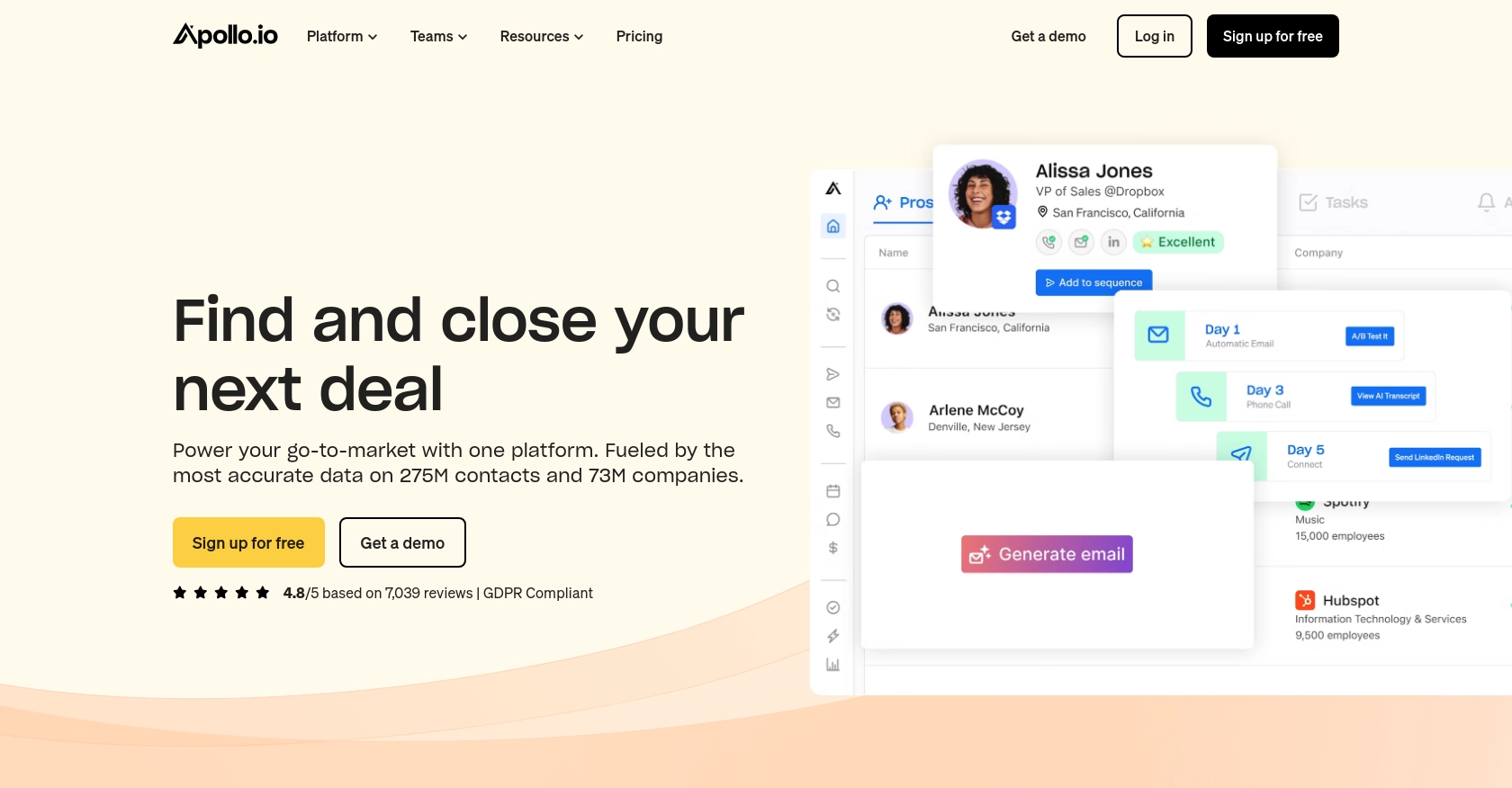
Introduction to Apollo API for Company Management
Apollo is a comprehensive sales intelligence and engagement platform that empowers businesses to enhance their growth strategies by providing access to a vast database of contacts and companies. With Apollo, companies can streamline their sales processes, improve lead generation, and optimize outreach efforts.
For developers, integrating with the Apollo API offers the ability to manage company data efficiently. By leveraging the API, developers can create or update company records, ensuring that their databases are always up-to-date with the latest information. This integration is particularly useful for automating CRM updates, enhancing data accuracy, and reducing manual data entry.
In this article, we will explore how to use PHP to interact with the Apollo API for creating or updating company records. This guide will provide step-by-step instructions and sample code to help you seamlessly integrate Apollo's powerful capabilities into your applications.
Setting Up Your Apollo API Test Account
Before you begin integrating with the Apollo API, it's essential to set up a test account. This will allow you to safely experiment with API calls without affecting live data. Apollo provides a straightforward process for obtaining an API key, which is necessary for authenticating your requests.
Steps to Create an Apollo API Test Account
- Sign Up for Apollo: Visit the Apollo website and sign up for a free trial or create a demo account. This will give you access to the Apollo platform and its API features.
- Access API Settings: Once your account is set up, log in and navigate to the API settings section. Here, you can manage your API keys and configure your integration settings.
- Generate an API Key: In the API settings, click on the option to generate a new API key. This key will be used to authenticate your API requests. Ensure you store this key securely, as it provides access to your Apollo account.
Authenticating with Apollo API Using API Key
The Apollo API uses API key-based authentication. When making API requests, include your API key in the request headers. Here's an example of how to set this up in PHP:
// Set the API endpoint and headers
$url = "https://api.apollo.io/v1/accounts";
$headers = [
'Content-Type: application/json',
'Cache-Control: no-cache',
'X-Api-Key: YOUR_API_KEY_HERE'
];
// Initialize a cURL session
$ch = curl_init($url);
// Set cURL options
curl_setopt($ch, CURLOPT_HTTPHEADER, $headers);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
// Execute the request
$response = curl_exec($ch);
// Close the cURL session
curl_close($ch);
// Output the response
echo $response;
Replace YOUR_API_KEY_HERE
with the API key you generated. This code snippet demonstrates how to set up a basic GET request to the Apollo API using PHP's cURL library.
By following these steps, you can effectively set up a test environment for interacting with the Apollo API, allowing you to create or update company records with ease.
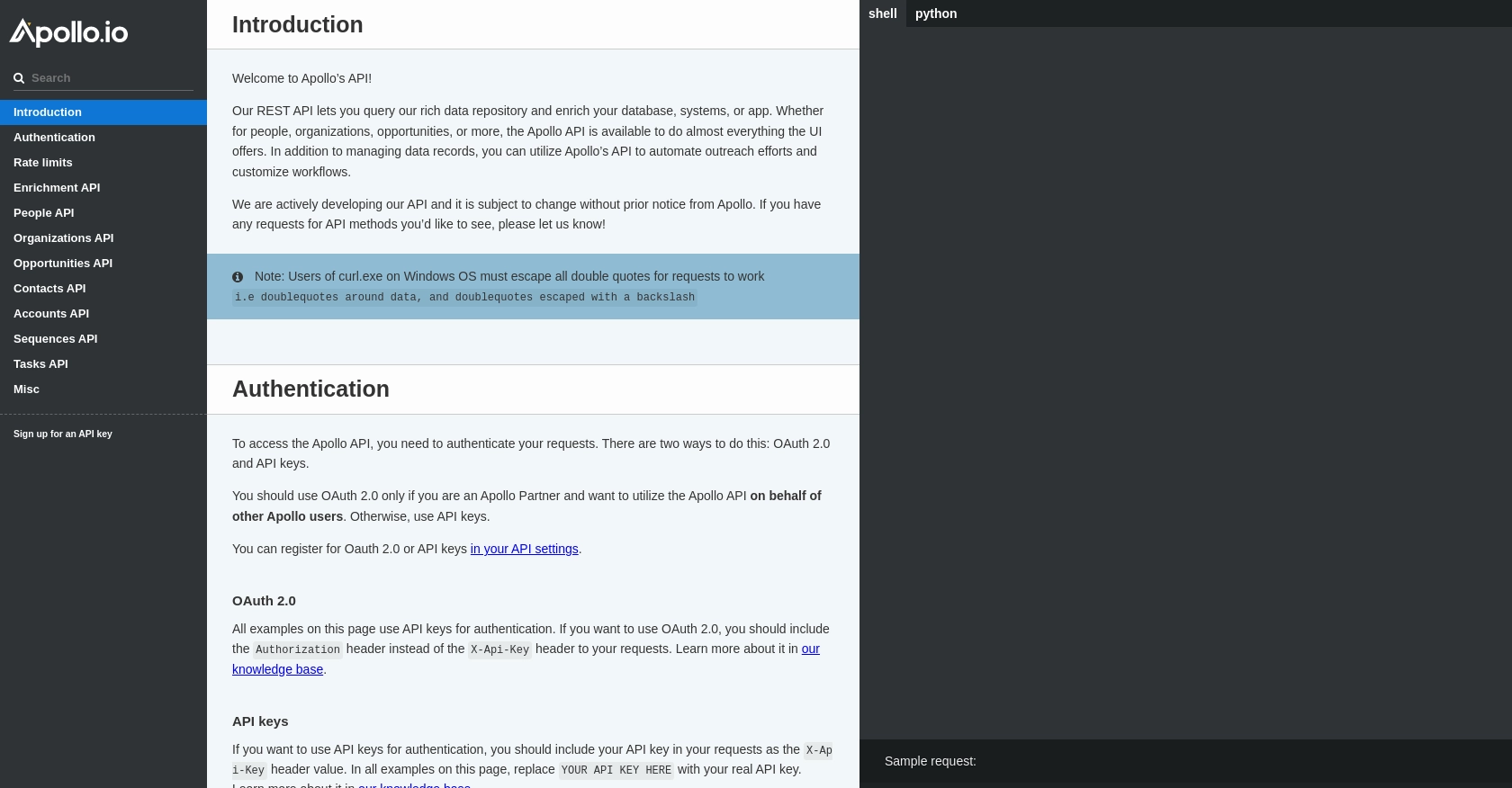
sbb-itb-96038d7
Making API Calls to Create or Update Companies with Apollo API in PHP
To interact with the Apollo API for creating or updating company records, you'll need to set up your PHP environment correctly. This section will guide you through the process of making API calls using PHP, ensuring you can efficiently manage company data within your applications.
Setting Up Your PHP Environment for Apollo API Integration
Before making API calls, ensure your PHP environment is ready. You'll need PHP 7.4 or later and the cURL extension enabled. If you're unsure about your PHP version or cURL setup, check your PHP configuration or consult your hosting provider.
Installing Necessary PHP Dependencies
To make HTTP requests, we'll use PHP's built-in cURL library. Ensure it's enabled in your php.ini
file:
; Enable cURL extension module
extension=curl
Example Code for Creating a Company with Apollo API
Here's how you can create a new company record using the Apollo API:
// Set the API endpoint and headers
$url = "https://api.apollo.io/v1/accounts";
$headers = [
'Content-Type: application/json',
'Cache-Control: no-cache',
'X-Api-Key: YOUR_API_KEY_HERE'
];
// Define the company data
$data = [
'name' => 'New Company',
'domain' => 'newcompany.com',
'phone_number' => '123-456-7890',
'raw_address' => '123 Main St, Anytown, USA'
];
// Initialize a cURL session
$ch = curl_init($url);
// Set cURL options
curl_setopt($ch, CURLOPT_HTTPHEADER, $headers);
curl_setopt($ch, CURLOPT_POST, true);
curl_setopt($ch, CURLOPT_POSTFIELDS, json_encode($data));
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
// Execute the request
$response = curl_exec($ch);
// Close the cURL session
curl_close($ch);
// Output the response
echo $response;
Replace YOUR_API_KEY_HERE
with your actual API key. This code snippet demonstrates how to send a POST request to create a new company record in Apollo.
Example Code for Updating a Company with Apollo API
To update an existing company record, use the following code:
// Set the API endpoint and headers
$url = "https://api.apollo.io/v1/accounts/YOUR_ACCOUNT_ID";
$headers = [
'Content-Type: application/json',
'Cache-Control: no-cache',
'X-Api-Key: YOUR_API_KEY_HERE'
];
// Define the updated company data
$data = [
'name' => 'Updated Company Name'
];
// Initialize a cURL session
$ch = curl_init($url);
// Set cURL options
curl_setopt($ch, CURLOPT_HTTPHEADER, $headers);
curl_setopt($ch, CURLOPT_CUSTOMREQUEST, 'PUT');
curl_setopt($ch, CURLOPT_POSTFIELDS, json_encode($data));
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
// Execute the request
$response = curl_exec($ch);
// Close the cURL session
curl_close($ch);
// Output the response
echo $response;
Replace YOUR_ACCOUNT_ID
with the ID of the company you wish to update. This code snippet shows how to send a PUT request to update company details in Apollo.
Handling API Responses and Errors
After making an API call, it's crucial to handle the response correctly. Check the HTTP status code to determine if the request was successful. Here's an example of how to handle responses and errors:
// Execute the request
$response = curl_exec($ch);
$httpCode = curl_getinfo($ch, CURLINFO_HTTP_CODE);
// Check for errors
if ($httpCode == 200) {
echo "Request successful: " . $response;
} else {
echo "Error: " . $httpCode . " - " . $response;
}
// Close the cURL session
curl_close($ch);
By checking the HTTP status code, you can determine if the API call was successful and handle any errors accordingly.
Verifying API Call Success in Apollo Dashboard
To ensure your API calls are successful, verify the changes in your Apollo dashboard. Check the company records to confirm that new entries are created or existing ones are updated as expected.
For more details on error codes and rate limits, refer to the Apollo API documentation.
Conclusion and Best Practices for Apollo API Integration in PHP
Integrating with the Apollo API using PHP provides a robust solution for managing company data efficiently. By following the steps outlined in this guide, developers can automate CRM updates, enhance data accuracy, and reduce manual data entry, ultimately streamlining business processes.
Best Practices for Secure and Efficient Apollo API Usage
- Secure API Key Storage: Always store your API keys securely, using environment variables or a secure vault, to prevent unauthorized access.
- Handle Rate Limits: Be mindful of Apollo's rate limits, which are 50 requests per minute, 100 per hour, and 300 per day. Implement logic to handle rate limit responses and retry requests as needed.
- Error Handling: Implement comprehensive error handling to manage API response codes effectively. This ensures that your application can gracefully handle any issues that arise during API interactions.
- Data Standardization: Ensure that data fields are standardized before sending them to the API. This helps maintain consistency and accuracy across your database.
Leverage Endgrate for Simplified Integration Solutions
While integrating with the Apollo API can be straightforward, managing multiple integrations can become complex. Endgrate offers a unified API endpoint that connects to various platforms, including Apollo, simplifying the integration process.
By using Endgrate, developers can save time and resources, focusing on core product development while outsourcing integration tasks. This approach allows for a more intuitive integration experience for your customers, enabling you to build once for each use case instead of multiple times for different integrations.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate today.
Read More
Ready to get started?