How to Create or Update Contact with the Microsoft Dynamics 365 API in Python
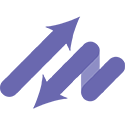
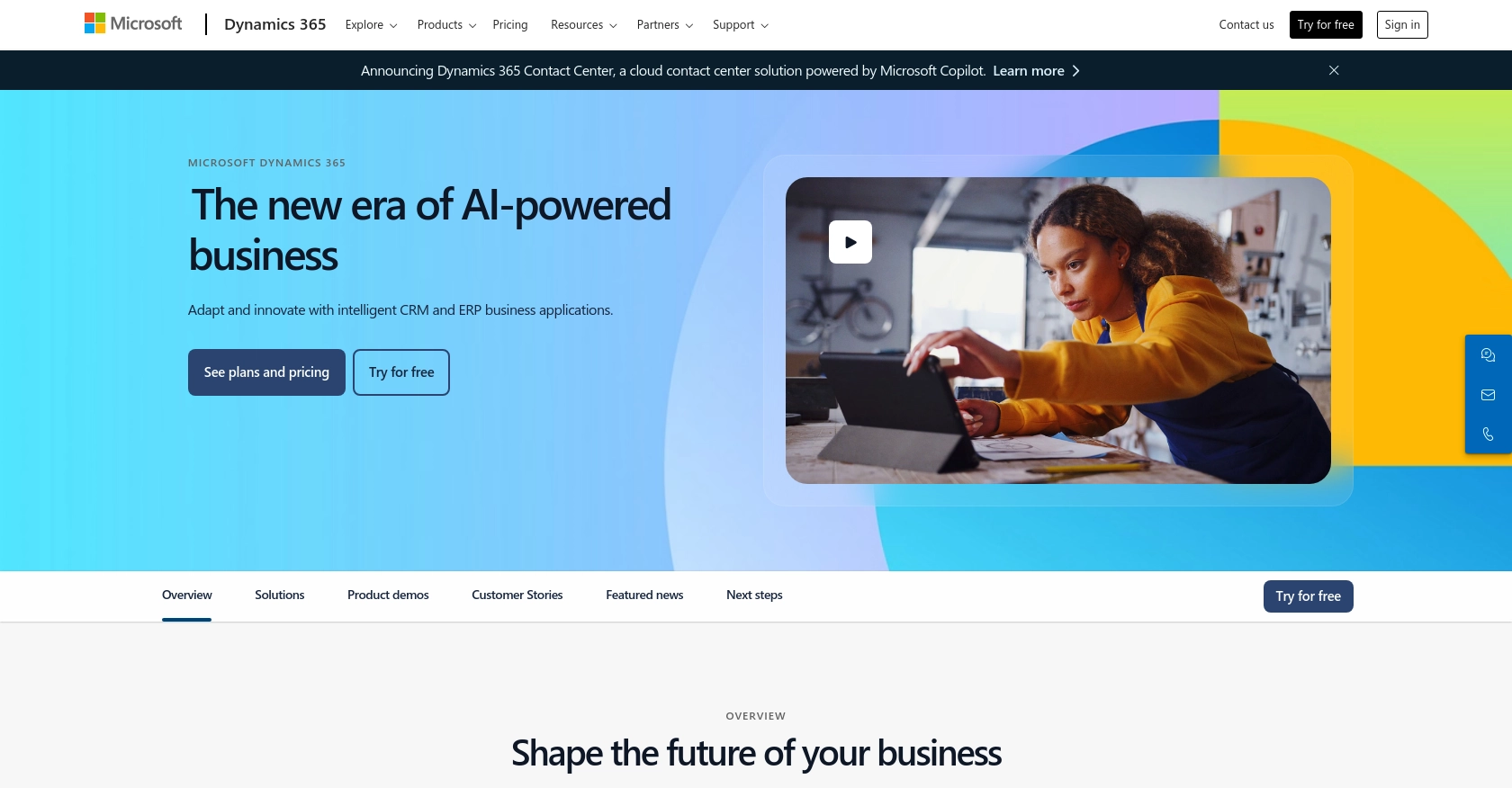
Introduction to Microsoft Dynamics 365 API Integration
Microsoft Dynamics 365 is a comprehensive suite of business applications that combines CRM and ERP capabilities to streamline business processes and improve customer engagement. It offers a range of tools for sales, customer service, finance, operations, and more, making it a versatile solution for businesses of all sizes.
Developers may want to integrate with Microsoft Dynamics 365 to manage customer data effectively, automate workflows, and enhance business intelligence. For example, using the Dynamics 365 API, a developer can create or update contact information directly from a custom application, ensuring that customer records are always up-to-date and accessible across the organization.
Setting Up a Microsoft Dynamics 365 Test/Sandbox Account
Before you can start integrating with the Microsoft Dynamics 365 API, you'll need to set up a test or sandbox account. This allows you to safely experiment with API calls without affecting live data. Microsoft Dynamics 365 offers a trial version that you can use for this purpose.
Step-by-Step Guide to Creating a Microsoft Dynamics 365 Sandbox Account
- Sign Up for a Free Trial: Visit the Microsoft Dynamics 365 Free Trial page. Follow the instructions to create a trial account. This will give you access to a sandbox environment where you can test API integrations.
- Access the Admin Center: Once your account is set up, log in to the Microsoft 365 Admin Center. Here, you can manage your Dynamics 365 environment and configure necessary settings.
- Create a Sandbox Environment: Navigate to the Dynamics 365 admin center. Under the "Environments" section, select "New" to create a sandbox environment. This is crucial for testing your API interactions without impacting production data.
Registering an Application for OAuth Authentication
Microsoft Dynamics 365 uses OAuth 2.0 for authentication. To interact with the API, you need to register an application in your Microsoft Entra ID tenant.
-
Register Your Application: Go to the Azure Portal and navigate to "Azure Active Directory" > "App registrations" > "New registration". Provide a name for your app and set the redirect URI to
https://localhost
for local development. - Configure API Permissions: After registering, select your app and navigate to "API permissions". Click "Add a permission" and choose "Dynamics CRM". Ensure you grant the necessary permissions for accessing and managing contacts.
- Generate Client Secret: Under "Certificates & secrets", create a new client secret. Note down the secret value as it will be used for authentication in your API calls.
For more detailed instructions on setting up OAuth authentication, refer to the official documentation: Use OAuth authentication with Microsoft Dataverse.
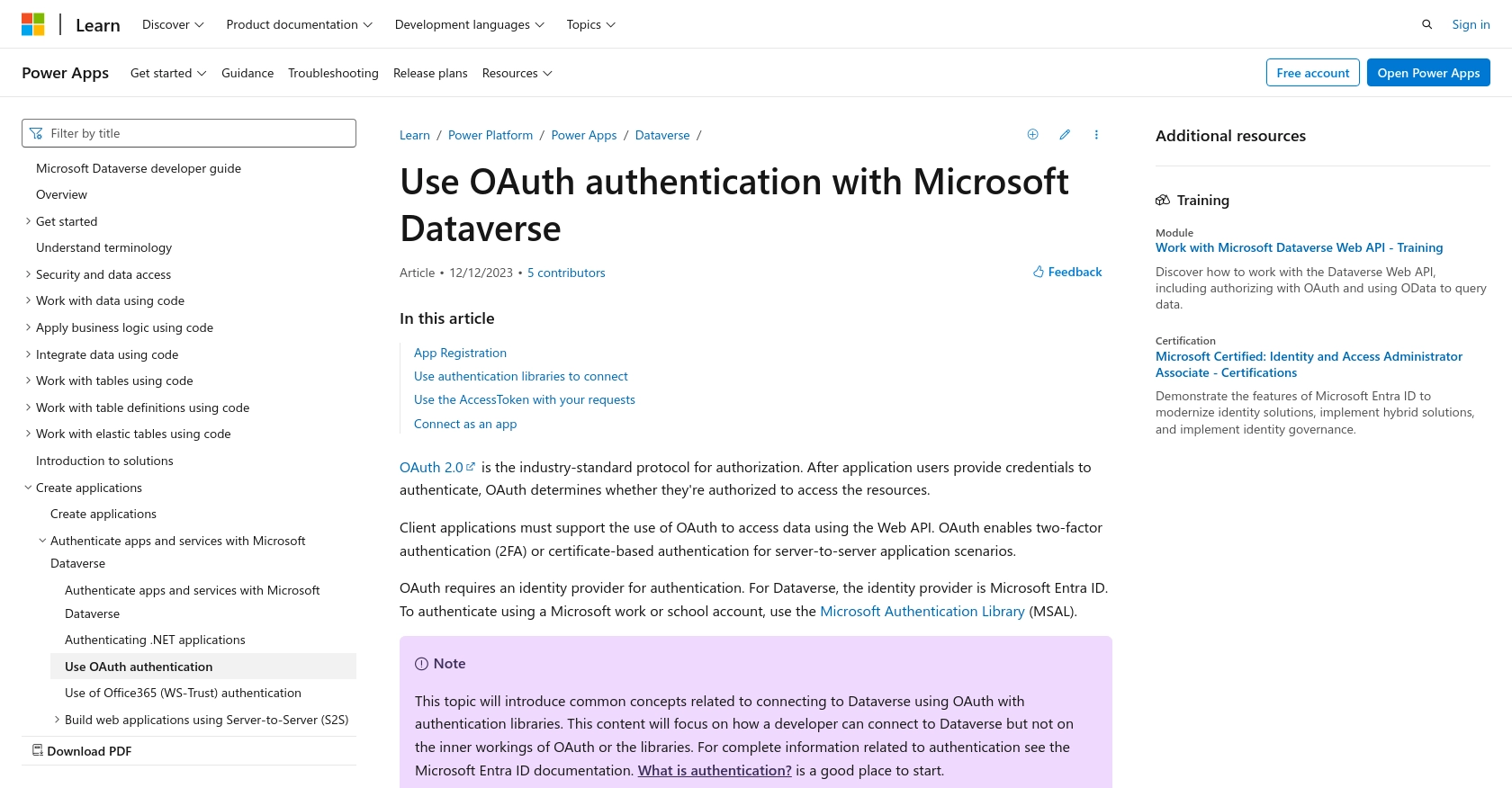
sbb-itb-96038d7
How to Make the Actual API Call to Create or Update Contacts in Microsoft Dynamics 365 Using Python
Setting Up Your Python Environment for Microsoft Dynamics 365 API Integration
To interact with the Microsoft Dynamics 365 API using Python, ensure you have Python 3.11.1 installed on your machine. Additionally, you'll need the requests
library to handle HTTP requests. Install it using the following command:
pip install requests
Creating a Python Script to Interact with Microsoft Dynamics 365 API
Once your environment is set up, you can create a Python script to make API calls. Below is an example of how to create or update a contact in Microsoft Dynamics 365.
import requests
import json
# Set the API endpoint and headers
url = "https://yourorg.api.crm.dynamics.com/api/data/v9.2/contacts"
headers = {
"Authorization": "Bearer Your_Access_Token",
"Content-Type": "application/json"
}
# Define the contact data
contact_data = {
"firstname": "John",
"lastname": "Doe",
"emailaddress1": "john.doe@example.com"
}
# Make a POST request to create a new contact
response = requests.post(url, headers=headers, data=json.dumps(contact_data))
# Check if the request was successful
if response.status_code == 201:
print("Contact created successfully.")
else:
print(f"Failed to create contact: {response.status_code} - {response.text}")
# To update an existing contact, use the PATCH method and specify the contact ID
contact_id = "contact_id_here"
update_url = f"{url}({contact_id})"
update_data = {
"lastname": "Smith"
}
response = requests.patch(update_url, headers=headers, data=json.dumps(update_data))
if response.status_code == 204:
print("Contact updated successfully.")
else:
print(f"Failed to update contact: {response.status_code} - {response.text}")
Verifying the API Call Success in Microsoft Dynamics 365 Sandbox
After running the script, verify the success of your API call by checking the Microsoft Dynamics 365 sandbox environment. If you created a contact, it should appear in the contacts list. If you updated a contact, the changes should be reflected in the contact's details.
Handling Errors and Understanding Error Codes
When making API calls, it's crucial to handle potential errors. Common HTTP status codes include:
- 201 Created: The contact was successfully created.
- 204 No Content: The contact was successfully updated.
- 400 Bad Request: The request was malformed.
- 401 Unauthorized: Authentication failed. Check your access token.
- 404 Not Found: The specified contact ID does not exist.
For more detailed error handling, refer to the Microsoft Dynamics 365 API documentation.
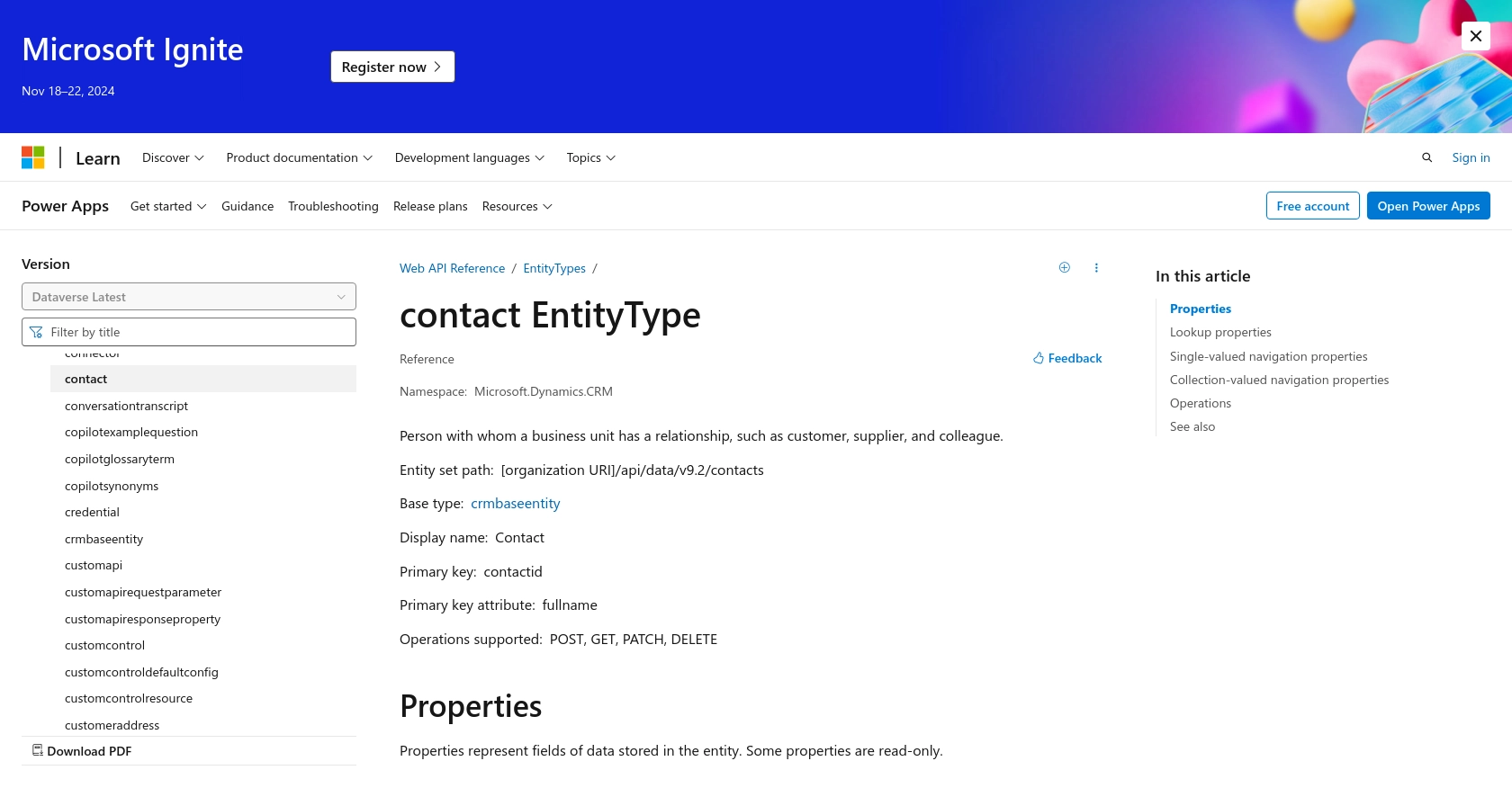
Best Practices for Microsoft Dynamics 365 API Integration
When integrating with the Microsoft Dynamics 365 API, it's essential to follow best practices to ensure security, efficiency, and maintainability. Here are some recommendations:
- Securely Store Credentials: Always store your client secrets and access tokens securely. Use environment variables or secure vaults to manage sensitive information.
- Handle Rate Limiting: Be aware of API rate limits to avoid throttling. Implement retry logic with exponential backoff to handle rate limit responses gracefully.
- Standardize Data Fields: Ensure that data fields are consistent across your application to avoid discrepancies and maintain data integrity.
- Monitor API Usage: Regularly monitor your API usage and performance to identify any potential issues or areas for optimization.
Streamline Your Integrations with Endgrate
Building and maintaining integrations can be time-consuming and complex. With Endgrate, you can simplify this process by leveraging a unified API endpoint that connects to multiple platforms, including Microsoft Dynamics 365. This allows you to focus on your core product while ensuring a seamless integration experience for your customers.
Visit Endgrate to learn more about how you can save time and resources by outsourcing your integration needs and enhancing your product offerings.
Read More
- https://endgrate.com/provider/microsoftdynamics365
- https://learn.microsoft.com/en-us/power-apps/developer/data-platform/authenticate-oauth
- https://learn.microsoft.com/en-us/graph/use-the-api
- https://learn.microsoft.com/en-us/power-apps/developer/data-platform/webapi/reference/contact?view=dataverse-latest
Ready to get started?