How to Create or Update Products with the Pipedrive API in PHP
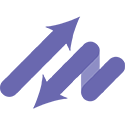
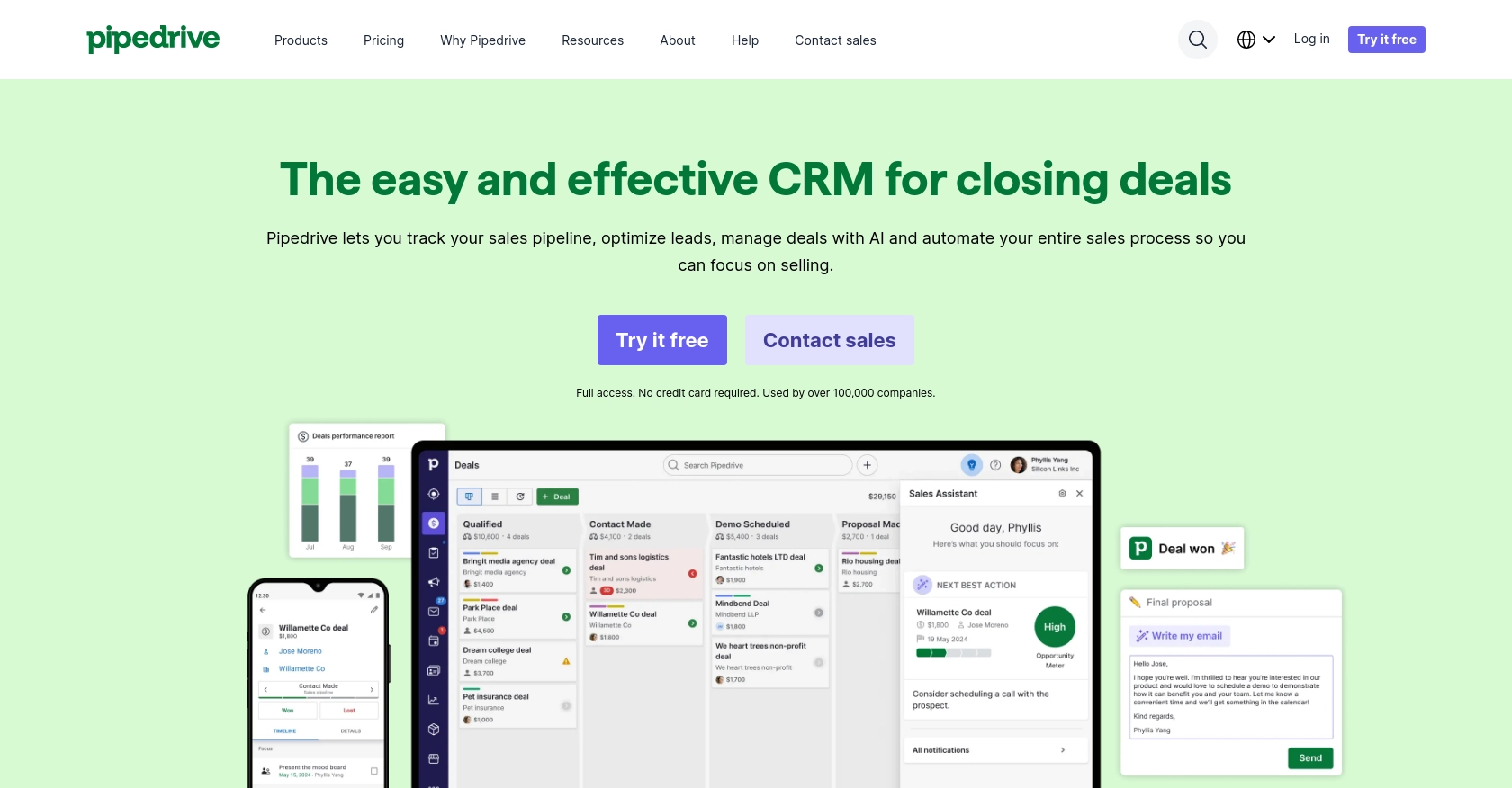
Introduction to Pipedrive CRM and API Integration
Pipedrive is a powerful sales CRM platform designed to help businesses manage their sales processes efficiently. Known for its intuitive interface and robust features, Pipedrive enables sales teams to track leads, manage sales pipelines, and close deals effectively.
Integrating with Pipedrive's API allows developers to automate and enhance various sales operations. For example, using the Pipedrive API, developers can create or update product information directly from their applications, ensuring that sales teams have access to the most current product data without manual entry.
This article will guide you through the process of creating or updating products in Pipedrive using PHP, providing you with the necessary steps and code examples to streamline your integration efforts.
Setting Up Your Pipedrive Developer Sandbox Account
Before you can start integrating with the Pipedrive API, you'll need to set up a developer sandbox account. This environment allows you to test and develop your application without affecting live data, providing a risk-free space to experiment with API calls.
Creating a Pipedrive Developer Sandbox Account
To begin, you'll need to request access to a Pipedrive developer sandbox account. This account functions similarly to a regular Pipedrive account but is designed specifically for development and testing purposes.
- Visit the Pipedrive Developer Sandbox Account page.
- Fill out the form to request access to your sandbox account.
- Once your request is approved, you'll receive an email with instructions on how to access your sandbox environment.
Creating a Pipedrive App for OAuth Authentication
Since the Pipedrive API uses OAuth 2.0 for authentication, you'll need to create an app in the Pipedrive Developer Hub to obtain your client ID and client secret.
- Log in to your Pipedrive sandbox account.
- Navigate to the Developer Hub and select "Create an App."
- Provide the necessary details for your app, such as the app name and description.
- Ensure your app uses OAuth 2.0 by setting up the appropriate authorization scopes and redirect URIs.
- After creating the app, note down the client ID and client secret, as you'll need these for authentication.
With your sandbox account and app set up, you're ready to start making API calls to Pipedrive. This setup ensures that you can safely test your integration without impacting any real data.
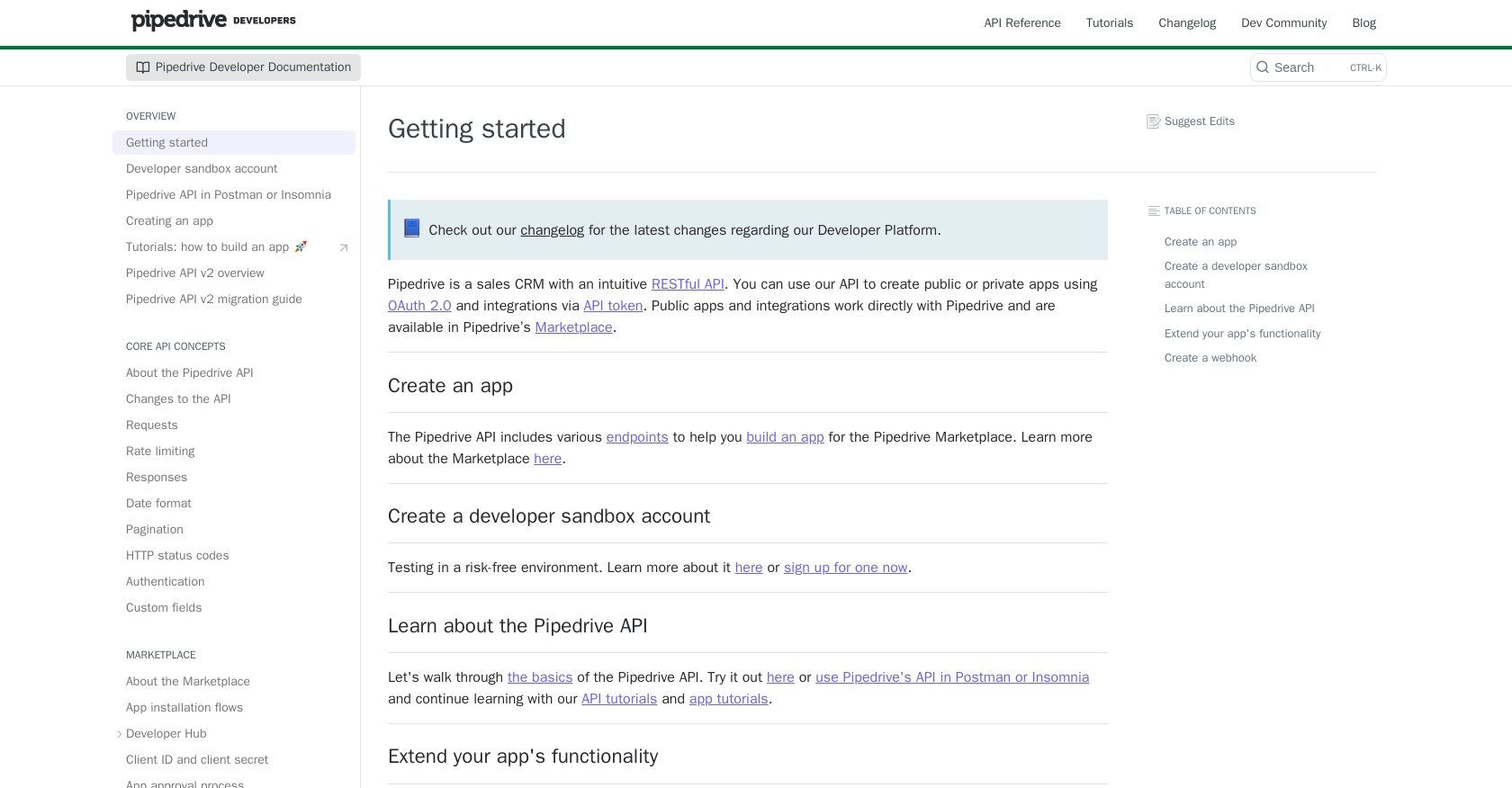
sbb-itb-96038d7
Making API Calls to Create or Update Products in Pipedrive Using PHP
With your Pipedrive developer sandbox account and app set up, you can now proceed to make API calls to create or update products. This section will guide you through the process using PHP, ensuring you have the right tools and code to interact with the Pipedrive API effectively.
Setting Up Your PHP Environment for Pipedrive API Integration
Before making API calls, ensure your PHP environment is correctly configured. You'll need the following:
- PHP 7.4 or higher
- cURL extension enabled
To verify that cURL is enabled, you can create a PHP file with the following content and run it:
<?php
phpinfo();
?>
Look for the cURL section in the output to confirm it's enabled.
Installing Required PHP Libraries for Pipedrive API
To simplify HTTP requests, you can use the Guzzle HTTP client. Install it via Composer:
composer require guzzlehttp/guzzle
Creating a New Product in Pipedrive Using PHP
To create a new product, you'll need to make a POST request to the Pipedrive API. Here's a sample PHP script to add a product:
<?php
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$accessToken = 'Your_Access_Token';
$response = $client->post('https://api.pipedrive.com/v1/products', [
'headers' => [
'Authorization' => 'Bearer ' . $accessToken,
'Content-Type' => 'application/json'
],
'json' => [
'name' => 'New Product',
'code' => 'NP123',
'description' => 'This is a new product.',
'unit' => 'piece',
'tax' => 10
]
]);
$data = json_decode($response->getBody(), true);
if ($data['success']) {
echo 'Product created successfully: ' . $data['data']['id'];
} else {
echo 'Failed to create product: ' . $data['error'];
}
?>
Replace Your_Access_Token
with the access token obtained during the OAuth setup. This script sends a POST request to create a new product with specified details.
Updating an Existing Product in Pipedrive Using PHP
To update an existing product, use a PATCH request. Here's how you can update a product:
<?php
$productId = 'Existing_Product_ID';
$response = $client->patch('https://api.pipedrive.com/v1/products/' . $productId, [
'headers' => [
'Authorization' => 'Bearer ' . $accessToken,
'Content-Type' => 'application/json'
],
'json' => [
'name' => 'Updated Product Name',
'description' => 'Updated description.'
]
]);
$data = json_decode($response->getBody(), true);
if ($data['success']) {
echo 'Product updated successfully: ' . $data['data']['id'];
} else {
echo 'Failed to update product: ' . $data['error'];
}
?>
Replace Existing_Product_ID
with the ID of the product you wish to update. This script modifies the product's name and description.
Handling API Responses and Errors
After making API calls, it's crucial to handle responses and potential errors. The Pipedrive API returns various HTTP status codes to indicate success or failure:
- 200 OK: Request was successful.
- 201 Created: Resource was successfully created.
- 400 Bad Request: Request was invalid.
- 401 Unauthorized: Authentication failed.
- 429 Too Many Requests: Rate limit exceeded.
Ensure your application checks these status codes and handles errors gracefully, such as retrying requests or logging errors for further investigation.
Verifying Product Creation or Update in Pipedrive
To verify that your product was created or updated successfully, log in to your Pipedrive sandbox account and navigate to the Products section. You should see the newly created or updated product listed there.
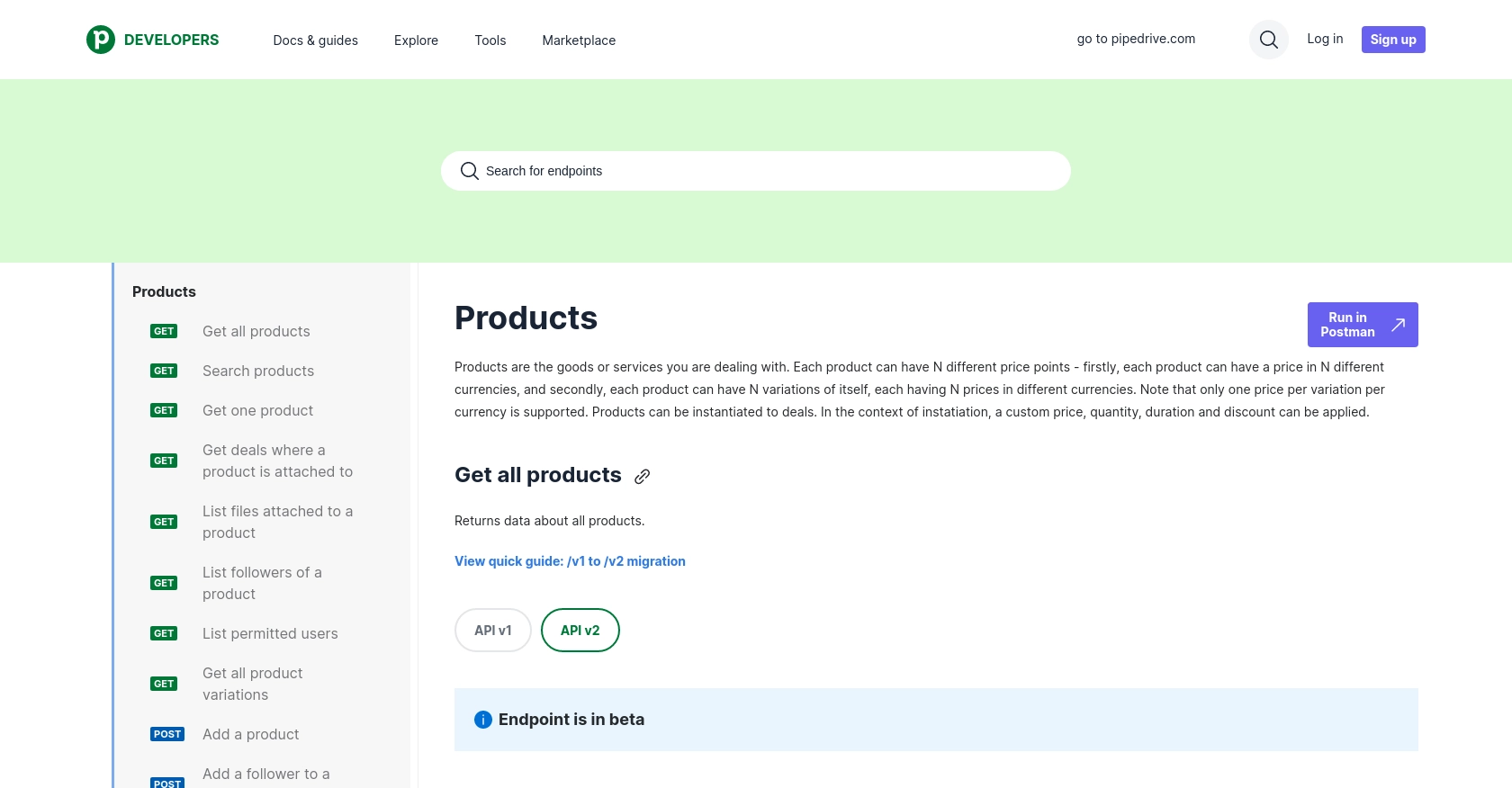
Best Practices for Pipedrive API Integration in PHP
When integrating with the Pipedrive API using PHP, it's essential to follow best practices to ensure a smooth and efficient process. Here are some recommendations to enhance your integration:
- Securely Store Credentials: Always store your client ID, client secret, and access tokens securely. Consider using environment variables or a secure vault to protect sensitive information.
- Handle Rate Limiting: Pipedrive's API has rate limits, such as 80 requests per 2 seconds for the Essential plan using OAuth. Implement logic to handle HTTP 429 errors by retrying requests after a delay. For more details, refer to the Pipedrive rate limiting documentation.
- Data Transformation and Standardization: Ensure that data sent to and received from Pipedrive is transformed and standardized according to your application's needs. This includes handling custom fields and data types.
- Error Handling: Implement robust error handling to manage different HTTP status codes. Log errors for debugging and provide user-friendly messages where applicable.
Streamlining Your Integration Process with Endgrate
Building and maintaining integrations can be time-consuming and complex. Endgrate offers a solution by providing a unified API endpoint that connects to multiple platforms, including Pipedrive. With Endgrate, you can:
- Save Time and Resources: Focus on your core product development while Endgrate handles the integration complexities.
- Build Once, Use Everywhere: Create a single integration for multiple platforms, reducing redundancy and effort.
- Enhance Customer Experience: Provide your users with a seamless and intuitive integration experience.
Explore how Endgrate can simplify your integration needs by visiting Endgrate.
Conclusion on Pipedrive API Integration with PHP
Integrating with the Pipedrive API using PHP allows developers to automate product management tasks, ensuring sales teams have up-to-date information. By following the steps outlined in this article, you can efficiently create or update products in Pipedrive, enhancing your application's capabilities.
Remember to adhere to best practices for security, error handling, and rate limiting to maintain a robust integration. Consider leveraging Endgrate for a streamlined integration process, allowing you to focus on delivering value to your users.
Read More
- https://endgrate.com/provider/pipedrive
- https://pipedrive.readme.io/docs/getting-started
- https://pipedrive.readme.io/docs/developer-sandbox-account
- https://pipedrive.readme.io/docs/marketplace-creating-a-proper-app
- https://pipedrive.readme.io/docs/core-api-concepts-rate-limiting
- https://pipedrive.readme.io/docs/core-api-concepts-pagination
- https://pipedrive.readme.io/docs/core-api-concepts-http-status-codes
- https://pipedrive.readme.io/docs/core-api-concepts-custom-fields
- https://developers.pipedrive.com/docs/api/v1/Products
- https://developers.pipedrive.com/docs/api/v1/ProductFields
Ready to get started?