How to Get Accounts with the Zoho CRM API in Javascript
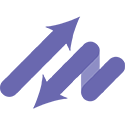
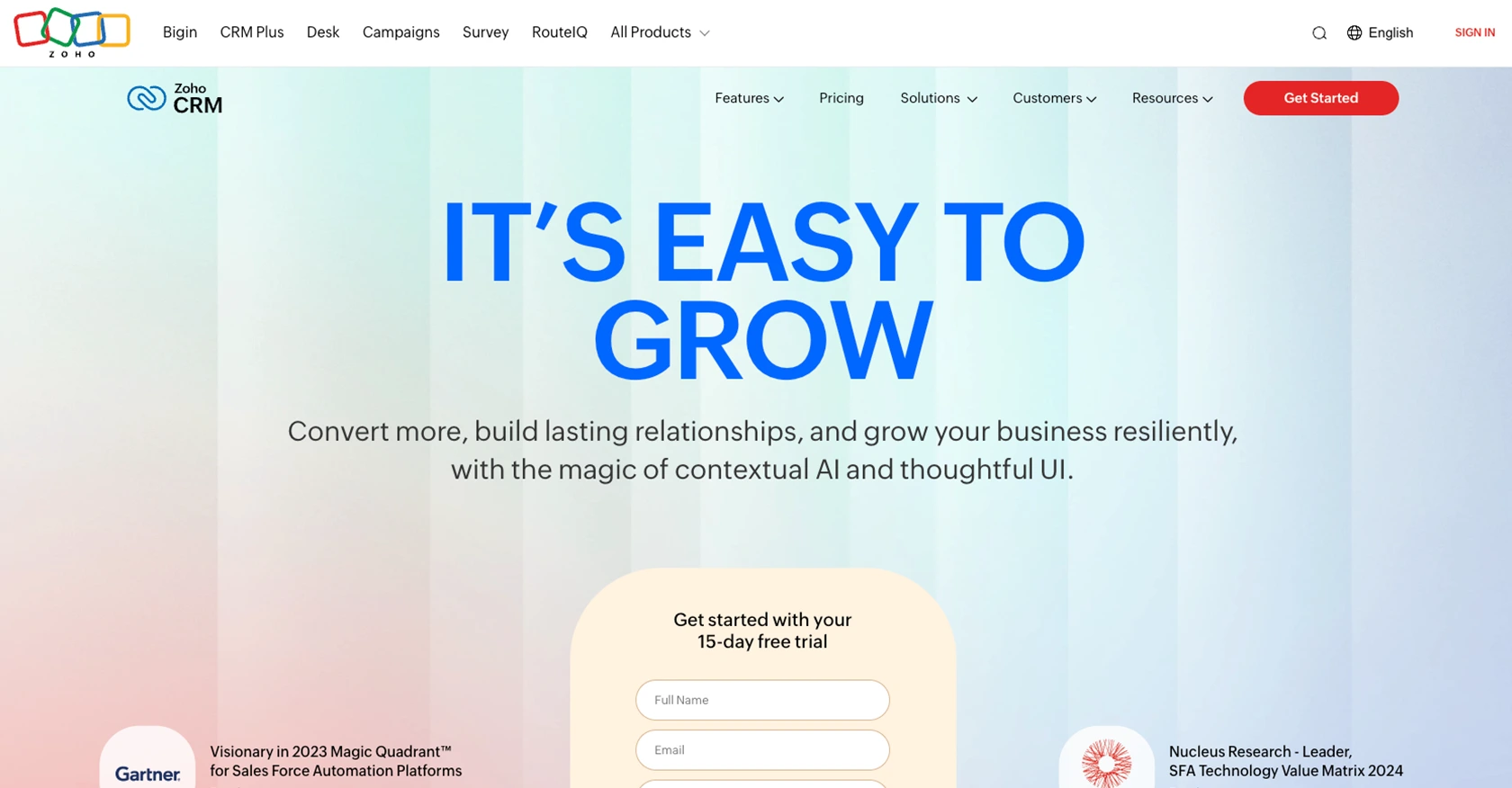
Introduction to Zoho CRM
Zoho CRM is a powerful customer relationship management platform that helps businesses manage their sales, marketing, and support in a single system. Known for its flexibility and comprehensive features, Zoho CRM is a popular choice for organizations looking to enhance their customer interactions and streamline their business processes.
Developers often integrate with Zoho CRM to access and manage customer data, such as accounts, to automate and personalize customer engagement. For example, using the Zoho CRM API, a developer can retrieve account information to generate detailed reports or synchronize data with other business applications.
This article will guide you through using JavaScript to interact with the Zoho CRM API, specifically focusing on retrieving account data. By following this tutorial, you will learn how to efficiently access and manage accounts within the Zoho CRM platform using JavaScript.
Setting Up Your Zoho CRM Test/Sandbox Account
Before you can start interacting with the Zoho CRM API using JavaScript, you need to set up a test or sandbox account. This will allow you to safely experiment with API calls without affecting live data. Zoho CRM offers a developer sandbox environment that mimics the production environment, providing a risk-free space for testing.
Creating a Zoho CRM Developer Account
If you don't have a Zoho CRM account, follow these steps to create one:
- Visit the Zoho CRM sign-up page.
- Fill out the registration form with your details and click Sign Up for Free.
- Once registered, you will receive a confirmation email. Click the link in the email to verify your account.
Accessing the Zoho CRM Sandbox
To access the sandbox environment, follow these steps:
- Log in to your Zoho CRM account.
- Navigate to Setup in the top-right corner.
- Under Developer Space, select Sandbox.
- Click Create Sandbox and follow the prompts to set up your sandbox environment.
Registering Your Application for OAuth Authentication
Zoho CRM uses OAuth 2.0 for authentication, which requires you to register your application to obtain the necessary credentials:
- Go to the Zoho API Console.
- Click Add Client and choose Server-based Applications.
- Fill in the required details, including Client Name, Homepage URL, and Authorized Redirect URIs.
- Click Create to generate your Client ID and Client Secret.
Generating Access and Refresh Tokens
With your application registered, you can now generate access and refresh tokens:
- Direct users to the authorization URL to obtain an authorization code:
- Exchange the authorization code for access and refresh tokens by making a POST request:
https://accounts.zoho.com/oauth/v2/auth?scope=ZohoCRM.modules.ALL&client_id=YOUR_CLIENT_ID&response_type=code&redirect_uri=YOUR_REDIRECT_URI
fetch('https://accounts.zoho.com/oauth/v2/token', {
method: 'POST',
headers: {
'Content-Type': 'application/x-www-form-urlencoded'
},
body: new URLSearchParams({
'grant_type': 'authorization_code',
'client_id': 'YOUR_CLIENT_ID',
'client_secret': 'YOUR_CLIENT_SECRET',
'redirect_uri': 'YOUR_REDIRECT_URI',
'code': 'AUTHORIZATION_CODE'
})
})
.then(response => response.json())
.then(data => console.log(data));
Store the access and refresh tokens securely, as they will be used to authenticate API requests.
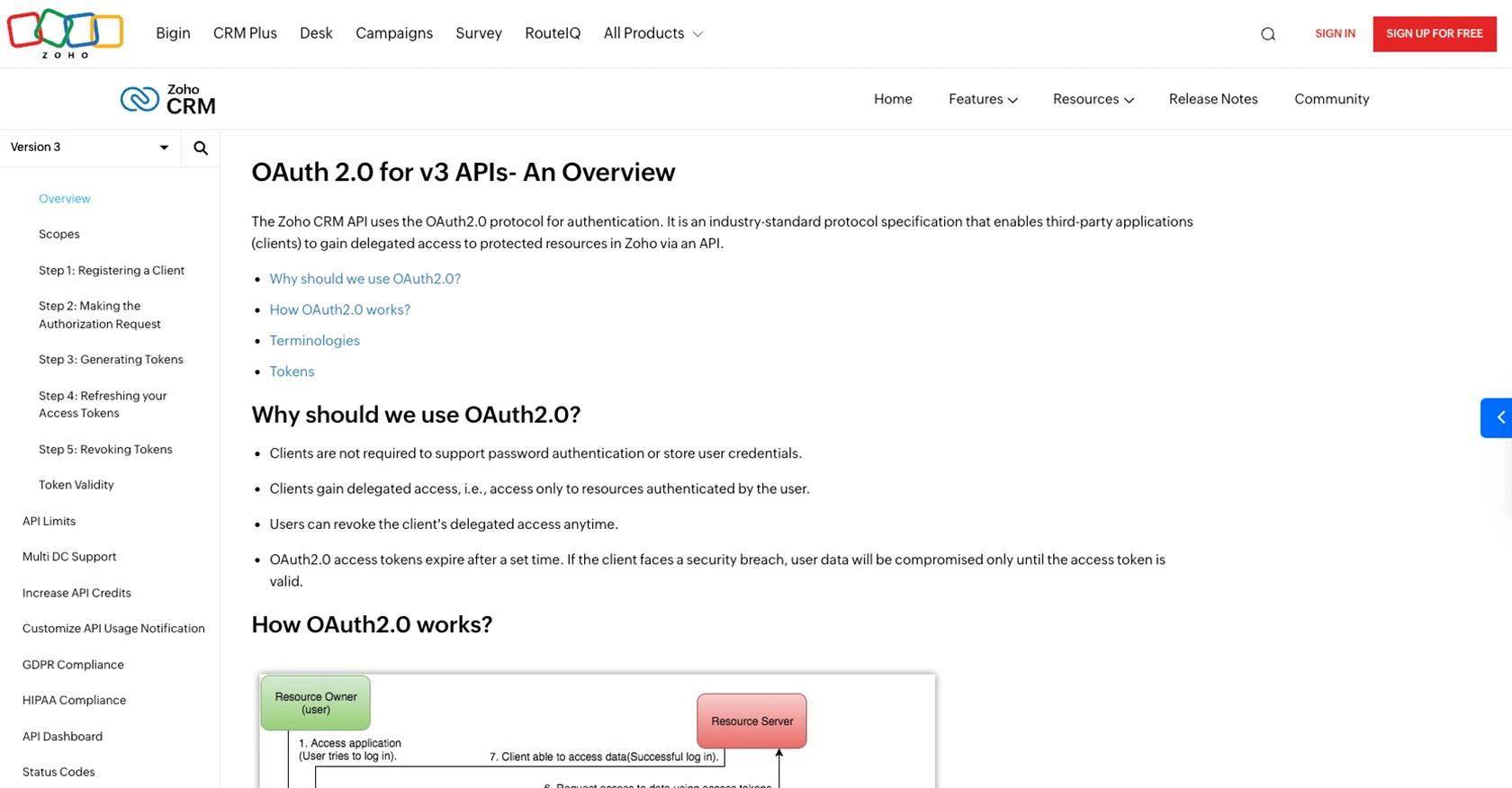
sbb-itb-96038d7
Making API Calls to Retrieve Accounts from Zoho CRM Using JavaScript
To interact with the Zoho CRM API and retrieve account data using JavaScript, you need to make HTTP requests to the appropriate endpoints. This section will guide you through the process of setting up your JavaScript environment, making the API call, and handling the response.
Setting Up Your JavaScript Environment
Before making API calls, ensure you have the following prerequisites:
- Node.js installed on your machine.
- A text editor like Visual Studio Code.
- Access to the Zoho CRM API with valid OAuth tokens.
To manage HTTP requests, you can use the node-fetch
library. Install it using the following command:
npm install node-fetch
Writing the JavaScript Code to Fetch Accounts
Create a new JavaScript file named getZohoAccounts.js
and add the following code:
const fetch = require('node-fetch');
// Define the API endpoint and headers
const endpoint = 'https://www.zohoapis.com/crm/v3/Accounts';
const headers = {
'Authorization': 'Zoho-oauthtoken YOUR_ACCESS_TOKEN',
'Content-Type': 'application/json'
};
// Function to fetch accounts from Zoho CRM
async function getAccounts() {
try {
const response = await fetch(endpoint, { headers });
if (!response.ok) {
throw new Error(`Error: ${response.status} ${response.statusText}`);
}
const data = await response.json();
console.log(data);
} catch (error) {
console.error('Failed to fetch accounts:', error);
}
}
// Call the function
getAccounts();
Replace YOUR_ACCESS_TOKEN
with the access token you obtained during the OAuth authentication process.
Running the JavaScript Code
To execute the script and retrieve account data, run the following command in your terminal:
node getZohoAccounts.js
If successful, the console will display the account data retrieved from Zoho CRM.
Handling API Response and Errors
When making API calls, it's essential to handle potential errors and verify the response. The code above checks if the response is successful and logs any errors encountered during the request. Refer to the Zoho CRM API status codes for more information on handling specific error codes.
By following these steps, you can efficiently retrieve and manage account data from Zoho CRM using JavaScript, enabling seamless integration with your business applications.
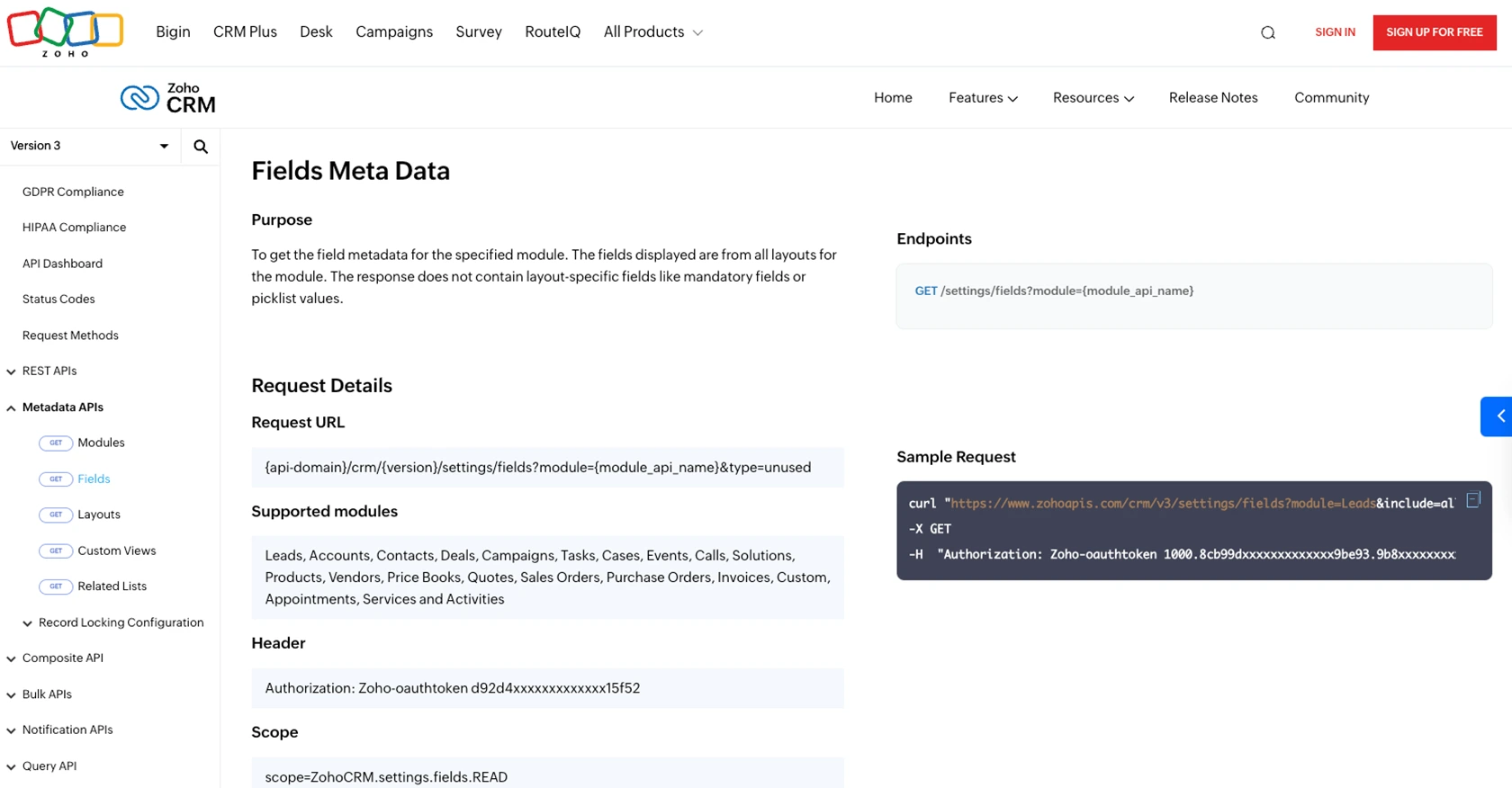
Best Practices for Zoho CRM API Integration Using JavaScript
When integrating with the Zoho CRM API using JavaScript, it's crucial to follow best practices to ensure a secure and efficient implementation. Here are some recommendations:
Securely Store OAuth Tokens
Always store your OAuth access and refresh tokens securely. Avoid hardcoding them directly into your application code. Instead, use environment variables or secure vaults to manage sensitive information.
Handle Zoho CRM API Rate Limits
Zoho CRM imposes API rate limits to ensure fair usage. Be mindful of these limits and implement logic to handle rate limiting gracefully. For example, you can implement exponential backoff strategies to retry requests when you encounter rate limit errors. For more details, refer to the Zoho CRM API limits documentation.
Transform and Standardize Data Fields
When retrieving data from Zoho CRM, ensure that you transform and standardize data fields to match your application's requirements. This can involve mapping Zoho CRM fields to your internal data structures and handling any necessary data conversions.
Monitor and Log API Interactions
Implement logging and monitoring for your API interactions to track usage patterns and diagnose issues. This will help you maintain a robust integration and quickly identify any problems that arise.
Utilize Endgrate for Simplified Integrations
Consider using Endgrate to streamline your integration process. Endgrate offers a unified API endpoint that connects to multiple platforms, including Zoho CRM, allowing you to build once for each use case instead of multiple times for different integrations. This can save you time and resources, enabling you to focus on your core product.
By adhering to these best practices, you can ensure a secure, efficient, and scalable integration with Zoho CRM using JavaScript. For more information on simplifying integrations, visit Endgrate.
Read More
- https://endgrate.com/provider/zohocrm
- https://www.zoho.com/crm/developer/docs/api/v3/oauth-overview.html
- https://www.zoho.com/crm/developer/docs/api/v3/scopes.html
- https://www.zoho.com/crm/developer/docs/api/v3/register-client.html
- https://www.zoho.com/crm/developer/docs/api/v3/api-limits.html
- https://www.zoho.com/crm/developer/docs/api/v3/status-codes.html
- https://www.zoho.com/crm/developer/docs/api/v3/field-meta.html
- https://www.zoho.com/crm/developer/docs/api/v3/get-records.html
Ready to get started?