How to Create or Update Users with the Iterable API in Javascript
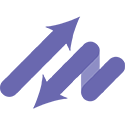
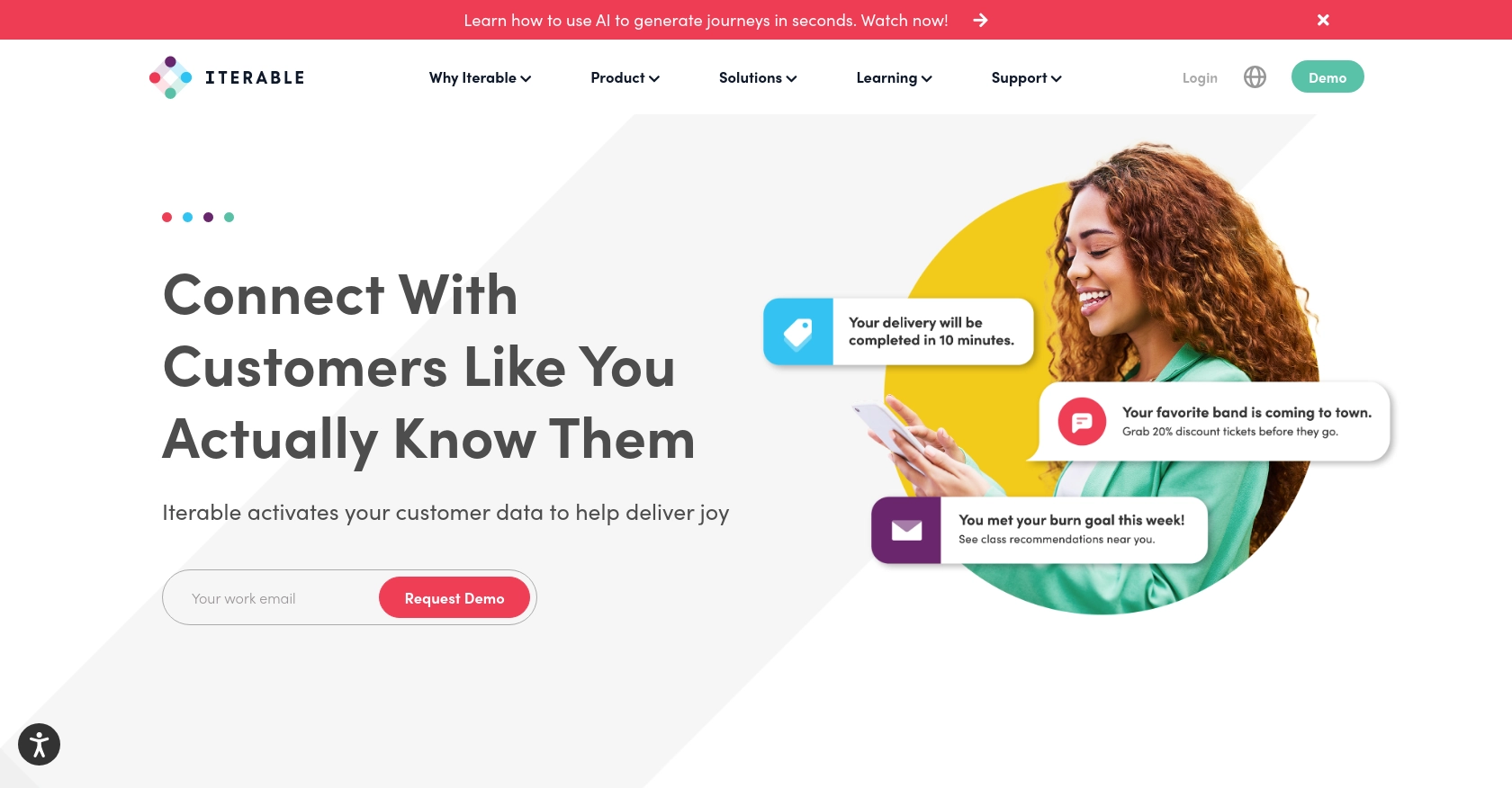
Introduction to Iterable API for User Management
Iterable is a powerful marketing automation platform that enables businesses to create, execute, and optimize cross-channel campaigns. It offers a robust set of tools for managing customer engagement through email, SMS, push notifications, and more.
For developers, integrating with Iterable's API can significantly enhance user management capabilities. By leveraging the API, you can automate the creation and updating of user profiles, ensuring that your marketing efforts are always targeted and relevant. For example, you might use the Iterable API to update user preferences based on their interactions with your app, allowing for more personalized communication.
Setting Up Your Iterable Test/Sandbox Account for API Integration
Before you can start creating or updating users with the Iterable API in JavaScript, you'll need to set up a test or sandbox account. This allows you to safely experiment with API calls without affecting live data.
Creating an Iterable Account
If you don't already have an Iterable account, you can sign up for a free trial on the Iterable website. This will give you access to the platform's features and allow you to test API interactions.
Generating an API Key for Authentication
Iterable uses API key-based authentication to secure API requests. Follow these steps to generate your API key:
- Log in to your Iterable account.
- Navigate to the Integrations section in the sidebar.
- Select API Keys from the dropdown menu.
- Click on Create New API Key.
- Provide a name for your API key and select the appropriate permissions for your use case.
- Click Create to generate the key.
- Copy the API key and store it securely, as you'll need it for authenticating your API requests.
For more details on API key management, refer to the Iterable API Keys documentation.
Configuring Your Development Environment
To interact with the Iterable API using JavaScript, ensure you have the following prerequisites:
- Node.js installed on your machine.
- A code editor, such as Visual Studio Code.
- Access to a terminal or command line interface.
With your Iterable account and API key ready, you're all set to start integrating with the Iterable API in JavaScript.
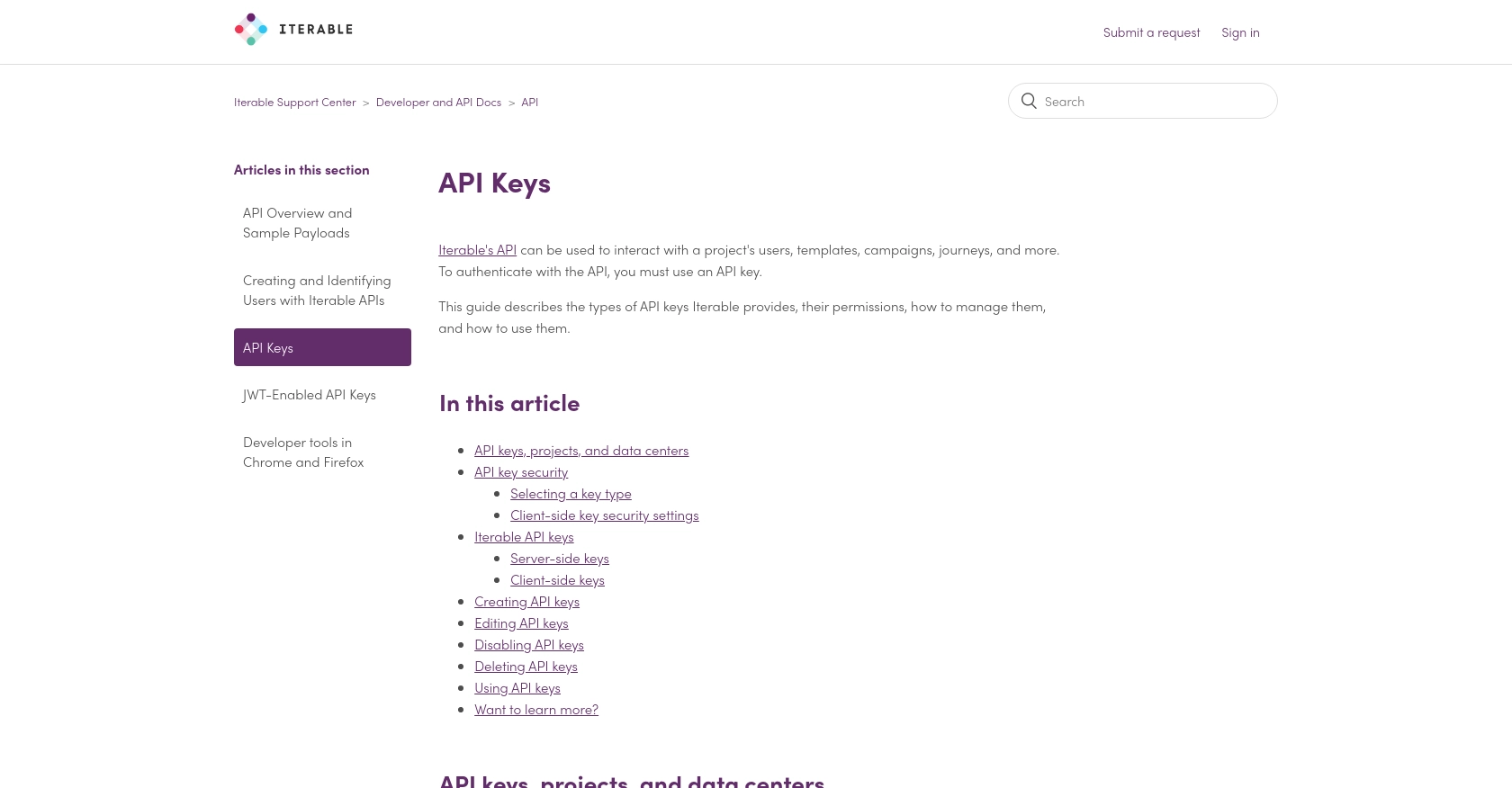
sbb-itb-96038d7
Making API Calls to Create or Update Users with Iterable in JavaScript
To effectively interact with the Iterable API for creating or updating users, you'll need to set up your JavaScript environment and understand the necessary API endpoints and request structures. This section will guide you through the process of making API calls using JavaScript.
Setting Up Your JavaScript Environment for Iterable API Integration
Before making API calls, ensure your development environment is ready:
- Ensure Node.js is installed on your machine.
- Use a code editor like Visual Studio Code for writing your scripts.
- Open a terminal or command line interface to run your JavaScript code.
Installing Required Dependencies for API Requests
To make HTTP requests in JavaScript, you can use the popular axios
library. Install it using npm:
npm install axios
Example Code for Creating or Updating Users with Iterable API
Below is an example of how to create or update a user using the Iterable API in JavaScript:
const axios = require('axios');
// Define the API endpoint and headers
const endpoint = 'https://api.iterable.com/api/users/update';
const apiKey = 'Your_API_Key';
const headers = {
'Content-Type': 'application/json',
'Api-Key': apiKey
};
// Define the user data to create or update
const userData = {
email: 'example@domain.com',
dataFields: {
firstName: 'John',
lastName: 'Doe'
}
};
// Make a POST request to the Iterable API
axios.post(endpoint, userData, { headers })
.then(response => {
console.log('User updated successfully:', response.data);
})
.catch(error => {
console.error('Error updating user:', error.response.data);
});
Replace Your_API_Key
with the API key you generated earlier. The userData
object contains the user information you want to create or update.
Handling API Responses and Errors
After making an API call, it's crucial to handle the response correctly. If the request is successful, you'll receive a confirmation message. If there's an error, the API will return an error code and message. Common error codes include:
- 400: Invalid parameters
- 401: Invalid API key
- 409: Email/userId conflict
Refer to the Iterable API Documentation for more details on error codes and handling.
Verifying API Call Success in Iterable
To verify that your API call was successful, check the user data in your Iterable account. Navigate to the user management section and confirm that the user information has been updated as expected.
Conclusion and Best Practices for Using Iterable API in JavaScript
Integrating with the Iterable API using JavaScript offers a powerful way to manage user data and enhance your marketing automation efforts. By following the steps outlined in this guide, you can efficiently create or update user profiles, ensuring your campaigns are always relevant and personalized.
Best Practices for Managing User Data with Iterable API
- Secure API Keys: Always store your API keys securely and avoid hardcoding them in your scripts. Consider using environment variables for added security.
- Handle Rate Limits: Be mindful of Iterable's rate limits to avoid throttling. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Data Validation: Validate user data before making API calls to prevent errors and ensure data integrity.
- Error Handling: Implement robust error handling to manage API response errors effectively. Log errors for troubleshooting and monitoring purposes.
Transforming and Standardizing Data Fields
When working with user data, ensure that data fields are standardized and transformed as needed. This helps maintain consistency across your marketing campaigns and improves data quality.
Leverage Endgrate for Streamlined Integrations
While integrating with the Iterable API can be straightforward, managing multiple integrations can become complex. Consider using Endgrate to simplify your integration processes. With Endgrate, you can:
- Save time and resources by outsourcing integrations and focusing on your core product.
- Build once for each use case instead of multiple times for different integrations.
- Provide an easy, intuitive integration experience for your customers.
Explore more about how Endgrate can enhance your integration strategy by visiting Endgrate.
Read More
Ready to get started?