How to Create or Update People with the Pipedrive API in Python
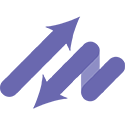
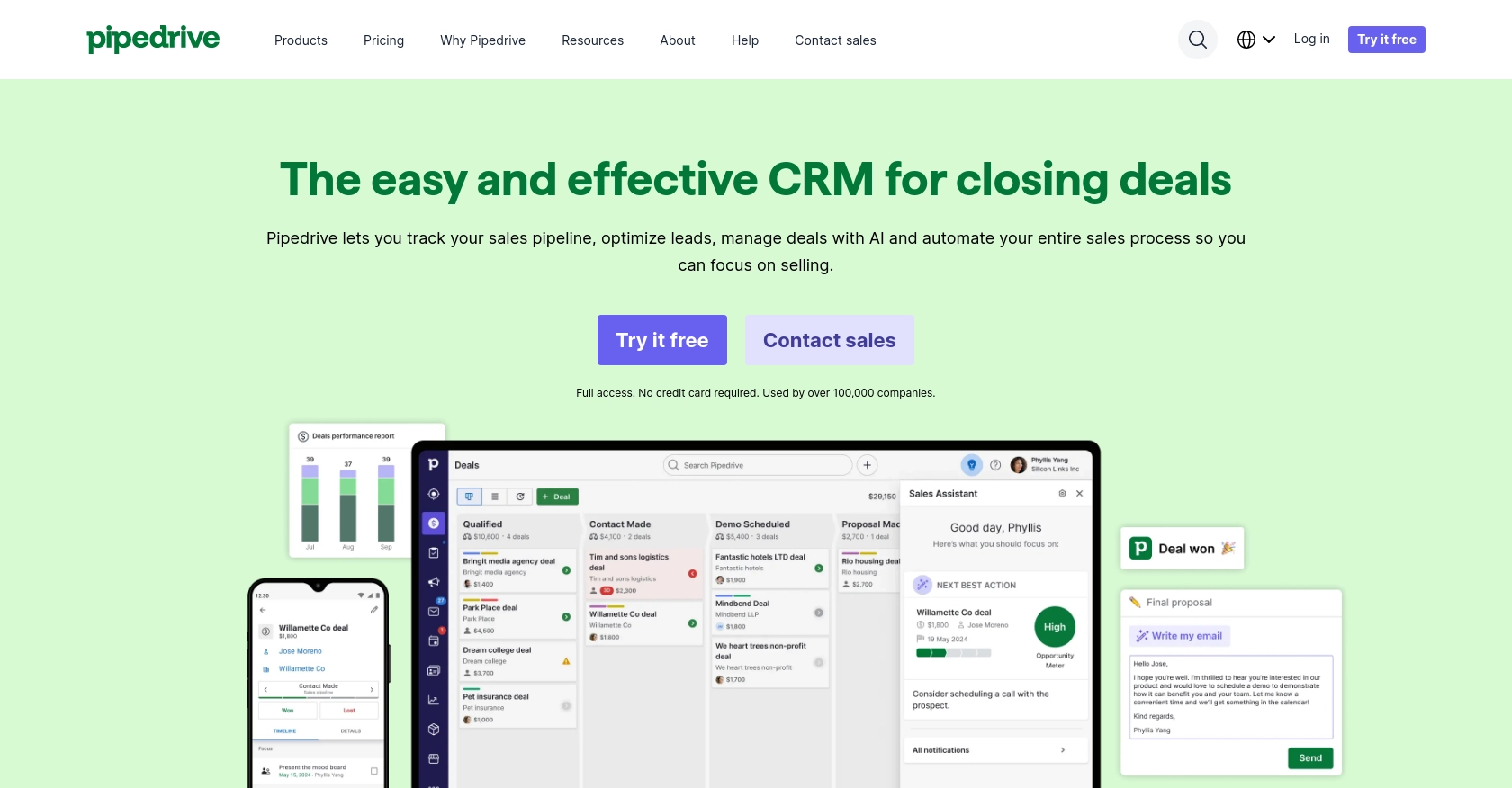
Introduction to Pipedrive CRM
Pipedrive is a powerful sales CRM platform designed to help businesses manage their sales processes more effectively. With its intuitive interface and robust features, Pipedrive enables sales teams to track leads, manage customer relationships, and close deals efficiently.
Developers often integrate with Pipedrive's API to automate and enhance their sales workflows. For example, using the Pipedrive API, a developer can create or update contact information seamlessly within the CRM, ensuring that sales teams have access to the most current data. This integration can streamline sales operations and improve data accuracy across platforms.
Setting Up Your Pipedrive Developer Sandbox Account
Before diving into the integration process with Pipedrive's API, it's essential to set up a developer sandbox account. This environment allows you to test and develop your applications without affecting live data, providing a risk-free space to experiment and refine your integration.
Creating a Pipedrive Developer Sandbox Account
To begin, you'll need to create a developer sandbox account. This account functions similarly to a regular Pipedrive company account but is specifically designed for development and testing purposes. Follow these steps to set up your sandbox account:
- Visit the Pipedrive Developer Sandbox Account page.
- Fill out the form to request access to a sandbox account. This will grant you access to a safe development environment with sample data.
- Once your account is approved, log in to the Pipedrive Developer Hub to manage your sandbox account and start building your integration.
Generating OAuth Credentials for Pipedrive API
Pipedrive uses OAuth 2.0 for authentication, which requires you to create an app to obtain the necessary credentials. Here's how to set up OAuth for your sandbox account:
- Navigate to the Developer Hub within your sandbox account.
- Register your app by providing the required details, such as the app name and description.
- Once registered, you'll receive a Client ID and Client Secret. These credentials are crucial for implementing the OAuth flow.
- Configure the redirect URI and scopes according to your application's needs.
Ensure you store these credentials securely, as they will be used to authenticate API requests.
Importing Sample Data into Your Pipedrive Sandbox
To effectively test your integration, you can import sample data into your sandbox account:
- Go to the Pipedrive web app and click on the “...” (More) menu.
- Select Import data and choose From a spreadsheet.
- Upload the template spreadsheet provided by Pipedrive to populate your sandbox with sample data.
This sample data will help you simulate real-world scenarios and validate your integration's functionality.
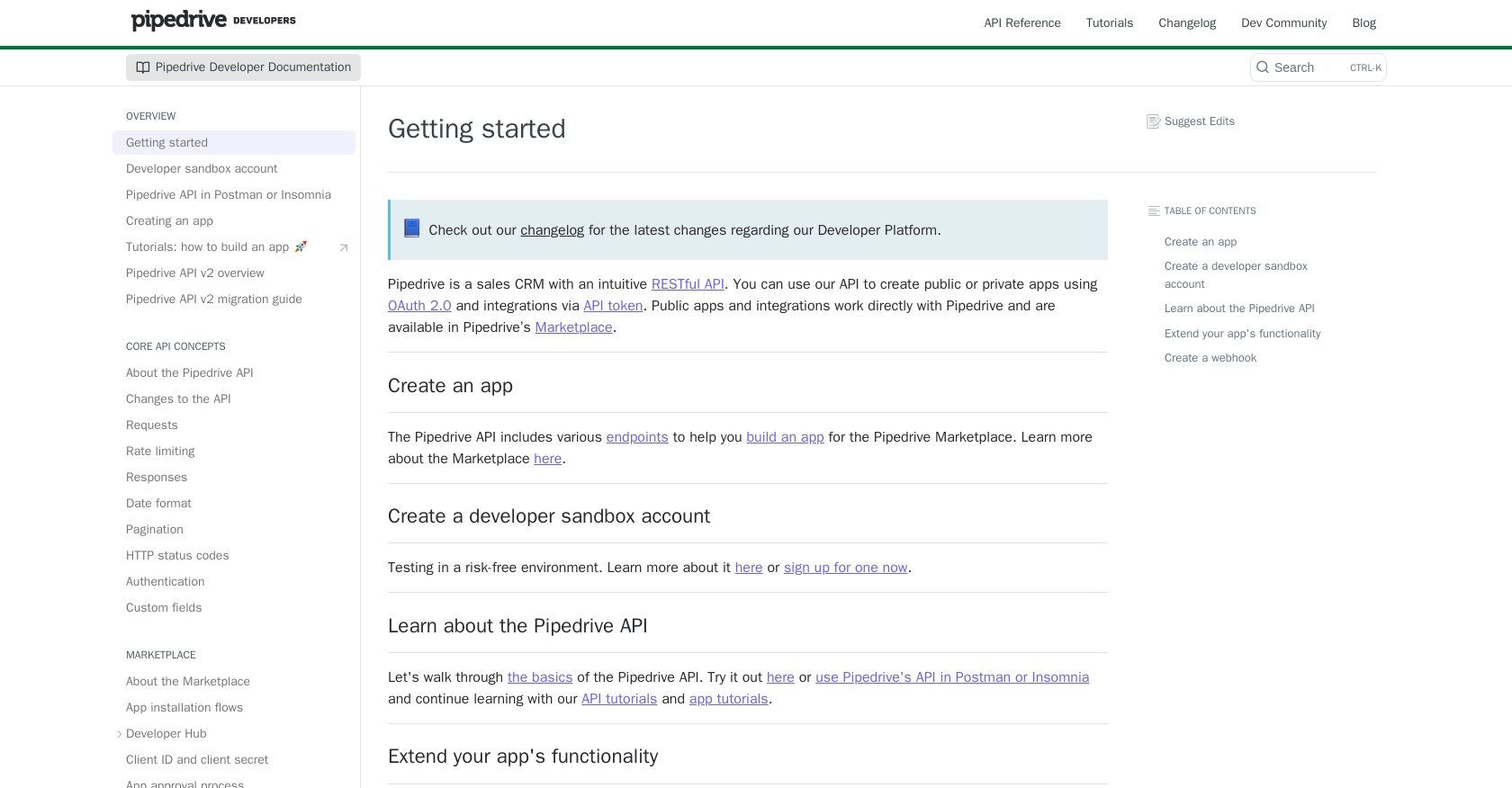
sbb-itb-96038d7
How to Make API Calls to Create or Update People in Pipedrive Using Python
Setting Up Your Python Environment for Pipedrive API Integration
Before you start making API calls to Pipedrive, ensure your Python environment is correctly set up. You'll need Python 3.11.1 and the requests
library to handle HTTP requests. Install the necessary dependencies using the following command:
pip install requests
Creating a New Person in Pipedrive Using Python
To create a new person in Pipedrive, you'll use the POST /v1/persons
endpoint. This allows you to add new contacts to your Pipedrive CRM. Here's a step-by-step guide:
- Import the
requests
library. - Set the API endpoint URL to
https://api.pipedrive.com/v1/persons
. - Prepare the headers with your OAuth token for authentication.
- Define the person data you want to create, such as name, email, and phone.
- Make a POST request to the API endpoint with the headers and data.
import requests
url = "https://api.pipedrive.com/v1/persons"
headers = {
"Authorization": "Bearer Your_Access_Token",
"Content-Type": "application/json"
}
data = {
"name": "John Doe",
"email": [{"value": "john.doe@example.com", "primary": "true"}],
"phone": [{"value": "1234567890", "primary": "true"}]
}
response = requests.post(url, json=data, headers=headers)
if response.status_code == 201:
print("Person created successfully:", response.json())
else:
print("Failed to create person:", response.json())
Replace Your_Access_Token
with your actual OAuth token. If successful, the response will include the details of the newly created person.
Updating an Existing Person in Pipedrive Using Python
To update an existing person, use the PUT /v1/persons/{id}
endpoint. This allows you to modify contact details in your Pipedrive CRM. Follow these steps:
- Import the
requests
library. - Set the API endpoint URL, replacing
{id}
with the person's ID. - Prepare the headers with your OAuth token for authentication.
- Define the updated data for the person, such as a new email or phone number.
- Make a PUT request to the API endpoint with the headers and data.
import requests
person_id = "12345" # Replace with the actual person ID
url = f"https://api.pipedrive.com/v1/persons/{person_id}"
headers = {
"Authorization": "Bearer Your_Access_Token",
"Content-Type": "application/json"
}
data = {
"email": [{"value": "new.email@example.com", "primary": "true"}]
}
response = requests.put(url, json=data, headers=headers)
if response.status_code == 200:
print("Person updated successfully:", response.json())
else:
print("Failed to update person:", response.json())
Ensure you replace Your_Access_Token
and person_id
with your actual OAuth token and the person's ID. A successful response will confirm the update.
Handling API Response and Errors in Pipedrive Integration
It's crucial to handle API responses and potential errors effectively. Pipedrive's API returns various HTTP status codes:
- 200 OK: Request fulfilled successfully.
- 201 Created: New resource created successfully.
- 400 Bad Request: Request not understood by the server.
- 401 Unauthorized: Invalid API token.
- 429 Too Many Requests: Rate limit exceeded.
For more details on handling errors, refer to the Pipedrive HTTP Status Codes documentation.
Verifying API Requests in Pipedrive Sandbox
After making API calls, verify the changes in your Pipedrive sandbox account. Check if the new person appears in your contacts or if updates reflect correctly. This ensures your integration works as expected before deploying it to a live environment.
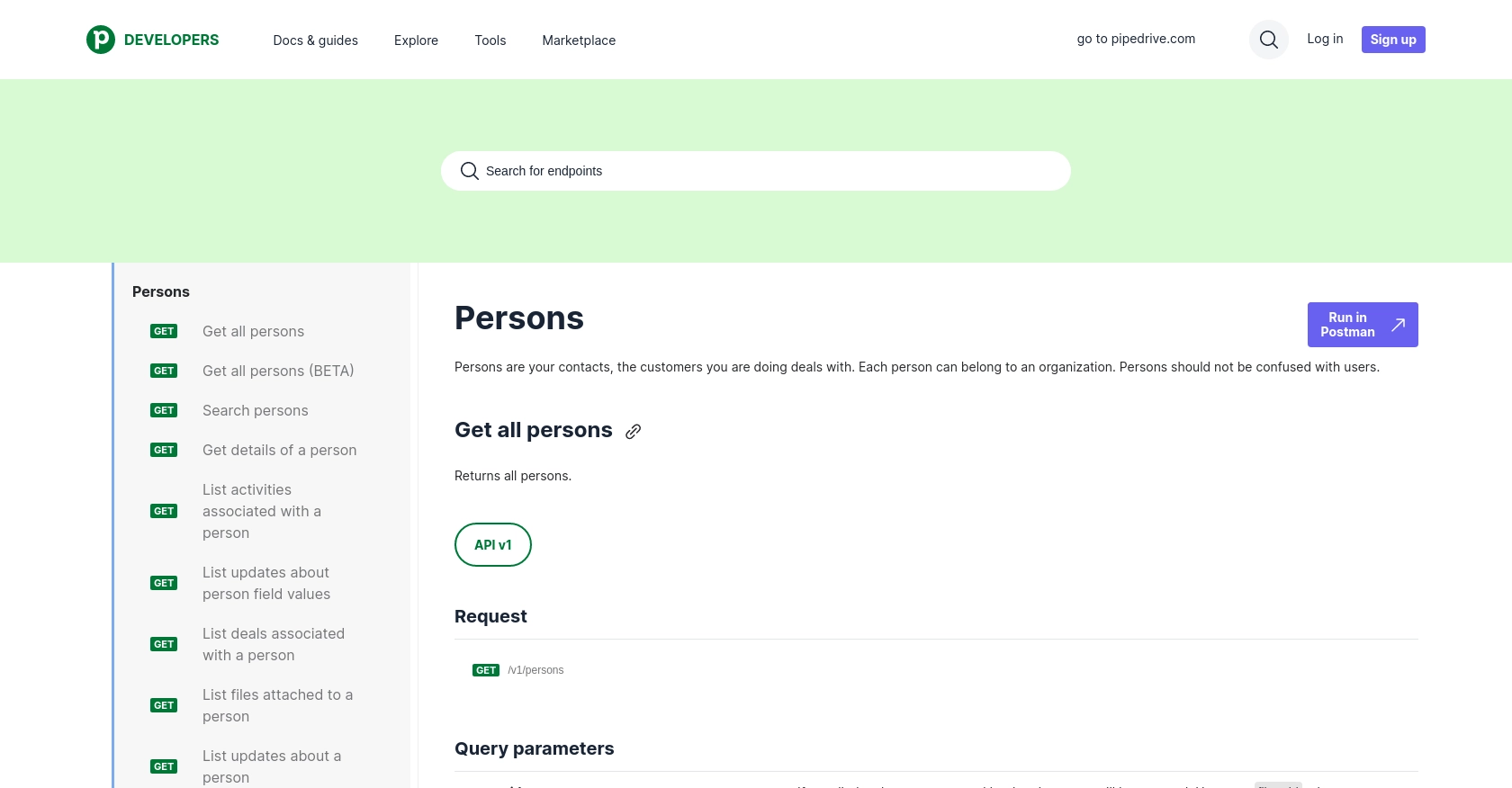
Conclusion and Best Practices for Pipedrive API Integration
Integrating with the Pipedrive API using Python can significantly enhance your sales operations by automating the management of contacts and ensuring data consistency across platforms. By following the steps outlined in this guide, you can efficiently create and update people in Pipedrive, streamlining your CRM processes.
Best Practices for Secure and Efficient Pipedrive API Usage
- Secure Storage of OAuth Credentials: Always store your Client ID, Client Secret, and OAuth tokens securely. Avoid hardcoding them in your source code and consider using environment variables or secure vaults.
- Handle Rate Limiting: Pipedrive's API has rate limits based on your plan. For OAuth apps, the limit is up to 480 requests per 2 seconds for Enterprise plans. Implement logic to handle HTTP 429 errors and consider using exponential backoff strategies. For more details, refer to the Pipedrive Rate Limiting documentation.
- Data Transformation and Standardization: Ensure that data fields are standardized across your systems to maintain consistency. This includes handling custom fields and ensuring data types match Pipedrive's requirements.
Leverage Endgrate for Simplified Integration Management
While integrating with Pipedrive directly offers flexibility, managing multiple integrations can become complex and time-consuming. Endgrate provides a unified API endpoint that simplifies integration management across various platforms, including Pipedrive. By using Endgrate, you can focus on your core product development while outsourcing the intricacies of integration management.
Explore how Endgrate can streamline your integration processes by visiting Endgrate's website. Save time and resources, and deliver a seamless integration experience for your customers.
Read More
- https://endgrate.com/provider/pipedrive
- https://pipedrive.readme.io/docs/getting-started
- https://pipedrive.readme.io/docs/developer-sandbox-account
- https://pipedrive.readme.io/docs/marketplace-creating-a-proper-app
- https://pipedrive.readme.io/docs/core-api-concepts-rate-limiting
- https://pipedrive.readme.io/docs/core-api-concepts-pagination
- https://pipedrive.readme.io/docs/core-api-concepts-http-status-codes
- https://pipedrive.readme.io/docs/core-api-concepts-custom-fields
- https://developers.pipedrive.com/docs/api/v1/Persons
- https://developers.pipedrive.com/docs/api/v1/PersonFields
Ready to get started?