How to Create or Update Items with the Zoho Books API in PHP
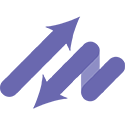
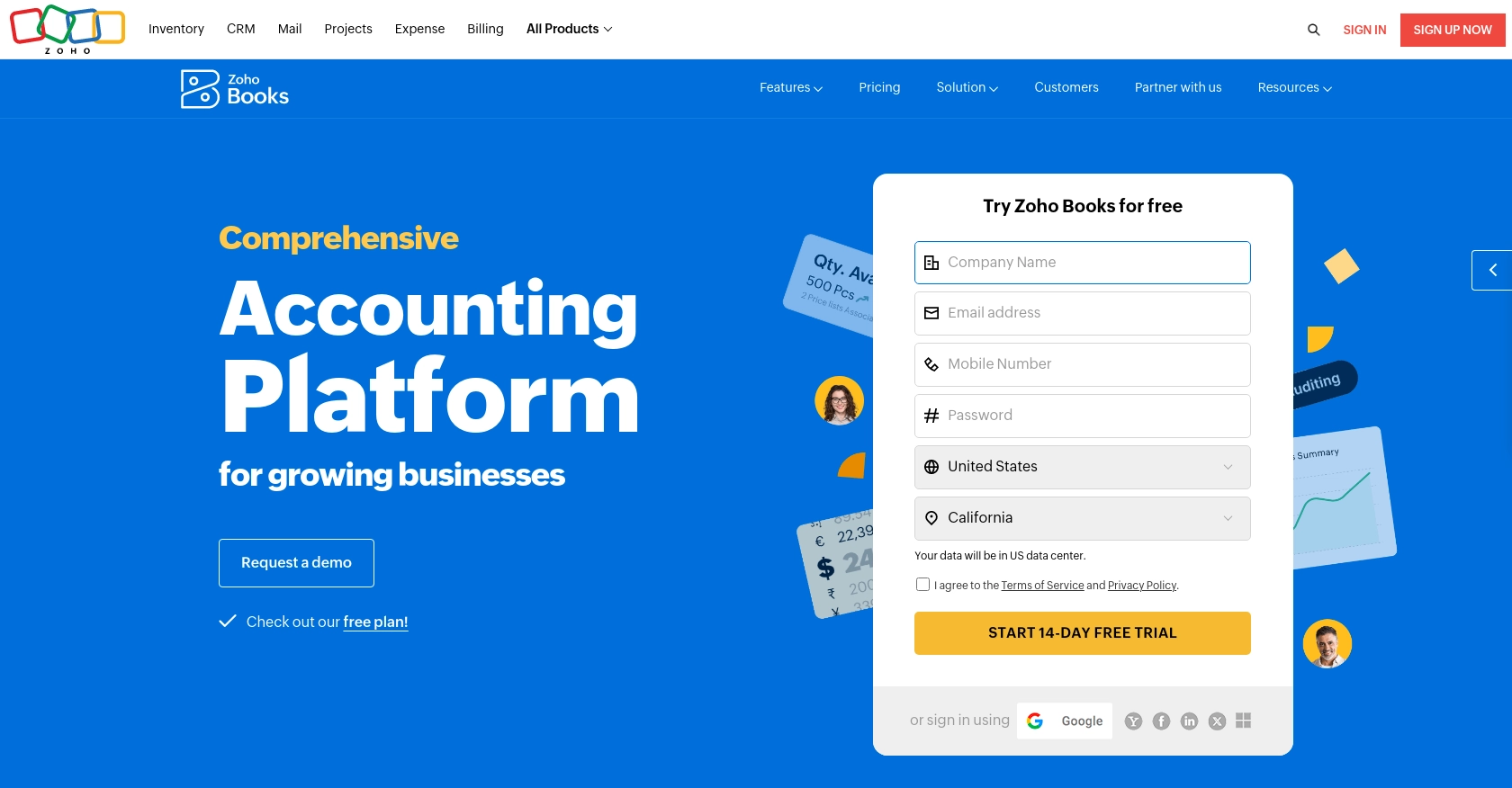
Introduction to Zoho Books API
Zoho Books is a comprehensive accounting software solution designed to simplify financial management for businesses of all sizes. It offers a wide range of features including invoicing, expense tracking, and inventory management, making it an essential tool for streamlining business operations.
Integrating with the Zoho Books API allows developers to automate and enhance various accounting processes. For example, you can create or update items in your inventory directly through the API, ensuring that your product data is always up-to-date and accurate. This is particularly useful for businesses that need to manage large inventories or frequently update product details.
Setting Up Your Zoho Books Test/Sandbox Account
Before you can start integrating with the Zoho Books API, you'll need to set up a test or sandbox account. This allows you to experiment with API calls without affecting your live data. Zoho Books offers a free trial that you can use for this purpose.
Creating a Zoho Books Account
To begin, visit the Zoho Books website and sign up for a free trial account. Follow the on-screen instructions to complete the registration process. Once your account is set up, you'll have access to the Zoho Books dashboard.
Registering a New Client in Zoho's Developer Console
Zoho Books uses OAuth 2.0 for authentication. To interact with the API, you'll need to register your application in Zoho's Developer Console to obtain your Client ID and Client Secret.
- Go to the Zoho Developer Console.
- Click on Add Client ID and fill in the required details.
- Upon successful registration, you'll receive your OAuth 2.0 credentials, including the Client ID and Client Secret. Keep these credentials secure.
Generating an OAuth Access Token
With your Client ID and Client Secret, you can now generate an OAuth access token to authenticate API requests.
- Redirect users to the following authorization URL with the necessary parameters:
- After the user grants permission, Zoho will redirect to your specified
redirect_uri
with acode
parameter. - Exchange this code for an access token by making a POST request to:
- Store the access token securely, as it will be used in the Authorization header for API requests.
https://accounts.zoho.com/oauth/v2/auth?scope=ZohoBooks.settings.CREATE,ZohoBooks.settings.UPDATE&client_id=YOUR_CLIENT_ID&response_type=code&redirect_uri=YOUR_REDIRECT_URI&access_type=offline
https://accounts.zoho.com/oauth/v2/token?code=YOUR_CODE&client_id=YOUR_CLIENT_ID&client_secret=YOUR_CLIENT_SECRET&redirect_uri=YOUR_REDIRECT_URI&grant_type=authorization_code
For more detailed information on OAuth setup, refer to the Zoho Books OAuth documentation.
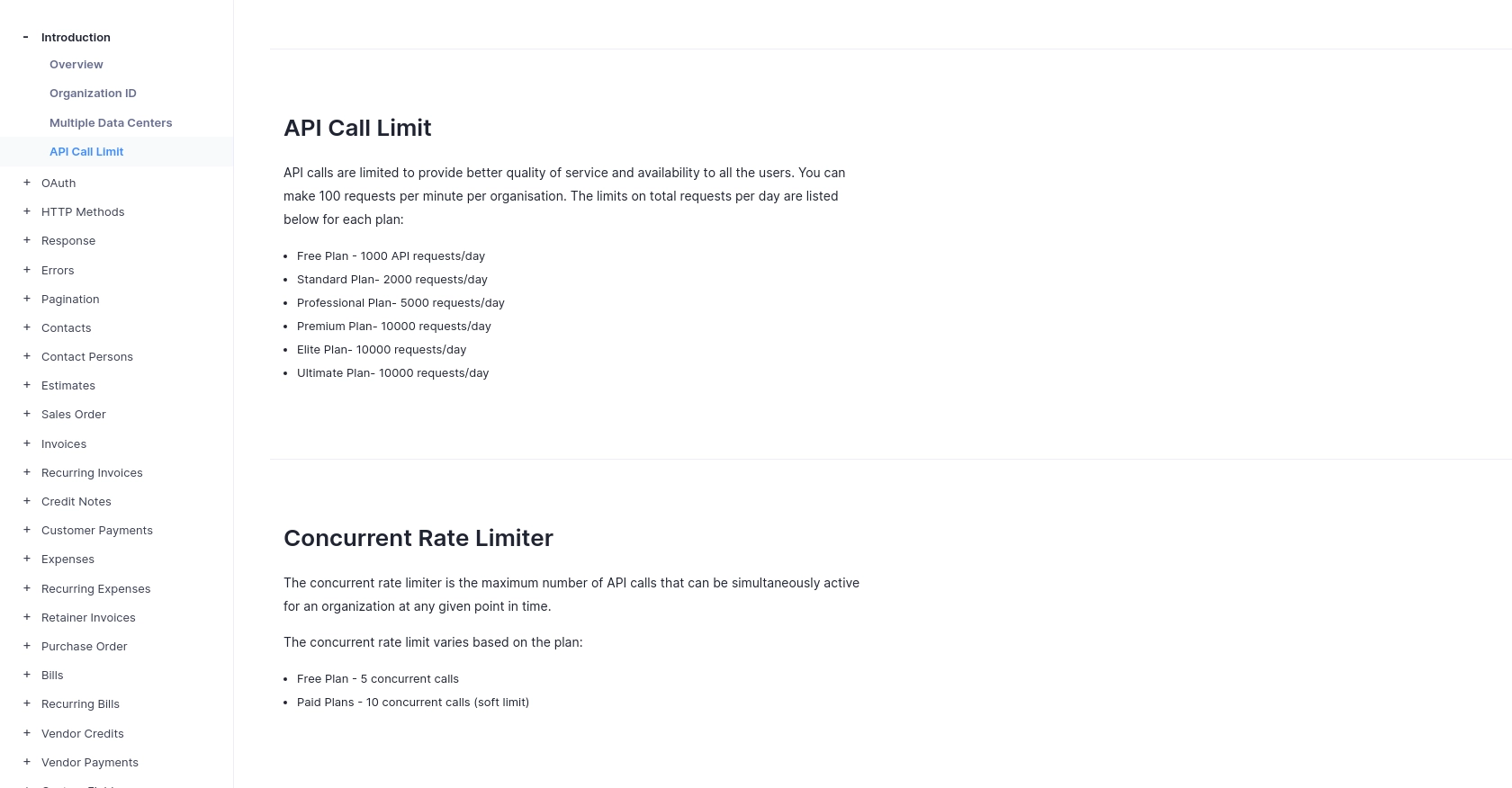
sbb-itb-96038d7
Making API Calls with Zoho Books API in PHP
To interact with the Zoho Books API using PHP, you'll need to set up your environment with the necessary tools and libraries. This section will guide you through the process of making API calls to create or update items in Zoho Books.
Setting Up Your PHP Environment
Before making API calls, ensure that you have PHP installed on your machine. You will also need the cURL
extension enabled to handle HTTP requests.
- Verify your PHP installation by running the following command in your terminal:
- Ensure the
cURL
extension is enabled by checking yourphp.ini
file or running:
php -v
php -m | grep curl
Creating an Item in Zoho Books Using PHP
To create an item in Zoho Books, you'll need to make a POST request to the API endpoint. Below is a sample PHP script to create an item:
<?php
$accessToken = 'YOUR_ACCESS_TOKEN';
$organizationId = 'YOUR_ORGANIZATION_ID';
$url = "https://www.zohoapis.com/books/v3/items?organization_id=$organizationId";
$data = [
'name' => 'Sample Item',
'rate' => 100,
'description' => 'A sample item description',
'sku' => 'SKU12345',
'product_type' => 'goods'
];
$headers = [
"Authorization: Zoho-oauthtoken $accessToken",
"Content-Type: application/json"
];
$ch = curl_init($url);
curl_setopt($ch, CURLOPT_POST, true);
curl_setopt($ch, CURLOPT_POSTFIELDS, json_encode($data));
curl_setopt($ch, CURLOPT_HTTPHEADER, $headers);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
$response = curl_exec($ch);
curl_close($ch);
echo $response;
?>
Replace YOUR_ACCESS_TOKEN
and YOUR_ORGANIZATION_ID
with your actual access token and organization ID. This script sends a POST request to create a new item in Zoho Books.
Updating an Item in Zoho Books Using PHP
To update an existing item, you'll need to make a PUT request. Here's how you can update an item using PHP:
<?php
$accessToken = 'YOUR_ACCESS_TOKEN';
$organizationId = 'YOUR_ORGANIZATION_ID';
$itemId = 'ITEM_ID_TO_UPDATE';
$url = "https://www.zohoapis.com/books/v3/items/$itemId?organization_id=$organizationId";
$data = [
'name' => 'Updated Item Name',
'rate' => 150,
'description' => 'Updated item description'
];
$headers = [
"Authorization: Zoho-oauthtoken $accessToken",
"Content-Type: application/json"
];
$ch = curl_init($url);
curl_setopt($ch, CURLOPT_CUSTOMREQUEST, 'PUT');
curl_setopt($ch, CURLOPT_POSTFIELDS, json_encode($data));
curl_setopt($ch, CURLOPT_HTTPHEADER, $headers);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
$response = curl_exec($ch);
curl_close($ch);
echo $response;
?>
Replace ITEM_ID_TO_UPDATE
with the ID of the item you wish to update. This script sends a PUT request to update the item's details in Zoho Books.
Handling API Responses and Errors
After making API calls, it's crucial to handle the responses and any potential errors. Zoho Books API uses HTTP status codes to indicate success or failure:
- 200 OK: The request was successful.
- 201 Created: The item was successfully created.
- 400 Bad Request: The request was invalid.
- 401 Unauthorized: Authentication failed.
- 429 Rate Limit Exceeded: Too many requests were made.
For more details on error handling, refer to the Zoho Books Error Documentation.
By following these steps, you can efficiently create and update items in Zoho Books using PHP, ensuring your inventory data remains accurate and up-to-date.
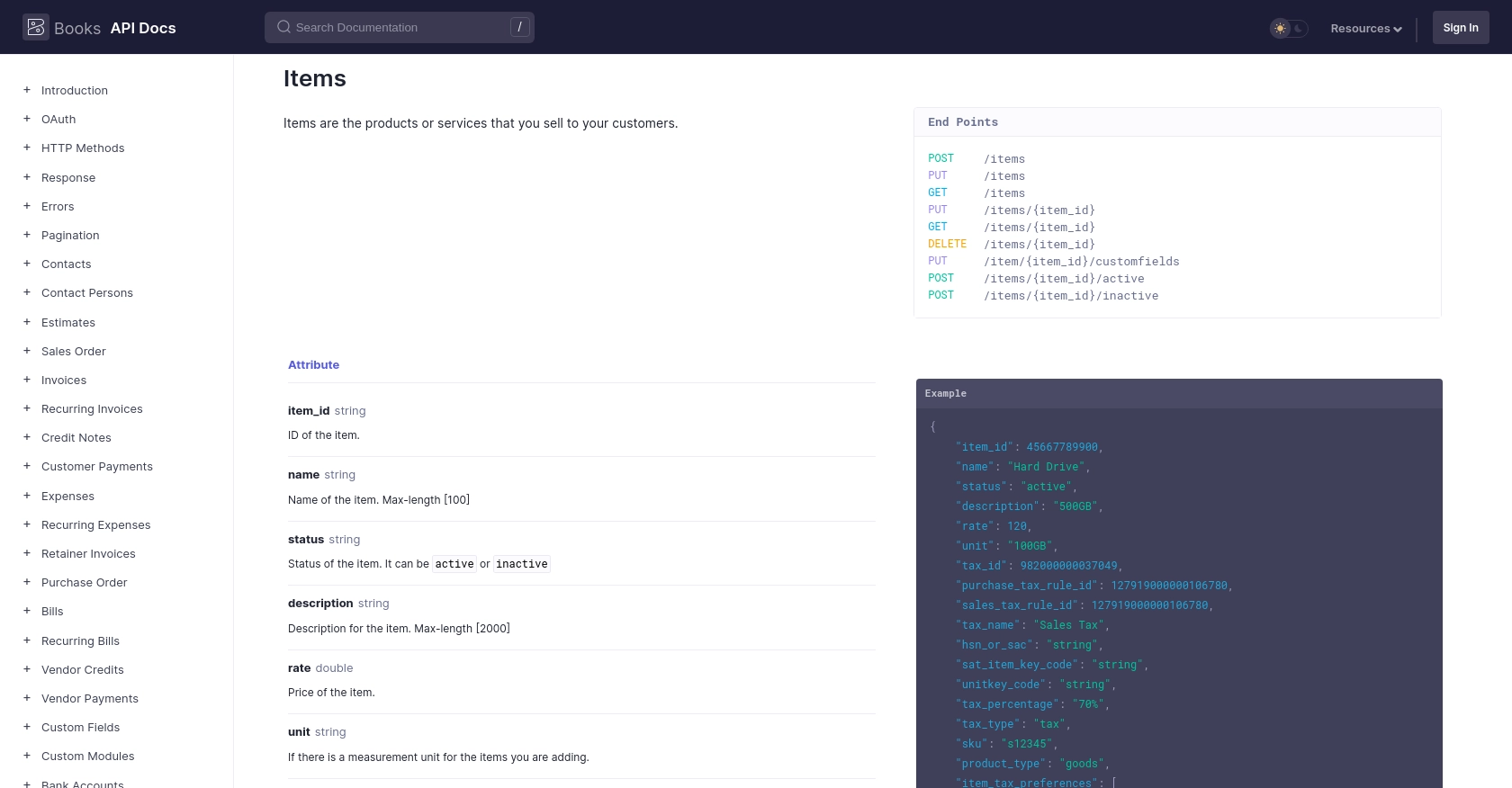
Conclusion and Best Practices for Zoho Books API Integration
Integrating with the Zoho Books API using PHP allows developers to automate and streamline inventory management processes, ensuring that product data is consistently accurate and up-to-date. By following the steps outlined in this guide, you can efficiently create and update items in Zoho Books, enhancing your business operations.
Best Practices for Secure and Efficient API Integration
- Securely Store Credentials: Always store your OAuth credentials, including the access token, securely. Avoid hardcoding them in your application code.
- Handle Rate Limiting: Zoho Books API has a rate limit of 100 requests per minute per organization. Implement logic to handle rate limiting gracefully to avoid disruptions. For more information, refer to the Zoho Books API Call Limit Documentation.
- Standardize Data Fields: Ensure that your data fields are standardized and consistent with Zoho Books' requirements to prevent errors during API calls.
- Implement Error Handling: Use appropriate error handling to manage API responses effectively. Refer to the Zoho Books Error Documentation for guidance on handling different error codes.
Enhance Your Integration Experience with Endgrate
For developers looking to simplify and enhance their integration processes, consider using Endgrate. With Endgrate, you can save time and resources by outsourcing integrations, allowing you to focus on your core product. Build once for each use case and enjoy an intuitive integration experience for your customers. Learn more about how Endgrate can streamline your integration efforts by visiting Endgrate.
By adhering to these best practices and leveraging tools like Endgrate, you can ensure a robust and efficient integration with Zoho Books, empowering your business with seamless accounting and inventory management capabilities.
Read More
- https://endgrate.com/provider/zohobooks
- https://www.zoho.com/books/api/v3/introduction/#api-call-limit
- https://www.zoho.com/books/api/v3/oauth/#overview
- https://www.zoho.com/books/api/v3/errors/#overview
- https://www.zoho.com/books/api/v3/pagination/#overview
- https://www.zoho.com/books/api/v3/items/#overview
Ready to get started?