How to Create or Update Vendors with the Sage 100 API in Python
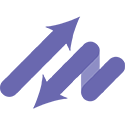
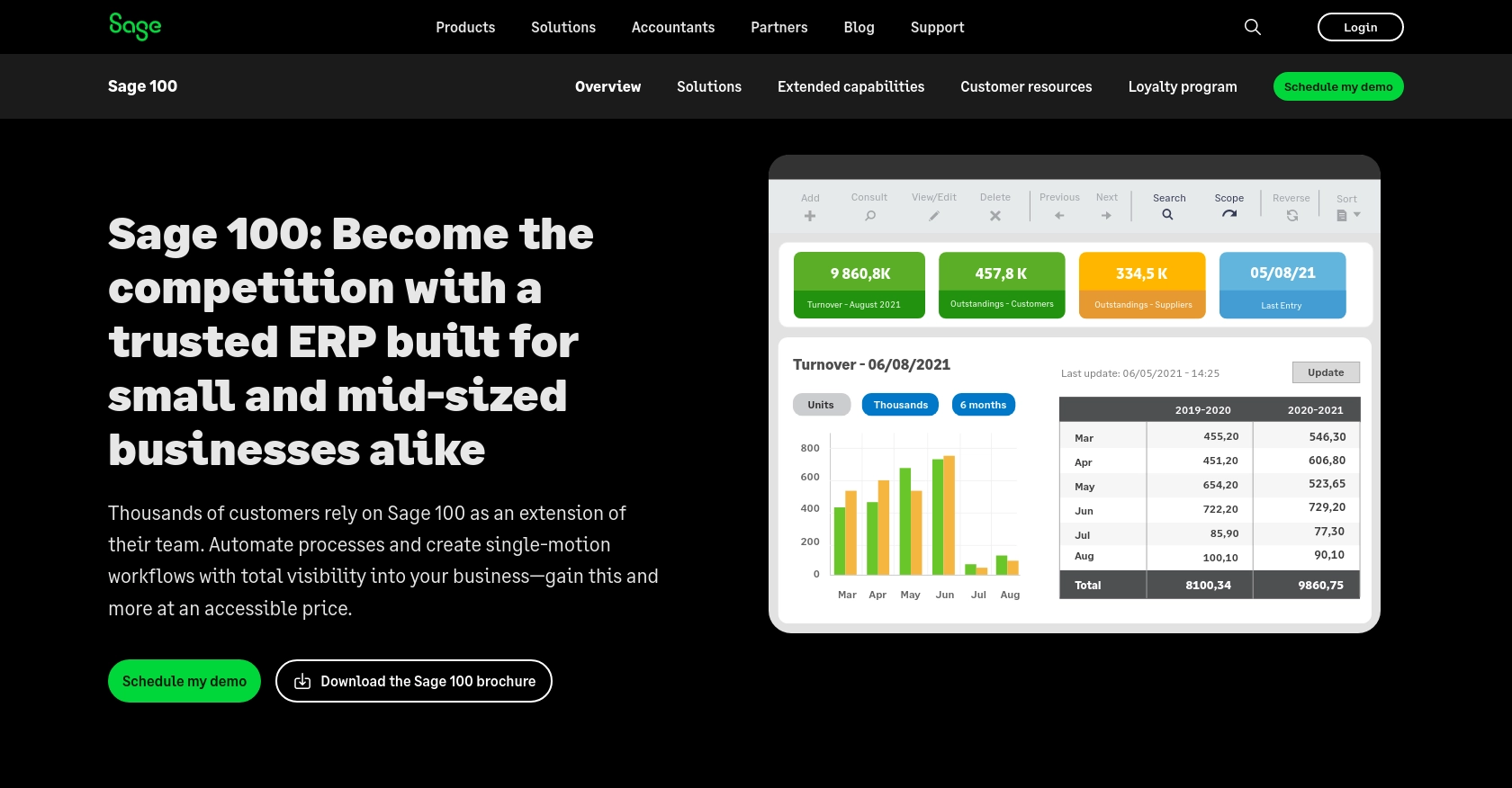
Introduction to Sage 100 API Integration
Sage 100 is a comprehensive ERP solution tailored for small to medium-sized businesses, offering robust features for accounting, manufacturing, distribution, and more. Its flexibility and scalability make it a preferred choice for businesses looking to streamline their operations.
Integrating with the Sage 100 API allows developers to automate and enhance various business processes. For example, you can create or update vendor information directly from your application, ensuring that your vendor data is always up-to-date and consistent across systems.
This article will guide you through the process of using Python to interact with the Sage 100 API, specifically focusing on creating or updating vendors. By following these steps, you can efficiently manage vendor data and improve your business workflows.
Setting Up Your Sage 100 Test Environment
Before you can start integrating with the Sage 100 API, you need to set up a test environment. This involves configuring the Sage 100 ODBC driver, which is essential for establishing a connection to the Sage 100 database. Follow these steps to ensure your environment is ready for development.
Installing and Configuring the Sage 100 ODBC Driver
To interact with the Sage 100 API, you must first install and configure the Sage 100 ODBC driver. This driver allows your application to communicate with the Sage 100 database. Here's how to set it up:
- Access the ODBC Data Source Administrator on your system.
- Create a Data Source Name (DSN) that connects to the Sage 100 ERP system. Ensure you use the correct server, database, and authentication settings.
- Configure the DSN to use the Sage 100 ODBC driver. This involves specifying the server name, database, and any necessary credentials.
For detailed instructions, refer to the Sage 100 ODBC Driver Configuration Guide.
Creating a Sage 100 Test Account
To test your integration, you'll need a Sage 100 test account. If you don't already have one, you can set it up by following these steps:
- Visit the Sage 100 website and sign up for a trial or demo account.
- Follow the on-screen instructions to complete the registration process.
- Once registered, log in to your Sage 100 account to access the test environment.
Configuring Authentication for Sage 100 API
Sage 100 uses a custom authentication method. Ensure you have the necessary credentials to authenticate your API requests:
- In your Sage 100 account, navigate to the API settings section.
- Generate the required API credentials, such as client ID and client secret, if applicable.
- Store these credentials securely, as you'll need them to authenticate your API calls.
With your test environment set up and authentication configured, you're ready to start integrating with the Sage 100 API using Python. In the next section, we'll explore how to make API calls to create or update vendor information.
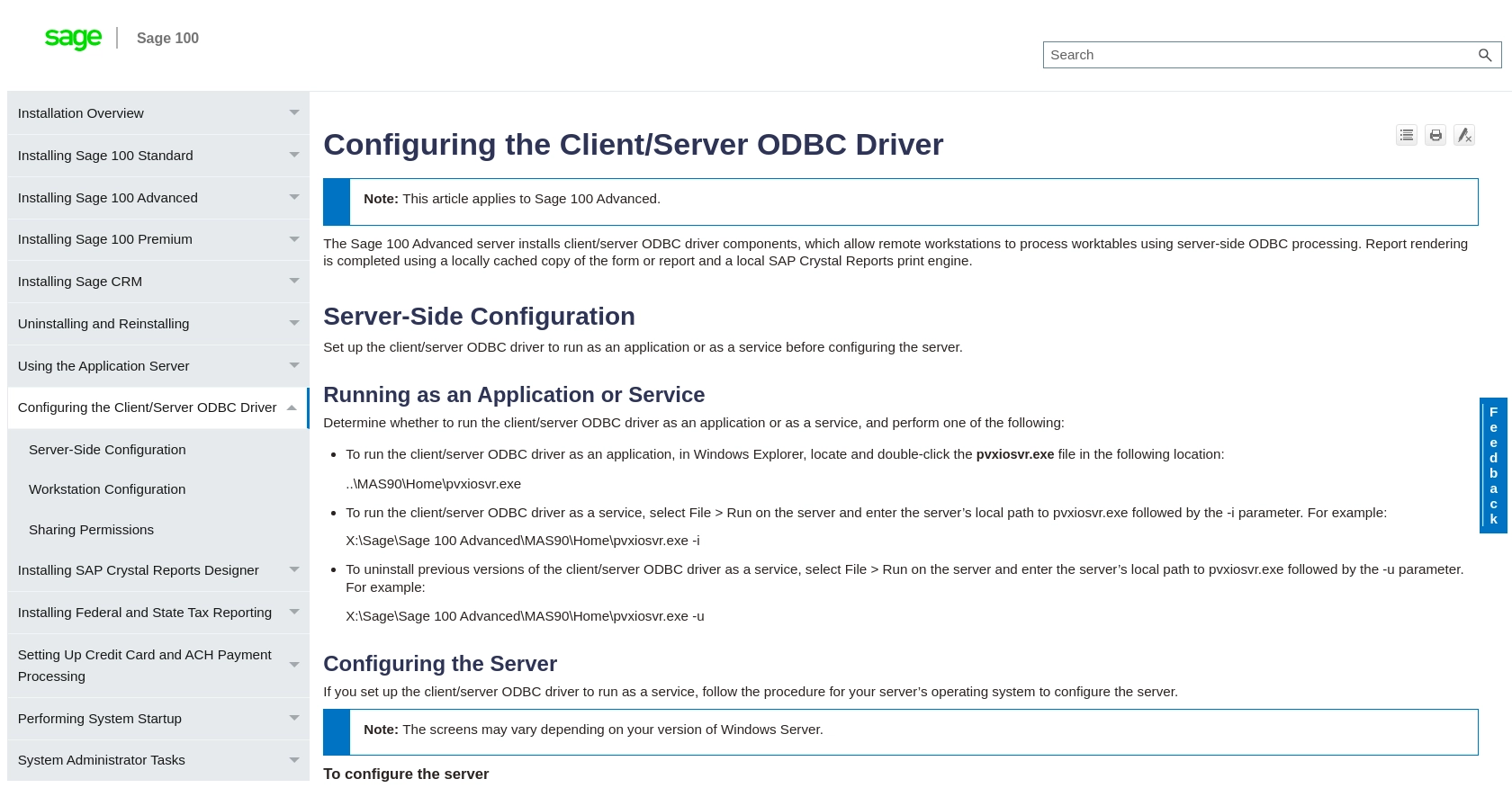
sbb-itb-96038d7
Making API Calls to Create or Update Vendors in Sage 100 Using Python
With your Sage 100 test environment set up, you can now proceed to make API calls to create or update vendor information. This section will guide you through the process of using Python to interact with the Sage 100 API, ensuring your vendor data is efficiently managed.
Setting Up Your Python Environment for Sage 100 API Integration
Before making API calls, ensure your Python environment is properly configured. You'll need Python 3.x installed on your machine, along with the necessary libraries to handle HTTP requests and ODBC connections.
- Ensure Python 3.x is installed. You can download it from the official Python website.
- Install the required libraries using pip:
pip install pyodbc requests
Creating or Updating Vendors with Sage 100 API
Now, let's dive into the code to create or update vendors using the Sage 100 API. The following Python script demonstrates how to establish a connection and perform the necessary operations.
import pyodbc
import requests
# Define the DSN and credentials
dsn = 'Your_DSN_Name'
user = 'Your_Username'
password = 'Your_Password'
# Establish a connection to the Sage 100 database
connection = pyodbc.connect(f'DSN={dsn};UID={user};PWD={password}')
# Define the API endpoint for creating or updating vendors
api_endpoint = "https://api.sage100.com/vendors"
# Define the vendor data
vendor_data = {
"VendorNo": "V001",
"VendorName": "New Vendor",
"Address": "123 Main St",
"City": "Anytown",
"State": "CA",
"ZipCode": "12345"
}
# Make a POST request to create or update the vendor
response = requests.post(api_endpoint, json=vendor_data, auth=(user, password))
# Check if the request was successful
if response.status_code == 200:
print("Vendor created or updated successfully.")
else:
print(f"Failed to create or update vendor. Status code: {response.status_code}")
Replace Your_DSN_Name
, Your_Username
, and Your_Password
with your actual DSN, username, and password. The vendor_data
dictionary contains the vendor details you wish to create or update.
Verifying API Call Success in Sage 100
After executing the script, verify the success of your API call by checking the Sage 100 test environment. Ensure the vendor information is correctly reflected in the system. If the operation was successful, the vendor should appear with the updated details.
Handling Errors and Troubleshooting Sage 100 API Calls
It's crucial to handle potential errors when making API calls. The script checks the response status code to determine success. If the status code is not 200, an error message is printed. Consider implementing additional error handling to manage specific error codes and exceptions.
For more detailed error information, refer to the Sage 100 API documentation.
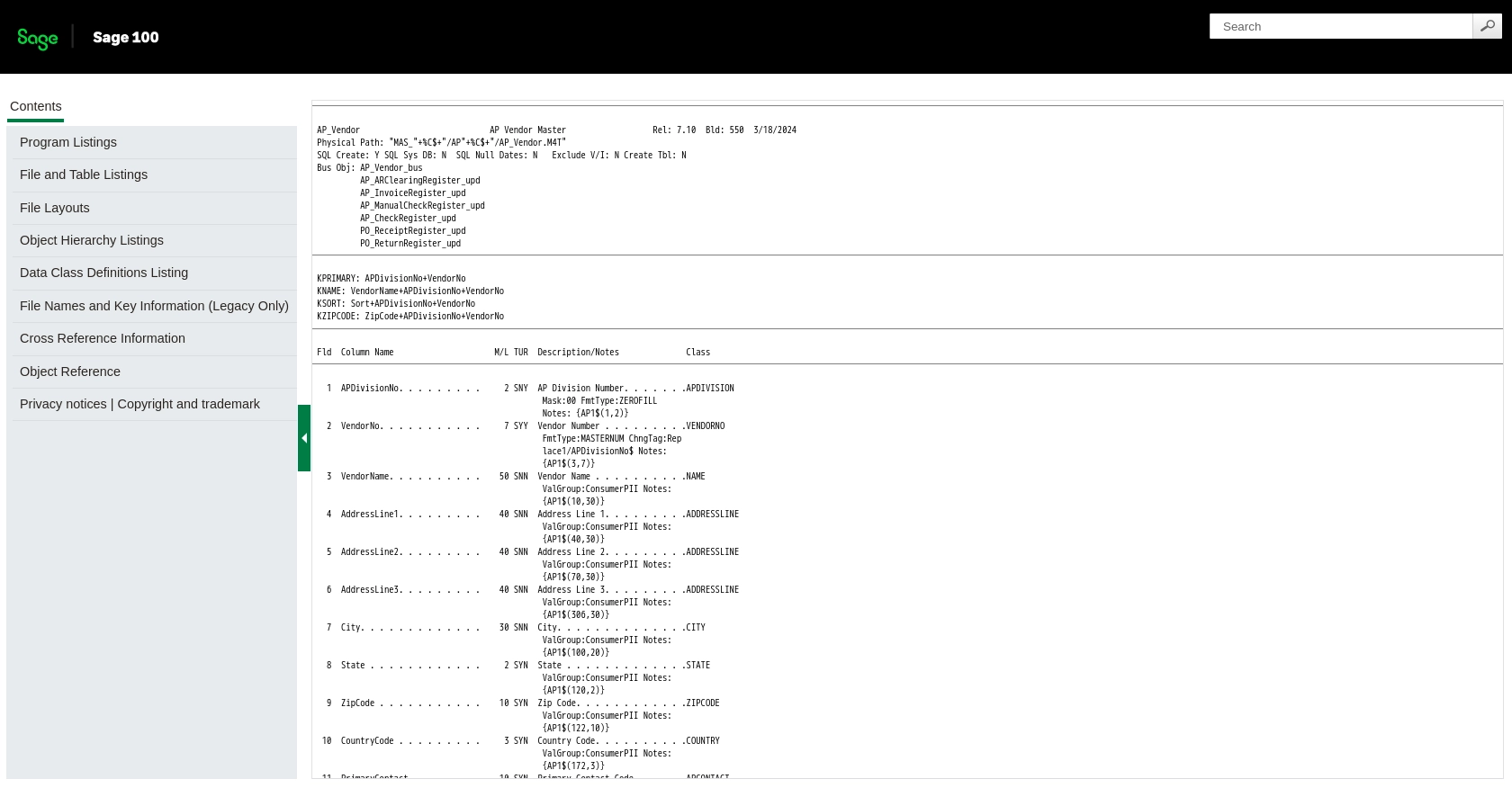
Best Practices for Sage 100 API Integration
Successfully integrating with the Sage 100 API requires adherence to best practices to ensure security, efficiency, and maintainability. Here are some key recommendations:
- Secure Credential Management: Store your API credentials securely, using environment variables or a secure vault, to prevent unauthorized access.
- Handle Rate Limiting: Be aware of any rate limits imposed by the Sage 100 API. Implement retry logic and exponential backoff to handle rate limit errors gracefully.
- Data Standardization: Ensure that data fields are standardized and consistent across systems to avoid discrepancies and data integrity issues.
- Error Handling: Implement robust error handling to manage exceptions and specific error codes, ensuring that your application can recover gracefully from failures.
Enhance Your Integration Strategy with Endgrate
Integrating with multiple platforms can be complex and time-consuming. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Sage 100. By leveraging Endgrate, you can:
- Save time and resources by outsourcing integrations and focusing on your core product development.
- Build once for each use case instead of multiple times for different integrations, streamlining your development process.
- Offer an intuitive integration experience for your customers, enhancing user satisfaction and engagement.
Explore how Endgrate can transform your integration strategy by visiting Endgrate's website today.
Read More
Ready to get started?