How to Create or Update Companies with the PipelineCRM API in Python
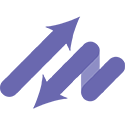
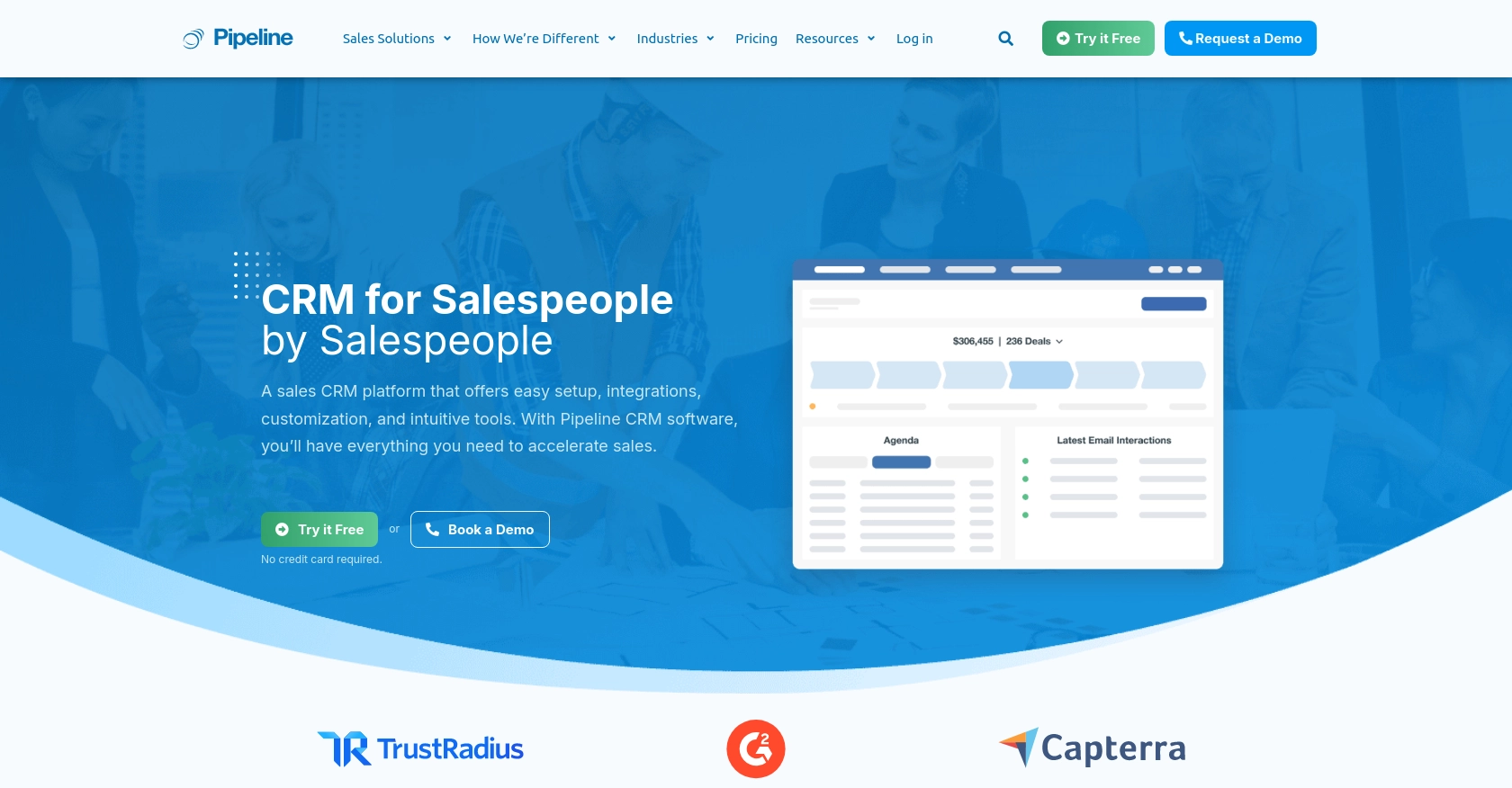
Introduction to PipelineCRM
PipelineCRM is a robust customer relationship management platform designed to help businesses manage their sales processes efficiently. With features like contact management, sales tracking, and reporting, PipelineCRM provides a comprehensive solution for sales teams looking to streamline their operations.
Developers may want to integrate with PipelineCRM's API to automate and enhance their sales workflows. For example, you could use the API to create or update company records directly from your application, ensuring that your sales data is always up-to-date and accessible.
Setting Up Your PipelineCRM Test Account
Before you can start integrating with the PipelineCRM API, you'll need to set up a test account. This will allow you to safely experiment with API calls without affecting your live data.
Creating a PipelineCRM Account
If you don't already have a PipelineCRM account, you can sign up for a free trial on their website. This trial will give you access to the features necessary for testing API interactions.
- Visit the PipelineCRM website.
- Click on the "Sign Up" button and follow the instructions to create your account.
- Once your account is set up, log in to access the dashboard.
Generating Your PipelineCRM API Key
PipelineCRM uses API key-based authentication to secure API requests. Follow these steps to generate your API key:
- Log in to your PipelineCRM account.
- Navigate to the "Settings" section from the dashboard.
- Find the "API Keys" tab and click on it.
- Click on "Generate New API Key" and provide a name for your key.
- Copy the generated API key and store it securely, as you'll need it for authentication in your API requests.
For more detailed information, you can refer to the PipelineCRM API documentation.
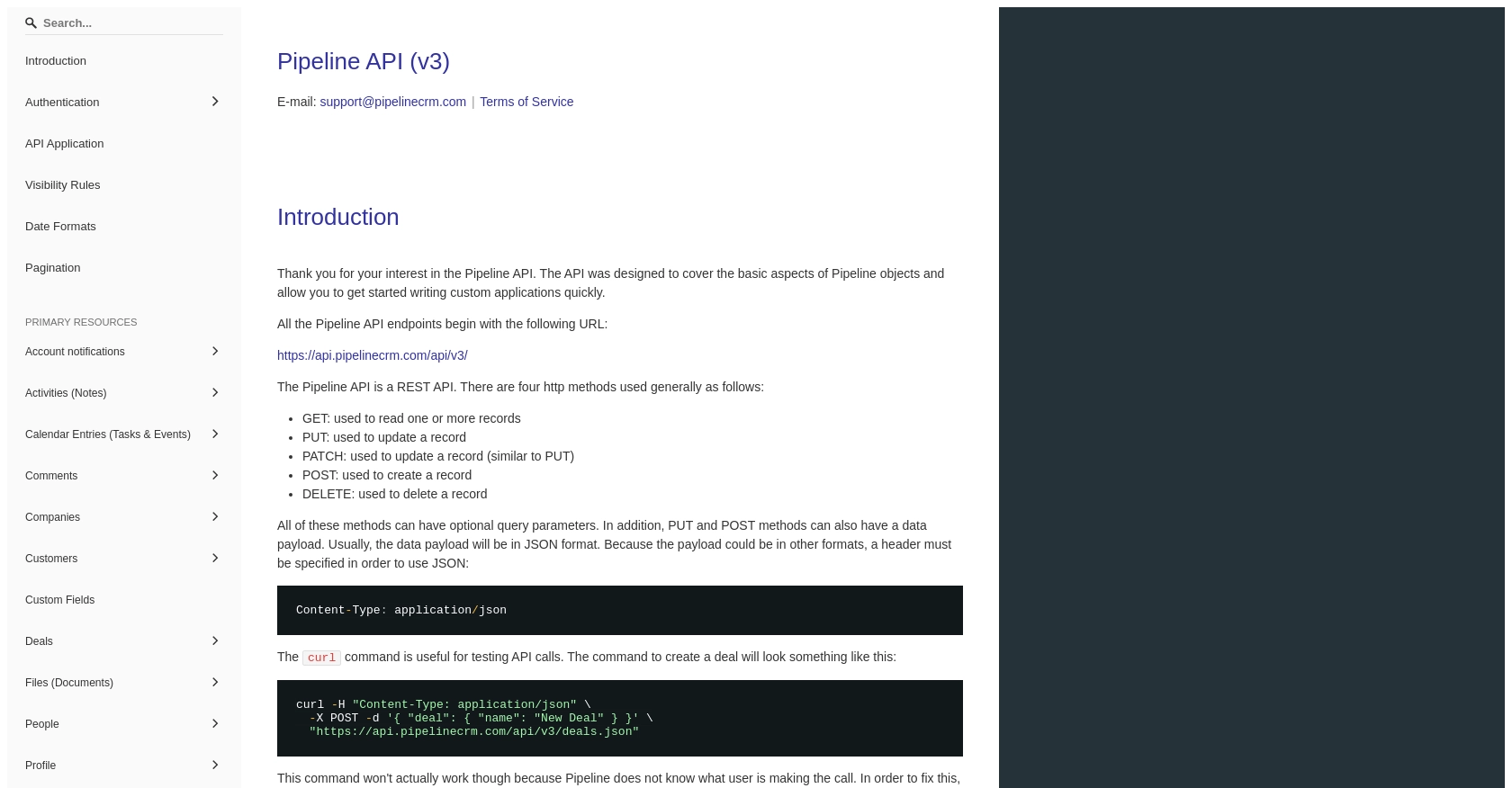
sbb-itb-96038d7
Making API Calls to Create or Update Companies with PipelineCRM in Python
To interact with the PipelineCRM API for creating or updating company records, you'll need to use Python. This section will guide you through the necessary steps, including setting up your environment, writing the code, and handling responses and errors effectively.
Setting Up Your Python Environment for PipelineCRM API Integration
Before you begin, ensure that you have Python installed on your machine. This tutorial uses Python 3.11.1. Additionally, you'll need the requests
library to make HTTP requests to the PipelineCRM API. Install it using the following command:
pip install requests
Creating or Updating Companies with the PipelineCRM API
Now that your environment is ready, you can proceed to write the code to create or update company records in PipelineCRM. Below is a sample script to help you get started:
import requests
# Define the API endpoint for creating or updating companies
url = "https://api.pipelinecrm.com/v3/companies"
# Set the request headers with your API key
headers = {
"Content-Type": "application/json",
"Authorization": "Bearer YOUR_API_KEY"
}
# Define the company data to be created or updated
company_data = {
"name": "New Company",
"industry": "Technology",
"website": "https://newcompany.com"
}
# Make a POST request to create or update the company
response = requests.post(url, json=company_data, headers=headers)
# Check the response status code
if response.status_code == 201:
print("Company created successfully.")
elif response.status_code == 200:
print("Company updated successfully.")
else:
print(f"Failed to create or update company. Status code: {response.status_code}")
print("Response:", response.json())
Replace YOUR_API_KEY
with the API key you generated from your PipelineCRM account.
Verifying API Call Success and Handling Errors
After running the script, you should verify that the company was created or updated successfully by checking your PipelineCRM test account. If the request fails, the script will print the status code and response message, which can help you diagnose the issue.
Common error codes include:
- 400 Bad Request: The request was malformed. Check the data you are sending.
- 401 Unauthorized: The API key is missing or invalid.
- 404 Not Found: The endpoint URL is incorrect.
- 500 Internal Server Error: An error occurred on the server side.
For more detailed information on error codes, refer to the PipelineCRM API documentation.
Conclusion: Best Practices for Integrating with PipelineCRM API
Integrating with the PipelineCRM API to create or update company records can significantly enhance your sales processes by ensuring that your data is always current and accessible. However, to make the most of this integration, it's essential to follow best practices.
Securely Storing API Keys and Credentials
Always store your API keys securely. Avoid hardcoding them directly into your scripts. Instead, use environment variables or secure vaults to manage sensitive information. This practice helps prevent unauthorized access to your PipelineCRM account.
Handling Rate Limits and Optimizing API Calls
Be mindful of any rate limits imposed by the PipelineCRM API. Although specific rate limit details are not provided in the documentation, it's a good practice to implement retry logic and exponential backoff strategies to handle potential rate limiting gracefully.
Standardizing and Transforming Data Fields
Ensure that the data you send to PipelineCRM is standardized and correctly formatted. This includes validating fields like email addresses and URLs to prevent errors during API calls. Consistent data formatting helps maintain data integrity across your systems.
Streamlining Integrations with Endgrate
If managing multiple integrations becomes overwhelming, consider using a tool like Endgrate. Endgrate allows you to build once for each use case and leverage a unified API endpoint for various platforms, including PipelineCRM. This approach saves time and resources, enabling you to focus on your core product development.
By following these best practices and utilizing tools like Endgrate, you can create a seamless and efficient integration experience for your team and customers. For more information on how Endgrate can simplify your integration needs, visit Endgrate.
Read More
Ready to get started?