Using the Sage 100 API to Create or Update Items in PHP
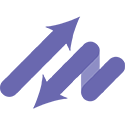
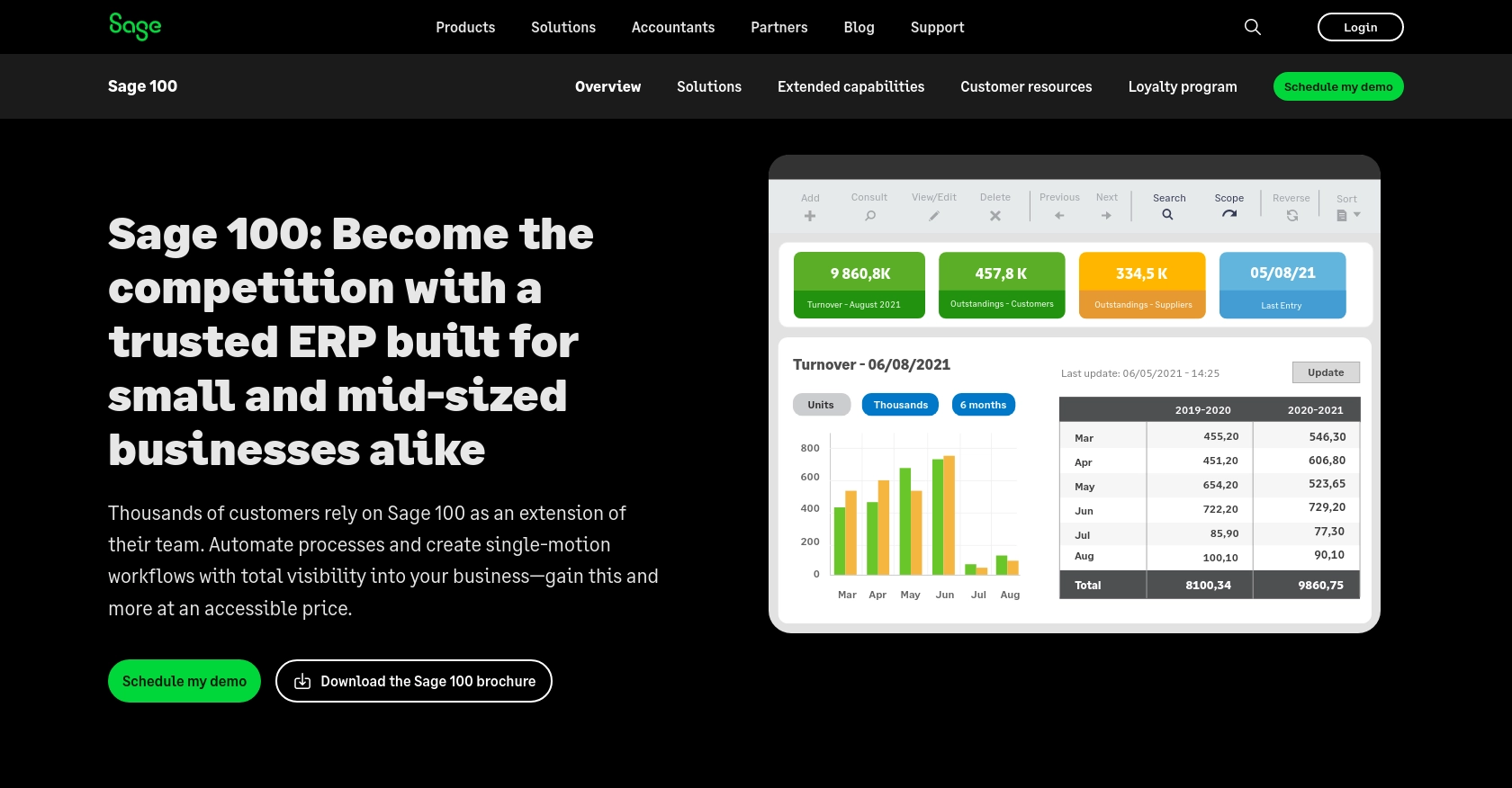
Introduction to Sage 100 API Integration
Sage 100 is a comprehensive ERP solution designed to help businesses manage their accounting, inventory, and operations efficiently. It offers robust features for financial management, sales, and customer service, making it a popular choice for small to medium-sized enterprises.
Integrating with the Sage 100 API allows developers to automate and streamline various business processes. For example, you can create or update inventory items directly from your application, ensuring that your product data is always up-to-date and reducing manual data entry errors.
This article will guide you through using PHP to interact with the Sage 100 API, focusing on creating or updating items. By following this tutorial, you can enhance your application's functionality and improve data management within your business operations.
Setting Up Your Sage 100 Test/Sandbox Account
Before you can start integrating with the Sage 100 API, it's essential to set up a test or sandbox account. This environment allows you to safely experiment with API calls without affecting your live data. Follow these steps to configure your Sage 100 environment for development:
Install and Configure the Sage 100 ODBC Driver
To interact with the Sage 100 API, you need to ensure that the Sage 100 ODBC driver is installed and properly configured. This involves setting up a Data Source Name (DSN) that connects to the Sage 100 ERP system. Here’s how to do it:
- Access the ODBC Data Source Administrator on your system.
- Create a new DSN that connects to the Sage 100 ERP system using the correct server, database, and authentication settings.
- Ensure that the Sage 100 ODBC driver is installed on your machine. You can download it from the Sage 100 website if needed.
For detailed instructions, refer to the Sage 100 ODBC Driver Configuration Guide.
Configure the Sage 100 Server
Once the ODBC driver is set up, configure the Sage 100 server to allow API access:
- Open the Server Manager on the Sage 100 Advanced server.
- Navigate to the Services section and locate the Sage 100 Client Server ODBC Driver Service.
- Set the Startup type to Automatic and start the service.
Set Up User Permissions
Ensure that the necessary permissions are granted to access the Sage 100 API:
- In Sage 100 Advanced, go to Library Master > Setup > System Configuration.
- Enable the C/S ODBC Driver for all users or specific users as needed.
Test the ODBC Connection
Verify that the ODBC connection is working correctly:
- Open the ODBC Data Source Administrator and test the connection for the DSN you created.
- If the connection is successful, you are ready to proceed with API integration.
For more information on troubleshooting connection issues, consult the Sage 100 ODBC Driver Configuration Guide.
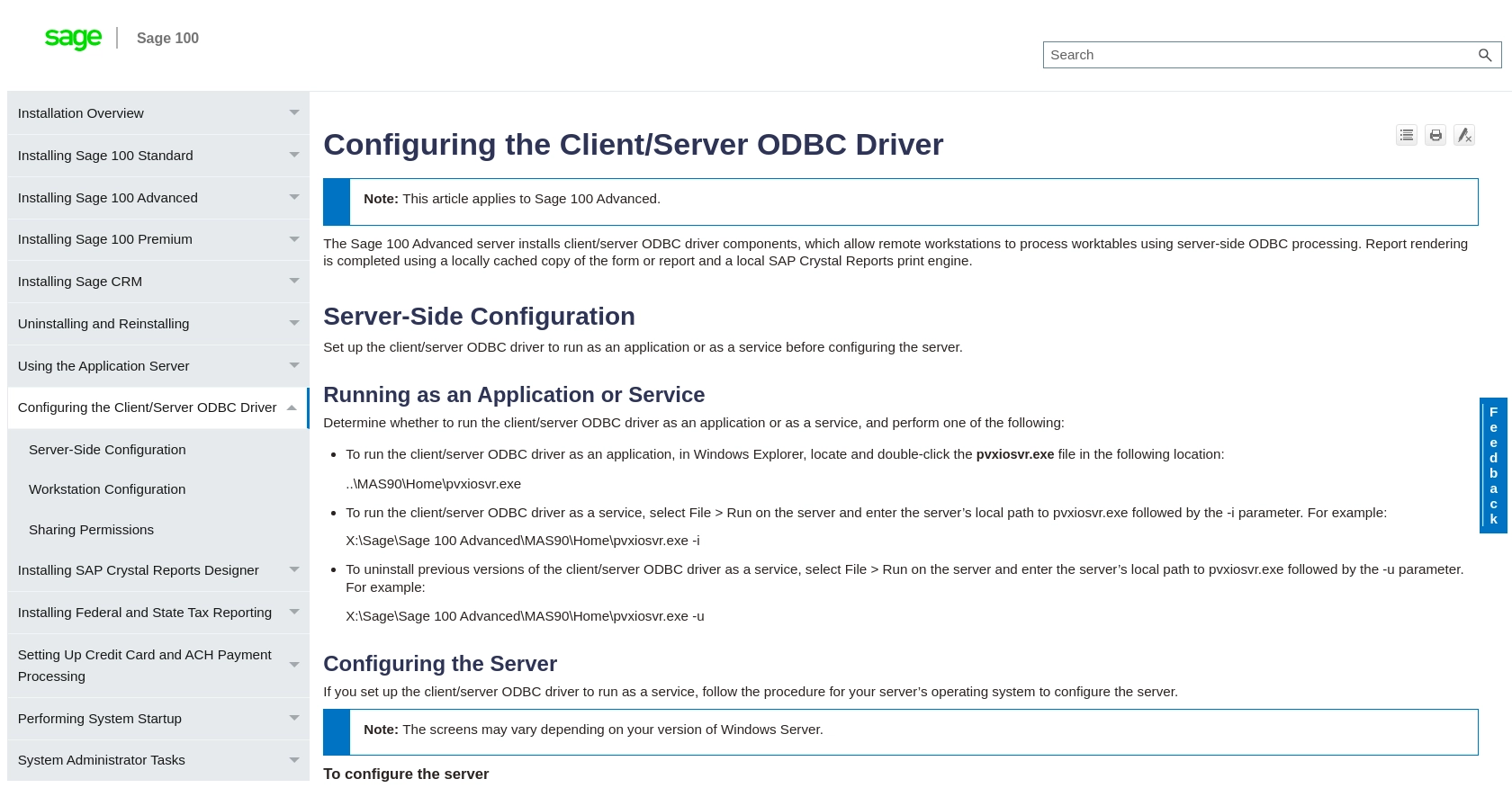
sbb-itb-96038d7
Making API Calls to Sage 100 for Creating or Updating Items Using PHP
To interact with the Sage 100 API for creating or updating items, you'll need to use PHP to establish a connection and execute the necessary SQL queries. This section will guide you through the process, including setting up your PHP environment and making the API call.
Setting Up Your PHP Environment for Sage 100 API Integration
Before making API calls, ensure your PHP environment is properly configured:
- Install PHP 7.4 or later on your system.
- Ensure the ODBC extension is enabled in your
php.ini
file. - Install necessary dependencies using Composer, if applicable.
Creating a Connection to the Sage 100 Database
To connect to the Sage 100 database, use the ODBC driver. Here's a sample PHP script to establish a connection:
<?php
$dsn = 'DSN_NAME'; // Replace with your DSN
$user = 'username'; // Replace with your username
$password = 'password'; // Replace with your password
try {
$connection = new PDO("odbc:$dsn", $user, $password);
echo "Connection successful!";
} catch (PDOException $e) {
echo "Connection failed: " . $e->getMessage();
}
?>
Replace DSN_NAME
, username
, and password
with your actual DSN, username, and password. This script uses PDO to connect to the Sage 100 database via ODBC.
Executing SQL Queries to Create or Update Items in Sage 100
Once connected, you can execute SQL queries to create or update items. Here's an example of how to insert a new item:
<?php
$itemCode = 'ITEM001';
$itemDescription = 'Sample Item';
$itemPrice = 100.00;
$sql = "INSERT INTO CI_Item (ItemCode, ItemDescription, ItemPrice) VALUES (?, ?, ?)";
$stmt = $connection->prepare($sql);
$stmt->execute([$itemCode, $itemDescription, $itemPrice]);
echo "Item created successfully!";
?>
This script prepares an SQL INSERT
statement to add a new item to the CI_Item
table. Adjust the table and column names as needed based on your Sage 100 setup.
Handling Errors and Verifying API Call Success
It's crucial to handle errors and verify the success of your API calls. Use try-catch blocks to manage exceptions:
<?php
try {
$stmt->execute([$itemCode, $itemDescription, $itemPrice]);
echo "Item created successfully!";
} catch (PDOException $e) {
echo "Error: " . $e->getMessage();
}
?>
Check your Sage 100 test environment to verify that the item was created or updated successfully. If the operation fails, review the error messages for troubleshooting.
For more detailed information on the Sage 100 API and SQL queries, refer to the Sage 100 File Layouts and Object Reference Documentation.
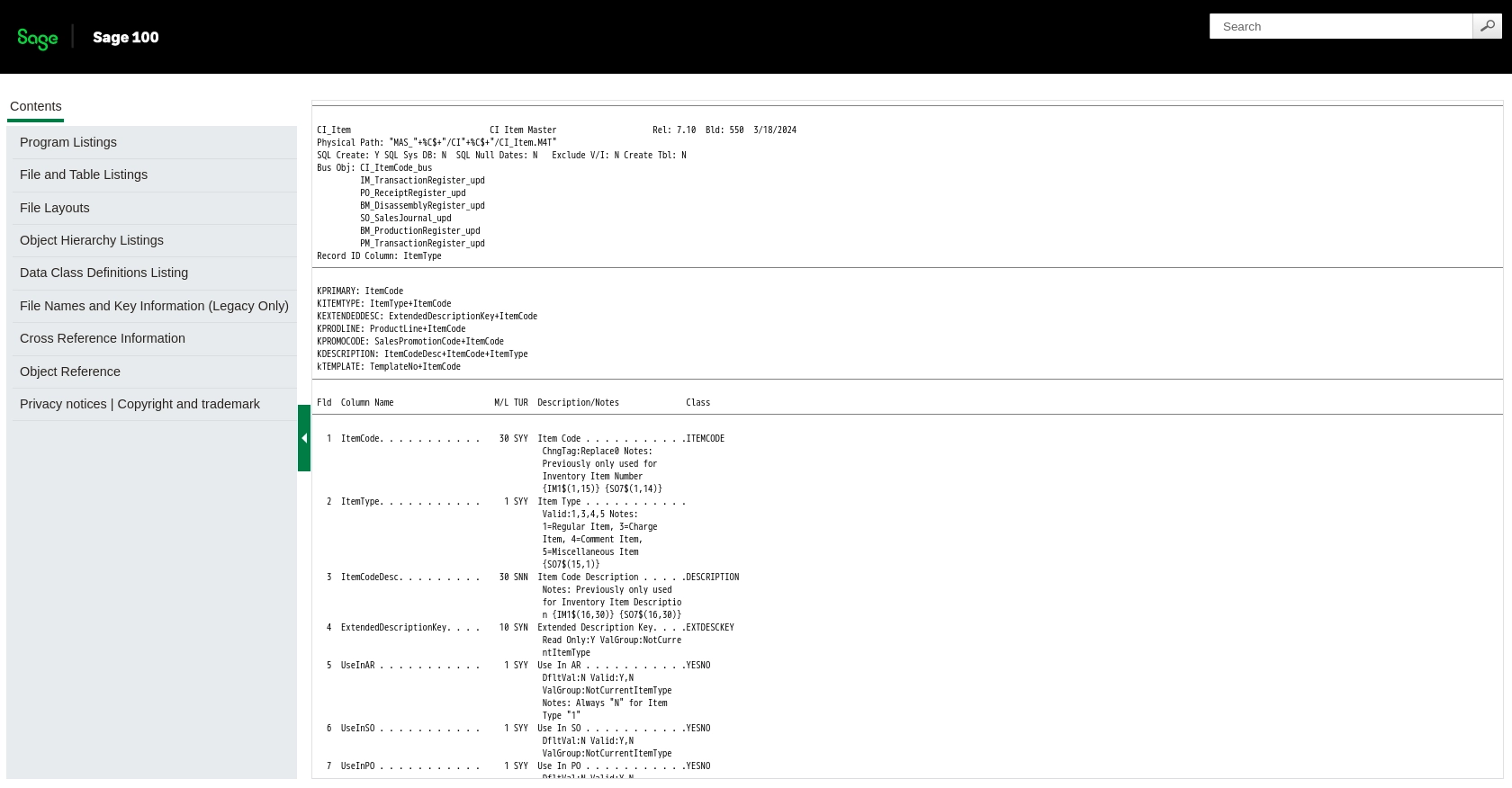
Conclusion and Best Practices for Sage 100 API Integration Using PHP
Integrating with the Sage 100 API using PHP can significantly enhance your business operations by automating inventory management and reducing manual data entry errors. By following the steps outlined in this guide, you can efficiently create or update items in Sage 100, ensuring your data remains accurate and up-to-date.
Best Practices for Secure and Efficient Sage 100 API Usage
- Secure User Credentials: Always store user credentials securely in your database. Use encryption to protect sensitive information and ensure that access is restricted to authorized personnel only.
- Handle Rate Limiting: Be mindful of any rate limits imposed by the Sage 100 API. Implement logic to handle rate limiting gracefully, such as retrying requests after a delay.
- Data Transformation and Standardization: Ensure that data fields are consistently formatted and standardized before sending them to the Sage 100 API. This helps maintain data integrity and prevents errors.
- Error Handling: Use try-catch blocks to manage exceptions and log errors for troubleshooting. This will help you quickly identify and resolve issues during API interactions.
Streamlining Integrations with Endgrate
If managing multiple integrations becomes overwhelming, consider using Endgrate to simplify the process. Endgrate allows you to build once for each use case and connect with multiple platforms, including Sage 100, through a single API endpoint. This approach saves time and resources, enabling you to focus on your core product development.
Visit Endgrate to learn more about how you can streamline your integration processes and provide an intuitive experience for your customers.
Read More
Ready to get started?