How to Create or Update Companies with the Cloze API in Python
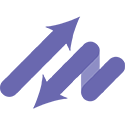
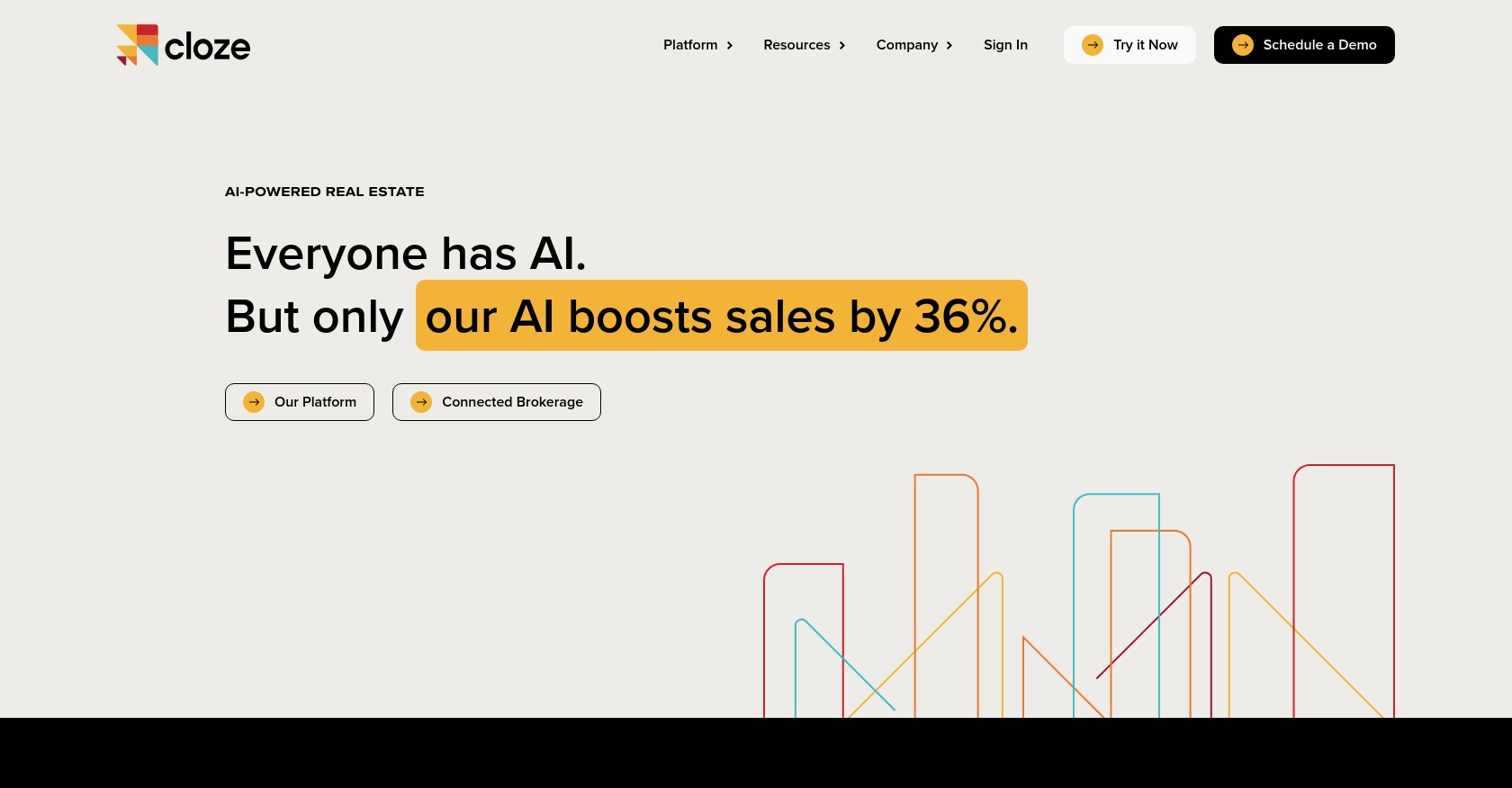
Introduction to Cloze API for Company Management
Cloze is a powerful relationship management platform that helps businesses streamline their interactions with clients, partners, and team members. With its comprehensive suite of tools, Cloze enables companies to manage contacts, projects, and communications effectively.
Developers may want to integrate with the Cloze API to automate and enhance their business processes. By connecting with Cloze, developers can create or update company records programmatically, ensuring that their CRM data is always up-to-date and accurate.
For example, a developer might use the Cloze API to automatically update company information from an external database, ensuring that all team members have access to the latest data without manual intervention.
Setting Up Your Cloze Test or Sandbox Account
Before you can start integrating with the Cloze API, you'll need to set up a test or sandbox account. This will allow you to safely experiment with API calls without affecting your live data.
Creating a Cloze Account
If you don't already have a Cloze account, you can sign up for a free trial on the Cloze website. This will give you access to the platform's features and allow you to explore its capabilities.
- Visit the Cloze website and click on the "Sign Up" button.
- Follow the on-screen instructions to create your account.
- Once your account is set up, log in to access the Cloze dashboard.
Generating an API Key for Cloze
To authenticate your API requests, you'll need to generate an API key. This key will be used to authorize your application to interact with the Cloze API.
- Log in to your Cloze account and navigate to the settings page.
- Under the "Integrations" section, find the option to create an API key.
- Follow the instructions to generate a new API key. Make sure to store this key securely, as it will be required for all API requests.
Configuring OAuth for Public Integrations
If you're planning to build a public integration with Cloze, you'll need to use OAuth for authentication. This allows users outside your organization to authorize your application to access their Cloze data.
- Contact Cloze support at support@cloze.com to get started with OAuth integration.
- During development, you can use your API key for testing purposes.
For more detailed instructions on setting up your API key and OAuth, refer to the Cloze API documentation.
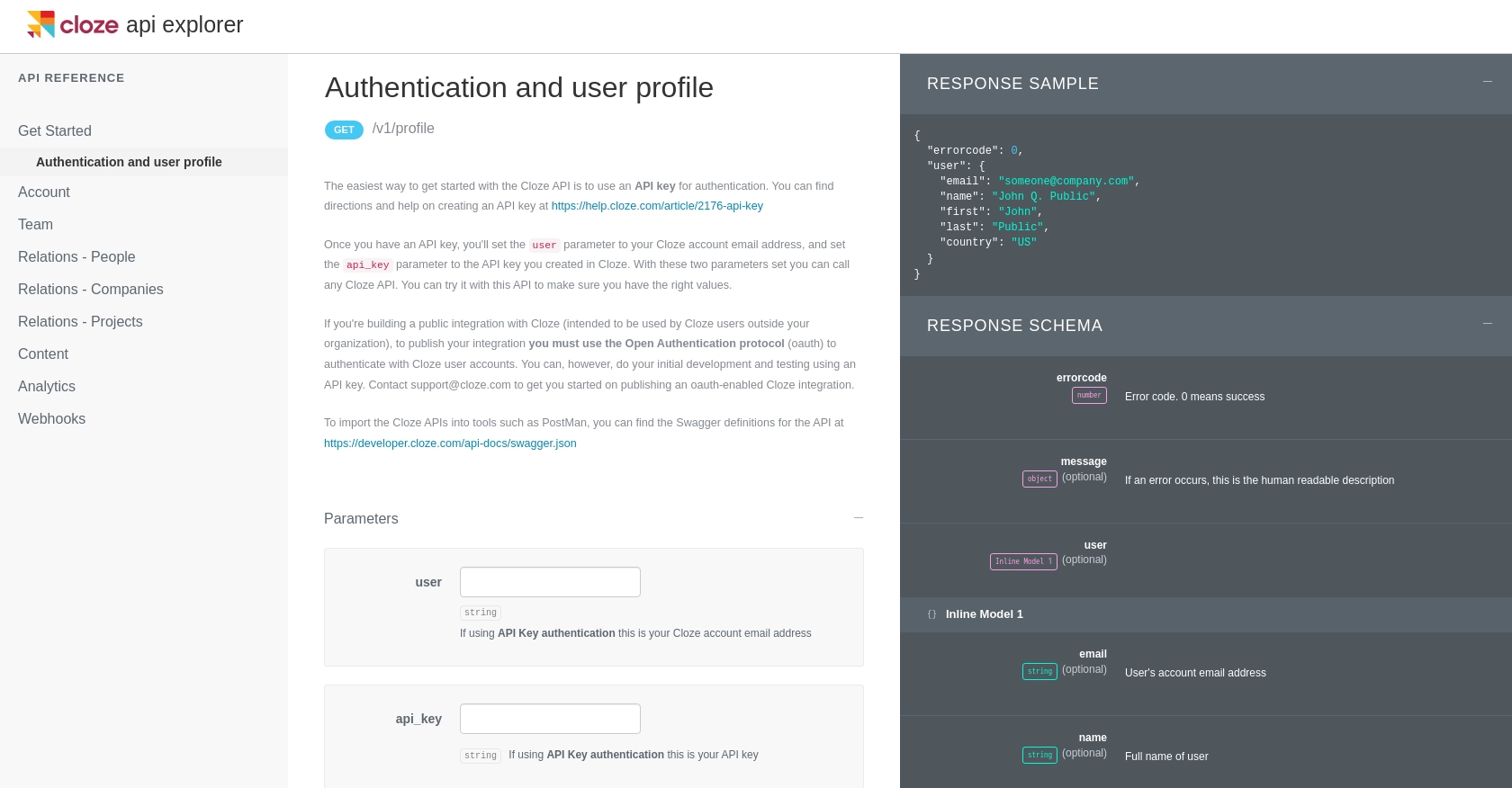
sbb-itb-96038d7
Making API Calls to Create or Update Companies with Cloze API in Python
To interact with the Cloze API for creating or updating company records, you'll need to use Python. This section will guide you through the necessary steps, including setting up your environment and executing API calls.
Setting Up Your Python Environment for Cloze API Integration
Before making API calls, ensure you have the following prerequisites installed on your machine:
- Python 3.11.1
- The Python package installer pip
Next, install the requests
library, which will be used to make HTTP requests to the Cloze API:
pip install requests
Creating a Company with Cloze API Using Python
To create a company, you'll need to send a POST request to the Cloze API endpoint. Here's a sample code snippet to get you started:
import requests
# Set the API endpoint
url = "https://api.cloze.com/v1/companies/create"
# Set the request headers
headers = {
"Content-Type": "application/json",
"Authorization": "Bearer Your_API_Key"
}
# Define the company data
company_data = {
"name": "Example Company",
"description": "A sample company for demonstration purposes",
"domains": ["example.com"]
}
# Send the POST request
response = requests.post(url, json=company_data, headers=headers)
# Check if the request was successful
if response.status_code == 200:
print("Company created successfully:", response.json())
else:
print("Failed to create company:", response.status_code, response.text)
Replace Your_API_Key
with the API key you generated earlier. This script sends a request to create a new company with the specified details. If successful, it will print the response from the API.
Updating a Company with Cloze API Using Python
To update an existing company, modify the endpoint to /v1/companies/update
and provide the necessary company details:
import requests
# Set the API endpoint
url = "https://api.cloze.com/v1/companies/update"
# Set the request headers
headers = {
"Content-Type": "application/json",
"Authorization": "Bearer Your_API_Key"
}
# Define the updated company data
company_update = {
"name": "Example Company",
"description": "Updated description for the company",
"domains": ["example.com"]
}
# Send the POST request
response = requests.post(url, json=company_update, headers=headers)
# Check if the request was successful
if response.status_code == 200:
print("Company updated successfully:", response.json())
else:
print("Failed to update company:", response.status_code, response.text)
This code snippet updates the company information. Ensure the company data matches the existing records in your Cloze account.
Handling API Response and Errors
After making an API call, it's crucial to handle the response correctly. The Cloze API returns a JSON response, which you can parse to verify the success of your request. If the request fails, the response will include an error code and message.
Refer to the Cloze API documentation for detailed information on error codes and handling.
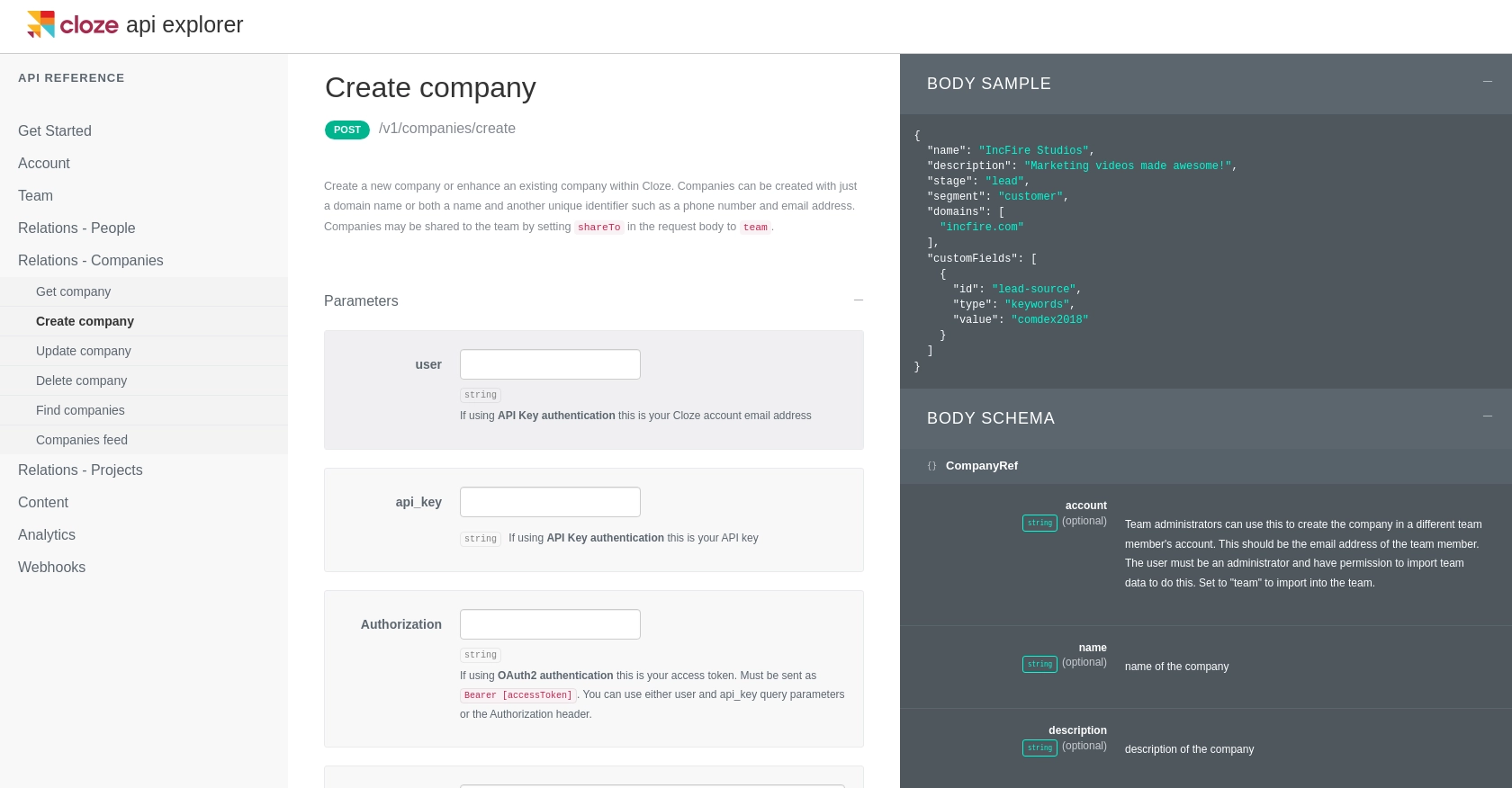
Conclusion and Best Practices for Using Cloze API in Python
Integrating with the Cloze API allows developers to efficiently manage company data, ensuring that CRM systems are always up-to-date. By automating the creation and updating of company records, businesses can save time and reduce the risk of errors associated with manual data entry.
Best Practices for Secure and Efficient API Integration with Cloze
- Secure API Keys: Always store your API keys securely and never expose them in your codebase. Consider using environment variables or secure vaults to manage sensitive information.
- Handle Rate Limiting: Be mindful of API rate limits to avoid throttling. Implement retry logic with exponential backoff to handle rate limit responses gracefully.
- Data Standardization: Ensure that data fields are standardized and consistent across your systems to facilitate seamless integration and data synchronization.
- Error Handling: Implement robust error handling to manage API response errors effectively. Log errors for troubleshooting and consider notifying relevant stakeholders when critical issues arise.
By following these best practices, you can enhance the reliability and security of your integration with the Cloze API.
Streamline Your Integration Process with Endgrate
For developers looking to simplify their integration efforts, consider using Endgrate. With Endgrate, you can save time and resources by outsourcing integrations, allowing you to focus on your core product. Endgrate offers a unified API endpoint that connects to multiple platforms, providing an easy and intuitive integration experience for your customers.
Visit Endgrate to learn more about how you can streamline your integration processes and enhance your business operations.
Read More
Ready to get started?