How to Create or Update Contacts with the Teamwork CRM API in PHP
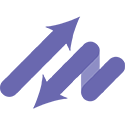
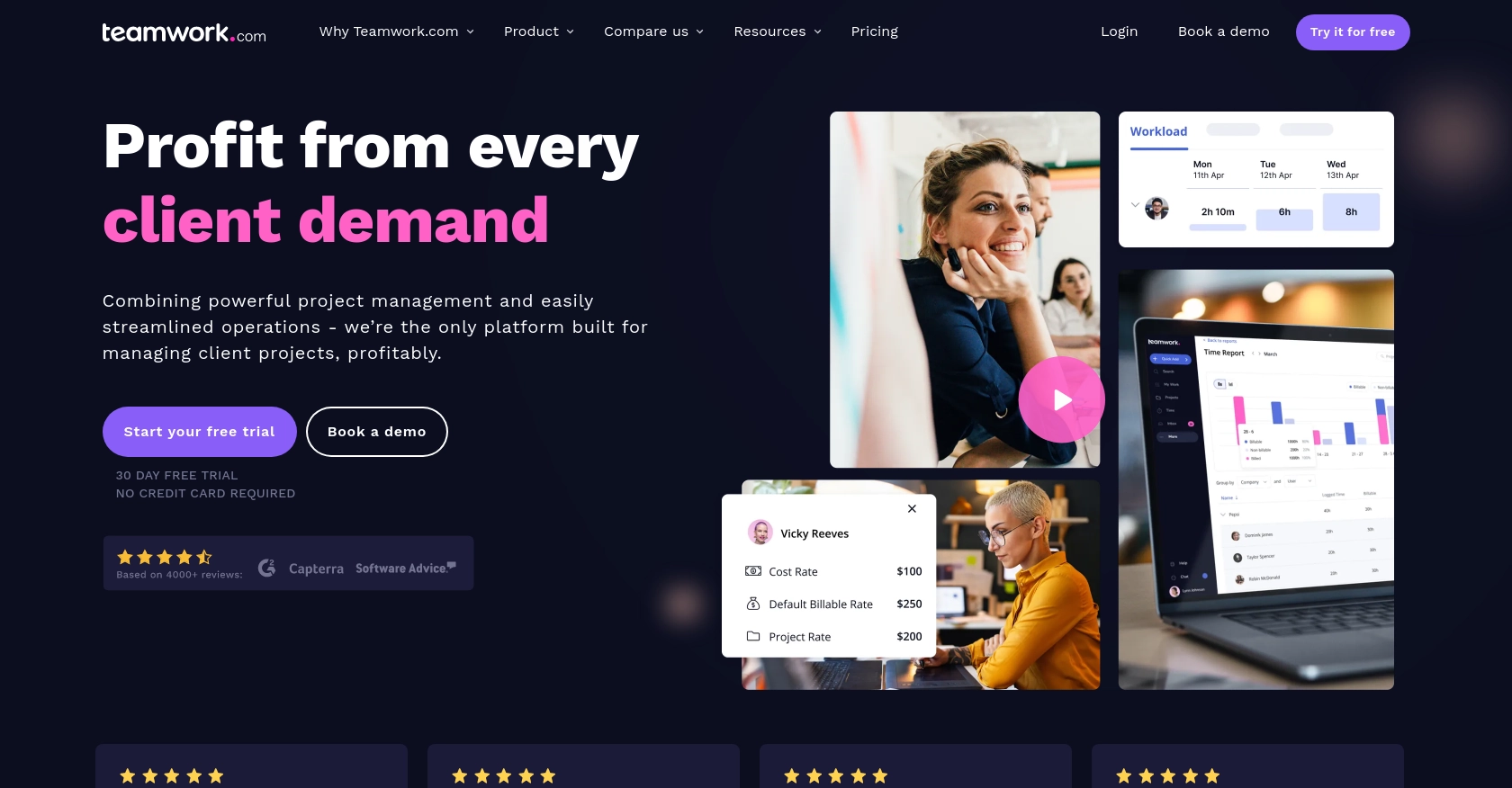
Introduction to Teamwork CRM API
Teamwork CRM is a powerful customer relationship management tool designed to help businesses manage their sales pipelines and customer interactions effectively. With its robust features, Teamwork CRM enables teams to track leads, manage contacts, and streamline communication, ensuring a seamless sales process.
Integrating with the Teamwork CRM API allows developers to automate and enhance CRM functionalities, such as creating or updating contact information programmatically. For example, a developer might want to automatically update contact details in Teamwork CRM whenever a customer fills out a form on the company's website, ensuring that the sales team always has the most up-to-date information.
Setting Up a Teamwork CRM Test or Sandbox Account
Before you can start integrating with the Teamwork CRM API, it's essential to set up a test or sandbox account. This allows you to safely experiment with API calls without affecting live data. Follow these steps to get started:
Create a Teamwork CRM Account
If you don't already have a Teamwork CRM account, you can sign up for a free trial on the Teamwork CRM website. This trial will give you access to all the features needed to test your API integrations.
Generate API Credentials for Teamwork CRM
To interact with the Teamwork CRM API, you'll need to generate API credentials. Follow these steps to obtain them:
- Log in to your Teamwork CRM account.
- Navigate to the "Settings" section from the main dashboard.
- Under "API & Webhooks," select "API Keys."
- Click on "Generate API Key" and provide a name for your key.
- Copy the generated API key and store it securely, as you'll need it for authentication in your API requests.
Authenticate Using Basic Authentication
Teamwork CRM supports Basic Authentication for API requests. Here's how to set it up:
- Encode your API key in base64 format. The format should be
{API_KEY}:password
, where "password" is left blank. - Include the encoded string in the Authorization header of your API requests as follows:
Authorization: Basic {base64_encoded_string}
Note: Ensure that all API requests are made over HTTPS to maintain security.
Testing Your API Setup
Once your API key is set up, you can test your integration by making a simple API call to retrieve existing contacts. This will confirm that your authentication is working correctly.
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, "https://{yourSiteName}.teamwork.com/crm/v2/contacts");
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_HTTPHEADER, [
"Authorization: Basic {base64_encoded_string}"
]);
$response = curl_exec($ch);
curl_close($ch);
echo $response;
Replace {yourSiteName}
with your actual Teamwork site name and {base64_encoded_string}
with your encoded API key. If successful, this call will return a list of contacts from your Teamwork CRM account.
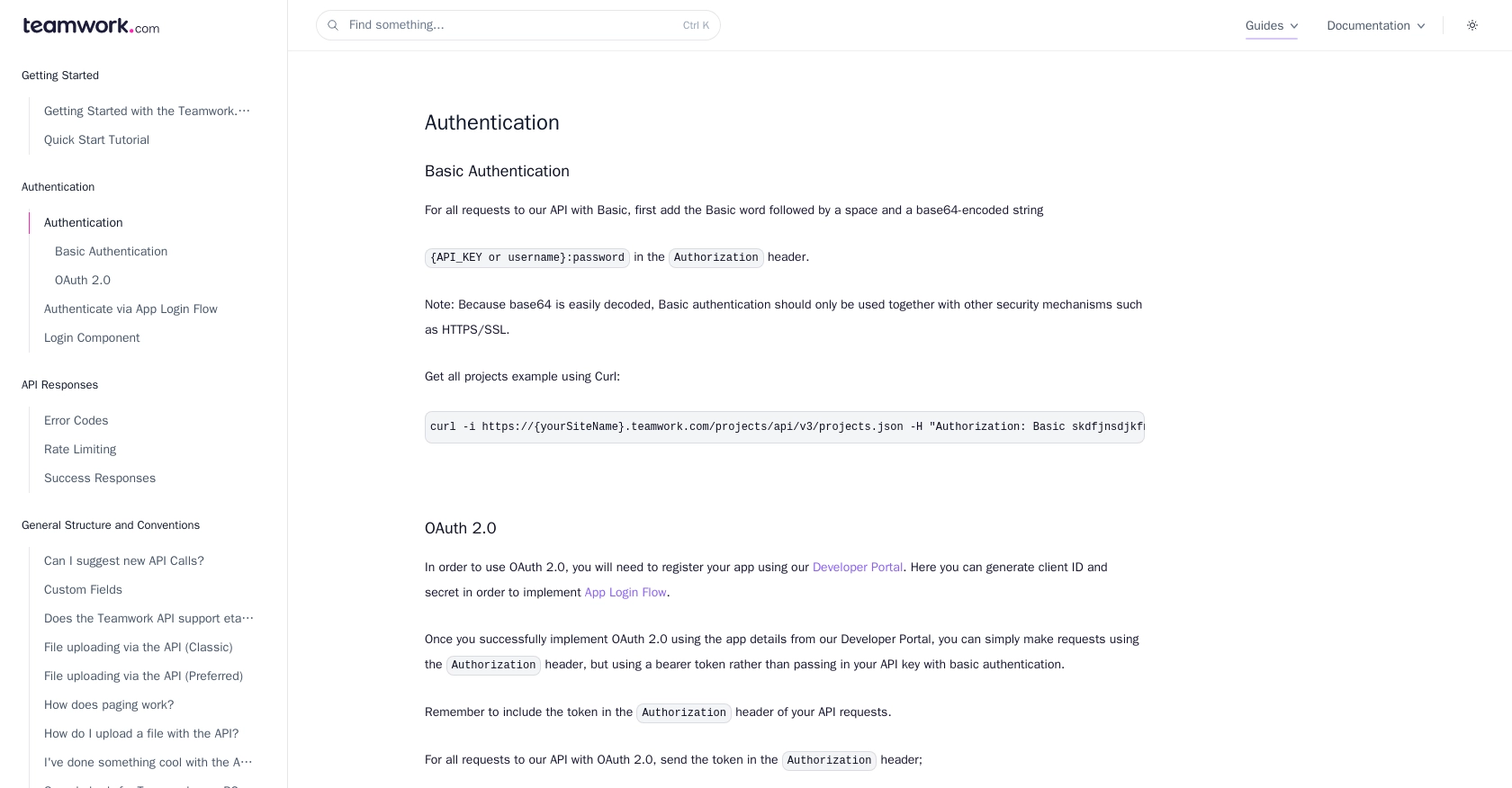
sbb-itb-96038d7
Making API Calls to Create or Update Contacts in Teamwork CRM Using PHP
To effectively interact with the Teamwork CRM API and manage contacts, you'll need to make API calls using PHP. This section will guide you through the process of creating or updating contacts, ensuring you have the necessary setup and understanding to perform these actions seamlessly.
Prerequisites for PHP Integration with Teamwork CRM API
Before diving into the code, ensure you have the following prerequisites:
- PHP 7.4 or higher installed on your machine.
- cURL extension enabled in your PHP environment.
These tools will allow you to make HTTP requests and handle responses effectively.
Creating a Contact in Teamwork CRM Using PHP
To create a new contact in Teamwork CRM, you'll need to send a POST request to the appropriate API endpoint. Here's a step-by-step guide:
$ch = curl_init();
$data = [
"contact" => [
"firstName" => "John",
"lastName" => "Doe",
"email" => "john.doe@example.com"
]
];
curl_setopt($ch, CURLOPT_URL, "https://{yourSiteName}.teamwork.com/crm/v2/contacts");
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_POST, true);
curl_setopt($ch, CURLOPT_HTTPHEADER, [
"Authorization: Basic {base64_encoded_string}",
"Content-Type: application/json"
]);
curl_setopt($ch, CURLOPT_POSTFIELDS, json_encode($data));
$response = curl_exec($ch);
curl_close($ch);
echo $response;
Replace {yourSiteName}
with your actual Teamwork site name and {base64_encoded_string}
with your encoded API key. This code sends a POST request to create a new contact with the specified details.
Updating a Contact in Teamwork CRM Using PHP
To update an existing contact, you'll need to send a PUT request. Here's how you can do it:
$ch = curl_init();
$data = [
"contact" => [
"firstName" => "Jane",
"lastName" => "Doe",
"email" => "jane.doe@example.com"
]
];
$contactId = "12345"; // Replace with the actual contact ID
curl_setopt($ch, CURLOPT_URL, "https://{yourSiteName}.teamwork.com/crm/v2/contacts/" . $contactId);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_CUSTOMREQUEST, "PUT");
curl_setopt($ch, CURLOPT_HTTPHEADER, [
"Authorization: Basic {base64_encoded_string}",
"Content-Type: application/json"
]);
curl_setopt($ch, CURLOPT_POSTFIELDS, json_encode($data));
$response = curl_exec($ch);
curl_close($ch);
echo $response;
Ensure you replace {yourSiteName}
and {base64_encoded_string}
with your site name and API key, respectively. Also, replace $contactId
with the ID of the contact you wish to update.
Handling API Responses and Errors
After making API calls, it's crucial to handle responses and potential errors. Teamwork CRM API returns various status codes:
- 200 OK: The request was successful.
- 201 Created: A new contact was successfully created.
- 400 Bad Request: The request was malformed. Check your data structure.
- 401 Unauthorized: Authentication failed. Verify your API key.
- 422 Unprocessable Entity: The data could not be processed. Ensure JSON format is correct.
Refer to the Teamwork API Error Codes documentation for more details.
Verifying API Call Success in Teamwork CRM
To confirm that your API calls have succeeded, log in to your Teamwork CRM account and check the Contacts section. Newly created or updated contacts should appear there, reflecting the changes made through your API requests.
Conclusion and Best Practices for Teamwork CRM API Integration
Integrating with the Teamwork CRM API using PHP can significantly enhance your ability to manage contacts and automate CRM tasks. By following the steps outlined in this guide, you can create and update contacts efficiently, ensuring your sales team has access to the most current information.
Best Practices for Secure and Efficient API Usage
- Secure Storage of API Credentials: Always store your API keys securely, using environment variables or a secure vault, to prevent unauthorized access.
- Handle Rate Limiting: Be mindful of the rate limits imposed by Teamwork CRM. The maximum is 150 requests per minute for most plans. Implement logic to handle HTTP 429 errors and retry requests after the specified time.
- Data Validation and Error Handling: Validate data before sending API requests and implement robust error handling to manage different response codes effectively. Refer to the Teamwork API Error Codes documentation for guidance.
- Optimize API Calls: Minimize the number of API calls by batching requests where possible and using efficient data structures.
Enhancing Integration with Endgrate
While integrating directly with Teamwork CRM API is powerful, managing multiple integrations can be complex and time-consuming. Endgrate offers a unified API solution that simplifies integration processes across various platforms, including Teamwork CRM.
By leveraging Endgrate, you can streamline your integration efforts, reduce development time, and focus on your core product. Explore how Endgrate can transform your integration strategy by visiting Endgrate's website.
Read More
Ready to get started?