How to Get Tax Rates with the Sage Accounting API in PHP
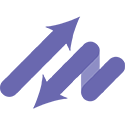
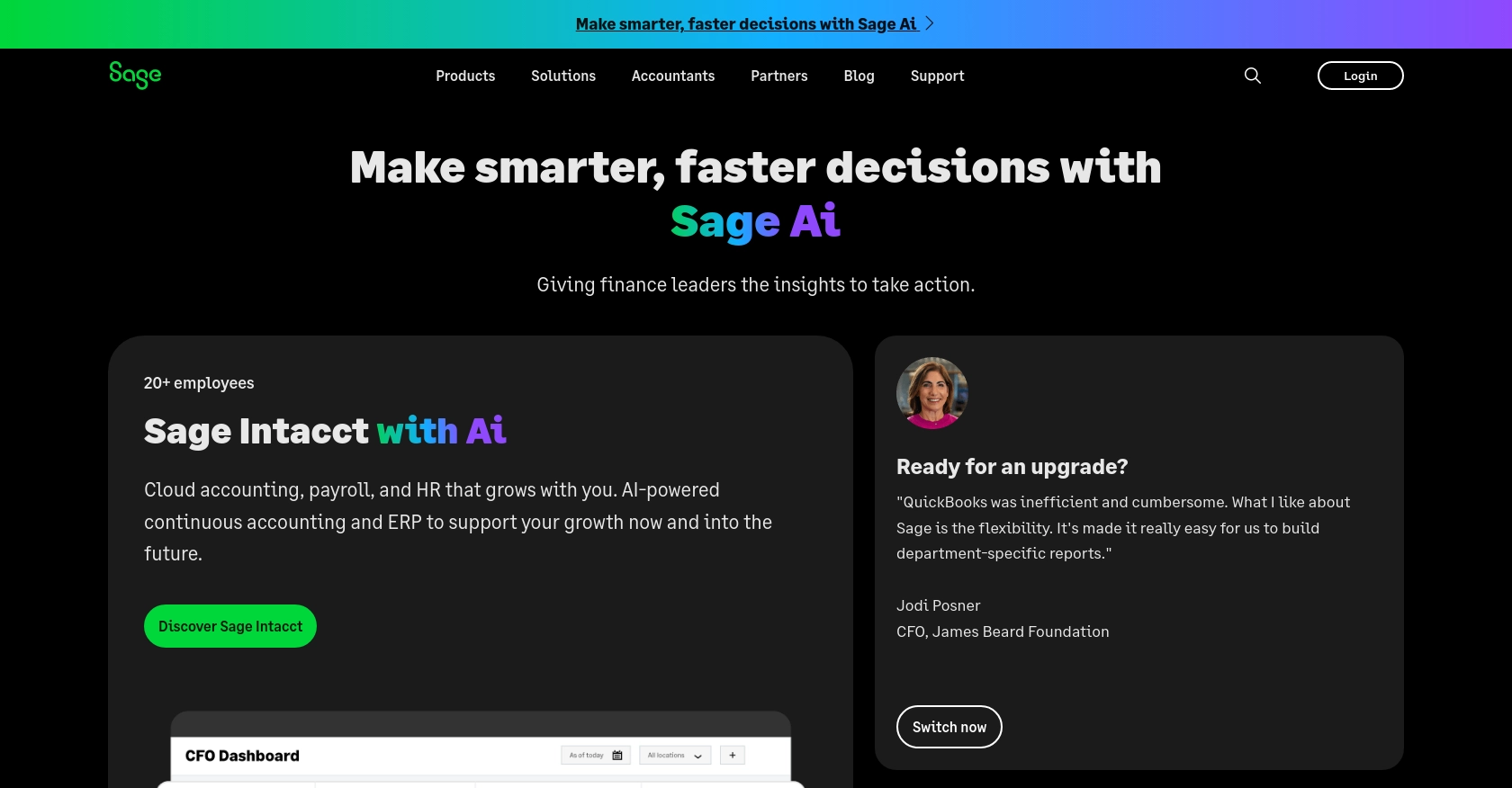
Introduction to Sage Accounting API
Sage Accounting is a robust cloud-based accounting solution designed to simplify financial management for small to medium-sized businesses. It offers a comprehensive suite of tools for managing invoices, expenses, and taxes, making it a popular choice for businesses looking to streamline their financial operations.
Integrating with the Sage Accounting API allows developers to automate and enhance accounting processes. For example, accessing tax rates through the API can help businesses ensure compliance with local tax regulations by automatically applying the correct rates to transactions. This integration can be particularly beneficial for developers looking to build applications that require accurate tax calculations and reporting.
Setting Up a Sage Accounting Test/Sandbox Account for API Integration
Before you can start integrating with the Sage Accounting API, you'll need to set up a test or sandbox account. This allows you to safely experiment with the API without affecting live data. Follow these steps to create your sandbox environment and obtain the necessary credentials for OAuth authentication.
Create a Sage Developer Account
To begin, you must have a Sage Developer account. This account will enable you to register and manage your applications, as well as obtain your client credentials.
- Visit the Sage Developer Portal and sign up using your GitHub account or an email address.
- Follow the instructions to complete your registration.
Register a Trial Business Account
Next, you'll need to set up a trial business account for development purposes. Sage offers trial accounts for various regions and subscription tiers.
- Navigate to the trial business setup page.
- Select your region and desired subscription tier, then sign up for a trial account.
- Ensure that your trial account is active before proceeding.
Create a Sage Accounting App for OAuth Authentication
With your developer and trial accounts ready, you can now create an app to obtain the OAuth credentials needed for API access.
- Log in to your Sage Developer account.
- Go to the Create an App section.
- Click on "Create App" and fill in the required details, such as the app name and callback URL.
- Save your app to generate a Client ID and Client Secret.
Upgrade to a Developer Account
To gain extended access for testing, upgrade your trial account to a developer account.
- Submit a request through the Upgrade Your Account page.
- Provide your name, email, app name, Client ID, and region.
- Wait for confirmation, which typically takes 3-5 working days.
Once you have completed these steps, you will have a fully functional sandbox environment to test your Sage Accounting API integrations. You can now proceed to make API calls using the credentials obtained from your app setup.
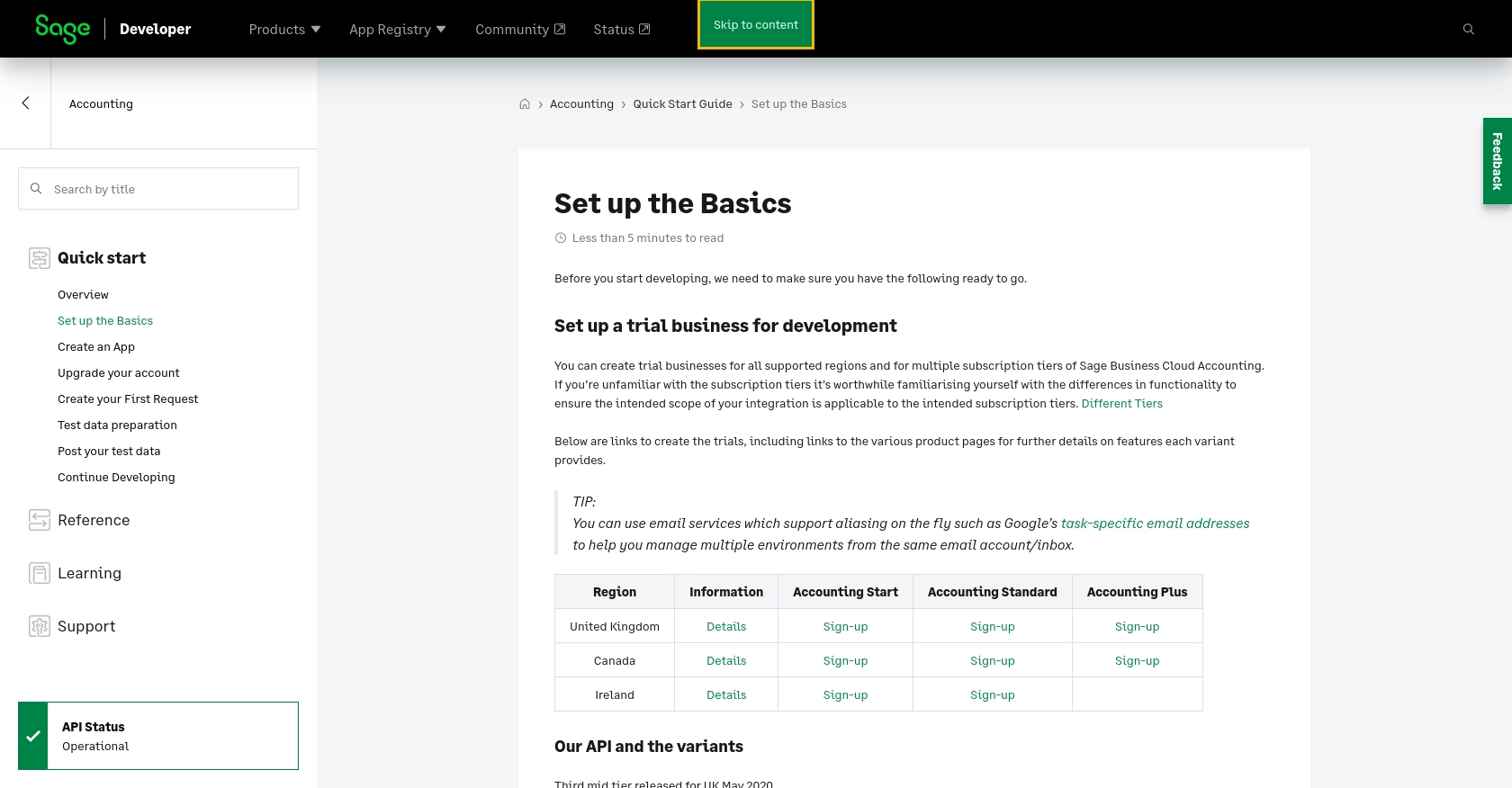
sbb-itb-96038d7
Making API Calls to Retrieve Tax Rates with Sage Accounting API in PHP
To interact with the Sage Accounting API and retrieve tax rates, you'll need to use PHP to make HTTP requests. This section will guide you through the necessary steps, including setting up your PHP environment, writing the code to make the API call, and handling the response.
Setting Up Your PHP Environment
Ensure you have the following prerequisites installed on your machine:
- PHP 7.4 or higher
- Composer, the PHP package manager
You'll also need to install the guzzlehttp/guzzle
library, which simplifies making HTTP requests in PHP. Run the following command in your terminal:
composer require guzzlehttp/guzzle
Writing PHP Code to Retrieve Tax Rates from Sage Accounting API
Create a new PHP file named get_tax_rates.php
and add the following code:
<?php
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$accessToken = 'Your_Access_Token'; // Replace with your actual access token
try {
$response = $client->request('GET', 'https://api.accounting.sage.com/v3.1/tax_rates', [
'headers' => [
'Authorization' => 'Bearer ' . $accessToken,
'Accept' => 'application/json',
],
]);
$data = json_decode($response->getBody(), true);
foreach ($data as $taxRate) {
echo 'Tax Rate: ' . $taxRate['name'] . ' - ' . $taxRate['percentage'] . '%' . PHP_EOL;
}
} catch (Exception $e) {
echo 'Error: ' . $e->getMessage();
}
Replace Your_Access_Token
with the OAuth access token you obtained during the app setup process.
Running the PHP Script and Verifying the Output
Execute the script from your terminal using the following command:
php get_tax_rates.php
If successful, you should see a list of tax rates displayed in your terminal. Verify the data by cross-referencing it with the tax rates available in your Sage Accounting sandbox account.
Handling Errors and Understanding API Response Codes
When making API calls, it's crucial to handle potential errors gracefully. The Sage Accounting API may return various HTTP status codes indicating the result of your request:
- 200 OK: The request was successful, and the response contains the requested data.
- 401 Unauthorized: The access token is missing or invalid. Ensure your token is correct and not expired.
- 403 Forbidden: The authenticated user does not have permission to access the resource.
- 404 Not Found: The requested resource could not be found.
- 500 Internal Server Error: An error occurred on the server. Try the request again later.
For more detailed information on error codes, refer to the Sage Accounting API documentation.
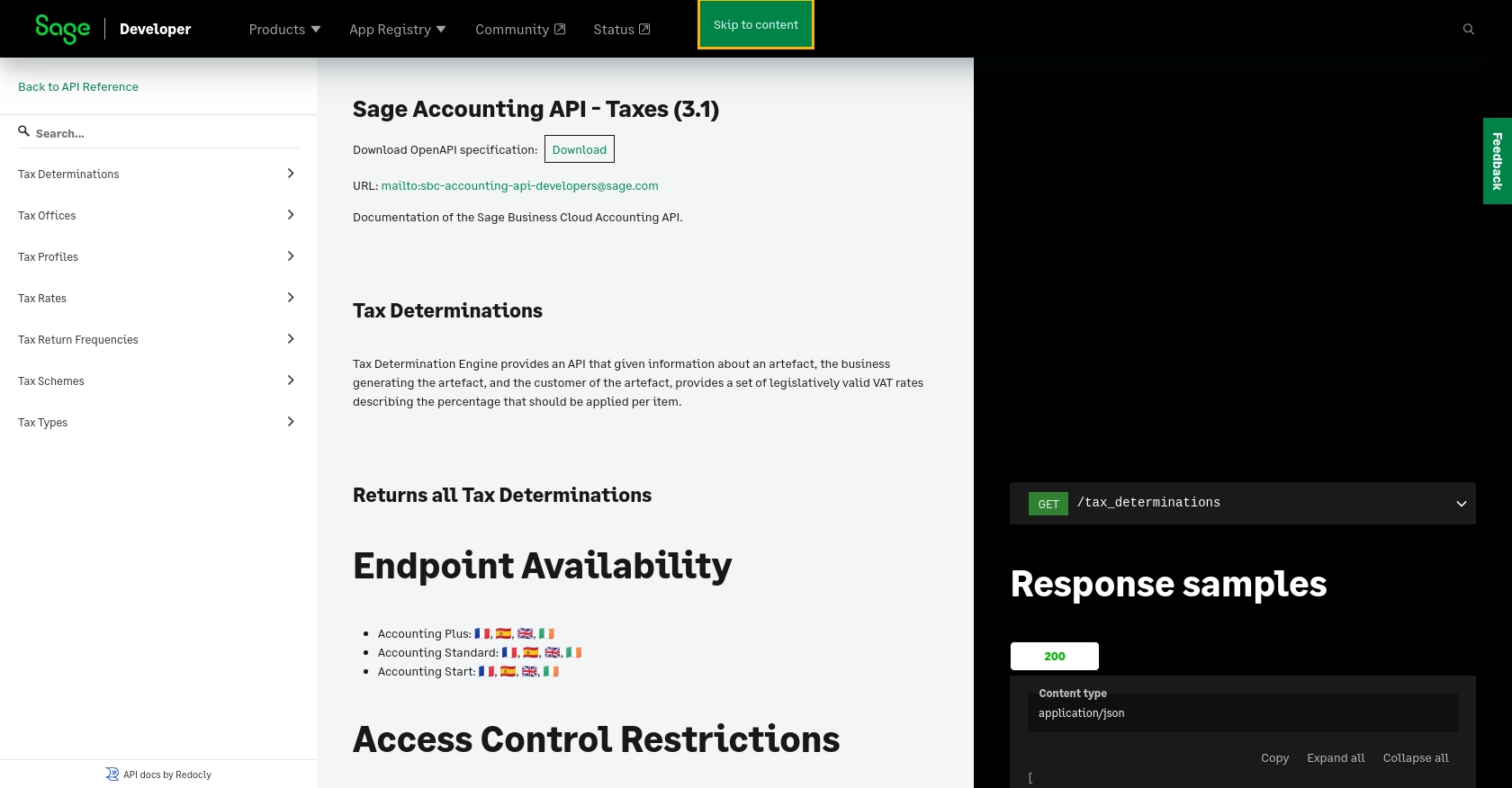
Conclusion and Best Practices for Using Sage Accounting API in PHP
Integrating with the Sage Accounting API to retrieve tax rates can significantly enhance your application's ability to manage financial data accurately. By automating tax calculations and ensuring compliance with local regulations, you can streamline your accounting processes and reduce manual errors.
Best Practices for Secure and Efficient API Integration
- Securely Store Credentials: Always store your OAuth credentials securely. Consider using environment variables or a secure vault to manage sensitive information like client IDs and secrets.
- Handle Rate Limiting: Be aware of any rate limits imposed by the Sage Accounting API. Implement retry logic and exponential backoff strategies to handle rate limit responses gracefully.
- Standardize Data Fields: Ensure that the data retrieved from the API is standardized and transformed as needed to fit your application's requirements.
- Monitor API Changes: Regularly check the Sage Accounting API documentation for updates or changes that might affect your integration.
Streamlining Integration with Endgrate
For developers looking to simplify their integration processes, consider using Endgrate. With Endgrate, you can save time and resources by outsourcing integrations, allowing you to focus on your core product. Build once for each use case and leverage an intuitive integration experience for your customers.
Visit Endgrate to learn more about how you can efficiently manage multiple integrations with a single API endpoint.
Read More
Ready to get started?