How to Get Invoices with the Zoho Books API in Python
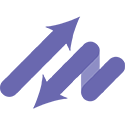
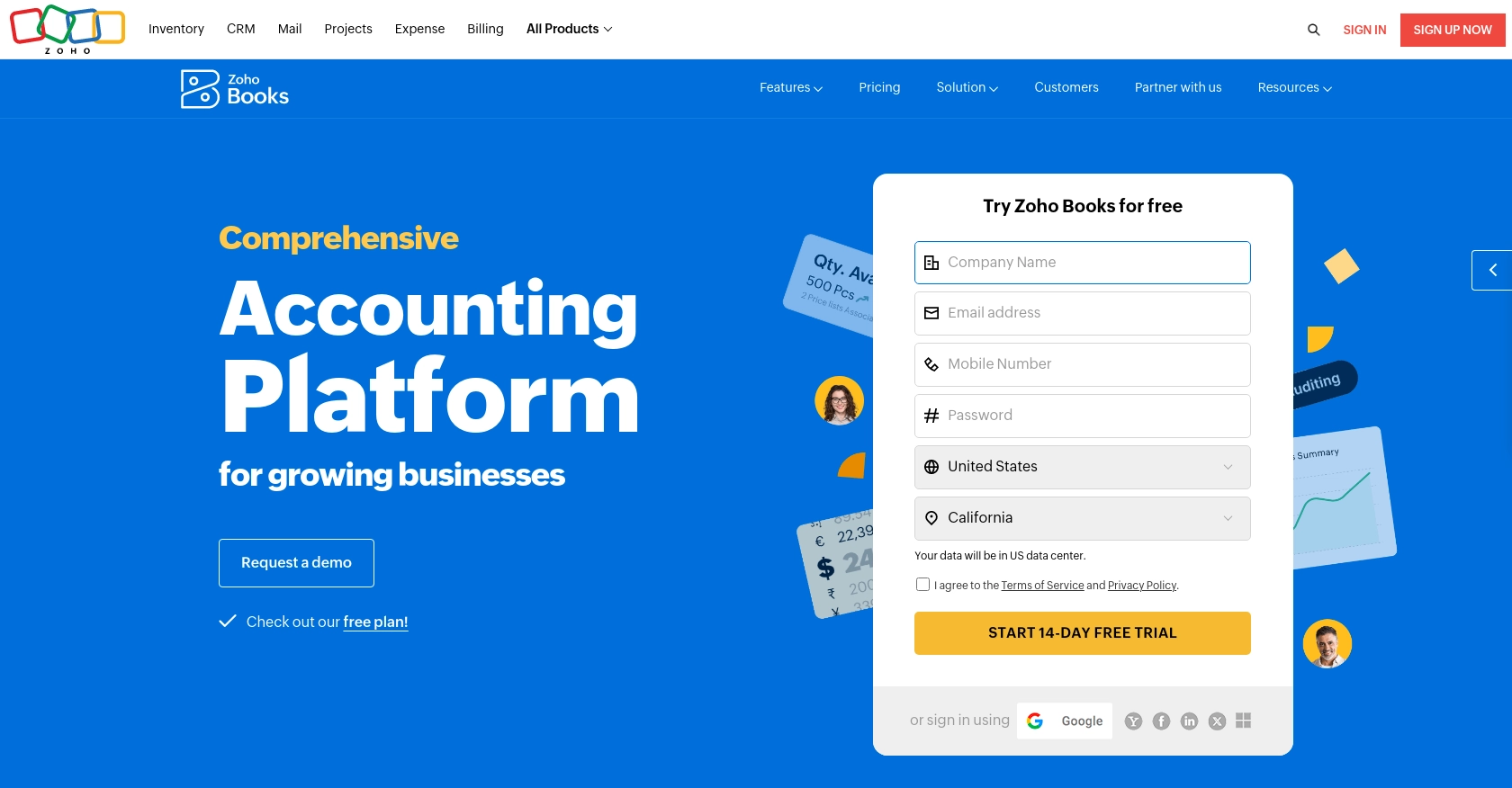
Introduction to Zoho Books API
Zoho Books is a comprehensive online accounting software that helps businesses manage their finances efficiently. It offers a wide range of features including invoicing, expense tracking, and financial reporting, making it an ideal choice for small to medium-sized enterprises.
Integrating with the Zoho Books API allows developers to automate and streamline financial operations. By accessing invoice data programmatically, businesses can enhance their financial workflows and improve accuracy. For example, a developer might use the Zoho Books API to automatically retrieve and analyze invoice data, enabling real-time financial insights and reporting.
Setting Up Your Zoho Books Test/Sandbox Account
Before you can start interacting with the Zoho Books API, you'll need to set up a test or sandbox account. This allows you to safely experiment with API calls without affecting your live data. Follow these steps to get started:
Create a Zoho Books Account
- Visit the Zoho Books signup page and create a new account. If you already have an account, simply log in.
- Once logged in, navigate to the dashboard to familiarize yourself with the interface.
Register a New Client in Zoho's Developer Console
To access the Zoho Books API, you'll need to register your application and obtain OAuth credentials:
- Go to the Zoho Developer Console.
- Click on Add Client ID and provide the necessary details to register your application.
- After registration, you'll receive a Client ID and Client Secret. Keep these credentials secure as they are essential for API authentication.
Generate OAuth Tokens for Zoho Books API Access
Zoho Books uses OAuth 2.0 for authentication. Follow these steps to generate the necessary tokens:
- Redirect to the following authorization URL, replacing the placeholders with your actual values:
https://accounts.zoho.com/oauth/v2/auth?scope=ZohoBooks.invoices.READ&client_id=YOUR_CLIENT_ID&response_type=code&redirect_uri=YOUR_REDIRECT_URI
- Upon successful authorization, you'll receive a code in the redirect URI. Use this code to request an access token:
https://accounts.zoho.com/oauth/v2/token?code=YOUR_CODE&client_id=YOUR_CLIENT_ID&client_secret=YOUR_CLIENT_SECRET&redirect_uri=YOUR_REDIRECT_URI&grant_type=authorization_code
- In the response, you'll receive an access_token and a refresh_token. The access token is used for API calls, while the refresh token is used to obtain a new access token when the current one expires.
Configure Your Zoho Books Organization
Each API request requires an organization_id. You can retrieve this by calling the organizations API:
curl -X GET 'https://www.zohoapis.com/books/v3/organizations' -H 'Authorization: Zoho-oauthtoken YOUR_ACCESS_TOKEN'
Note the organization_id from the response, as you'll need it for subsequent API calls.
With your Zoho Books account and API credentials set up, you're now ready to start making API calls to retrieve invoices and other financial data.
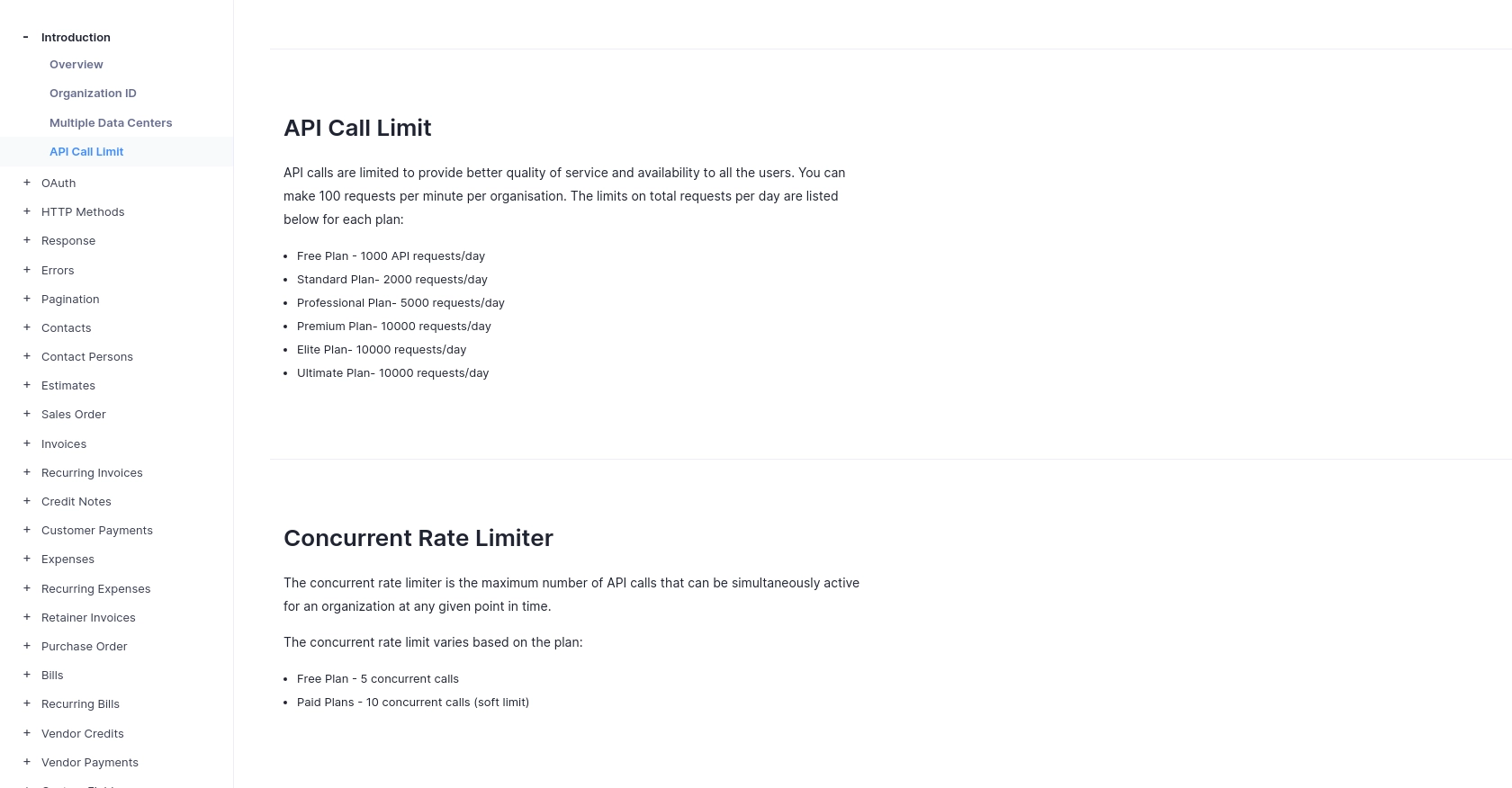
sbb-itb-96038d7
Making API Calls to Retrieve Invoices from Zoho Books Using Python
To interact with the Zoho Books API and retrieve invoices, you'll need to use Python, a versatile programming language known for its simplicity and readability. This section will guide you through the process of making API calls to Zoho Books using Python.
Prerequisites for Zoho Books API Integration with Python
Before proceeding, ensure you have the following installed on your machine:
- Python 3.11.1 or later
- The Python package installer, pip
Once you have these installed, open your terminal or command prompt and install the requests
library, which is essential for making HTTP requests:
pip install requests
Writing Python Code to Fetch Invoices from Zoho Books
Now, let's write a Python script to retrieve invoices from Zoho Books. Create a file named get_zoho_invoices.py
and add the following code:
import requests
# Set the API endpoint and headers
endpoint = "https://www.zohoapis.com/books/v3/invoices"
headers = {
"Authorization": "Zoho-oauthtoken YOUR_ACCESS_TOKEN",
"Content-Type": "application/json"
}
# Set the organization ID
params = {
"organization_id": "YOUR_ORGANIZATION_ID"
}
# Make a GET request to the API
response = requests.get(endpoint, headers=headers, params=params)
# Check if the request was successful
if response.status_code == 200:
invoices = response.json().get("invoices", [])
for invoice in invoices:
print(f"Invoice ID: {invoice['invoice_id']}, Total: {invoice['total']}")
else:
print(f"Failed to retrieve invoices: {response.status_code} - {response.text}")
Replace YOUR_ACCESS_TOKEN
and YOUR_ORGANIZATION_ID
with your actual access token and organization ID obtained during the setup process.
Running the Python Script and Verifying the Output
Run the script from your terminal or command prompt using the following command:
python get_zoho_invoices.py
If successful, you should see a list of invoices printed to the console, each displaying its ID and total amount. This confirms that your API call to Zoho Books was successful.
Handling Errors and Understanding Zoho Books API Response Codes
It's crucial to handle potential errors when making API calls. Zoho Books uses standard HTTP status codes to indicate the result of an API call:
- 200 OK: The request was successful.
- 400 Bad Request: The request was invalid or cannot be otherwise served.
- 401 Unauthorized: Authentication failed or user does not have permissions for the requested operation.
- 404 Not Found: The requested resource could not be found.
- 429 Rate Limit Exceeded: Too many requests hit the API too quickly.
- 500 Internal Server Error: An error occurred on the server.
For more details on error handling, refer to the Zoho Books API documentation.
Verifying API Call Success in Zoho Books Dashboard
After running your script, you can verify the retrieved invoices by logging into your Zoho Books account and checking the invoices section. This ensures that the data fetched matches the records in your Zoho Books account.
By following these steps, you can efficiently retrieve invoice data from Zoho Books using Python, streamlining your financial data management processes.
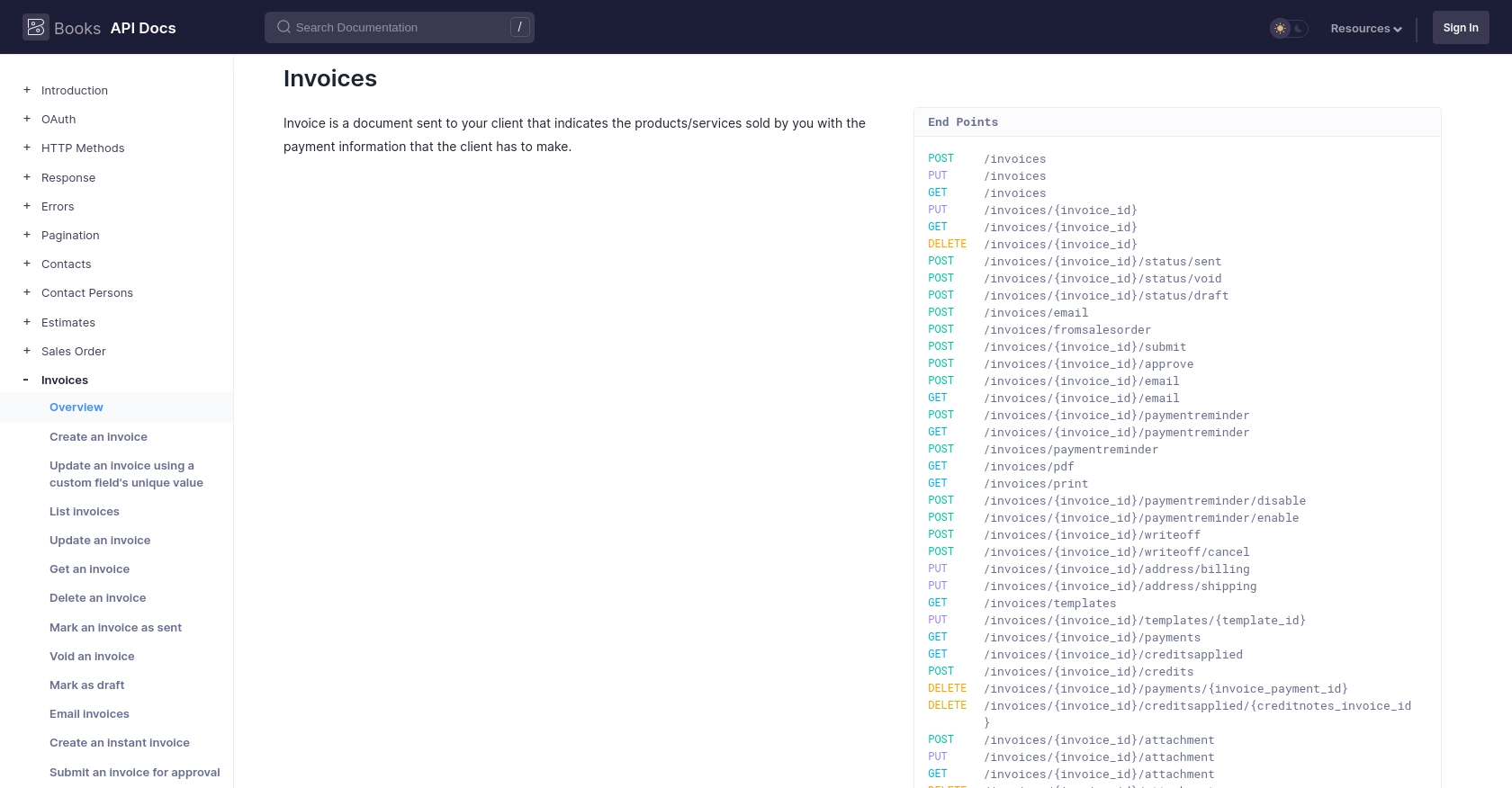
Conclusion and Best Practices for Zoho Books API Integration
Integrating with the Zoho Books API using Python offers a powerful way to automate and enhance your financial processes. By retrieving invoice data programmatically, you can streamline operations, improve accuracy, and gain real-time insights into your financial health.
Best Practices for Secure and Efficient Zoho Books API Usage
- Securely Store Credentials: Always store your OAuth credentials securely. Avoid hardcoding them in your scripts. Use environment variables or secure vaults to manage sensitive information.
- Handle Rate Limiting: Zoho Books imposes a rate limit of 100 requests per minute per organization. Implement retry logic and exponential backoff to handle rate limit errors gracefully. For more details, refer to the API call limit documentation.
- Standardize Data: Ensure that data retrieved from Zoho Books is standardized and transformed as needed for your application. This helps maintain consistency across different systems.
- Monitor API Usage: Regularly monitor your API usage and logs to identify any anomalies or issues. This proactive approach helps in maintaining a smooth integration experience.
Streamline Your Integrations with Endgrate
While integrating with Zoho Books API can be highly beneficial, it may require significant time and resources. Endgrate offers a solution by providing a unified API endpoint that connects to multiple platforms, including Zoho Books. This allows you to build once for each use case, saving time and capital, and focusing on your core product.
With Endgrate, you can offer an easy and intuitive integration experience for your customers, ensuring seamless connectivity across various platforms. Explore how Endgrate can enhance your integration strategy by visiting Endgrate.
Read More
- https://endgrate.com/provider/zohobooks
- https://www.zoho.com/books/api/v3/introduction/#api-call-limit
- https://www.zoho.com/books/api/v3/oauth/#overview
- https://www.zoho.com/books/api/v3/errors/#overview
- https://www.zoho.com/books/api/v3/pagination/#overview
- https://www.zoho.com/books/api/v3/invoices/#overview
Ready to get started?